Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / KeyEvent.cs / 1 / KeyEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Diagnostics.CodeAnalysis; ////// /// [System.Runtime.InteropServices.ComVisible(true)] public class KeyEventArgs : EventArgs { ////// Provides data for the ///or /// event. /// /// /// Contains key data for KeyDown and KeyUp events. This is a combination /// of keycode and modifer flags. /// private readonly Keys keyData; ////// /// Determines if this event has been handled by a handler. If handled, the /// key event will not be sent along to Windows. If not handled, the event /// will be sent to Windows for default processing. /// private bool handled = false; ////// /// private bool suppressKeyPress = false; ////// /// public KeyEventArgs(Keys keyData) { this.keyData = keyData; } ////// Initializes a new /// instance of the ///class. /// /// /// public virtual bool Alt { get { return (keyData & Keys.Alt) == Keys.Alt; } } ////// Gets a value indicating whether the ALT key was pressed. /// ////// /// public bool Control { get { return (keyData & Keys.Control) == Keys.Control; } } ////// Gets a value indicating whether the CTRL key was pressed. /// ////// /// // public bool Handled { get { return handled; } set { handled = value; } } ////// Gets or sets a value /// indicating whether the event was handled. /// ////// /// //subhag : changed the behaviour of the KeyCode as per the new requirements. public Keys KeyCode { [ // Keys is discontiguous so we have to use Enum.IsDefined. SuppressMessage("Microsoft.Performance", "CA1803:AvoidCostlyCallsWherePossible") ] get { Keys keyGenerated = keyData & Keys.KeyCode; // since Keys can be discontiguous, keeping Enum.IsDefined. if (!Enum.IsDefined(typeof(Keys),(int)keyGenerated)) return Keys.None; else return keyGenerated; } } ////// Gets the keyboard code for a ///or /// event. /// /// /// //subhag : added the KeyValue as per the new requirements. public int KeyValue { get { return (int)(keyData & Keys.KeyCode); } } ////// Gets the keyboard value for a ///or /// event. /// /// /// public Keys KeyData { get { return keyData; } } ////// Gets the key data for a ///or /// event. /// /// /// public Keys Modifiers { get { return keyData & Keys.Modifiers; } } ////// Gets the modifier flags for a ///or event. /// This indicates which modifier keys (CTRL, SHIFT, and/or ALT) were pressed. /// /// /// public virtual bool Shift { get { return (keyData & Keys.Shift) == Keys.Shift; } } ////// Gets /// a value indicating whether the SHIFT key was pressed. /// ////// /// // public bool SuppressKeyPress { get { return suppressKeyPress; } set { suppressKeyPress = value; handled = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Diagnostics.CodeAnalysis; ////// /// [System.Runtime.InteropServices.ComVisible(true)] public class KeyEventArgs : EventArgs { ////// Provides data for the ///or /// event. /// /// /// Contains key data for KeyDown and KeyUp events. This is a combination /// of keycode and modifer flags. /// private readonly Keys keyData; ////// /// Determines if this event has been handled by a handler. If handled, the /// key event will not be sent along to Windows. If not handled, the event /// will be sent to Windows for default processing. /// private bool handled = false; ////// /// private bool suppressKeyPress = false; ////// /// public KeyEventArgs(Keys keyData) { this.keyData = keyData; } ////// Initializes a new /// instance of the ///class. /// /// /// public virtual bool Alt { get { return (keyData & Keys.Alt) == Keys.Alt; } } ////// Gets a value indicating whether the ALT key was pressed. /// ////// /// public bool Control { get { return (keyData & Keys.Control) == Keys.Control; } } ////// Gets a value indicating whether the CTRL key was pressed. /// ////// /// // public bool Handled { get { return handled; } set { handled = value; } } ////// Gets or sets a value /// indicating whether