Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / InterOp / HwndAppCommandInputProvider.cs / 1 / HwndAppCommandInputProvider.cs
using System.Windows.Input; using System.Windows.Media; using System.Windows.Threading; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using MS.Utility; using MS.Internal; using MS.Win32; namespace System.Windows.Interop { internal sealed class HwndAppCommandInputProvider : DispatcherObject, IInputProvider, IDisposable { ////// Accesses and store critical data. This class is also critical (_site and _source) /// [SecurityCritical] internal HwndAppCommandInputProvider( HwndSource source ) { (new UIPermission(PermissionState.Unrestricted)).Assert(); try { _site = new SecurityCriticalDataClass(InputManager.Current.RegisterInputProvider(this)); } finally { UIPermission.RevertAssert(); } _source = new SecurityCriticalDataClass (source); } /// /// Critical:This class accesses critical data, _site. /// TreatAsSafe: This class does not expose the critical data /// [SecurityCritical, SecurityTreatAsSafe] public void Dispose( ) { if (_site != null) { _site.Value.Dispose(); _site = null; } _source = null; } ////// Critical: As this accesses critical data HwndSource /// TreatAsSafe:Information about whether a given input provider services /// a visual is safe to expose. This method does not expose the critical data either. /// [SecurityCritical, SecurityTreatAsSafe] bool IInputProvider.ProvidesInputForRootVisual( Visual v ) { Debug.Assert(null != _source); return _source.Value.RootVisual == v; } void IInputProvider.NotifyDeactivate() {} ////// Critical: As this accesses critical data HwndSource /// [SecurityCritical] internal IntPtr FilterMessage( IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled ) { // It is possible to be re-entered during disposal. Just return. if(null == _source || null == _source.Value) { return IntPtr.Zero; } if (msg == NativeMethods.WM_APPCOMMAND) { // WM_APPCOMMAND message notifies a window that the user generated an application command event, // for example, by clicking an application command button using the mouse or typing an application command // key on the keyboard. RawAppCommandInputReport report = new RawAppCommandInputReport( _source.Value, InputMode.Foreground, SafeNativeMethods.GetMessageTime(), GetAppCommand(lParam), GetDevice(lParam), InputType.Command); handled = _site.Value.ReportInput(report); } return handled ? new IntPtr(1) : IntPtr.Zero ; } ////// Implementation of the GET_APPCOMMAND_LPARAM macro defined in Winuser.h /// /// ///private static int GetAppCommand( IntPtr lParam ) { return ((short)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & ~NativeMethods.FAPPCOMMAND_MASK)); } /// /// Returns the input device that originated this app command. /// InputType.Hid represents an unspecified device that is neither the /// keyboard nor the mouse. /// /// ///private static InputType GetDevice(IntPtr lParam) { InputType inputType = InputType.Hid; // Implementation of the GET_DEVICE_LPARAM macro defined in Winuser.h // Returns either FAPPCOMMAND_KEY (the user pressed a key), FAPPCOMMAND_MOUSE // (the user clicked a mouse button) or FAPPCOMMAND_OEM (unknown device) ushort deviceId = (ushort)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & NativeMethods.FAPPCOMMAND_MASK); switch (deviceId) { case NativeMethods.FAPPCOMMAND_MOUSE: inputType = InputType.Mouse; break; case NativeMethods.FAPPCOMMAND_KEY: