Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / MS / Internal / Shaping / GlyphingCache.cs / 1 / GlyphingCache.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: High level glyphing cache // // History: 5-19-2005 garyyang created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; using MS.Internal.TextFormatting; namespace MS.Internal.Shaping { ////// GlyphingCache stores the runtime state of shaping engines and the mapping between Unicode scalar /// value and the physical font being used to display it. This class is not thread-safe. The client is /// responsible for synchronizing access from multiple threads. It is generally recommended that the client /// manages a single instance of this class per text formatting session. /// /// It currently only caches one key-value pair: /// o Typeface - TypefaceMap /// /// This pair is cached in SizeLimitedCache which implement LRU algorithm through /// a linked list. When cache is full, the least used entry in the cache will be replaced /// by the latest entry. /// internal class GlyphingCache { private SizeLimitedCache_sizeLimitedCache; private ShapingEngineManager _shapeManager; internal GlyphingCache(int capacity) { _sizeLimitedCache = new SizeLimitedCache (capacity); _shapeManager = new ShapingEngineManager(); } internal void GetShapeableText( Typeface typeface, CharacterBufferReference characterBufferReference, int stringLength, TextRunProperties textRunProperties, CultureInfo digitCulture, bool rightToLeft, IList shapeableList, IShapeableTextCollector collector ) { if (!typeface.Symbol) { Lookup(typeface).GetShapeableText( characterBufferReference, stringLength, textRunProperties, digitCulture, rightToLeft, shapeableList, collector ); } else { // It's a non-Unicode ("symbol") font, where code points have non-standard meanings. We // therefore want to bypass the usual itemization and font linking. Instead, just map // everything to the default script and first GlyphTypeface. ShapeTypeface shapeTypeface = new ShapeTypeface( _shapeManager, typeface.TryGetGlyphTypeface(), null // device font ); collector.Add( shapeableList, new CharacterBufferRange(characterBufferReference, stringLength), textRunProperties, new Item(ScriptID.Default, ItemFlags.Default), shapeTypeface, 1.0, // scale in Em false // null shape ); } } /// /// Look up the font mapping data for a typeface. /// private TypefaceMap Lookup(Typeface key) { TypefaceMap typefaceMap = _sizeLimitedCache.Get(key); if (typefaceMap == null) { typefaceMap = new TypefaceMap( key.FontFamily, key.FallbackFontFamily, key.CanonicalStyle, key.CanonicalWeight, key.CanonicalStretch, key.NullFont, _shapeManager ); _sizeLimitedCache.Add( key, typefaceMap, false // is not permanent in the cache. ); } return typefaceMap; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: High level glyphing cache // // History: 5-19-2005 garyyang created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Globalization; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal; using MS.Internal.TextFormatting; namespace MS.Internal.Shaping { ////// GlyphingCache stores the runtime state of shaping engines and the mapping between Unicode scalar /// value and the physical font being used to display it. This class is not thread-safe. The client is /// responsible for synchronizing access from multiple threads. It is generally recommended that the client /// manages a single instance of this class per text formatting session. /// /// It currently only caches one key-value pair: /// o Typeface - TypefaceMap /// /// This pair is cached in SizeLimitedCache which implement LRU algorithm through /// a linked list. When cache is full, the least used entry in the cache will be replaced /// by the latest entry. /// internal class GlyphingCache { private SizeLimitedCache_sizeLimitedCache; private ShapingEngineManager _shapeManager; internal GlyphingCache(int capacity) { _sizeLimitedCache = new SizeLimitedCache (capacity); _shapeManager = new