Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / BezierSegment.cs / 1 / BezierSegment.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: BezierSegment.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using MS.Internal.PresentationCore; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Composition; using System.Windows.Media.Animation; using System.Diagnostics; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// BezierSegment /// public sealed partial class BezierSegment : PathSegment { #region Constructors ////// /// public BezierSegment() : base() { } ////// /// public BezierSegment(Point point1, Point point2, Point point3, bool isStroked) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; } // Internal constructor supporting smooth joins between segments internal BezierSegment(Point point1, Point point2, Point point3, bool isStroked, bool isSmoothJoin) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; IsSmoothJoin = isSmoothJoin; } #endregion #region AddToFigure internal override void AddToFigure( Matrix matrix, // The transformation matrid PathFigure figure, // The figure to add to ref Point current) // Out: Segment endpoint, not transformed { current = Point3; if (matrix.IsIdentity) { figure.Segments.Add(this); } else { Point pt1 = Point1; pt1 *= matrix; Point pt2 = Point2; pt2 *= matrix; Point pt3 = current; pt3 *= matrix; figure.Segments.Add(new BezierSegment(pt1, pt2, pt3, IsStroked, IsSmoothJoin)); } } #endregion #region Resource ////// SerializeData - Serialize the contents of this Segment to the provided context. /// internal override void SerializeData(StreamGeometryContext ctx) { ctx.BezierTo(Point1, Point2, Point3, IsStroked, IsSmoothJoin); } internal override bool IsCurved() { return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "C{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}", separator, Point1, Point2, Point3 ); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2001 // // File: BezierSegment.cs //----------------------------------------------------------------------------- using System; using MS.Internal; using MS.Internal.PresentationCore; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Text.RegularExpressions; using System.Windows.Media.Composition; using System.Windows.Media.Animation; using System.Diagnostics; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// BezierSegment /// public sealed partial class BezierSegment : PathSegment { #region Constructors ////// /// public BezierSegment() : base() { } ////// /// public BezierSegment(Point point1, Point point2, Point point3, bool isStroked) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; } // Internal constructor supporting smooth joins between segments internal BezierSegment(Point point1, Point point2, Point point3, bool isStroked, bool isSmoothJoin) { Point1 = point1; Point2 = point2; Point3 = point3; IsStroked = isStroked; IsSmoothJoin = isSmoothJoin; } #endregion #region AddToFigure internal override void AddToFigure( Matrix matrix, // The transformation matrid PathFigure figure, // The figure to add to ref Point current) // Out: Segment endpoint, not transformed { current = Point3; if (matrix.IsIdentity) { figure.Segments.Add(this); } else { Point pt1 = Point1; pt1 *= matrix; Point pt2 = Point2; pt2 *= matrix; Point pt3 = current; pt3 *= matrix; figure.Segments.Add(new BezierSegment(pt1, pt2, pt3, IsStroked, IsSmoothJoin)); } } #endregion #region Resource ////// SerializeData - Serialize the contents of this Segment to the provided context. /// internal override void SerializeData(StreamGeometryContext ctx) { ctx.BezierTo(Point1, Point2, Point3, IsStroked, IsSmoothJoin); } internal override bool IsCurved() { return true; } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// internal override string ConvertToString(string format, IFormatProvider provider) { // Helper to get the numeric list separator for a given culture. char separator = MS.Internal.TokenizerHelper.GetNumericListSeparator(provider); return String.Format(provider, "C{1:" + format + "}{0}{2:" + format + "}{0}{3:" + format + "}", separator, Point1, Point2, Point3 ); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
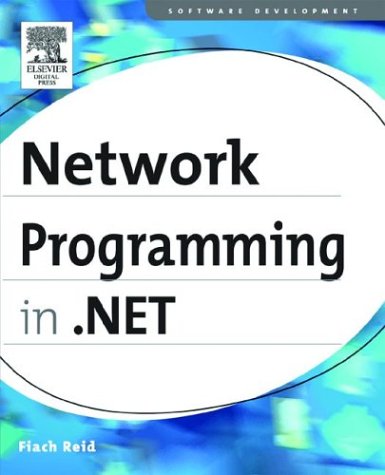
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- METAHEADER.cs
- Facet.cs
- Decoder.cs
- DelegatingStream.cs
- DataGridToolTip.cs
- StreamWithDictionary.cs
- GeneralTransform3DGroup.cs
- Nodes.cs
- TextElementCollection.cs
- Mappings.cs
- StatusBarPanelClickEvent.cs
- SqlGatherProducedAliases.cs
- ImageAnimator.cs
- BitmapCodecInfoInternal.cs
- TypeExtensionConverter.cs
- XsltException.cs
- NavigationWindowAutomationPeer.cs
- TempFiles.cs
- COSERVERINFO.cs
- AssemblyCollection.cs
- DictionaryGlobals.cs
- MiniMapControl.xaml.cs
- OracleDataAdapter.cs
- HttpCacheVaryByContentEncodings.cs
- SmiSettersStream.cs
- SHA512.cs
- ApplicationSecurityManager.cs
- SchemaSetCompiler.cs
- ExpressionPrefixAttribute.cs
- TypefaceMetricsCache.cs
- ToolboxItemCollection.cs
- SettingsBindableAttribute.cs
- EditorBrowsableAttribute.cs
- StringUtil.cs
- ExtendedPropertyCollection.cs
- KeyboardEventArgs.cs
- OAVariantLib.cs
- ResXResourceSet.cs
- MetadataSource.cs
- LoginUtil.cs
- SafePEFileHandle.cs
- InputScope.cs
- CommandLibraryHelper.cs
- DependencyObject.cs
- SqlCacheDependency.cs
- ClientBuildManagerCallback.cs
- DiscreteKeyFrames.cs
- PixelFormats.cs
- DateTimeHelper.cs
- QueryOperationResponseOfT.cs
- SingleObjectCollection.cs
- SchemaInfo.cs
- SerialPinChanges.cs
- DataControlImageButton.cs
- CodeDOMUtility.cs
- AppModelKnownContentFactory.cs
- CacheForPrimitiveTypes.cs
- TableLayoutPanelCellPosition.cs
- MethodToken.cs
- SelectionPattern.cs
- DBDataPermissionAttribute.cs
- InertiaExpansionBehavior.cs
- ToolbarAUtomationPeer.cs
- RenderContext.cs
- DataGridColumnStyleMappingNameEditor.cs
- CellParagraph.cs
- FontUnit.cs
- ObjectHandle.cs
- StrokeNodeOperations2.cs
- DBConnection.cs
- ColumnReorderedEventArgs.cs
- SemaphoreFullException.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- MembershipUser.cs
- ReadWriteObjectLock.cs
- Rectangle.cs
- XamlSerializerUtil.cs
- ObjectIDGenerator.cs
- DispatcherFrame.cs
- EncryptedPackage.cs
- Message.cs
- FactoryMaker.cs
- __ComObject.cs
- MDIWindowDialog.cs
- DataGridViewSelectedCellCollection.cs
- CollectionBase.cs
- DataControlFieldCollection.cs
- processwaithandle.cs
- ParserStack.cs
- WmlValidationSummaryAdapter.cs
- FtpWebRequest.cs
- StandardOleMarshalObject.cs
- RelOps.cs
- XPathSingletonIterator.cs
- Automation.cs
- ResourceSetExpression.cs
- CompilerScopeManager.cs
- StylusDownEventArgs.cs
- AdRotatorDesigner.cs
- SemanticTag.cs