inputType = InputType.Keyboard; break; case NativeMethods.FAPPCOMMAND_OEM: default: // Unknown device id or FAPPCOMMAND_OEM. // In either cases we set it to the generic human interface device. inputType=InputType.Hid; break; } return inputType; } /// /// This is got under an elevation and is hence critical. This data is not ok to expose. /// private SecurityCriticalDataClass_source; /// /// This is got under an elevation and is hence critical.This data is not ok to expose. /// private SecurityCriticalDataClass_site; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Windows.Input; using System.Windows.Media; using System.Windows.Threading; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using MS.Utility; using MS.Internal; using MS.Win32; namespace System.Windows.Interop { internal sealed class HwndAppCommandInputProvider : DispatcherObject, IInputProvider, IDisposable { /// /// Accesses and store critical data. This class is also critical (_site and _source) /// [SecurityCritical] internal HwndAppCommandInputProvider( HwndSource source ) { (new UIPermission(PermissionState.Unrestricted)).Assert(); try { _site = new SecurityCriticalDataClass(InputManager.Current.RegisterInputProvider(this)); } finally { UIPermission.RevertAssert(); } _source = new SecurityCriticalDataClass (source); } /// /// Critical:This class accesses critical data, _site. /// TreatAsSafe: This class does not expose the critical data /// [SecurityCritical, SecurityTreatAsSafe] public void Dispose( ) { if (_site != null) { _site.Value.Dispose(); _site = null; } _source = null; } ////// Critical: As this accesses critical data HwndSource /// TreatAsSafe:Information about whether a given input provider services /// a visual is safe to expose. This method does not expose the critical data either. /// [SecurityCritical, SecurityTreatAsSafe] bool IInputProvider.ProvidesInputForRootVisual( Visual v ) { Debug.Assert(null != _source); return _source.Value.RootVisual == v; } void IInputProvider.NotifyDeactivate() {} ////// Critical: As this accesses critical data HwndSource /// [SecurityCritical] internal IntPtr FilterMessage( IntPtr hwnd, int msg, IntPtr wParam, IntPtr lParam, ref bool handled ) { // It is possible to be re-entered during disposal. Just return. if(null == _source || null == _source.Value) { return IntPtr.Zero; } if (msg == NativeMethods.WM_APPCOMMAND) { // WM_APPCOMMAND message notifies a window that the user generated an application command event, // for example, by clicking an application command button using the mouse or typing an application command // key on the keyboard. RawAppCommandInputReport report = new RawAppCommandInputReport( _source.Value, InputMode.Foreground, SafeNativeMethods.GetMessageTime(), GetAppCommand(lParam), GetDevice(lParam), InputType.Command); handled = _site.Value.ReportInput(report); } return handled ? new IntPtr(1) : IntPtr.Zero ; } ////// Implementation of the GET_APPCOMMAND_LPARAM macro defined in Winuser.h /// /// ///private static int GetAppCommand( IntPtr lParam ) { return ((short)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & ~NativeMethods.FAPPCOMMAND_MASK)); } /// /// Returns the input device that originated this app command. /// InputType.Hid represents an unspecified device that is neither the /// keyboard nor the mouse. /// /// ///private static InputType GetDevice(IntPtr lParam) { InputType inputType = InputType.Hid; // Implementation of the