Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Security / RightsManagement / Exceptions.cs / 1 / Exceptions.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: RightsManagement exceptions // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Windows; using MS.Internal.Security.RightsManagement; namespace System.Security.RightsManagement { ////// Rights Management exception class is thrown when an RM Operation can not be completed for a variety of reasons. /// Some of the failure codes codes application, can potentially, mitigate automatically. Some failure codes can be /// mitigated by coordinated action of the user and the application. /// [Serializable()] public class RightsManagementException : Exception { ////// Creates an new instance of the RightsManagementException class. /// This constructor initializes the Message property of the new instance to a system-supplied message /// that describes the error. This message takes into account the current system culture. /// public RightsManagementException() : base(SR.Get(SRID.RmExceptionGenericMessage)) {} ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// /// The message that describes the error. public RightsManagementException(string message) : base(message) {} ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// The InnerException property is initialized using the innerException parameter. /// /// The error message that explains the reason for the exception. /// The exception that is the cause of the current exception. public RightsManagementException(string message, Exception innerException) : base(message, innerException) { } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance to a system-supplied message /// that describes the error. This message takes into account the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// /// The FailureCode that indicates specific reason for the exception. public RightsManagementException(RightsManagementFailureCode failureCode) : base(Errors.GetLocalizedFailureCodeMessageWithDefault(failureCode)) { _failureCode = failureCode; // we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// /// The FailureCode that indicates specific reason for the exception. /// The message that describes the error. public RightsManagementException(RightsManagementFailureCode failureCode, string message) : base(message) { _failureCode = failureCode;// we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance to a system-supplied message /// that describes the error. This message takes into account the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The FailureCode that indicates specific reason for the exception. /// The exception that is the cause of the current exception. public RightsManagementException(RightsManagementFailureCode failureCode, Exception innerException) : base(Errors.GetLocalizedFailureCodeMessageWithDefault(failureCode), innerException) { _failureCode = failureCode;// we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The FailureCode that indicates specific reason for the exception. /// The message that describes the error. /// The exception that is the cause of the current exception. public RightsManagementException(RightsManagementFailureCode failureCode, string message, Exception innerException) : base(message, innerException) { _failureCode = failureCode;// we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class and initializes it with serialized data. /// This constructor is called during deserialization to reconstitute the exception object transmitted over a stream. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected RightsManagementException(SerializationInfo info, StreamingContext context) : base(info, context) { _failureCode = (RightsManagementFailureCode)info.GetInt32(_serializationFailureCodeAttributeName); // we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Sets the SerializationInfo object with the Failure Code and additional exception information. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. ////// Critical: calls Exception.GetObjectData which LinkDemands /// PublicOK: a demand exists here /// [SecurityCritical] [SecurityPermissionAttribute(SecurityAction.Demand, Flags = SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue(_serializationFailureCodeAttributeName, (Int32)_failureCode); } ////// Returns the specific error code that can be used to indetify and mitigate the reason which caused the exception. /// public RightsManagementFailureCode FailureCode { get { return _failureCode; } } private RightsManagementFailureCode _failureCode; private const string _serializationFailureCodeAttributeName = "FailureCode"; } /* ////// NotActivatedException /// [Serializable()] public class NotActivatedException : RightsManagementException { ////// Initializes a new instance of the NotActivatedException class. /// public NotActivatedException() : base() {} ////// Initializes a new instance of the NotActivatedException class with a specified error message. /// /// public NotActivatedException(string message) : base(message) {} ////// Initializes a new instance of the NotActivatedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public NotActivatedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the NotActivatedException class with serialized data. /// /// /// protected NotActivatedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServiceDiscoveryException /// [Serializable()] public class ServiceDiscoveryException : RightsManagementException { ////// Initializes a new instance of the ServiceDiscoveryException class. /// public ServiceDiscoveryException() : base() {} ////// Initializes a new instance of the ServiceDiscoveryException class with a specified error message. /// /// public ServiceDiscoveryException(string message) : base(message) {} ////// Initializes a new instance of the ServiceDiscoveryException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServiceDiscoveryException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServiceDiscoveryException class with serialized data. /// /// /// protected ServiceDiscoveryException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServerConnectionFailureException /// [Serializable()] public class ServerConnectionFailureException : RightsManagementException { ////// Initializes a new instance of the ServerConnectionFailureException class. /// public ServerConnectionFailureException() : base() {} ////// Initializes a new instance of the ServerConnectionFailureException class with a specified error message. /// /// public ServerConnectionFailureException(string message) : base(message) {} ////// Initializes a new instance of the ServerConnectionFailureException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServerConnectionFailureException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServerConnectionFailureException class with serialized data. /// /// /// protected ServerConnectionFailureException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServerNotFoundException /// [Serializable()] public class ServerNotFoundException : RightsManagementException { ////// Initializes a new instance of the ServerNotFoundException class. /// public ServerNotFoundException() : base() {} ////// Initializes a new instance of the ServerNotFoundException class with a specified error message. /// /// public ServerNotFoundException(string message) : base(message) {} ////// Initializes a new instance of the ServerNotFoundException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServerNotFoundException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServerNotFoundException class with serialized data. /// /// /// protected ServerNotFoundException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidServerResponseException /// [Serializable()] public class InvalidServerResponseException : RightsManagementException { ////// Initializes a new instance of the InvalidServerResponseException class. /// public InvalidServerResponseException() : base() {} ////// Initializes a new instance of the InvalidServerResponseException class with a specified error message. /// /// public InvalidServerResponseException(string message) : base(message) {} ////// Initializes a new instance of the InvalidServerResponseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidServerResponseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidServerResponseException class with serialized data. /// /// /// protected InvalidServerResponseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ActiveDirectoryEntryNotFoundException /// [Serializable()] public class ActiveDirectoryEntryNotFoundException : RightsManagementException { ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class. /// public ActiveDirectoryEntryNotFoundException() : base() {} ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class with a specified error message. /// /// public ActiveDirectoryEntryNotFoundException(string