Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ButtonInternal / RadioButtonBaseAdapter.cs / 1 / RadioButtonBaseAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ButtonInternal { using System; using System.Diagnostics; using System.Drawing; using System.Windows.Forms.Internal; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Drawing.Text; using System.Windows.Forms; using System.Windows.Forms.VisualStyles; internal abstract class RadioButtonBaseAdapter : CheckableControlBaseAdapter { internal RadioButtonBaseAdapter(ButtonBase control) : base(control) {} protected new RadioButton Control { get { return ((RadioButton)base.Control); } } #region Drawing helpers protected void DrawCheckFlat(PaintEventArgs e, LayoutData layout, Color checkColor, Color checkBackground, Color checkBorder) { DrawCheckBackgroundFlat(e, layout.checkBounds, checkBorder, checkBackground, true); DrawCheckOnly(e, layout, checkColor, checkBackground, true); } protected void DrawCheckBackground3DLite(PaintEventArgs e, Rectangle bounds, Color checkColor, Color checkBackground, ColorData colors, bool disabledColors) { Graphics g = e.Graphics; Color field = checkBackground; if (!Control.Enabled && disabledColors) { field = SystemColors.Control; } using (Brush fieldBrush = new SolidBrush(field)) { using (Pen dark = new Pen(colors.buttonShadow), light = new Pen(colors.buttonFace), lightlight = new Pen(colors.highlight)) { bounds.Width--; bounds.Height--; // fall a little short of SW, NW, NE, SE because corners come out nasty g.DrawPie(dark, bounds, (float)(135 + 1), (float)(90 - 2)); g.DrawPie(dark, bounds, (float)(225 + 1), (float)(90 - 2)); g.DrawPie(lightlight, bounds, (float)(315 + 1), (float)(90 - 2)); g.DrawPie(lightlight, bounds, (float)(45 + 1), (float)(90 - 2)); bounds.Inflate(-1, -1); g.FillEllipse(fieldBrush, bounds); g.DrawEllipse(light, bounds); } } } protected void DrawCheckBackgroundFlat(PaintEventArgs e, Rectangle bounds, Color borderColor, Color checkBackground, bool disabledColors) { Color field = checkBackground; Color border = borderColor; if (!Control.Enabled && disabledColors) { border = ControlPaint.ContrastControlDark; field = SystemColors.Control; } using( WindowsGraphics wg = WindowsGraphics.FromGraphics(e.Graphics) ) { using( WindowsPen borderPen = new WindowsPen(wg.DeviceContext, border) ) { using( WindowsBrush fieldBrush = new WindowsSolidBrush(wg.DeviceContext, field) ) { DrawAndFillEllipse(wg, borderPen, fieldBrush, bounds); } } } } // Helper method to overcome the poor GDI ellipse drawing routine // VSWhidbey #334097 private static void DrawAndFillEllipse(WindowsGraphics wg, WindowsPen borderPen, WindowsBrush fieldBrush, Rectangle bounds) { Debug.Assert(wg != null,"Calling DrawAndFillEllipse with null wg"); if (wg == null) { return; } wg.FillRectangle(fieldBrush, new Rectangle(bounds.X + 2, bounds.Y + 2, 8, 8)); wg.FillRectangle(fieldBrush, new Rectangle(bounds.X + 4, bounds.Y + 1, 4, 10)); wg.FillRectangle(fieldBrush, new Rectangle(bounds.X + 1, bounds.Y + 4, 10, 4)); wg.DrawLine(borderPen, new Point(bounds.X + 4, bounds.Y + 0), new Point(bounds.X + 8, bounds.Y + 0)); wg.DrawLine(borderPen, new Point(bounds.X + 4, bounds.Y + 11), new Point(bounds.X + 8, bounds.Y + 11)); wg.DrawLine(borderPen, new Point(bounds.X + 2, bounds.Y + 1), new Point(bounds.X + 4, bounds.Y + 1)); wg.DrawLine(borderPen, new Point(bounds.X + 8, bounds.Y + 1), new Point(bounds.X + 10, bounds.Y + 1)); wg.DrawLine(borderPen, new Point(bounds.X + 2, bounds.Y + 10), new Point(bounds.X + 4, bounds.Y + 10)); wg.DrawLine(borderPen, new Point(bounds.X + 8, bounds.Y + 10), new Point(bounds.X + 10, bounds.Y + 10)); wg.DrawLine(borderPen, new Point(bounds.X + 0, bounds.Y + 4), new Point(bounds.X + 0, bounds.Y + 8)); wg.DrawLine(borderPen, new Point(bounds.X + 11, bounds.Y + 4), new Point(bounds.X + 11, bounds.Y + 8)); wg.DrawLine(borderPen, new Point(bounds.X + 1, bounds.Y + 2), new Point(bounds.X + 1, bounds.Y + 4)); wg.DrawLine(borderPen, new Point(bounds.X + 1, bounds.Y + 8), new Point(bounds.X + 1, bounds.Y + 10)); wg.DrawLine(borderPen, new Point(bounds.X + 10, bounds.Y + 2), new Point(bounds.X + 10, bounds.Y + 4)); wg.DrawLine(borderPen, new Point(bounds.X + 10, bounds.Y + 8), new Point(bounds.X + 10, bounds.Y + 10)); } protected void DrawCheckOnly(PaintEventArgs e, LayoutData layout, Color checkColor, Color checkBackground, bool disabledColors) { // check // if (Control.Checked) { if (!Control.Enabled && disabledColors) { checkColor = SystemColors.ControlDark; } using( WindowsGraphics wg = WindowsGraphics.FromGraphics(e.Graphics) ) { using (WindowsBrush brush = new WindowsSolidBrush(wg.DeviceContext, checkColor)) { // circle drawing doesn't work at this size int offset = 5; Rectangle vCross = new Rectangle (layout.checkBounds.X + offset, layout.checkBounds.Y + offset - 1, 2, 4); wg.FillRectangle(brush, vCross); Rectangle hCross = new Rectangle (layout.checkBounds.X + offset - 1, layout.checkBounds.Y + offset, 4, 2); wg.FillRectangle(brush, hCross); } } } } protected ButtonState GetState() { ButtonState style = (ButtonState)0; if (Control.Checked) { style |= ButtonState.Checked; } else { style |= ButtonState.Normal; } if (!Control.Enabled) { style |= ButtonState.Inactive; } if (Control.MouseIsDown) { style |= ButtonState.Pushed; } return style; } protected void DrawCheckBox(PaintEventArgs e, LayoutData layout) { Graphics g = e.Graphics; Rectangle check = layout.checkBounds; if (!Application.RenderWithVisualStyles) { check.X--; // compensate for Windows drawing slightly offset to right } ButtonState style = GetState(); if (Application.RenderWithVisualStyles) { RadioButtonRenderer.DrawRadioButton(g, new Point(check.Left, check.Top), RadioButtonRenderer.ConvertFromButtonState(style, Control.MouseIsOver)); } else { ControlPaint.DrawRadioButton(g, check, style); } } #endregion internal override LayoutOptions CommonLayout() { LayoutOptions layout = base.CommonLayout(); layout.checkAlign = Control.CheckAlign; return layout; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ButtonInternal { using System; using System.Diagnostics; using System.Drawing; using System.Windows.Forms.Internal; using System.Drawing.Drawing2D; using System.Drawing.Imaging; using System.Drawing.Text; using System.Windows.Forms; using System.Windows.Forms.VisualStyles; internal abstract class RadioButtonBaseAdapter : CheckableControlBaseAdapter { internal RadioButtonBaseAdapter(ButtonBase control) : base(control) {} protected new RadioButton Control { get { return ((RadioButton)base.Control); } } #region Drawing helpers protected void DrawCheckFlat(PaintEventArgs e, LayoutData layout, Color checkColor, Color checkBackground, Color checkBorder) { DrawCheckBackgroundFlat(e, layout.checkBounds, checkBorder, checkBackground, true); DrawCheckOnly(e, layout, checkColor, checkBackground, true); } protected void DrawCheckBackground3DLite(PaintEventArgs e, Rectangle bounds, Color checkColor, Color checkBackground, ColorData colors, bool disabledColors) { Graphics g = e.Graphics; Color field = checkBackground; if (!Control.Enabled && disabledColors) { field = SystemColors.Control; } using (Brush