ShapingEngineManager(); } internal void GetShapeableText( Typeface typeface, CharacterBufferReference characterBufferReference, int stringLength, TextRunProperties textRunProperties, CultureInfo digitCulture, bool rightToLeft, IList shapeableList, IShapeableTextCollector collector ) { if (!typeface.Symbol) { Lookup(typeface).GetShapeableText( characterBufferReference, stringLength, textRunProperties, digitCulture, rightToLeft, shapeableList, collector ); } else { // It's a non-Unicode ("symbol") font, where code points have non-standard meanings. We // therefore want to bypass the usual itemization and font linking. Instead, just map // everything to the default script and first GlyphTypeface. ShapeTypeface shapeTypeface = new ShapeTypeface( _shapeManager, typeface.TryGetGlyphTypeface(), null // device font ); collector.Add( shapeableList, new CharacterBufferRange(characterBufferReference, stringLength), textRunProperties, new Item(ScriptID.Default, ItemFlags.Default), shapeTypeface, 1.0, // scale in Em false // null shape ); } } /// /// Look up the font mapping data for a typeface. /// private TypefaceMap Lookup(Typeface key) { TypefaceMap typefaceMap = _sizeLimitedCache.Get(key); if (typefaceMap == null) { typefaceMap = new TypefaceMap( key.FontFamily, key.FallbackFontFamily, key.CanonicalStyle, key.CanonicalWeight, key.CanonicalStretch, key.NullFont, _shapeManager ); _sizeLimitedCache.Add( key, typefaceMap, false // is not permanent in the cache. ); } return typefaceMap; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
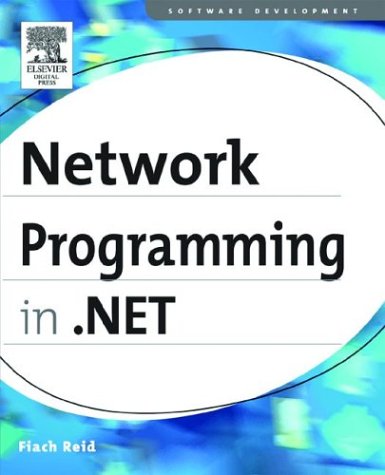
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputLanguageProfileNotifySink.cs
- PerspectiveCamera.cs
- BindingManagerDataErrorEventArgs.cs
- RotateTransform3D.cs
- UdpDiscoveryEndpointProvider.cs
- LongValidatorAttribute.cs
- StringArrayEditor.cs
- DbSetClause.cs
- XPathDocumentIterator.cs
- DynamicResourceExtensionConverter.cs
- XmlSchemaImport.cs
- NestPullup.cs
- BoolExpression.cs
- Point.cs
- CollectionConverter.cs
- QilSortKey.cs
- NativeRightsManagementAPIsStructures.cs
- HttpHandlerActionCollection.cs
- CookieHandler.cs
- RawStylusInputReport.cs
- VectorCollection.cs
- MobileListItemCollection.cs
- MultiTrigger.cs
- BamlTreeUpdater.cs
- ManagementBaseObject.cs
- ScrollData.cs
- Clipboard.cs
- AsymmetricSignatureFormatter.cs
- SystemIcons.cs
- XmlTextReaderImpl.cs
- CalloutQueueItem.cs
- XPathSelfQuery.cs
- DataGridTablesFactory.cs
- TerminateWorkflow.cs
- HMACSHA256.cs
- PrintPageEvent.cs
- DataControlFieldCollection.cs
- DescriptionAttribute.cs
- NegotiateStream.cs
- BitmapCodecInfoInternal.cs
- XslAstAnalyzer.cs
- HighlightVisual.cs
- HttpWebResponse.cs
- UxThemeWrapper.cs
- OpacityConverter.cs
- DbConnectionPoolOptions.cs
- UnsafeNativeMethods.cs
- BridgeDataRecord.cs
- JumpPath.cs
- webclient.cs
- StyleSheetRefUrlEditor.cs
- WebPartZoneCollection.cs
- Slider.cs
- SequenceNumber.cs
- RSAOAEPKeyExchangeFormatter.cs
- Point3DValueSerializer.cs
- TemplateControlBuildProvider.cs
- MenuEventArgs.cs
- InputLanguageProfileNotifySink.cs
- WSHttpBinding.cs
- NullRuntimeConfig.cs
- FileSecurity.cs
- FrameworkName.cs
- ClockController.cs
- QuadraticBezierSegment.cs
- HandleRef.cs
- UIPropertyMetadata.cs
- LineBreakRecord.cs
- WindowsScroll.cs
- SafeNativeMethodsCLR.cs
- PasswordRecovery.cs
- SqlCharStream.cs
- NativeRecognizer.cs
- DesignerAdapterUtil.cs
- RichTextBoxConstants.cs
- SynchronizingStream.cs
- WhitespaceRule.cs
- StatusBarPanel.cs
- Matrix3DValueSerializer.cs
- ImageSource.cs
- BitArray.cs
- ContentPathSegment.cs
- GlyphShapingProperties.cs
- CommentAction.cs
- ConcurrencyBehavior.cs
- TextEditorTyping.cs
- CodeAttributeDeclaration.cs
- CompModSwitches.cs
- X509Extension.cs
- HtmlAnchor.cs
- Authorization.cs
- CodeTypeOfExpression.cs
- DataViewSettingCollection.cs
- CannotUnloadAppDomainException.cs
- MouseButtonEventArgs.cs
- ConfigurationManagerInternalFactory.cs
- SerializerProvider.cs
- DynamicDataRoute.cs
- SqlUDTStorage.cs
- DataSourceView.cs