message) : base(message) {} ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ActiveDirectoryEntryNotFoundException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class with serialized data. /// /// /// protected ActiveDirectoryEntryNotFoundException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServerErrorException /// [Serializable()] public class ServerErrorException : RightsManagementException { ////// Initializes a new instance of the ServerErrorException class. /// public ServerErrorException() : base() {} ////// Initializes a new instance of the ServerErrorException class with a specified error message. /// /// public ServerErrorException(string message) : base(message) {} ////// Initializes a new instance of the ServerErrorException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServerErrorException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServerErrorException class with serialized data. /// /// /// protected ServerErrorException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// HardwareIdCorruptionException /// [Serializable()] public class HardwareIdCorruptionException : RightsManagementException { ////// Initializes a new instance of the HardwareIdCorruptionException class. /// public HardwareIdCorruptionException() : base() {} ////// Initializes a new instance of the HardwareIdCorruptionException class with a specified error message. /// /// public HardwareIdCorruptionException(string message) : base(message) {} ////// Initializes a new instance of the HardwareIdCorruptionException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public HardwareIdCorruptionException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the HardwareIdCorruptionException class with serialized data. /// /// /// protected HardwareIdCorruptionException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InstallationFailedException /// [Serializable()] public class InstallationFailedException : RightsManagementException { ////// Initializes a new instance of the InstallationFailedException class. /// public InstallationFailedException() : base() {} ////// Initializes a new instance of the InstallationFailedException class with a specified error message. /// /// public InstallationFailedException(string message) : base(message) {} ////// Initializes a new instance of the InstallationFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InstallationFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InstallationFailedException class with serialized data. /// /// /// protected InstallationFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// AuthenticationFailedException /// [Serializable()] public class AuthenticationFailedException : RightsManagementException { ////// Initializes a new instance of the AuthenticationFailedException class. /// public AuthenticationFailedException() : base() {} ////// Initializes a new instance of the AuthenticationFailedException class with a specified error message. /// /// public AuthenticationFailedException(string message) : base(message) {} ////// Initializes a new instance of the AuthenticationFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public AuthenticationFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the AuthenticationFailedException class with serialized data. /// /// /// protected AuthenticationFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ActivationFailedException /// [Serializable()] public class ActivationFailedException : RightsManagementException { ////// Initializes a new instance of the ActivationFailedException class. /// public ActivationFailedException() : base() {} ////// Initializes a new instance of the ActivationFailedException class with a specified error message. /// /// public ActivationFailedException(string message) : base(message) {} ////// Initializes a new instance of the ActivationFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ActivationFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ActivationFailedException class with serialized data. /// /// /// protected ActivationFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// OutOfQuotaException /// [Serializable()] public class OutOfQuotaException : RightsManagementException { ////// Initializes a new instance of the OutOfQuotaException class. /// public OutOfQuotaException() : base() {} ////// Initializes a new instance of the OutOfQuotaException class with a specified error message. /// /// public OutOfQuotaException(string message) : base(message) {} ////// Initializes a new instance of the OutOfQuotaException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public OutOfQuotaException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the OutOfQuotaException class with serialized data. /// /// /// protected OutOfQuotaException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidLicenseException /// [Serializable()] public class InvalidLicenseException : RightsManagementException { ////// Initializes a new instance of the InvalidLicenseException class. /// public InvalidLicenseException() : base() {} ////// Initializes a new instance of the InvalidLicenseException class with a specified error message. /// /// public InvalidLicenseException(string message) : base(message) {} ////// Initializes a new instance of the InvalidLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidLicenseException class with serialized data. /// /// /// protected InvalidLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidLicenseSignatureException /// [Serializable()] public class InvalidLicenseSignatureException : RightsManagementException { ////// Initializes a new instance of the InvalidLicenseSignatureException class. /// public InvalidLicenseSignatureException() : base() {} ////// Initializes a new instance of the InvalidLicenseSignatureException class with a specified error message. /// /// public InvalidLicenseSignatureException(string message) : base(message) {} ////// Initializes a new instance of the InvalidLicenseSignatureException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidLicenseSignatureException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidLicenseSignatureException class with serialized data. /// /// /// protected InvalidLicenseSignatureException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// EncryptionNotPermittedException /// [Serializable()] public class EncryptionNotPermittedException : RightsManagementException { ////// Initializes a new instance of the EncryptionNotPermittedException class. /// public EncryptionNotPermittedException() : base() {} ////// Initializes a new instance of the EncryptionNotPermittedException class with a specified error message. /// /// public EncryptionNotPermittedException(string message) : base(message) {} ////// Initializes a new instance of the EncryptionNotPermittedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public EncryptionNotPermittedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the EncryptionNotPermittedException class with serialized data. /// /// /// protected EncryptionNotPermittedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// RightNotGrantedException /// [Serializable()] public class RightNotGrantedException : RightsManagementException { ////// Initializes a new instance of the RightNotGrantedException class. /// public RightNotGrantedException() : base() {} ////// Initializes a new instance of the RightNotGrantedException class with a specified error message. /// /// public RightNotGrantedException(string message) : base(message) {} ////// Initializes a new instance of the RightNotGrantedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public RightNotGrantedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the RightNotGrantedException class with serialized data. /// /// /// protected RightNotGrantedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidVersionException /// [Serializable()] public class InvalidVersionException : RightsManagementException { ////// Initializes a new instance of the InvalidVersionException class. /// public InvalidVersionException() : base() {} ////// Initializes a new instance of the InvalidVersionException class with a specified error message. /// /// public InvalidVersionException(string message) : base(message) {} ////// Initializes a new instance of the InvalidVersionException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidVersionException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidVersionException class with serialized data. /// /// /// protected InvalidVersionException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// SecurityProcessorNotLoadedException /// [Serializable()] public class SecurityProcessorNotLoadedException : RightsManagementException { ////// Initializes a new instance of the SecurityProcessorNotLoadedException class. /// public SecurityProcessorNotLoadedException() : base() {} ////// Initializes a new instance of the