Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Log / System / IO / Log / AppendHelper.cs / 1 / AppendHelper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Runtime.InteropServices; namespace System.IO.Log { class AppendHelper : IDisposable { SequenceNumber prev; SequenceNumber next; FileLogRecordHeader header; UnmanagedBlob[] blobs; GCHandle[] handles; public AppendHelper(IList> data, SequenceNumber prev, SequenceNumber next, bool restartArea) { this.prev = prev; this.next = next; this.header = new FileLogRecordHeader(null); this.header.IsRestartArea = restartArea; this.header.PreviousLsn = prev; this.header.NextUndoLsn = next; this.blobs = new UnmanagedBlob[data.Count + 1]; this.handles = new GCHandle[data.Count + 1]; try { this.handles[0] = GCHandle.Alloc(header.Bits, GCHandleType.Pinned); this.blobs[0].cbSize = (uint)FileLogRecordHeader.Size; this.blobs[0].pBlobData = Marshal.UnsafeAddrOfPinnedArrayElement(header.Bits, 0); for (int i = 0; i < data.Count; i++) { handles[i + 1] = GCHandle.Alloc(data[i].Array, GCHandleType.Pinned); blobs[i + 1].cbSize = (uint)data[i].Count; blobs[i + 1].pBlobData = Marshal.UnsafeAddrOfPinnedArrayElement(data[i].Array, data[i].Offset); } } catch { Dispose(); throw; } } public UnmanagedBlob[] Blobs { get { return this.blobs; } } // Caller should always call Dispose. Finalizer not implemented. public void Dispose() { try { lock(this) { for (int i = 0; i < handles.Length; i++) { if (handles[i].IsAllocated) handles[i].Free(); } } } catch(InvalidOperationException exception) { // This indicates something is broken in IO.Log's memory management, // so it's not safe to continue executing DiagnosticUtility.InvokeFinalHandler(exception); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
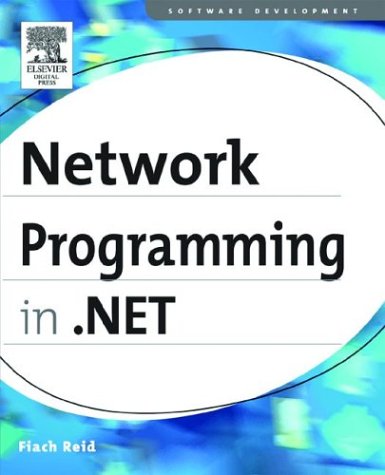
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AmbientLight.cs
- TextTreeTextNode.cs
- __Error.cs
- hebrewshape.cs
- XMLUtil.cs
- XmlAttributeProperties.cs
- sqlinternaltransaction.cs
- Style.cs
- LowerCaseStringConverter.cs
- CursorConverter.cs
- DoubleLinkList.cs
- SecurityDescriptor.cs
- HtmlDocument.cs
- ResourceDisplayNameAttribute.cs
- ChineseLunisolarCalendar.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- CodeMemberMethod.cs
- CfgArc.cs
- ValidationPropertyAttribute.cs
- OletxCommittableTransaction.cs
- ChildTable.cs
- MasterPageBuildProvider.cs
- SkewTransform.cs
- ImmutableCollection.cs
- ExternalException.cs
- GreenMethods.cs
- BinaryCommonClasses.cs
- DbConnectionPoolCounters.cs
- DelegatingHeader.cs
- RegexMatchCollection.cs
- _RequestCacheProtocol.cs
- ManifestSignedXml.cs
- ProfileGroupSettings.cs
- PnrpPermission.cs
- ContextMenuStrip.cs
- ClonableStack.cs
- _Events.cs
- SafeNativeMethods.cs
- OpCodes.cs
- dataobject.cs
- Types.cs
- OpCodes.cs
- RowCache.cs
- XslTransform.cs
- ServiceChannelProxy.cs
- CodeStatement.cs
- NameValueConfigurationElement.cs
- SendingRequestEventArgs.cs
- Animatable.cs
- OdbcStatementHandle.cs
- WebScriptServiceHost.cs
- ReferenceEqualityComparer.cs
- NativeMethods.cs
- WindowsIdentity.cs
- ObjectListItemCollection.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- MobileErrorInfo.cs
- BitArray.cs
- XmlSchemaExporter.cs
- TagPrefixCollection.cs
- DropDownButton.cs
- WindowsListViewSubItem.cs
- ControlDesigner.cs
- SemaphoreFullException.cs
- DataGridViewCellStateChangedEventArgs.cs
- XPathScanner.cs
- CapabilitiesPattern.cs
- xmlglyphRunInfo.cs
- FlowPosition.cs
- WarningException.cs
- UiaCoreProviderApi.cs
- ChangeConflicts.cs
- Pen.cs
- XmlSchemaCollection.cs
- StateBag.cs
- TagMapCollection.cs
- JpegBitmapEncoder.cs
- SnapshotChangeTrackingStrategy.cs
- SessionStateSection.cs
- CrossContextChannel.cs
- Property.cs
- RequestCacheManager.cs
- _ContextAwareResult.cs
- ToolStripMenuItem.cs
- SafeTokenHandle.cs
- ServiceObjectContainer.cs
- Collection.cs
- BooleanStorage.cs
- FilterException.cs
- CultureTable.cs
- UIntPtr.cs
- StorageMappingItemCollection.cs
- StorageInfo.cs
- XPathMultyIterator.cs
- MemberRestriction.cs
- WinCategoryAttribute.cs
- StackOverflowException.cs
- RC2.cs
- TextDecorationLocationValidation.cs
- EDesignUtil.cs