Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Sys / System / Configuration / SettingsPropertyCollection.cs / 1 / SettingsPropertyCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Globalization; using System.IO; using System.Runtime.Serialization.Formatters.Binary; using System.Xml.Serialization; using System.ComponentModel; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public class SettingsPropertyCollection : IEnumerable, ICloneable, ICollection { private Hashtable _Hashtable = null; private bool _ReadOnly = false; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsPropertyCollection() { _Hashtable = new Hashtable(10, CaseInsensitiveHashCodeProvider.Default, CaseInsensitiveComparer.Default); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Add(SettingsProperty property) { if (_ReadOnly) throw new NotSupportedException(); OnAdd(property); _Hashtable.Add(property.Name, property); try { OnAddComplete(property); } catch { _Hashtable.Remove(property.Name); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Remove(string name) { if (_ReadOnly) throw new NotSupportedException(); SettingsProperty toRemove = (SettingsProperty)_Hashtable[name]; if (toRemove == null) return; OnRemove(toRemove); _Hashtable.Remove(name); try { OnRemoveComplete(toRemove); } catch { _Hashtable.Add(name, toRemove); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsProperty this[string name] { get { return _Hashtable[name] as SettingsProperty; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public IEnumerator GetEnumerator() { return _Hashtable.Values.GetEnumerator(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public object Clone() { return new SettingsPropertyCollection(_Hashtable); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void SetReadOnly() { if (_ReadOnly) return; _ReadOnly = true; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Clear() { if (_ReadOnly) throw new NotSupportedException(); OnClear(); _Hashtable.Clear(); OnClearComplete(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // On* Methods for deriving classes to override. These have // been modeled after the CollectionBase class but have // been stripped of their "index" parameters as there is no // visible index to this collection. protected virtual void OnAdd(SettingsProperty property) {} protected virtual void OnAddComplete(SettingsProperty property) {} protected virtual void OnClear() {} protected virtual void OnClearComplete() {} protected virtual void OnRemove(SettingsProperty property) {} protected virtual void OnRemoveComplete(SettingsProperty property) {} //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // ICollection interface public int Count { get { return _Hashtable.Count; } } public bool IsSynchronized { get { return false; } } public object SyncRoot { get { return this; } } public void CopyTo(Array array, int index) { _Hashtable.Values.CopyTo(array, index); } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// private SettingsPropertyCollection(Hashtable h) { _Hashtable = (Hashtable)h.Clone(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Globalization; using System.IO; using System.Runtime.Serialization.Formatters.Binary; using System.Xml.Serialization; using System.ComponentModel; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public class SettingsPropertyCollection : IEnumerable, ICloneable, ICollection { private Hashtable _Hashtable = null; private bool _ReadOnly = false; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsPropertyCollection() { _Hashtable = new Hashtable(10, CaseInsensitiveHashCodeProvider.Default, CaseInsensitiveComparer.Default); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Add(SettingsProperty property) { if (_ReadOnly) throw new NotSupportedException(); OnAdd(property); _Hashtable.Add(property.Name, property); try { OnAddComplete(property); } catch { _Hashtable.Remove(property.Name); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Remove(string name) { if (_ReadOnly) throw new NotSupportedException(); SettingsProperty toRemove = (SettingsProperty)_Hashtable[name]; if (toRemove == null) return; OnRemove(toRemove); _Hashtable.Remove(name); try { OnRemoveComplete(toRemove); } catch { _Hashtable.Add(name, toRemove); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsProperty this[string name] { get { return _Hashtable[name] as SettingsProperty; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public IEnumerator GetEnumerator() { return _Hashtable.Values.GetEnumerator(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public object Clone() { return new SettingsPropertyCollection(_Hashtable); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void SetReadOnly() { if (_ReadOnly) return; _ReadOnly = true; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Clear() { if (_ReadOnly) throw new NotSupportedException(); OnClear(); _Hashtable.Clear(); OnClearComplete(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // On* Methods for deriving classes to override. These have // been modeled after the CollectionBase class but have // been stripped of their "index" parameters as there is no // visible index to this collection. protected virtual void OnAdd(SettingsProperty property) {} protected virtual void OnAddComplete(SettingsProperty property) {} protected virtual void OnClear() {} protected virtual void OnClearComplete() {} protected virtual void OnRemove(SettingsProperty property) {} protected virtual void OnRemoveComplete(SettingsProperty property) {} //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // ICollection interface public int Count { get { return _Hashtable.Count; } } public bool IsSynchronized { get { return false; } } public object SyncRoot { get { return this; } } public void CopyTo(Array array, int index) { _Hashtable.Values.CopyTo(array, index); } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// private SettingsPropertyCollection(Hashtable h) { _Hashtable = (Hashtable)h.Clone(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
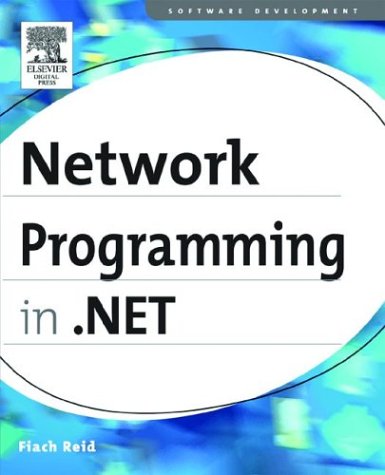
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EncryptedPackage.cs
- GlobalItem.cs
- WeakReference.cs
- AttachedPropertyBrowsableAttribute.cs
- EventDriven.cs
- SchemaCollectionPreprocessor.cs
- HandlerBase.cs
- Geometry3D.cs
- LambdaCompiler.ControlFlow.cs
- PageBuildProvider.cs
- StatusBar.cs
- LambdaCompiler.Unary.cs
- XamlRtfConverter.cs
- DeclarativeCatalogPartDesigner.cs
- CRYPTPROTECT_PROMPTSTRUCT.cs
- Font.cs
- BooleanKeyFrameCollection.cs
- SqlCacheDependencyDatabaseCollection.cs
- DatagridviewDisplayedBandsData.cs
- CapabilitiesAssignment.cs
- UrlPath.cs
- XhtmlConformanceSection.cs
- AppSettingsExpressionBuilder.cs
- XmlCountingReader.cs
- HttpRawResponse.cs
- VScrollBar.cs
- ReadOnlyPropertyMetadata.cs
- ReadingWritingEntityEventArgs.cs
- BamlRecords.cs
- ToolBarPanel.cs
- Label.cs
- WebPartsPersonalization.cs
- XmlAnyAttributeAttribute.cs
- UserControlParser.cs
- XmlNamedNodeMap.cs
- SystemNetworkInterface.cs
- StylusPointPropertyInfo.cs
- EndEvent.cs
- FormsAuthenticationEventArgs.cs
- ProviderBase.cs
- WindowsToolbarAsMenu.cs
- FragmentQueryProcessor.cs
- ThrowOnMultipleAssignment.cs
- ParentUndoUnit.cs
- NeutralResourcesLanguageAttribute.cs
- ZipPackage.cs
- MasterPageCodeDomTreeGenerator.cs
- SafeEventLogWriteHandle.cs
- Int32Converter.cs
- ErrorTolerantObjectWriter.cs
- DatagridviewDisplayedBandsData.cs
- XmlSchemaInferenceException.cs
- Enum.cs
- ThreadStartException.cs
- ComplexTypeEmitter.cs
- TableLayoutPanel.cs
- EncoderParameter.cs
- Math.cs
- BlurEffect.cs
- iisPickupDirectory.cs
- AsyncOperation.cs
- WindowsNonControl.cs
- SparseMemoryStream.cs
- PeerPresenceInfo.cs
- Schedule.cs
- ProfileModule.cs
- Matrix.cs
- EntityKeyElement.cs
- OpenTypeLayoutCache.cs
- typedescriptorpermissionattribute.cs
- ImageList.cs
- ConstructorNeedsTagAttribute.cs
- PersonalizablePropertyEntry.cs
- SqlParameterCollection.cs
- InvalidDataException.cs
- Rule.cs
- Atom10FormatterFactory.cs
- SqlBulkCopyColumnMappingCollection.cs
- PackageProperties.cs
- GlyphingCache.cs
- GeneralTransform3D.cs
- PrintDialogDesigner.cs
- Psha1DerivedKeyGeneratorHelper.cs
- XmlDeclaration.cs
- SystemColorTracker.cs
- GridItemPattern.cs
- ChtmlPhoneCallAdapter.cs
- ValidatorCollection.cs
- InteropEnvironment.cs
- Parameter.cs
- CompressionTransform.cs
- GradientStop.cs
- ISAPIWorkerRequest.cs
- XmlSecureResolver.cs
- ActivationServices.cs
- FieldNameLookup.cs
- ListGeneralPage.cs
- StringPropertyBuilder.cs
- XamlGridLengthSerializer.cs
- WpfPayload.cs