SecurityProcessorNotLoadedException class with a specified error message. /// /// public SecurityProcessorNotLoadedException(string message) : base(message) {} ////// Initializes a new instance of the SecurityProcessorNotLoadedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public SecurityProcessorNotLoadedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the SecurityProcessorNotLoadedException class with serialized data. /// /// /// protected SecurityProcessorNotLoadedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// SecurityProcessorAlreadyLoadedException /// [Serializable()] public class SecurityProcessorAlreadyLoadedException : RightsManagementException { ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class. /// public SecurityProcessorAlreadyLoadedException() : base() {} ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class with a specified error message. /// /// public SecurityProcessorAlreadyLoadedException(string message) : base(message) {} ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public SecurityProcessorAlreadyLoadedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class with serialized data. /// /// /// protected SecurityProcessorAlreadyLoadedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ClockRollbackDetectedException /// [Serializable()] public class ClockRollbackDetectedException : RightsManagementException { ////// Initializes a new instance of the ClockRollbackDetectedException class. /// public ClockRollbackDetectedException() : base() {} ////// Initializes a new instance of the ClockRollbackDetectedException class with a specified error message. /// /// public ClockRollbackDetectedException(string message) : base(message) {} ////// Initializes a new instance of the ClockRollbackDetectedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ClockRollbackDetectedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ClockRollbackDetectedException class with serialized data. /// /// /// protected ClockRollbackDetectedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// UnexpectedErrorException /// [Serializable()] public class UnexpectedErrorException : RightsManagementException { ////// Initializes a new instance of the UnexpectedErrorException class. /// public UnexpectedErrorException() : base() {} ////// Initializes a new instance of the UnexpectedErrorException class with a specified error message. /// /// public UnexpectedErrorException(string message) : base(message) {} ////// Initializes a new instance of the UnexpectedErrorException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public UnexpectedErrorException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the UnexpectedErrorException class with serialized data. /// /// /// protected UnexpectedErrorException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ValidityTimeViolatedException /// [Serializable()] public class ValidityTimeViolatedException : RightsManagementException { ////// Initializes a new instance of the ValidityTimeViolatedException class. /// public ValidityTimeViolatedException() : base() {} ////// Initializes a new instance of the ValidityTimeViolatedException class with a specified error message. /// /// public ValidityTimeViolatedException(string message) : base(message) {} ////// Initializes a new instance of the ValidityTimeViolatedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ValidityTimeViolatedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ValidityTimeViolatedException class with serialized data. /// /// /// protected ValidityTimeViolatedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// BrokenCertChainException /// [Serializable()] public class BrokenCertChainException : RightsManagementException { ////// Initializes a new instance of the BrokenCertChainException class. /// public BrokenCertChainException() : base() {} ////// Initializes a new instance of the BrokenCertChainException class with a specified error message. /// /// public BrokenCertChainException(string message) : base(message) {} ////// Initializes a new instance of the BrokenCertChainException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public BrokenCertChainException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the BrokenCertChainException class with serialized data. /// /// /// protected BrokenCertChainException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// PolicyViolationException /// [Serializable()] public class PolicyViolationException : RightsManagementException { ////// Initializes a new instance of the PolicyViolationException class. /// public PolicyViolationException() : base() {} ////// Initializes a new instance of the PolicyViolationException class with a specified error message. /// /// public PolicyViolationException(string message) : base(message) {} ////// Initializes a new instance of the PolicyViolationException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public PolicyViolationException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the PolicyViolationException class with serialized data. /// /// /// protected PolicyViolationException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// RevokedLicenseException /// [Serializable()] public class RevokedLicenseException : RightsManagementException { ////// Initializes a new instance of the RevokedLicenseException class. /// public RevokedLicenseException() : base() {} ////// Initializes a new instance of the RevokedLicenseException class with a specified error message. /// /// public RevokedLicenseException(string message) : base(message) {} ////// Initializes a new instance of the RevokedLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public RevokedLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the RevokedLicenseException class with serialized data. /// /// /// protected RevokedLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ContentNotInUseLicenseException /// [Serializable()] public class ContentNotInUseLicenseException : RightsManagementException { ////// Initializes a new instance of the ContentNotInUseLicenseException class. /// public ContentNotInUseLicenseException() : base() {} ////// Initializes a new instance of the ContentNotInUseLicenseException class with a specified error message. /// /// public ContentNotInUseLicenseException(string message) : base(message) {} ////// Initializes a new instance of the ContentNotInUseLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ContentNotInUseLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ContentNotInUseLicenseException class with serialized data. /// /// /// protected ContentNotInUseLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// IndicatedPrincipalMissingException /// [Serializable()] public class IndicatedPrincipalMissingException : RightsManagementException { ////// Initializes a new instance of the IndicatedPrincipalMissingException class. /// public IndicatedPrincipalMissingException() : base() {} ////// Initializes a new instance of the IndicatedPrincipalMissingException class with a specified error message. /// /// public IndicatedPrincipalMissingException(string message) : base(message) {} ////// Initializes a new instance of the IndicatedPrincipalMissingException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public IndicatedPrincipalMissingException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the IndicatedPrincipalMissingException class with serialized data. /// /// /// protected IndicatedPrincipalMissingException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// MachineNotFoundInGroupIdentityException /// [Serializable()] public class MachineNotFoundInGroupIdentityException : RightsManagementException { ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class. /// public MachineNotFoundInGroupIdentityException() : base() {} ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class with a specified error message. /// /// public MachineNotFoundInGroupIdentityException(string message) : base(message) {} ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public MachineNotFoundInGroupIdentityException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class with serialized data. /// /// /// protected MachineNotFoundInGroupIdentityException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// IntervalTimeViolatedException /// [Serializable()] public class IntervalTimeViolatedException : RightsManagementException { ////// Initializes a new instance of the IntervalTimeViolatedException class. /// public IntervalTimeViolatedException() : base() {} ////// Initializes a new instance of the IntervalTimeViolatedException class with a specified error message. /// /// public IntervalTimeViolatedException(string message) : base(message) {} ////// Initializes a new instance of the IntervalTimeViolatedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public IntervalTimeViolatedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the IntervalTimeViolatedException