Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / HostedImpersonationContext.cs / 1 / HostedImpersonationContext.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System.ServiceModel; using System.ServiceModel.Diagnostics; using System.Security.Principal; using System.ServiceModel.ComIntegration; using System.ServiceModel.Security; using System.Web.Hosting; using System.Runtime.InteropServices; using System.ComponentModel; using System.Threading; using System.Security; using SafeCloseHandle = System.IdentityModel.SafeCloseHandleCritical; using SMD = System.ServiceModel.Diagnostics; ////// RequiresReview - All member methods are security critical. The class might be used outside of the restricted SecurityContext. /// Ensure they do not accidentally satisfy any demands /// [SecurityRequiresReview] class HostedImpersonationContext { ////// Critical - stores the impersonation token handle. since we're allowing "safe" Impersonation of the token we got from asp.net /// we need to protect this value /// [SecurityCritical] SafeCloseHandle tokenHandle; ////// Critical - controls lifetime of token handle, caller must use care /// [SecurityCritical] int refCount = 0; ////// Critical - a security critical field, caller must use care /// [SecurityCritical] bool isImpersonated; ////// Critical - calls two safe native methods: GetCurrentThread and OpenCurrentThreadToken; and Marshal.GetLastWin32Error /// captures current thread token in a SecurityCritical field /// [SecurityCritical] public HostedImpersonationContext() { if (ServiceHostingEnvironment.AspNetCompatibilityEnabled) { bool isSuccess = SafeNativeMethods.OpenCurrentThreadTokenCritical(SafeNativeMethods.GetCurrentThread(), TokenAccessLevels.Query | TokenAccessLevels.Impersonate, true, out tokenHandle); int error = Marshal.GetLastWin32Error(); if (isSuccess) { isImpersonated = true; Interlocked.Increment(ref refCount); } else { SMD.Utility.CloseInvalidOutSafeHandleCritical(tokenHandle); tokenHandle = null; if (error != (int)Win32Error.ERROR_NO_TOKEN) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new Win32Exception(error, SR.GetString(SR.Hosting_ImpersonationFailed))); } } } } public bool IsImpersonated { ////// Critical - accesses SecurityCritical isImpersonated field /// Safe - does not leak control or mutable/harmful data, no potential for harm /// [SecurityCritical, SecurityTreatAsSafe] get { return isImpersonated; } } ////// Critical - accesses SecurityCritical tokenHandle field and uses LinkDemanded DangerousGetHandle method as well as UnsafeCreate /// caller should use with care, must take responsibility for reverting impersonation /// [SecurityCritical] public IDisposable Impersonate() { if (!isImpersonated) return null; DiagnosticUtility.DebugAssert(tokenHandle != null, "The token handle was incorrectly released earlier."); HostedInnerImpersonationContext context = null; lock(tokenHandle) { context = HostedInnerImpersonationContext.UnsafeCreate(tokenHandle.DangerousGetHandle()); GC.KeepAlive(tokenHandle); } return context; } ////// Critical - controls lifetime of token handle, caller must use care /// [SecurityCritical] public void AddRef() { if (IsImpersonated) { Interlocked.Increment(ref refCount); } } ////// Critical - controls lifetime of token handle, caller must use care /// [SecurityCritical] public void Release() { if (IsImpersonated) { DiagnosticUtility.DebugAssert(tokenHandle != null, "The token handle is incorrectly