Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Common / QueryCache / EntityClientCacheKey.cs / 3 / EntityClientCacheKey.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Collections.Generic; using System.Text; using System.Data.EntityClient; using System.Globalization; using System.Diagnostics; using System.Data.Metadata.Edm; ////// Represents EntityCommand Cache key context /// internal sealed class EntityClientCacheKey : QueryCacheKey { ////// Stored procedure or command text? /// readonly CommandType _commandType; ////// Entity Sql statement /// readonly string _eSqlStatement; ////// parameter collection token /// readonly string _parametersToken; ////// number of parameters /// readonly int _parameterCount; ////// Combined Hashcode based on component hashcodes /// readonly int _hashCode; ////// Creates a new instance of EntityClientCacheKey given a entityCommand instance /// /// internal EntityClientCacheKey(EntityCommand entityCommand) : base() { // Command Type _commandType = entityCommand.CommandType; // Statement _eSqlStatement = entityCommand.CommandText; // Parameters _parametersToken = GetParametersToken(entityCommand); _parameterCount = entityCommand.Parameters.Count; // Hashcode _hashCode = _commandType.GetHashCode() ^ _eSqlStatement.GetHashCode() ^ _parametersToken.GetHashCode(); } ////// determines equality of two cache keys based on cache context values /// /// ///public override bool Equals( object otherObject ) { Debug.Assert(null != otherObject, "otherObject must not be null"); if (typeof(EntityClientCacheKey) != otherObject.GetType()) { return false; } EntityClientCacheKey otherEntityClientCacheKey = (EntityClientCacheKey)otherObject; return (_commandType == otherEntityClientCacheKey._commandType && _parameterCount == otherEntityClientCacheKey._parameterCount) && Equals(otherEntityClientCacheKey._eSqlStatement, _eSqlStatement) && Equals(otherEntityClientCacheKey._parametersToken, _parametersToken); } /// /// Returns Context Hash Code /// ///public override int GetHashCode() { return _hashCode; } /// /// Returns a string representation of the parameter list /// /// ///private static string GetParametersToken(EntityCommand entityCommand) { if (null == entityCommand.Parameters || 0 == entityCommand.Parameters.Count) { // // means no parameters // return "@@0"; } else if (1 == entityCommand.Parameters.Count) { // if its one parameter only, there is no need to use stringbuilder Dictionary paramTypeUsage = entityCommand.GetParameterTypeUsage(); Debug.Assert(paramTypeUsage.Count == entityCommand.Parameters.Count, "entityParameter collection and query parameter collection must have the same number of entries"); return "@@1:" + entityCommand.Parameters[0].ParameterName + ":" + paramTypeUsage[entityCommand.Parameters[0].ParameterName].EdmType.FullName; } else { StringBuilder sb = new StringBuilder(entityCommand.Parameters.Count * EstimatedParameterStringSize); Dictionary paramTypeUsage = entityCommand.GetParameterTypeUsage(); Debug.Assert(paramTypeUsage.Count == entityCommand.Parameters.Count, "entityParameter collection and query parameter collection must have the same number of entries"); sb.Append("@@"); sb.Append(entityCommand.Parameters.Count); sb.Append(":"); string separator = ""; foreach (KeyValuePair param in paramTypeUsage) { sb.Append(separator); sb.Append(param.Key); sb.Append(":"); sb.Append(param.Value.EdmType.FullName); separator = ";"; } return sb.ToString(); } } /// /// returns the composed cache key /// ///public override string ToString() { return String.Join("|", new string[] { Enum.GetName(typeof(CommandType), _commandType), _eSqlStatement, _parametersToken }); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Collections.Generic; using System.Text; using System.Data.EntityClient; using System.Globalization; using System.Diagnostics; using System.Data.Metadata.Edm; ////// Represents EntityCommand Cache key context /// internal sealed class EntityClientCacheKey : QueryCacheKey { ////// Stored procedure or command text? /// readonly CommandType _commandType; ////// Entity Sql statement /// readonly string _eSqlStatement; ////// parameter collection token /// readonly string _parametersToken; ////// number of parameters /// readonly int _parameterCount; ////// Combined Hashcode based on component hashcodes /// readonly int _hashCode; ////// Creates a new instance of EntityClientCacheKey given a entityCommand instance /// /// internal EntityClientCacheKey(EntityCommand entityCommand) : base() { // Command Type _commandType = entityCommand.CommandType; // Statement _eSqlStatement = entityCommand.CommandText; // Parameters _parametersToken = GetParametersToken(entityCommand); _parameterCount = entityCommand.Parameters.Count; // Hashcode _hashCode = _commandType.GetHashCode() ^ _eSqlStatement.GetHashCode() ^ _parametersToken.GetHashCode(); } ////// determines