class with serialized data. /// /// /// protected IntervalTimeViolatedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidHardwareIdException /// [Serializable()] public class InvalidHardwareIdException : RightsManagementException { ////// Initializes a new instance of the InvalidHardwareIdException class. /// public InvalidHardwareIdException() : base() {} ////// Initializes a new instance of the InvalidHardwareIdException class with a specified error message. /// /// public InvalidHardwareIdException(string message) : base(message) {} ////// Initializes a new instance of the InvalidHardwareIdException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidHardwareIdException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidHardwareIdException class with serialized data. /// /// /// protected InvalidHardwareIdException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// DebuggerDetectedException /// [Serializable()] public class DebuggerDetectedException : RightsManagementException { ////// Initializes a new instance of the DebuggerDetectedException class. /// public DebuggerDetectedException() : base() {} ////// Initializes a new instance of the DebuggerDetectedException class with a specified error message. /// /// public DebuggerDetectedException(string message) : base(message) {} ////// Initializes a new instance of the DebuggerDetectedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public DebuggerDetectedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the DebuggerDetectedException class with serialized data. /// /// /// protected DebuggerDetectedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// EmailNotVerifiedException /// [Serializable()] public class EmailNotVerifiedException : RightsManagementException { ////// Initializes a new instance of the EmailNotVerifiedException class. /// public EmailNotVerifiedException() : base() {} ////// Initializes a new instance of the EmailNotVerifiedException class with a specified error message. /// /// public EmailNotVerifiedException(string message) : base(message) {} ////// Initializes a new instance of the EmailNotVerifiedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public EmailNotVerifiedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the EmailNotVerifiedException class with serialized data. /// /// /// protected EmailNotVerifiedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// NotCertifiedException /// [Serializable()] public class NotCertifiedException : RightsManagementException { ////// Initializes a new instance of the NotCertifiedException class. /// public NotCertifiedException() : base() {} ////// Initializes a new instance of the NotCertifiedException class with a specified error message. /// /// public NotCertifiedException(string message) : base(message) {} ////// Initializes a new instance of the NotCertifiedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public NotCertifiedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the NotCertifiedException class with serialized data. /// /// /// protected NotCertifiedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidUnsignedPublishLicenseException /// [Serializable()] public class InvalidUnsignedPublishLicenseException : RightsManagementException { ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class. /// public InvalidUnsignedPublishLicenseException() : base() {} ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class with a specified error message. /// /// public InvalidUnsignedPublishLicenseException(string message) : base(message) {} ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidUnsignedPublishLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class with serialized data. /// /// /// protected InvalidUnsignedPublishLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidContentKeyLengthException /// [Serializable()] public class InvalidContentKeyLengthException : RightsManagementException { ////// Initializes a new instance of the InvalidContentKeyLengthException class. /// public InvalidContentKeyLengthException() : base() {} ////// Initializes a new instance of the InvalidContentKeyLengthException class with a specified error message. /// /// public InvalidContentKeyLengthException(string message) : base(message) {} ////// Initializes a new instance of the InvalidContentKeyLengthException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidContentKeyLengthException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidContentKeyLengthException class with serialized data. /// /// /// protected InvalidContentKeyLengthException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ExpiredPublishLicenseException /// [Serializable()] public class ExpiredPublishLicenseException : RightsManagementException { ////// Initializes a new instance of the ExpiredPublishLicenseException class. /// public ExpiredPublishLicenseException() : base() {} ////// Initializes a new instance of the ExpiredPublishLicenseException class with a specified error message. /// /// public ExpiredPublishLicenseException(string message) : base(message) {} ////// Initializes a new instance of the ExpiredPublishLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ExpiredPublishLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ExpiredPublishLicenseException class with serialized data. /// /// /// protected ExpiredPublishLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// UseLicenseAcquisitionFailedException /// [Serializable()] public class UseLicenseAcquisitionFailedException : RightsManagementException { ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class. /// public UseLicenseAcquisitionFailedException() : base() {} ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class with a specified error message. /// /// public UseLicenseAcquisitionFailedException(string message) : base(message) {} ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public UseLicenseAcquisitionFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class with serialized data. /// /// /// protected UseLicenseAcquisitionFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// IncompatiblePublishLicenseException /// [Serializable()] public class IncompatiblePublishLicenseException : RightsManagementException { ////// Initializes a new instance of the IncompatiblePublishLicenseException class. /// public IncompatiblePublishLicenseException() : base() {} ////// Initializes a new instance of the IncompatiblePublishLicenseException class with a specified error message. /// /// public IncompatiblePublishLicenseException(string message) : base(message) {} ////// Initializes a new instance of the IncompatiblePublishLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public IncompatiblePublishLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the IncompatiblePublishLicenseException class with serialized data. /// /// /// protected IncompatiblePublishLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } */ } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: RightsManagement exceptions // // History: // 06/01/2005: IgorBel : Initial Implementation // //----------------------------------------------------------------------------- using System; using System.Runtime.Serialization; using System.Security; using System.Security.Permissions; using System.Windows; using MS.Internal.Security.RightsManagement; namespace System.Security.RightsManagement { ////// Rights Management exception class is thrown when an RM Operation can not be completed for a variety of reasons. /// Some of the failure codes codes application, can potentially, mitigate automatically. Some failure codes can be /// mitigated by coordinated action of the user and the application. /// [Serializable()] public class RightsManagementException : Exception { ////// Creates an new instance of the RightsManagementException class. /// This constructor initializes the Message property of the new instance to a system-supplied message /// that describes the error. This message takes into account the current system culture. /// public RightsManagementException() : base(SR.Get(SRID.RmExceptionGenericMessage)) {} ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// /// The message that describes the error. public RightsManagementException(string message) : base(message) {} ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance with a specified error message. /// The InnerException property is initialized using the innerException parameter. /// /// The error message that explains the reason for the exception. /// The exception that is the cause of the current exception. public RightsManagementException(string message, Exception innerException) : base(message, innerException) { } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance to a system-supplied message /// that describes the error. This message takes into account the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// /// The