fieldBrush = new SolidBrush(field)) { using (Pen dark = new Pen(colors.buttonShadow), light = new Pen(colors.buttonFace), lightlight = new Pen(colors.highlight)) { bounds.Width--; bounds.Height--; // fall a little short of SW, NW, NE, SE because corners come out nasty g.DrawPie(dark, bounds, (float)(135 + 1), (float)(90 - 2)); g.DrawPie(dark, bounds, (float)(225 + 1), (float)(90 - 2)); g.DrawPie(lightlight, bounds, (float)(315 + 1), (float)(90 - 2)); g.DrawPie(lightlight, bounds, (float)(45 + 1), (float)(90 - 2)); bounds.Inflate(-1, -1); g.FillEllipse(fieldBrush, bounds); g.DrawEllipse(light, bounds); } } } protected void DrawCheckBackgroundFlat(PaintEventArgs e, Rectangle bounds, Color borderColor, Color checkBackground, bool disabledColors) { Color field = checkBackground; Color border = borderColor; if (!Control.Enabled && disabledColors) { border = ControlPaint.ContrastControlDark; field = SystemColors.Control; } using( WindowsGraphics wg = WindowsGraphics.FromGraphics(e.Graphics) ) { using( WindowsPen borderPen = new WindowsPen(wg.DeviceContext, border) ) { using( WindowsBrush fieldBrush = new WindowsSolidBrush(wg.DeviceContext, field) ) { DrawAndFillEllipse(wg, borderPen, fieldBrush, bounds); } } } } // Helper method to overcome the poor GDI ellipse drawing routine // VSWhidbey #334097 private static void DrawAndFillEllipse(WindowsGraphics wg, WindowsPen borderPen, WindowsBrush fieldBrush, Rectangle bounds) { Debug.Assert(wg != null,"Calling DrawAndFillEllipse with null wg"); if (wg == null) { return; } wg.FillRectangle(fieldBrush, new Rectangle(bounds.X + 2, bounds.Y + 2, 8, 8)); wg.FillRectangle(fieldBrush, new Rectangle(bounds.X + 4, bounds.Y + 1, 4, 10)); wg.FillRectangle(fieldBrush, new Rectangle(bounds.X + 1, bounds.Y + 4, 10, 4)); wg.DrawLine(borderPen, new Point(bounds.X + 4, bounds.Y + 0), new Point(bounds.X + 8, bounds.Y + 0)); wg.DrawLine(borderPen, new Point(bounds.X + 4, bounds.Y + 11), new Point(bounds.X + 8, bounds.Y + 11)); wg.DrawLine(borderPen, new Point(bounds.X + 2, bounds.Y + 1), new Point(bounds.X + 4, bounds.Y + 1)); wg.DrawLine(borderPen, new Point(bounds.X + 8, bounds.Y + 1), new Point(bounds.X + 10, bounds.Y + 1)); wg.DrawLine(borderPen, new Point(bounds.X + 2, bounds.Y + 10), new Point(bounds.X + 4, bounds.Y + 10)); wg.DrawLine(borderPen, new Point(bounds.X + 8, bounds.Y + 10), new Point(bounds.X + 10, bounds.Y + 10)); wg.DrawLine(borderPen, new Point(bounds.X + 0, bounds.Y + 4), new Point(bounds.X + 0, bounds.Y + 8)); wg.DrawLine(borderPen, new Point(bounds.X + 11, bounds.Y + 4), new Point(bounds.X + 11, bounds.Y + 8)); wg.DrawLine(borderPen, new Point(bounds.X + 1, bounds.Y + 2), new Point(bounds.X + 1, bounds.Y + 4)); wg.DrawLine(borderPen, new Point(bounds.X + 1, bounds.Y + 8), new Point(bounds.X + 1, bounds.Y + 10)); wg.DrawLine(borderPen, new Point(bounds.X + 10, bounds.Y + 2), new Point(bounds.X + 10, bounds.Y + 4)); wg.DrawLine(borderPen, new Point(bounds.X + 10, bounds.Y + 8), new Point(bounds.X + 10, bounds.Y + 10)); } protected void DrawCheckOnly(PaintEventArgs e, LayoutData layout, Color checkColor, Color checkBackground, bool disabledColors) { // check // if (Control.Checked) { if (!Control.Enabled && disabledColors) { checkColor = SystemColors.ControlDark; } using( WindowsGraphics wg = WindowsGraphics.FromGraphics(e.Graphics) ) { using (WindowsBrush brush = new WindowsSolidBrush(wg.DeviceContext, checkColor)) { // circle drawing doesn't work at this size int offset = 5; Rectangle vCross = new Rectangle (layout.checkBounds.X + offset, layout.checkBounds.Y + offset - 