released earlier."); int currentCount = Interlocked.Decrement(ref refCount); if (currentCount == 0) { lock(tokenHandle) { tokenHandle.Close(); tokenHandle = null; } } } } ////// Critical - keeps track of impersonated user, caller must use with care and call Dispose at the appropriate time /// [SecurityCritical(SecurityCriticalScope.Everything)] class HostedInnerImpersonationContext : IDisposable { IDisposable impersonatedContext; HostedInnerImpersonationContext(IDisposable impersonatedContext) { if (impersonatedContext == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException( SR.GetString(SR.Hosting_ImpersonationFailed))); } this.impersonatedContext = impersonatedContext; } public static HostedInnerImpersonationContext UnsafeCreate(IntPtr token) { return new HostedInnerImpersonationContext(HostingEnvironmentWrapper.UnsafeImpersonate(token)); } public void Dispose() { if (impersonatedContext != null) { impersonatedContext.Dispose(); impersonatedContext = null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
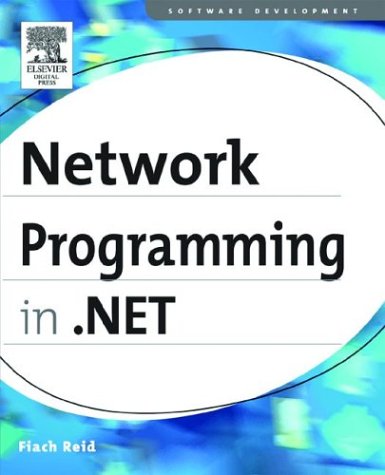
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- baseaxisquery.cs
- CodeChecksumPragma.cs
- DataRowComparer.cs
- ResourceReader.cs
- ColorKeyFrameCollection.cs
- TaskFileService.cs
- ToolBarPanel.cs
- MarkupCompilePass1.cs
- FilterException.cs
- PixelFormat.cs
- TextElement.cs
- AsyncResult.cs
- ListViewItem.cs
- WindowsToolbar.cs
- Int32AnimationUsingKeyFrames.cs
- DesignUtil.cs
- Int16AnimationBase.cs
- IconBitmapDecoder.cs
- Descriptor.cs
- InputMethodStateTypeInfo.cs
- SystemIcons.cs
- Scanner.cs
- CodeStatement.cs
- HtmlElementEventArgs.cs
- HelpKeywordAttribute.cs
- CompilerGlobalScopeAttribute.cs
- TextBreakpoint.cs
- ProcessStartInfo.cs
- SamlDoNotCacheCondition.cs
- ToolStripItemRenderEventArgs.cs
- ParserExtension.cs
- SchemaImporter.cs
- hwndwrapper.cs
- ChtmlFormAdapter.cs
- DigitalSignatureProvider.cs
- WebPartConnectionsConnectVerb.cs
- ExpandCollapsePattern.cs
- X509SecurityTokenProvider.cs
- XmlSchema.cs
- WorkflowQueueInfo.cs
- DataFieldConverter.cs
- SimpleTextLine.cs
- CqlLexer.cs
- RenderingEventArgs.cs
- UTF32Encoding.cs
- WsdlInspector.cs
- ApplicationSettingsBase.cs
- XmlSignatureManifest.cs
- FlatButtonAppearance.cs
- UIElement3DAutomationPeer.cs
- PeerToPeerException.cs
- PageStatePersister.cs
- SynchronousChannel.cs
- Symbol.cs
- DataPagerFieldCommandEventArgs.cs
- WorkflowCreationContext.cs
- BoolExpr.cs
- StatusStrip.cs
- HttpAsyncResult.cs
- SocketPermission.cs
- Int32KeyFrameCollection.cs
- ContentType.cs
- IgnoreSectionHandler.cs
- InputLangChangeRequestEvent.cs
- InputChannel.cs
- SqlDataSourceConfigureSelectPanel.cs
- FloaterBaseParaClient.cs
- CommentEmitter.cs
- Int32CAMarshaler.cs
- IPeerNeighbor.cs
- DBCommand.cs
- ToolStrip.cs
- MatrixUtil.cs
- RuleSetDialog.Designer.cs
- Transform3DGroup.cs
- GridViewDeletedEventArgs.cs
- Selection.cs
- SQLByte.cs
- SerializationBinder.cs
- EntityContainerEmitter.cs
- SafeIUnknown.cs
- OleDbStruct.cs
- CodeLabeledStatement.cs
- SemanticResultKey.cs
- Helper.cs
- ObjectParameterCollection.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- ReachSerializableProperties.cs
- SslStream.cs
- MobileSysDescriptionAttribute.cs
- Size.cs
- OptimalBreakSession.cs
- SuspendDesigner.cs
- Control.cs
- EdmSchemaAttribute.cs
- DBCSCodePageEncoding.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- LogicalMethodInfo.cs
- TableItemProviderWrapper.cs
- ZipPackagePart.cs