Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Configuration / System / Configuration / ProtectedConfigurationSection.cs / 1 / ProtectedConfigurationSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Xml; using System.Globalization; using System.Security.Permissions; public sealed class ProtectedConfigurationSection : ConfigurationSection { internal ProtectedConfigurationProvider GetProviderFromName(string providerName) { ProviderSettings ps = Providers[providerName]; if (ps == null) { throw new Exception(SR.GetString(SR.ProtectedConfigurationProvider_not_found, providerName)); } return InstantiateProvider(ps); } internal ProtectedConfigurationProviderCollection GetAllProviders() { ProtectedConfigurationProviderCollection coll = new ProtectedConfigurationProviderCollection(); foreach(ProviderSettings ps in Providers) { coll.Add(InstantiateProvider(ps)); } return coll; } [PermissionSet(SecurityAction.Assert, Unrestricted=true)] private ProtectedConfigurationProvider CreateAndInitializeProviderWithAssert(Type t, ProviderSettings pn) { ProtectedConfigurationProvider provider = (ProtectedConfigurationProvider)TypeUtil.CreateInstanceWithReflectionPermission(t); NameValueCollection pars = pn.Parameters; NameValueCollection cloneParams = new NameValueCollection(pars.Count); foreach (string key in pars) { cloneParams[key] = pars[key]; } provider.Initialize(pn.Name, cloneParams); return provider; } private ProtectedConfigurationProvider InstantiateProvider(ProviderSettings pn) { Type t = TypeUtil.GetTypeWithReflectionPermission(pn.Type, true); if (!typeof(ProtectedConfigurationProvider).IsAssignableFrom(t)) { throw new Exception(SR.GetString(SR.WrongType_of_Protected_provider)); } // Needs to check APTCA bit. See VSWhidbey 429996. if (!TypeUtil.IsTypeAllowedInConfig(t)) { throw new Exception(SR.GetString(SR.Type_from_untrusted_assembly, t.FullName)); } // Needs to check Assert Fulltrust in order for runtime to work. See VSWhidbey 429996. return CreateAndInitializeProviderWithAssert(t, pn); } internal static string DecryptSection(string encryptedXml, ProtectedConfigurationProvider provider) { XmlDocument doc = new XmlDocument(); doc.LoadXml(encryptedXml); XmlNode resultNode = provider.Decrypt(doc.DocumentElement); return resultNode.OuterXml; } private const string EncryptedSectionTemplate = "<{0} {1}=\"{2}\"> {3} {0}>"; internal static string FormatEncryptedSection(string encryptedXml, string sectionName, string providerName) { return String.Format(CultureInfo.InvariantCulture, EncryptedSectionTemplate, sectionName, // The section to encrypt BaseConfigurationRecord.KEYWORD_PROTECTION_PROVIDER, // protectionProvider keyword providerName, // The provider name encryptedXml // the encrypted xml ); } internal static string EncryptSection(string clearXml, ProtectedConfigurationProvider provider) { XmlDocument xmlDocument = new XmlDocument(); xmlDocument.PreserveWhitespace = true; xmlDocument.LoadXml(clearXml); string sectionName = xmlDocument.DocumentElement.Name; XmlNode encNode = provider.Encrypt(xmlDocument.DocumentElement); return encNode.OuterXml; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProtectedProviderSettings), new ProtectedProviderSettings(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "RsaProtectedConfigurationProvider", null, ConfigurationProperty.NonEmptyStringValidator, ConfigurationPropertyOptions.None); static ProtectedConfigurationSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propProviders); _properties.Add(_propDefaultProvider); } public ProtectedConfigurationSection() { } protected internal override ConfigurationPropertyCollection Properties { get { return _properties; } } private ProtectedProviderSettings _Providers { get { return (ProtectedProviderSettings)base[_propProviders]; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return _Providers.Providers; } } [ConfigurationProperty("defaultProvider", DefaultValue = "RsaProtectedConfigurationProvider")] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Xml; using System.Globalization; using System.Security.Permissions; public sealed class ProtectedConfigurationSection : ConfigurationSection { internal ProtectedConfigurationProvider GetProviderFromName(string providerName) { ProviderSettings ps = Providers[providerName]; if (ps == null) { throw new Exception(SR.GetString(SR.ProtectedConfigurationProvider_not_found, providerName)); } return InstantiateProvider(ps); } internal ProtectedConfigurationProviderCollection GetAllProviders() { ProtectedConfigurationProviderCollection coll = new ProtectedConfigurationProviderCollection(); foreach(ProviderSettings ps in Providers) { coll.Add(InstantiateProvider(ps)); } return coll; } [PermissionSet(SecurityAction.Assert, Unrestricted=true)] private ProtectedConfigurationProvider CreateAndInitializeProviderWithAssert(Type t, ProviderSettings pn) { ProtectedConfigurationProvider provider = (ProtectedConfigurationProvider)TypeUtil.CreateInstanceWithReflectionPermission(t); NameValueCollection pars = pn.Parameters; NameValueCollection cloneParams = new NameValueCollection(pars.Count); foreach (string key in pars) { cloneParams[key] = pars[key]; } provider.Initialize(pn.Name, cloneParams); return provider; } private ProtectedConfigurationProvider InstantiateProvider(ProviderSettings pn) { Type t = TypeUtil.GetTypeWithReflectionPermission(pn.Type, true); if (!typeof(ProtectedConfigurationProvider).IsAssignableFrom(t)) { throw new Exception(SR.GetString(SR.WrongType_of_Protected_provider)); } // Needs to check APTCA bit. See VSWhidbey 429996. if (!TypeUtil.IsTypeAllowedInConfig(t)) { throw new Exception(SR.GetString(SR.Type_from_untrusted_assembly, t.FullName)); } // Needs to check Assert Fulltrust in order for runtime to work. See VSWhidbey 429996. return CreateAndInitializeProviderWithAssert(t, pn); } internal static string DecryptSection(string encryptedXml, ProtectedConfigurationProvider provider) { XmlDocument doc = new XmlDocument(); doc.LoadXml(encryptedXml); XmlNode resultNode = provider.Decrypt(doc.DocumentElement); return resultNode.OuterXml; } private const string EncryptedSectionTemplate = "<{0} {1}=\"{2}\"> {3} {0}>"; internal static string FormatEncryptedSection(string encryptedXml, string sectionName, string providerName) { return String.Format(CultureInfo.InvariantCulture, EncryptedSectionTemplate, sectionName, // The section to encrypt BaseConfigurationRecord.KEYWORD_PROTECTION_PROVIDER, // protectionProvider keyword providerName, // The provider name encryptedXml // the encrypted xml ); } internal static string EncryptSection(string clearXml, ProtectedConfigurationProvider provider) { XmlDocument xmlDocument = new XmlDocument(); xmlDocument.PreserveWhitespace = true; xmlDocument.LoadXml(clearXml); string sectionName = xmlDocument.DocumentElement.Name; XmlNode encNode = provider.Encrypt(xmlDocument.DocumentElement); return