1, 2, 4); wg.FillRectangle(brush, vCross); Rectangle hCross = new Rectangle (layout.checkBounds.X + offset - 1, layout.checkBounds.Y + offset, 4, 2); wg.FillRectangle(brush, hCross); } } } } protected ButtonState GetState() { ButtonState style = (ButtonState)0; if (Control.Checked) { style |= ButtonState.Checked; } else { style |= ButtonState.Normal; } if (!Control.Enabled) { style |= ButtonState.Inactive; } if (Control.MouseIsDown) { style |= ButtonState.Pushed; } return style; } protected void DrawCheckBox(PaintEventArgs e, LayoutData layout) { Graphics g = e.Graphics; Rectangle check = layout.checkBounds; if (!Application.RenderWithVisualStyles) { check.X--; // compensate for Windows drawing slightly offset to right } ButtonState style = GetState(); if (Application.RenderWithVisualStyles) { RadioButtonRenderer.DrawRadioButton(g, new Point(check.Left, check.Top), RadioButtonRenderer.ConvertFromButtonState(style, Control.MouseIsOver)); } else { ControlPaint.DrawRadioButton(g, check, style); } } #endregion internal override LayoutOptions CommonLayout() { LayoutOptions layout = base.CommonLayout(); layout.checkAlign = Control.CheckAlign; return layout; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
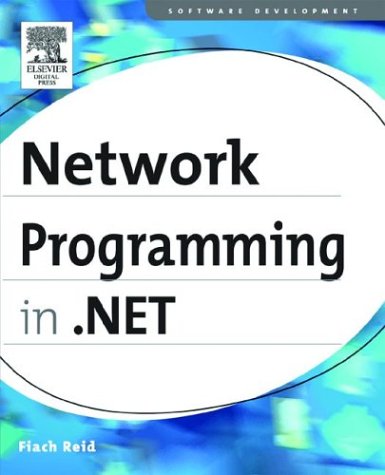
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _HeaderInfoTable.cs
- TransformDescriptor.cs
- XmlQuerySequence.cs
- TextLineResult.cs
- HostingEnvironment.cs
- DynamicControlParameter.cs
- SQLBinary.cs
- CollectionViewGroupRoot.cs
- CacheChildrenQuery.cs
- DBParameter.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- ButtonField.cs
- DrawTreeNodeEventArgs.cs
- DriveNotFoundException.cs
- ProfileSettings.cs
- CommonDialog.cs
- _AutoWebProxyScriptWrapper.cs
- DataBindingCollection.cs
- HtmlTableRow.cs
- ZoneButton.cs
- XmlValueConverter.cs
- ContextMenu.cs
- Win32KeyboardDevice.cs
- AlphaSortedEnumConverter.cs
- Track.cs
- VisualStyleRenderer.cs
- KeyConverter.cs
- DesignerProperties.cs
- PackagePart.cs
- Literal.cs
- ParameterCollection.cs
- thaishape.cs
- TextFormatter.cs
- HandlerBase.cs
- Security.cs
- FontFamilyValueSerializer.cs
- Rect.cs
- SimpleParser.cs
- SafeCoTaskMem.cs
- RSAPKCS1KeyExchangeFormatter.cs
- CancellationScope.cs
- _SecureChannel.cs
- ButtonChrome.cs
- ShapingEngine.cs
- ObjectContext.cs
- FontFaceLayoutInfo.cs
- PropertyBuilder.cs
- DataGridViewColumnConverter.cs
- SqlResolver.cs
- WindowsIPAddress.cs
- CommandExpr.cs
- PauseStoryboard.cs
- UndoUnit.cs
- ConfigWriter.cs
- DataSourceUtil.cs
- TransformCollection.cs
- ProfileSettingsCollection.cs
- NavigatingCancelEventArgs.cs
- TargetConverter.cs
- ADRoleFactoryConfiguration.cs
- RoleGroupCollection.cs
- latinshape.cs
- ProtocolViolationException.cs
- COM2Properties.cs
- webclient.cs
- DataGridViewCellParsingEventArgs.cs
- ScriptMethodAttribute.cs
- IPEndPoint.cs
- InstanceNotFoundException.cs
- AuthenticationService.cs
- OperationCanceledException.cs
- ContextMenuAutomationPeer.cs
- FormsAuthenticationEventArgs.cs
- HttpPostProtocolImporter.cs
- DataSourceControlBuilder.cs
- DataMisalignedException.cs
- glyphs.cs
- Timer.cs
- MissingSatelliteAssemblyException.cs
- DispatchOperationRuntime.cs
- ValidatingCollection.cs
- TextViewBase.cs
- TextParaClient.cs
- Canvas.cs
- HtmlTableCell.cs
- ConfigUtil.cs
- Screen.cs
- FastPropertyAccessor.cs
- HttpListenerContext.cs
- Bold.cs
- MaterialCollection.cs
- DelayLoadType.cs
- SmtpFailedRecipientException.cs
- PaintEvent.cs
- SafeReversePInvokeHandle.cs
- handlecollector.cs
- Utils.cs
- OdbcDataAdapter.cs
- EntityProviderServices.cs
- SimpleExpression.cs