FailureCode that indicates specific reason for the exception. public RightsManagementException(RightsManagementFailureCode failureCode) : base(Errors.GetLocalizedFailureCodeMessageWithDefault(failureCode)) { _failureCode = failureCode; // we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// /// The FailureCode that indicates specific reason for the exception. /// The message that describes the error. public RightsManagementException(RightsManagementFailureCode failureCode, string message) : base(message) { _failureCode = failureCode;// we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance to a system-supplied message /// that describes the error. This message takes into account the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The FailureCode that indicates specific reason for the exception. /// The exception that is the cause of the current exception. public RightsManagementException(RightsManagementFailureCode failureCode, Exception innerException) : base(Errors.GetLocalizedFailureCodeMessageWithDefault(failureCode), innerException) { _failureCode = failureCode;// we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class. /// This constructor initializes the Message property of the new instance using the message parameter. /// The content of message is intended to be understood by humans. /// The caller of this constructor is required to ensure that this string has been localized for the current system culture. /// The FailureCode property is initialized using the failureCode parameter. /// The InnerException property is initialized using the innerException parameter. /// /// The FailureCode that indicates specific reason for the exception. /// The message that describes the error. /// The exception that is the cause of the current exception. public RightsManagementException(RightsManagementFailureCode failureCode, string message, Exception innerException) : base(message, innerException) { _failureCode = failureCode;// we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Creates a new instance of RightsManagementException class and initializes it with serialized data. /// This constructor is called during deserialization to reconstitute the exception object transmitted over a stream. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. protected RightsManagementException(SerializationInfo info, StreamingContext context) : base(info, context) { _failureCode = (RightsManagementFailureCode)info.GetInt32(_serializationFailureCodeAttributeName); // we do not check the validity of the failureCode range , as it might contain a generic // HR code, not covered by the RightsManagementFailureCode enumeration } ////// Sets the SerializationInfo object with the Failure Code and additional exception information. /// /// The object that holds the serialized object data. /// The contextual information about the source or destination. ////// Critical: calls Exception.GetObjectData which LinkDemands /// PublicOK: a demand exists here /// [SecurityCritical] [SecurityPermissionAttribute(SecurityAction.Demand, Flags = SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info == null) throw new ArgumentNullException("info"); base.GetObjectData(info, context); info.AddValue(_serializationFailureCodeAttributeName, (Int32)_failureCode); } ////// Returns the specific error code that can be used to indetify and mitigate the reason which caused the exception. /// public RightsManagementFailureCode FailureCode { get { return _failureCode; } } private RightsManagementFailureCode _failureCode; private const string _serializationFailureCodeAttributeName = "FailureCode"; } /* ////// NotActivatedException /// [Serializable()] public class NotActivatedException : RightsManagementException { ////// Initializes a new instance of the NotActivatedException class. /// public NotActivatedException() : base() {} ////// Initializes a new instance of the NotActivatedException class with a specified error message. /// /// public NotActivatedException(string message) : base(message) {} ////// Initializes a new instance of the NotActivatedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public NotActivatedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the NotActivatedException class with serialized data. /// /// /// protected NotActivatedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServiceDiscoveryException /// [Serializable()] public class ServiceDiscoveryException : RightsManagementException { ////// Initializes a new instance of the ServiceDiscoveryException class. /// public ServiceDiscoveryException() : base() {} ////// Initializes a new instance of the ServiceDiscoveryException class with a specified error message. /// /// public ServiceDiscoveryException(string message) : base(message) {} ////// Initializes a new instance of the ServiceDiscoveryException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServiceDiscoveryException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServiceDiscoveryException class with serialized data. /// /// /// protected ServiceDiscoveryException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServerConnectionFailureException /// [Serializable()] public class ServerConnectionFailureException : RightsManagementException { ////// Initializes a new instance of the ServerConnectionFailureException class. /// public ServerConnectionFailureException() : base() {} ////// Initializes a new instance of the ServerConnectionFailureException class with a specified error message. /// /// public ServerConnectionFailureException(string message) : base(message) {} ////// Initializes a new instance of the ServerConnectionFailureException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServerConnectionFailureException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServerConnectionFailureException class with serialized data. /// /// /// protected ServerConnectionFailureException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServerNotFoundException /// [Serializable()] public class ServerNotFoundException : RightsManagementException { ////// Initializes a new instance of the ServerNotFoundException class. /// public ServerNotFoundException() : base() {} ////// Initializes a new instance of the ServerNotFoundException class with a specified error message. /// /// public ServerNotFoundException(string message) : base(message) {} ////// Initializes a new instance of the ServerNotFoundException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServerNotFoundException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServerNotFoundException class with serialized data. /// /// /// protected ServerNotFoundException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidServerResponseException /// [Serializable()] public class InvalidServerResponseException : RightsManagementException { ////// Initializes a new instance of the InvalidServerResponseException class. /// public InvalidServerResponseException() : base() {} ////// Initializes a new instance of the InvalidServerResponseException class with a specified error message. /// /// public InvalidServerResponseException(string message) : base(message) {} ////// Initializes a new instance of the InvalidServerResponseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidServerResponseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidServerResponseException class with serialized data. /// /// /// protected InvalidServerResponseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ActiveDirectoryEntryNotFoundException /// [Serializable()] public class ActiveDirectoryEntryNotFoundException : RightsManagementException { ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class. /// public ActiveDirectoryEntryNotFoundException() : base() {} ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class with a specified error message. /// /// public ActiveDirectoryEntryNotFoundException(string message) : base(message) {} ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ActiveDirectoryEntryNotFoundException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ActiveDirectoryEntryNotFoundException class with serialized data. /// /// /// protected ActiveDirectoryEntryNotFoundException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ServerErrorException /// [Serializable()] public class ServerErrorException : RightsManagementException { ////// Initializes a new instance of the ServerErrorException class. /// public ServerErrorException() : base() {} ////// Initializes a new instance of the ServerErrorException class with a specified error message. /// /// public ServerErrorException(string message) : base(message) {} ////// Initializes a new instance of the ServerErrorException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ServerErrorException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ServerErrorException class with serialized data. /// /// /// protected