encNode.OuterXml; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProtectedProviderSettings), new ProtectedProviderSettings(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "RsaProtectedConfigurationProvider", null, ConfigurationProperty.NonEmptyStringValidator, ConfigurationPropertyOptions.None); static ProtectedConfigurationSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propProviders); _properties.Add(_propDefaultProvider); } public ProtectedConfigurationSection() { } protected internal override ConfigurationPropertyCollection Properties { get { return _properties; } } private ProtectedProviderSettings _Providers { get { return (ProtectedProviderSettings)base[_propProviders]; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return _Providers.Providers; } } [ConfigurationProperty("defaultProvider", DefaultValue = "RsaProtectedConfigurationProvider")] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
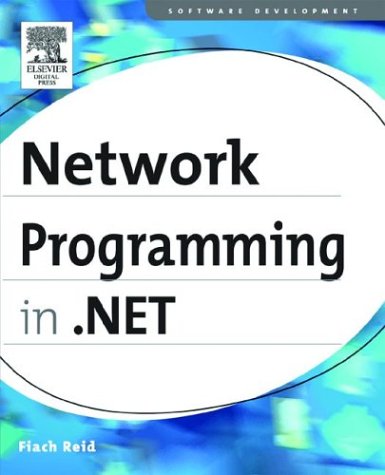
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeArgumentReferenceExpression.cs
- DropShadowEffect.cs
- SemanticAnalyzer.cs
- InitializationEventAttribute.cs
- ConfigurationException.cs
- PaginationProgressEventArgs.cs
- IgnoreSectionHandler.cs
- DrawingState.cs
- XmlObjectSerializerWriteContextComplex.cs
- WebServiceMethodData.cs
- CallbackHandler.cs
- EqualityComparer.cs
- NullRuntimeConfig.cs
- UIElement3D.cs
- UnsafeNativeMethods.cs
- AttributeSetAction.cs
- DataGridViewCellConverter.cs
- SafeCertificateStore.cs
- ColumnProvider.cs
- RangeContentEnumerator.cs
- DataBinder.cs
- SiteMapNodeItem.cs
- x509utils.cs
- PerformanceCounterCategory.cs
- SelectiveScrollingGrid.cs
- Wizard.cs
- AdRotatorDesigner.cs
- WebPartsPersonalizationAuthorization.cs
- PerformanceCounterPermission.cs
- SizeFConverter.cs
- SystemIcmpV6Statistics.cs
- Deserializer.cs
- MouseEventArgs.cs
- MissingManifestResourceException.cs
- PassportAuthenticationEventArgs.cs
- UserControlAutomationPeer.cs
- _PooledStream.cs
- RequestResizeEvent.cs
- SByteStorage.cs
- CustomError.cs
- WebPartManager.cs
- SessionStateSection.cs
- Duration.cs
- XPathParser.cs
- ScriptingRoleServiceSection.cs
- BaseCodeDomTreeGenerator.cs
- RuntimeHelpers.cs
- QuadraticBezierSegment.cs
- XPathNodeInfoAtom.cs
- ControlParameter.cs
- Size3DValueSerializer.cs
- httpapplicationstate.cs
- SelectionBorderGlyph.cs
- RightsManagementPermission.cs
- SByte.cs
- Pens.cs
- RegexCapture.cs
- TextHidden.cs
- SiteMap.cs
- localization.cs
- MatrixTransform3D.cs
- BitmapEffectDrawing.cs
- Array.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- xmlfixedPageInfo.cs
- ObservableDictionary.cs
- PropertyMapper.cs
- SafeHandles.cs
- TraceRecord.cs
- CellTreeNode.cs
- ConstraintCollection.cs
- RichTextBoxAutomationPeer.cs
- DataPagerFieldItem.cs
- DataBoundControlAdapter.cs
- RoutedEventValueSerializer.cs
- XamlPathDataSerializer.cs
- LayoutEvent.cs
- WebDescriptionAttribute.cs
- ResourceDescriptionAttribute.cs
- AccessViolationException.cs
- HwndSourceParameters.cs
- PageBuildProvider.cs
- CorrelationActionMessageFilter.cs
- WriteFileContext.cs
- PersonalizationState.cs
- UIntPtr.cs
- DrawingContextFlattener.cs
- DesignerAttribute.cs
- ImportCatalogPart.cs
- DataPagerFieldItem.cs
- CodeGotoStatement.cs
- StructuralComparisons.cs
- processwaithandle.cs
- SynchronizedKeyedCollection.cs
- BitSet.cs
- ProcessManager.cs
- SortQuery.cs
- BamlCollectionHolder.cs
- DependentList.cs
- SQLByte.cs