Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Syndication / SyndicationContent.cs / 1 / SyndicationContent.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Syndication { using System; using System.Collections.Generic; using System.Text; using System.Xml; using System.Xml.Serialization; using System.Runtime.Serialization; using System.Diagnostics.CodeAnalysis; public abstract class SyndicationContent { DictionaryattributeExtensions; protected SyndicationContent() { } protected SyndicationContent(SyndicationContent source) { CopyAttributeExtensions(source); } public Dictionary AttributeExtensions { get { if (this.attributeExtensions == null) { this.attributeExtensions = new Dictionary (); } return this.attributeExtensions; } } public abstract string Type { get; } public static TextSyndicationContent CreateHtmlContent(string content) { return new TextSyndicationContent(content, TextSyndicationContentKind.Html); } public static TextSyndicationContent CreatePlaintextContent(string content) { return new TextSyndicationContent(content); } public static UrlSyndicationContent CreateUrlContent(Uri url, string mediaType) { return new UrlSyndicationContent(url, mediaType); } public static TextSyndicationContent CreateXhtmlContent(string content) { return new TextSyndicationContent(content, TextSyndicationContentKind.XHtml); } public static XmlSyndicationContent CreateXmlContent(object dataContractObject) { return new XmlSyndicationContent(Atom10Constants.XmlMediaType, dataContractObject, (DataContractSerializer) null); } public static XmlSyndicationContent CreateXmlContent(object dataContractObject, XmlObjectSerializer dataContractSerializer) { return new XmlSyndicationContent(Atom10Constants.XmlMediaType, dataContractObject, dataContractSerializer); } public static XmlSyndicationContent CreateXmlContent(XmlReader xmlReader) { return new XmlSyndicationContent(xmlReader); } public static XmlSyndicationContent CreateXmlContent(object xmlSerializerObject, XmlSerializer serializer) { return new XmlSyndicationContent(Atom10Constants.XmlMediaType, xmlSerializerObject, serializer); } public abstract SyndicationContent Clone(); public void WriteTo(XmlWriter writer, string outerElementName, string outerElementNamespace) { if (writer == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("writer"); } if (string.IsNullOrEmpty(outerElementName)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR2.GetString(SR2.OuterElementNameNotSpecified)); } writer.WriteStartElement(outerElementName, outerElementNamespace); writer.WriteAttributeString(Atom10Constants.TypeTag, string.Empty, this.Type); if (this.attributeExtensions != null) { foreach (XmlQualifiedName key in this.attributeExtensions.Keys) { if (key.Name == Atom10Constants.TypeTag && key.Namespace == string.Empty) { continue; } string attrValue; if (this.attributeExtensions.TryGetValue(key, out attrValue)) { writer.WriteAttributeString(key.Name, key.Namespace, attrValue); } } } WriteContentsTo(writer); writer.WriteEndElement(); } internal void CopyAttributeExtensions(SyndicationContent source) { if (source == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("source"); } if (source.attributeExtensions != null) { foreach (XmlQualifiedName key in source.attributeExtensions.Keys) { this.AttributeExtensions.Add(key, source.attributeExtensions[key]); } } } protected abstract void WriteContentsTo(XmlWriter writer); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
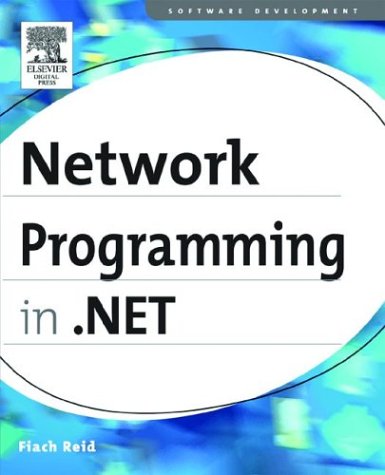
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectSecurity.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- ChannelReliableSession.cs
- PeerChannelListener.cs
- WebPartConnection.cs
- BitStack.cs
- ElementsClipboardData.cs
- CaseExpr.cs
- ResourceExpression.cs
- ListViewItemMouseHoverEvent.cs
- GridViewAutomationPeer.cs
- ColumnMapCopier.cs
- ClaimComparer.cs
- SubstitutionList.cs
- _RequestCacheProtocol.cs
- SystemIcmpV6Statistics.cs
- WebConfigurationManager.cs
- PagedDataSource.cs
- Utils.cs
- IIS7WorkerRequest.cs
- Error.cs
- Action.cs
- DesignerSerializerAttribute.cs
- RootProfilePropertySettingsCollection.cs
- WorkflowLayouts.cs
- Viewport3DAutomationPeer.cs
- backend.cs
- OleDbReferenceCollection.cs
- TimeZone.cs
- GZipObjectSerializer.cs
- TextEmbeddedObject.cs
- FileSystemWatcher.cs
- ExpressionBuilder.cs
- LineInfo.cs
- TextRangeEditLists.cs
- EdgeModeValidation.cs
- ItemDragEvent.cs
- Point3DConverter.cs
- securitycriticaldata.cs
- WebContext.cs
- StrongTypingException.cs
- ListSortDescriptionCollection.cs
- ResourceSetExpression.cs
- SelectedDatesCollection.cs
- SID.cs
- AdRotator.cs
- Converter.cs
- DataListGeneralPage.cs
- Pen.cs
- FragmentNavigationEventArgs.cs
- CryptoStream.cs
- Configuration.cs
- FunctionDetailsReader.cs
- SafeThreadHandle.cs
- Timer.cs
- GridView.cs
- Deserializer.cs
- UiaCoreTypesApi.cs
- LingerOption.cs
- ContextMenu.cs
- ClusterSafeNativeMethods.cs
- ProjectedSlot.cs
- WebPartUtil.cs
- MetadataSource.cs
- TimeoutException.cs
- PlatformCulture.cs
- ContainerParaClient.cs
- FrameworkRichTextComposition.cs
- BinaryObjectInfo.cs
- DBConnectionString.cs
- LowerCaseStringConverter.cs
- TriggerAction.cs
- StandardToolWindows.cs
- AdditionalEntityFunctions.cs
- TextServicesHost.cs
- BuildDependencySet.cs
- HopperCache.cs
- GenericAuthenticationEventArgs.cs
- MediaContextNotificationWindow.cs
- DataGridViewColumnStateChangedEventArgs.cs
- XPathDocument.cs
- ApplicationException.cs
- CLSCompliantAttribute.cs
- Environment.cs
- WindowsTokenRoleProvider.cs
- GregorianCalendar.cs
- XmlTextReaderImpl.cs
- TypeDescriptionProvider.cs
- DiagnosticTrace.cs
- BookmarkEventArgs.cs
- StyleXamlTreeBuilder.cs
- ConsumerConnectionPoint.cs
- CodeTryCatchFinallyStatement.cs
- ContentElement.cs
- ControlTemplate.cs
- PipelineModuleStepContainer.cs
- UnsafeNativeMethods.cs
- RuntimeCompatibilityAttribute.cs
- SqlPersonalizationProvider.cs
- Select.cs