ServerErrorException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// HardwareIdCorruptionException /// [Serializable()] public class HardwareIdCorruptionException : RightsManagementException { ////// Initializes a new instance of the HardwareIdCorruptionException class. /// public HardwareIdCorruptionException() : base() {} ////// Initializes a new instance of the HardwareIdCorruptionException class with a specified error message. /// /// public HardwareIdCorruptionException(string message) : base(message) {} ////// Initializes a new instance of the HardwareIdCorruptionException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public HardwareIdCorruptionException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the HardwareIdCorruptionException class with serialized data. /// /// /// protected HardwareIdCorruptionException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InstallationFailedException /// [Serializable()] public class InstallationFailedException : RightsManagementException { ////// Initializes a new instance of the InstallationFailedException class. /// public InstallationFailedException() : base() {} ////// Initializes a new instance of the InstallationFailedException class with a specified error message. /// /// public InstallationFailedException(string message) : base(message) {} ////// Initializes a new instance of the InstallationFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InstallationFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InstallationFailedException class with serialized data. /// /// /// protected InstallationFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// AuthenticationFailedException /// [Serializable()] public class AuthenticationFailedException : RightsManagementException { ////// Initializes a new instance of the AuthenticationFailedException class. /// public AuthenticationFailedException() : base() {} ////// Initializes a new instance of the AuthenticationFailedException class with a specified error message. /// /// public AuthenticationFailedException(string message) : base(message) {} ////// Initializes a new instance of the AuthenticationFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public AuthenticationFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the AuthenticationFailedException class with serialized data. /// /// /// protected AuthenticationFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ActivationFailedException /// [Serializable()] public class ActivationFailedException : RightsManagementException { ////// Initializes a new instance of the ActivationFailedException class. /// public ActivationFailedException() : base() {} ////// Initializes a new instance of the ActivationFailedException class with a specified error message. /// /// public ActivationFailedException(string message) : base(message) {} ////// Initializes a new instance of the ActivationFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ActivationFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ActivationFailedException class with serialized data. /// /// /// protected ActivationFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// OutOfQuotaException /// [Serializable()] public class OutOfQuotaException : RightsManagementException { ////// Initializes a new instance of the OutOfQuotaException class. /// public OutOfQuotaException() : base() {} ////// Initializes a new instance of the OutOfQuotaException class with a specified error message. /// /// public OutOfQuotaException(string message) : base(message) {} ////// Initializes a new instance of the OutOfQuotaException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public OutOfQuotaException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the OutOfQuotaException class with serialized data. /// /// /// protected OutOfQuotaException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidLicenseException /// [Serializable()] public class InvalidLicenseException : RightsManagementException { ////// Initializes a new instance of the InvalidLicenseException class. /// public InvalidLicenseException() : base() {} ////// Initializes a new instance of the InvalidLicenseException class with a specified error message. /// /// public InvalidLicenseException(string message) : base(message) {} ////// Initializes a new instance of the InvalidLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidLicenseException class with serialized data. /// /// /// protected InvalidLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidLicenseSignatureException /// [Serializable()] public class InvalidLicenseSignatureException : RightsManagementException { ////// Initializes a new instance of the InvalidLicenseSignatureException class. /// public InvalidLicenseSignatureException() : base() {} ////// Initializes a new instance of the InvalidLicenseSignatureException class with a specified error message. /// /// public InvalidLicenseSignatureException(string message) : base(message) {} ////// Initializes a new instance of the InvalidLicenseSignatureException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidLicenseSignatureException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidLicenseSignatureException class with serialized data. /// /// /// protected InvalidLicenseSignatureException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// EncryptionNotPermittedException /// [Serializable()] public class EncryptionNotPermittedException : RightsManagementException { ////// Initializes a new instance of the EncryptionNotPermittedException class. /// public EncryptionNotPermittedException() : base() {} ////// Initializes a new instance of the EncryptionNotPermittedException class with a specified error message. /// /// public EncryptionNotPermittedException(string message) : base(message) {} ////// Initializes a new instance of the EncryptionNotPermittedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public EncryptionNotPermittedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the EncryptionNotPermittedException class with serialized data. /// /// /// protected EncryptionNotPermittedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// RightNotGrantedException /// [Serializable()] public class RightNotGrantedException : RightsManagementException { ////// Initializes a new instance of the RightNotGrantedException class. /// public RightNotGrantedException() : base() {} ////// Initializes a new instance of the RightNotGrantedException class with a specified error message. /// /// public RightNotGrantedException(string message) : base(message) {} ////// Initializes a new instance of the RightNotGrantedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public RightNotGrantedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the RightNotGrantedException class with serialized data. /// /// /// protected RightNotGrantedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidVersionException /// [Serializable()] public class InvalidVersionException : RightsManagementException { ////// Initializes a new instance of the InvalidVersionException class. /// public InvalidVersionException() : base() {} ////// Initializes a new instance of the InvalidVersionException class with a specified error message. /// /// public InvalidVersionException(string message) : base(message) {} ////// Initializes a new instance of the InvalidVersionException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidVersionException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidVersionException class with serialized data. /// /// /// protected InvalidVersionException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// SecurityProcessorNotLoadedException /// [Serializable()] public class SecurityProcessorNotLoadedException : RightsManagementException { ////// Initializes a new instance of the SecurityProcessorNotLoadedException class. /// public SecurityProcessorNotLoadedException() : base() {} ////// Initializes a new instance of the SecurityProcessorNotLoadedException class with a specified error message. /// /// public SecurityProcessorNotLoadedException(string message) : base(message) {} ////// Initializes a new instance of the SecurityProcessorNotLoadedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public SecurityProcessorNotLoadedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the SecurityProcessorNotLoadedException class with serialized data. /// /// /// protected SecurityProcessorNotLoadedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// SecurityProcessorAlreadyLoadedException /// [Serializable()] public class SecurityProcessorAlreadyLoadedException : RightsManagementException { ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class. /// public SecurityProcessorAlreadyLoadedException() : base() {} ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class with a specified error message. /// /// public SecurityProcessorAlreadyLoadedException(string message) : base(message) {} ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public SecurityProcessorAlreadyLoadedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the SecurityProcessorAlreadyLoadedException class with serialized data. /// /// /// protected SecurityProcessorAlreadyLoadedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ClockRollbackDetectedException /// [Serializable()] public class ClockRollbackDetectedException : RightsManagementException { ////// Initializes a new instance of the ClockRollbackDetectedException class. /// public ClockRollbackDetectedException() : base() {} ////// Initializes a new instance of the ClockRollbackDetectedException class with a specified error message. /// /// public ClockRollbackDetectedException(string message) : base(message) {} ////// Initializes a new instance of the ClockRollbackDetectedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ClockRollbackDetectedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ClockRollbackDetectedException class with serialized data. /// /// /// protected ClockRollbackDetectedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// UnexpectedErrorException /// [Serializable()] public class UnexpectedErrorException : RightsManagementException { ////// Initializes a new instance of the UnexpectedErrorException class. /// public UnexpectedErrorException() : base() {} ////// Initializes a new instance of the UnexpectedErrorException class with a specified error message. /// /// public UnexpectedErrorException(string message) : base(message) {} ////// Initializes a new instance of the UnexpectedErrorException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public UnexpectedErrorException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the UnexpectedErrorException class with serialized data. /// /// /// protected UnexpectedErrorException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ValidityTimeViolatedException /// [Serializable()] public class ValidityTimeViolatedException : RightsManagementException { ////// Initializes a new instance of the ValidityTimeViolatedException class. /// public ValidityTimeViolatedException() : base() {} ////// Initializes a new instance of the ValidityTimeViolatedException class with a specified error message. /// /// public ValidityTimeViolatedException(string message) : base(message) {} ////// Initializes a new instance of the ValidityTimeViolatedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ValidityTimeViolatedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ValidityTimeViolatedException class with serialized data. /// /// /// protected ValidityTimeViolatedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// BrokenCertChainException /// [Serializable()] public class BrokenCertChainException : RightsManagementException { ////// Initializes a new instance of the BrokenCertChainException class. /// public BrokenCertChainException() : base() {} ////// Initializes a new instance of the BrokenCertChainException class with a specified error message. /// /// public BrokenCertChainException(string message) : base(message) {} ////// Initializes a new instance of the BrokenCertChainException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public BrokenCertChainException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the BrokenCertChainException class with serialized data. /// /// /// protected BrokenCertChainException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// PolicyViolationException /// [Serializable()] public class PolicyViolationException : RightsManagementException { ////// Initializes a new instance of the PolicyViolationException class. /// public PolicyViolationException() : base() {} ////// Initializes a new instance of the PolicyViolationException class with a specified error message. /// /// public PolicyViolationException(string message) : base(message) {} ////// Initializes a new instance of the PolicyViolationException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public PolicyViolationException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the PolicyViolationException class with serialized data. /// /// /// protected PolicyViolationException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// RevokedLicenseException /// [Serializable()] public class RevokedLicenseException : RightsManagementException { ////// Initializes a new instance of the RevokedLicenseException class. /// public RevokedLicenseException() : base() {} ////// Initializes a new instance of the RevokedLicenseException class with a specified error message. /// /// public RevokedLicenseException(string message) : base(message) {} ////// Initializes a new instance of the RevokedLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public RevokedLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the RevokedLicenseException class with serialized data. /// /// /// protected RevokedLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ContentNotInUseLicenseException /// [Serializable()] public class ContentNotInUseLicenseException : RightsManagementException { ////// Initializes a new instance of the ContentNotInUseLicenseException class. /// public ContentNotInUseLicenseException() : base() {} ////// Initializes a new instance of the ContentNotInUseLicenseException class with a specified error message. /// /// public ContentNotInUseLicenseException(string message) : base(message) {} ////// Initializes a new instance of the ContentNotInUseLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ContentNotInUseLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ContentNotInUseLicenseException class with serialized data. /// /// /// protected ContentNotInUseLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// IndicatedPrincipalMissingException /// [Serializable()] public class IndicatedPrincipalMissingException : RightsManagementException { ////// Initializes a new instance of the IndicatedPrincipalMissingException class. /// public IndicatedPrincipalMissingException() : base() {} ////// Initializes a new instance of the IndicatedPrincipalMissingException class with a specified error message. /// /// public IndicatedPrincipalMissingException(string message) : base(message) {} ////// Initializes a new instance of the IndicatedPrincipalMissingException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public IndicatedPrincipalMissingException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the IndicatedPrincipalMissingException class with serialized data. /// /// /// protected IndicatedPrincipalMissingException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// MachineNotFoundInGroupIdentityException /// [Serializable()] public class MachineNotFoundInGroupIdentityException : RightsManagementException { ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class. /// public MachineNotFoundInGroupIdentityException() : base() {} ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class with a specified error message. /// /// public MachineNotFoundInGroupIdentityException(string message) : base(message) {} ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public MachineNotFoundInGroupIdentityException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the MachineNotFoundInGroupIdentityException class with serialized data. /// /// /// protected MachineNotFoundInGroupIdentityException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// IntervalTimeViolatedException /// [Serializable()] public class IntervalTimeViolatedException : RightsManagementException { ////// Initializes a new instance of the IntervalTimeViolatedException class. /// public IntervalTimeViolatedException() : base() {} ////// Initializes a new instance of the IntervalTimeViolatedException class with a specified error message. /// /// public IntervalTimeViolatedException(string message) : base(message) {} ////// Initializes a new instance of the IntervalTimeViolatedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public IntervalTimeViolatedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the IntervalTimeViolatedException class with serialized data. /// /// /// protected IntervalTimeViolatedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidHardwareIdException /// [Serializable()] public class InvalidHardwareIdException : RightsManagementException { ////// Initializes a new instance of the InvalidHardwareIdException class. /// public InvalidHardwareIdException() : base() {} ////// Initializes a new instance of the InvalidHardwareIdException class with a specified error message. /// /// public InvalidHardwareIdException(string message) : base(message) {} ////// Initializes a new instance of the InvalidHardwareIdException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidHardwareIdException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidHardwareIdException class with serialized data. /// /// /// protected InvalidHardwareIdException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// DebuggerDetectedException /// [Serializable()] public class DebuggerDetectedException : RightsManagementException { ////// Initializes a new instance of the DebuggerDetectedException class. /// public DebuggerDetectedException() : base() {} ////// Initializes a new instance of the DebuggerDetectedException class with a specified error message. /// /// public DebuggerDetectedException(string message) : base(message) {} ////// Initializes a new instance of the DebuggerDetectedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public DebuggerDetectedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the DebuggerDetectedException class with serialized data. /// /// /// protected DebuggerDetectedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// EmailNotVerifiedException /// [Serializable()] public class EmailNotVerifiedException : RightsManagementException { ////// Initializes a new instance of the EmailNotVerifiedException class. /// public EmailNotVerifiedException() : base() {} ////// Initializes a new instance of the EmailNotVerifiedException class with a specified error message. /// /// public EmailNotVerifiedException(string message) : base(message) {} ////// Initializes a new instance of the EmailNotVerifiedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public EmailNotVerifiedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the EmailNotVerifiedException class with serialized data. /// /// /// protected EmailNotVerifiedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// NotCertifiedException /// [Serializable()] public class NotCertifiedException : RightsManagementException { ////// Initializes a new instance of the NotCertifiedException class. /// public NotCertifiedException() : base() {} ////// Initializes a new instance of the NotCertifiedException class with a specified error message. /// /// public NotCertifiedException(string message) : base(message) {} ////// Initializes a new instance of the NotCertifiedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public NotCertifiedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the NotCertifiedException class with serialized data. /// /// /// protected NotCertifiedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidUnsignedPublishLicenseException /// [Serializable()] public class InvalidUnsignedPublishLicenseException : RightsManagementException { ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class. /// public InvalidUnsignedPublishLicenseException() : base() {} ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class with a specified error message. /// /// public InvalidUnsignedPublishLicenseException(string message) : base(message) {} ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidUnsignedPublishLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidUnsignedPublishLicenseException class with serialized data. /// /// /// protected InvalidUnsignedPublishLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// InvalidContentKeyLengthException /// [Serializable()] public class InvalidContentKeyLengthException : RightsManagementException { ////// Initializes a new instance of the InvalidContentKeyLengthException class. /// public InvalidContentKeyLengthException() : base() {} ////// Initializes a new instance of the InvalidContentKeyLengthException class with a specified error message. /// /// public InvalidContentKeyLengthException(string message) : base(message) {} ////// Initializes a new instance of the InvalidContentKeyLengthException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public InvalidContentKeyLengthException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the InvalidContentKeyLengthException class with serialized data. /// /// /// protected InvalidContentKeyLengthException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// ExpiredPublishLicenseException /// [Serializable()] public class ExpiredPublishLicenseException : RightsManagementException { ////// Initializes a new instance of the ExpiredPublishLicenseException class. /// public ExpiredPublishLicenseException() : base() {} ////// Initializes a new instance of the ExpiredPublishLicenseException class with a specified error message. /// /// public ExpiredPublishLicenseException(string message) : base(message) {} ////// Initializes a new instance of the ExpiredPublishLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public ExpiredPublishLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the ExpiredPublishLicenseException class with serialized data. /// /// /// protected ExpiredPublishLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// UseLicenseAcquisitionFailedException /// [Serializable()] public class UseLicenseAcquisitionFailedException : RightsManagementException { ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class. /// public UseLicenseAcquisitionFailedException() : base() {} ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class with a specified error message. /// /// public UseLicenseAcquisitionFailedException(string message) : base(message) {} ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public UseLicenseAcquisitionFailedException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the UseLicenseAcquisitionFailedException class with serialized data. /// /// /// protected UseLicenseAcquisitionFailedException(SerializationInfo info, StreamingContext context) : base(info, context) {} } ////// IncompatiblePublishLicenseException /// [Serializable()] public class IncompatiblePublishLicenseException : RightsManagementException { ////// Initializes a new instance of the IncompatiblePublishLicenseException class. /// public IncompatiblePublishLicenseException() : base() {} ////// Initializes a new instance of the IncompatiblePublishLicenseException class with a specified error message. /// /// public IncompatiblePublishLicenseException(string message) : base(message) {} ////// Initializes a new instance of the IncompatiblePublishLicenseException class with a specified error message and a reference to /// the inner exception that is the cause of this exception. /// /// /// public IncompatiblePublishLicenseException(string message, Exception innerException) : base(message, innerException) { } ////// Initializes a new instance of the IncompatiblePublishLicenseException class with serialized data. /// /// /// protected IncompatiblePublishLicenseException(SerializationInfo info, StreamingContext context) : base(info, context) {} } */ } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
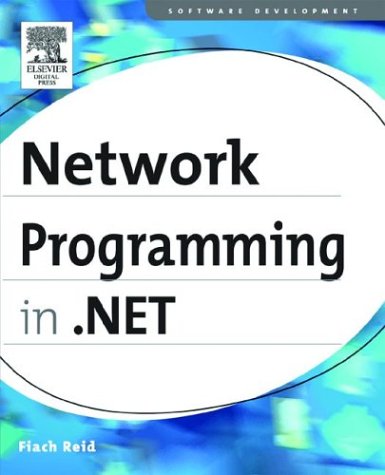
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BasicHttpBindingElement.cs
- MetadataPropertyCollection.cs
- AxisAngleRotation3D.cs
- SinglePageViewer.cs
- ChameleonKey.cs
- DesignerTransaction.cs
- WSHttpBinding.cs
- RegistrySecurity.cs
- ThreadAbortException.cs
- DeflateStream.cs
- StringValueSerializer.cs
- ThemeableAttribute.cs
- UITypeEditors.cs
- Splitter.cs
- TriggerActionCollection.cs
- IntSecurity.cs
- GeometryModel3D.cs
- SecurityPolicySection.cs
- SystemIcons.cs
- TypedTableHandler.cs
- WorkflowServiceOperationListItem.cs
- LookupBindingPropertiesAttribute.cs
- AttributeUsageAttribute.cs
- PseudoWebRequest.cs
- TextStore.cs
- EventTrigger.cs
- _SslStream.cs
- XPathNavigator.cs
- HttpHandlerAction.cs
- MDIClient.cs
- MenuItemStyleCollection.cs
- Visual.cs
- ImageFormatConverter.cs
- FixedDocumentSequencePaginator.cs
- ChangeProcessor.cs
- HttpWebResponse.cs
- StateBag.cs
- PersonalizationDictionary.cs
- HebrewCalendar.cs
- ServiceDeploymentInfo.cs
- CounterCreationData.cs
- ConfigsHelper.cs
- GetLastErrorDetailsRequest.cs
- XmlSerializerNamespaces.cs
- FixUp.cs
- MergablePropertyAttribute.cs
- MarkupCompiler.cs
- ResumeStoryboard.cs
- DesignerActionUIService.cs
- ShaderRenderModeValidation.cs
- SafeFileMappingHandle.cs
- RSAPKCS1SignatureDeformatter.cs
- AttributeAction.cs
- TargetException.cs
- XpsFixedDocumentReaderWriter.cs
- Types.cs
- TypeConstant.cs
- SupportsEventValidationAttribute.cs
- RequestCacheEntry.cs
- BitmapDecoder.cs
- CapabilitiesSection.cs
- SettingsSection.cs
- NamespaceExpr.cs
- webclient.cs
- HttpContextServiceHost.cs
- CultureInfoConverter.cs
- FaultBookmark.cs
- Util.cs
- QueryValue.cs
- DiagnosticsConfigurationHandler.cs
- LongValidatorAttribute.cs
- CompositionTarget.cs
- DataGridViewCellPaintingEventArgs.cs
- MouseButton.cs
- MembershipSection.cs
- StylusShape.cs
- ColorMap.cs
- CodeIdentifiers.cs
- PeerName.cs
- ComponentCollection.cs
- DocumentPageViewAutomationPeer.cs
- TypePropertyEditor.cs
- RenderingEventArgs.cs
- TextSelectionProcessor.cs
- EditableLabelControl.cs
- RequestUriProcessor.cs
- CardSpaceShim.cs
- EntityDesignerBuildProvider.cs
- CompareValidator.cs
- __ConsoleStream.cs
- DetailsViewPageEventArgs.cs
- RoleManagerEventArgs.cs
- LocalizationParserHooks.cs
- RichTextBox.cs
- CurrencyWrapper.cs
- ExpressionServices.cs
- ArgumentNullException.cs
- AnnouncementClient.cs
- PageSetupDialog.cs
- DataGridAutoFormat.cs