GET_DEVICE_LPARAM macro defined in Winuser.h // Returns either FAPPCOMMAND_KEY (the user pressed a key), FAPPCOMMAND_MOUSE // (the user clicked a mouse button) or FAPPCOMMAND_OEM (unknown device) ushort deviceId = (ushort)(NativeMethods.SignedHIWORD(NativeMethods.IntPtrToInt32(lParam)) & NativeMethods.FAPPCOMMAND_MASK); switch (deviceId) { case NativeMethods.FAPPCOMMAND_MOUSE: inputType = InputType.Mouse; break; case NativeMethods.FAPPCOMMAND_KEY: inputType = InputType.Keyboard; break; case NativeMethods.FAPPCOMMAND_OEM: default: // Unknown device id or FAPPCOMMAND_OEM. // In either cases we set it to the generic human interface device. inputType=InputType.Hid; break; } return inputType; } /// /// This is got under an elevation and is hence critical. This data is not ok to expose. /// private SecurityCriticalDataClass_source; /// /// This is got under an elevation and is hence critical.This data is not ok to expose. /// private SecurityCriticalDataClass_site; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
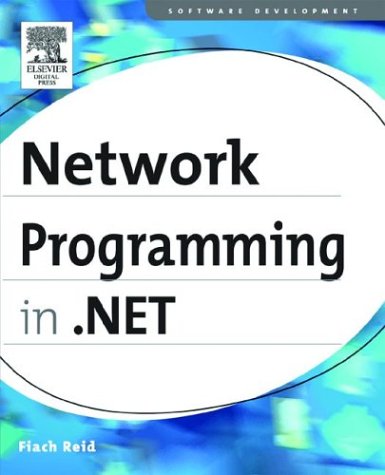
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataKey.cs
- TextRangeBase.cs
- DefaultAsyncDataDispatcher.cs
- WebPartUtil.cs
- DataTemplate.cs
- QueryOpeningEnumerator.cs
- WindowsGraphics.cs
- WebConfigurationHost.cs
- EntityDataSourceColumn.cs
- DocumentOrderQuery.cs
- IPGlobalProperties.cs
- ClientRoleProvider.cs
- PngBitmapEncoder.cs
- NativeActivityAbortContext.cs
- autovalidator.cs
- XmlDesigner.cs
- ButtonStandardAdapter.cs
- BamlMapTable.cs
- KeyValuePair.cs
- SqlDataSourceSelectingEventArgs.cs
- OperationFormatUse.cs
- HttpChannelBindingToken.cs
- Rectangle.cs
- RuntimeResourceSet.cs
- SolidColorBrush.cs
- DeploymentSection.cs
- baseshape.cs
- SolidColorBrush.cs
- SolidBrush.cs
- ScriptingSectionGroup.cs
- Math.cs
- IPAddressCollection.cs
- HttpConfigurationContext.cs
- SymDocumentType.cs
- IResourceProvider.cs
- Int32RectValueSerializer.cs
- OdbcEnvironment.cs
- DatatypeImplementation.cs
- MSHTMLHost.cs
- DataBinder.cs
- WeakReference.cs
- SchemaTableOptionalColumn.cs
- RowUpdatingEventArgs.cs
- UnknownBitmapDecoder.cs
- TableLayoutStyleCollection.cs
- StrokeCollectionDefaultValueFactory.cs
- LicenseException.cs
- DataGrid.cs
- DataGridViewControlCollection.cs
- BevelBitmapEffect.cs
- IpcChannelHelper.cs
- InternalConfigHost.cs
- DescendantQuery.cs
- AudioFileOut.cs
- ExtendedProtectionPolicy.cs
- ProviderSettingsCollection.cs
- Soap.cs
- XmlQueryType.cs
- MDIWindowDialog.cs
- RealizationDrawingContextWalker.cs
- IResourceProvider.cs
- ToolStripOverflowButton.cs
- MDIControlStrip.cs
- sqlinternaltransaction.cs
- DataGridViewComboBoxCell.cs
- SchemaDeclBase.cs
- KeyInterop.cs
- PageAdapter.cs
- StylusButtonEventArgs.cs
- ObjectConverter.cs
- ServiceReference.cs
- BoundPropertyEntry.cs
- CodeSnippetExpression.cs
- GridView.cs
- MonthCalendar.cs
- CodeDirectionExpression.cs
- TextEditorLists.cs
- IPAddress.cs
- EditorPartCollection.cs
- FieldBuilder.cs
- InitializingNewItemEventArgs.cs
- InputScope.cs
- Privilege.cs
- FSWPathEditor.cs
- ChangePassword.cs
- CodeEntryPointMethod.cs
- RefreshPropertiesAttribute.cs
- GeneralTransform2DTo3D.cs
- GroupBoxRenderer.cs
- TileBrush.cs
- httpapplicationstate.cs
- ContainerVisual.cs
- FixedPage.cs
- DateBoldEvent.cs
- DefaultSerializationProviderAttribute.cs
- DeflateStream.cs
- XsltLibrary.cs
- DataGridColumnReorderingEventArgs.cs
- AsymmetricKeyExchangeDeformatter.cs
- ProcessModelInfo.cs