equality of two cache keys based on cache context values /// /// ///public override bool Equals( object otherObject ) { Debug.Assert(null != otherObject, "otherObject must not be null"); if (typeof(EntityClientCacheKey) != otherObject.GetType()) { return false; } EntityClientCacheKey otherEntityClientCacheKey = (EntityClientCacheKey)otherObject; return (_commandType == otherEntityClientCacheKey._commandType && _parameterCount == otherEntityClientCacheKey._parameterCount) && Equals(otherEntityClientCacheKey._eSqlStatement, _eSqlStatement) && Equals(otherEntityClientCacheKey._parametersToken, _parametersToken); } /// /// Returns Context Hash Code /// ///public override int GetHashCode() { return _hashCode; } /// /// Returns a string representation of the parameter list /// /// ///private static string GetParametersToken(EntityCommand entityCommand) { if (null == entityCommand.Parameters || 0 == entityCommand.Parameters.Count) { // // means no parameters // return "@@0"; } else if (1 == entityCommand.Parameters.Count) { // if its one parameter only, there is no need to use stringbuilder Dictionary paramTypeUsage = entityCommand.GetParameterTypeUsage(); Debug.Assert(paramTypeUsage.Count == entityCommand.Parameters.Count, "entityParameter collection and query parameter collection must have the same number of entries"); return "@@1:" + entityCommand.Parameters[0].ParameterName + ":" + paramTypeUsage[entityCommand.Parameters[0].ParameterName].EdmType.FullName; } else { StringBuilder sb = new StringBuilder(entityCommand.Parameters.Count * EstimatedParameterStringSize); Dictionary paramTypeUsage = entityCommand.GetParameterTypeUsage(); Debug.Assert(paramTypeUsage.Count == entityCommand.Parameters.Count, "entityParameter collection and query parameter collection must have the same number of entries"); sb.Append("@@"); sb.Append(entityCommand.Parameters.Count); sb.Append(":"); string separator = ""; foreach (KeyValuePair param in paramTypeUsage) { sb.Append(separator); sb.Append(param.Key); sb.Append(":"); sb.Append(param.Value.EdmType.FullName); separator = ";"; } return sb.ToString(); } } /// /// returns the composed cache key /// ///public override string ToString() { return String.Join("|", new string[] { Enum.GetName(typeof(CommandType), _commandType), _eSqlStatement, _parametersToken }); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
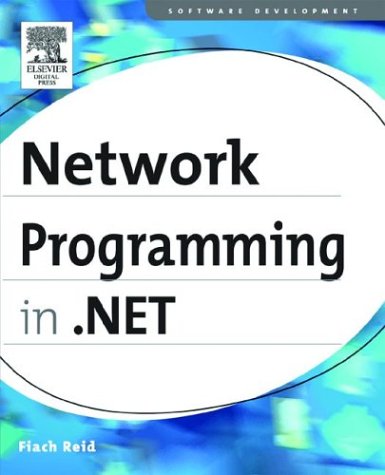
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OwnerDrawPropertyBag.cs
- InkCanvas.cs
- ExpressionPrinter.cs
- EntityClassGenerator.cs
- NeutralResourcesLanguageAttribute.cs
- x509utils.cs
- COMException.cs
- StorageComplexPropertyMapping.cs
- RenameRuleObjectDialog.Designer.cs
- ParseNumbers.cs
- PersonalizationStateInfo.cs
- _NetworkingPerfCounters.cs
- Exception.cs
- CodeExpressionCollection.cs
- BaseResourcesBuildProvider.cs
- ReaderWriterLockWrapper.cs
- WaitingCursor.cs
- HtmlInputSubmit.cs
- SponsorHelper.cs
- DispatcherObject.cs
- EntityDesignerDataSourceView.cs
- ClipboardProcessor.cs
- CurrentTimeZone.cs
- BaseTemplateParser.cs
- WebEventCodes.cs
- DataGridViewDesigner.cs
- ToolStripDropDownClosingEventArgs.cs
- MediaCommands.cs
- _BasicClient.cs
- BitmapMetadataBlob.cs
- M3DUtil.cs
- Color.cs
- SqlInternalConnectionTds.cs
- ParentUndoUnit.cs
- BidPrivateBase.cs
- XdrBuilder.cs
- JsonWriter.cs
- PropertyValueChangedEvent.cs
- ComponentResourceKey.cs
- SmiSettersStream.cs
- ElementFactory.cs
- KnownTypeAttribute.cs
- TableCellCollection.cs
- ValidatedControlConverter.cs
- GradientStopCollection.cs
- AsyncOperation.cs
- OutputCacheProfile.cs
- TextEditorCopyPaste.cs
- ServicePointManagerElement.cs
- X509Certificate2Collection.cs
- XamlSerializerUtil.cs
- SizeAnimationUsingKeyFrames.cs
- ApplicationServicesHostFactory.cs
- Constraint.cs
- SessionStateUtil.cs
- WebPartConnectVerb.cs
- WebPartDisplayModeCancelEventArgs.cs
- ImportOptions.cs
- SourceFileInfo.cs
- ArrayWithOffset.cs
- RegexBoyerMoore.cs
- SpellerStatusTable.cs
- Utils.cs
- OneOfElement.cs
- OracleDateTime.cs
- SqlTrackingQuery.cs
- ProxyGenerationError.cs
- NamespaceMapping.cs
- HtmlElement.cs
- PrtCap_Reader.cs
- BindingContext.cs
- ViewStateModeByIdAttribute.cs
- TextMetrics.cs
- SystemTcpConnection.cs
- WebPartTransformer.cs
- ViewGenResults.cs
- GlobalizationSection.cs
- Peer.cs
- ClrProviderManifest.cs
- WmlSelectionListAdapter.cs
- ToolStripComboBox.cs
- Utility.cs
- TypeInitializationException.cs
- NonClientArea.cs
- ListItem.cs
- SystemSounds.cs
- XmlReaderSettings.cs
- BinaryKeyIdentifierClause.cs
- ParallelTimeline.cs
- SystemWebSectionGroup.cs
- EmbeddedMailObjectsCollection.cs
- MemberDomainMap.cs
- ConfigurationLockCollection.cs
- PreservationFileWriter.cs
- SourceChangedEventArgs.cs
- Debug.cs
- SerializerProvider.cs
- MenuItemCollection.cs
- EntityDataSourceQueryBuilder.cs
- DataGridCell.cs