the event was handled. /// ////// /// //subhag : changed the behaviour of the KeyCode as per the new requirements. public Keys KeyCode { [ // Keys is discontiguous so we have to use Enum.IsDefined. SuppressMessage("Microsoft.Performance", "CA1803:AvoidCostlyCallsWherePossible") ] get { Keys keyGenerated = keyData & Keys.KeyCode; // since Keys can be discontiguous, keeping Enum.IsDefined. if (!Enum.IsDefined(typeof(Keys),(int)keyGenerated)) return Keys.None; else return keyGenerated; } } ////// Gets the keyboard code for a ///or /// event. /// /// /// //subhag : added the KeyValue as per the new requirements. public int KeyValue { get { return (int)(keyData & Keys.KeyCode); } } ////// Gets the keyboard value for a ///or /// event. /// /// /// public Keys KeyData { get { return keyData; } } ////// Gets the key data for a ///or /// event. /// /// /// public Keys Modifiers { get { return keyData & Keys.Modifiers; } } ////// Gets the modifier flags for a ///or event. /// This indicates which modifier keys (CTRL, SHIFT, and/or ALT) were pressed. /// /// /// public virtual bool Shift { get { return (keyData & Keys.Shift) == Keys.Shift; } } ////// Gets /// a value indicating whether the SHIFT key was pressed. /// ////// /// // public bool SuppressKeyPress { get { return suppressKeyPress; } set { suppressKeyPress = value; handled = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
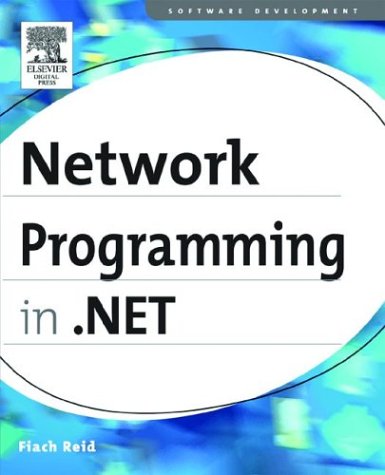
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IPipelineRuntime.cs
- Int32CollectionValueSerializer.cs
- MessageQueueEnumerator.cs
- ManualResetEvent.cs
- DataSourceCache.cs
- OletxResourceManager.cs
- FontFamilyValueSerializer.cs
- ExtractorMetadata.cs
- XmlWriterSettings.cs
- WebBaseEventKeyComparer.cs
- BindingParameterCollection.cs
- TemplatedControlDesigner.cs
- DetailsViewUpdateEventArgs.cs
- graph.cs
- GridViewRowPresenter.cs
- MsdtcClusterUtils.cs
- XamlSerializer.cs
- MetadataCache.cs
- JapaneseLunisolarCalendar.cs
- HierarchicalDataTemplate.cs
- PointAnimationBase.cs
- BmpBitmapDecoder.cs
- CompositeFontFamily.cs
- DataTransferEventArgs.cs
- SvcMapFileLoader.cs
- TextModifier.cs
- iisPickupDirectory.cs
- ResourceDescriptionAttribute.cs
- CodeSubDirectory.cs
- AxisAngleRotation3D.cs
- ConstructorExpr.cs
- GridPattern.cs
- DataGridCell.cs
- WhitespaceReader.cs
- NullableDecimalAverageAggregationOperator.cs
- ExtendLockAsyncResult.cs
- MultiPropertyDescriptorGridEntry.cs
- EmissiveMaterial.cs
- InternalConfigConfigurationFactory.cs
- RtType.cs
- RectangleConverter.cs
- FreeIndexList.cs
- BrowserCapabilitiesFactory.cs
- COM2EnumConverter.cs
- LinkedResource.cs
- CFStream.cs
- Panel.cs
- ReturnEventArgs.cs
- MetadataItemEmitter.cs
- RenderingBiasValidation.cs
- XmlDocumentType.cs
- DataControlCommands.cs
- SpeechAudioFormatInfo.cs
- DSASignatureFormatter.cs
- CheckedPointers.cs
- TraceSection.cs
- SaveLedgerEntryRequest.cs
- UIElementPropertyUndoUnit.cs
- UriExt.cs
- LinkTarget.cs
- HandlerFactoryCache.cs
- RegistrySecurity.cs
- FaultDesigner.cs
- BindingGroup.cs
- TerminatorSinks.cs
- IndexedWhereQueryOperator.cs
- NativeMethods.cs
- sortedlist.cs
- StringWriter.cs
- MetadataException.cs
- BooleanConverter.cs
- ProcessProtocolHandler.cs
- XmlDataSource.cs
- XmlDataSourceNodeDescriptor.cs
- UIElementCollection.cs
- KnownBoxes.cs
- DayRenderEvent.cs
- VisualStateChangedEventArgs.cs
- XpsSerializationManager.cs
- xml.cs
- FontConverter.cs
- ValueChangedEventManager.cs
- SynthesizerStateChangedEventArgs.cs
- DataGridViewColumnHeaderCell.cs
- XmlBinaryReaderSession.cs
- CheckedListBox.cs
- RadialGradientBrush.cs
- MeasureItemEvent.cs
- CodeDirectionExpression.cs
- IndexedDataBuffer.cs
- WebFaultClientMessageInspector.cs
- HtmlShim.cs
- __Error.cs
- BulletedListEventArgs.cs
- PropertyInfo.cs
- XmlSerializerVersionAttribute.cs
- DataColumnMappingCollection.cs
- PageRanges.cs
- X509CertificateValidationMode.cs
- AttachedPropertyMethodSelector.cs