Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / NetworkInformation / SystemTcpStatistics.cs / 1 / SystemTcpStatistics.cs
////// namespace System.Net.NetworkInformation { using System.Net.Sockets; using System; using System.ComponentModel; /// /// Provides support for ip configuation information and statistics. /// Tcp specific statistics. internal class SystemTcpStatistics:TcpStatistics { MibTcpStats stats; private SystemTcpStatistics(){} internal SystemTcpStatistics(AddressFamily family){ uint result; if (!ComNetOS.IsPostWin2K){ if (family!= AddressFamily.InterNetwork){ throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); } result = UnsafeNetInfoNativeMethods.GetTcpStatistics(out stats); } else result = UnsafeNetInfoNativeMethods.GetTcpStatisticsEx(out stats, family); if (result != IpHelperErrors.Success) { throw new NetworkInformationException((int)result); } } public override long MinimumTransmissionTimeout{get {return stats.minimumRetransmissionTimeOut;}} public override long MaximumTransmissionTimeout{get {return stats.maximumRetransmissionTimeOut;}} public override long MaximumConnections{get {return stats.maximumConnections;}} public override long ConnectionsInitiated{get {return stats.activeOpens;}} public override long ConnectionsAccepted{get {return stats.passiveOpens;}}// is this true? We should check public override long FailedConnectionAttempts{get {return stats.failedConnectionAttempts;}} public override long ResetConnections{get {return stats.resetConnections;}} public override long CurrentConnections{get {return stats.currentConnections;}} public override long SegmentsReceived{get {return stats.segmentsReceived;}} public override long SegmentsSent{get {return stats.segmentsSent;}} public override long SegmentsResent{get {return stats.segmentsResent;}} public override long ErrorsReceived{get {return stats.errorsReceived;}} public override long ResetsSent{get {return stats.segmentsSentWithReset;}} public override long CumulativeConnections{get {return stats.cumulativeConnections;}} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. ////// namespace System.Net.NetworkInformation { using System.Net.Sockets; using System; using System.ComponentModel; /// /// Provides support for ip configuation information and statistics. /// Tcp specific statistics. internal class SystemTcpStatistics:TcpStatistics { MibTcpStats stats; private SystemTcpStatistics(){} internal SystemTcpStatistics(AddressFamily family){ uint result; if (!ComNetOS.IsPostWin2K){ if (family!= AddressFamily.InterNetwork){ throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); } result = UnsafeNetInfoNativeMethods.GetTcpStatistics(out stats); } else result = UnsafeNetInfoNativeMethods.GetTcpStatisticsEx(out stats, family); if (result != IpHelperErrors.Success) { throw new NetworkInformationException((int)result); } } public override long MinimumTransmissionTimeout{get {return stats.minimumRetransmissionTimeOut;}} public override long MaximumTransmissionTimeout{get {return stats.maximumRetransmissionTimeOut;}} public override long MaximumConnections{get {return stats.maximumConnections;}} public override long ConnectionsInitiated{get {return stats.activeOpens;}} public override long ConnectionsAccepted{get {return stats.passiveOpens;}}// is this true? We should check public override long FailedConnectionAttempts{get {return stats.failedConnectionAttempts;}} public override long ResetConnections{get {return stats.resetConnections;}} public override long CurrentConnections{get {return stats.currentConnections;}} public override long SegmentsReceived{get {return stats.segmentsReceived;}} public override long SegmentsSent{get {return stats.segmentsSent;}} public override long SegmentsResent{get {return stats.segmentsResent;}} public override long ErrorsReceived{get {return stats.errorsReceived;}} public override long ResetsSent{get {return stats.segmentsSentWithReset;}} public override long CumulativeConnections{get {return stats.cumulativeConnections;}} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
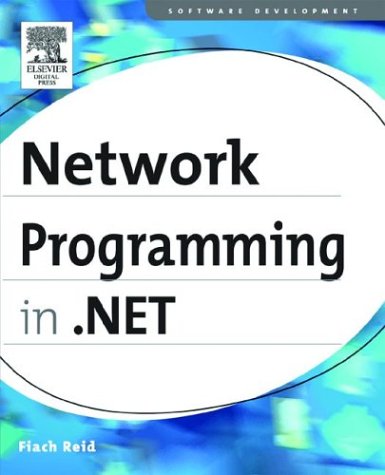
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeMethods.cs
- ByteAnimationBase.cs
- AgileSafeNativeMemoryHandle.cs
- DecimalKeyFrameCollection.cs
- EmbeddedObject.cs
- StylusEditingBehavior.cs
- Error.cs
- XmlSchemaAnyAttribute.cs
- StandardToolWindows.cs
- WebPartEventArgs.cs
- AssemblyName.cs
- GroupBox.cs
- DbConnectionPoolOptions.cs
- Item.cs
- DynamicMethod.cs
- RegexReplacement.cs
- HTTPNotFoundHandler.cs
- WebServiceErrorEvent.cs
- ExtractedStateEntry.cs
- SqlDataReader.cs
- FixUp.cs
- EllipticalNodeOperations.cs
- ChannelToken.cs
- SqlNodeAnnotation.cs
- XmlAtomicValue.cs
- StringToken.cs
- SQLMembershipProvider.cs
- SettingsProperty.cs
- EmptyControlCollection.cs
- MenuItemStyleCollection.cs
- CheckBoxAutomationPeer.cs
- PageSettings.cs
- ContentPosition.cs
- PeerTransportListenAddressValidator.cs
- ExecutionTracker.cs
- SyndicationSerializer.cs
- NonClientArea.cs
- StandardToolWindows.cs
- NotSupportedException.cs
- SubtreeProcessor.cs
- TemplateControlParser.cs
- ResourceDescriptionAttribute.cs
- ValidationHelper.cs
- CapabilitiesState.cs
- LostFocusEventManager.cs
- FrameworkTemplate.cs
- PropertyGridView.cs
- DictionaryTraceRecord.cs
- NetStream.cs
- InfocardChannelParameter.cs
- Select.cs
- Soap.cs
- RemotingSurrogateSelector.cs
- CurrentChangingEventArgs.cs
- MetadataArtifactLoaderCompositeResource.cs
- FontEditor.cs
- ISAPIWorkerRequest.cs
- FilterException.cs
- RowCache.cs
- TemplateColumn.cs
- Block.cs
- ModuleBuilderData.cs
- FormsAuthenticationEventArgs.cs
- ObjectTypeMapping.cs
- MimeBasePart.cs
- Win32SafeHandles.cs
- SqlDataSourceFilteringEventArgs.cs
- ClientSponsor.cs
- adornercollection.cs
- TitleStyle.cs
- HttpEncoder.cs
- XamlReaderConstants.cs
- EmbeddedMailObject.cs
- AxisAngleRotation3D.cs
- MetadataProperty.cs
- WebPartPersonalization.cs
- ResizeGrip.cs
- WpfKnownType.cs
- TrustManagerPromptUI.cs
- TreeViewEvent.cs
- DesignSurfaceEvent.cs
- DrawItemEvent.cs
- DecoderFallback.cs
- HttpWebRequestElement.cs
- AnnouncementService.cs
- DataPagerFieldCollection.cs
- WindowsScrollBarBits.cs
- SafeFileMapViewHandle.cs
- WebServiceClientProxyGenerator.cs
- ResXResourceReader.cs
- BrowserCapabilitiesFactory.cs
- TreeNodeConverter.cs
- DataColumnCollection.cs
- AsymmetricSignatureFormatter.cs
- OneOfTypeConst.cs
- ButtonRenderer.cs
- FontFaceLayoutInfo.cs
- PlainXmlWriter.cs
- ModelItemKeyValuePair.cs
- ExternalException.cs