Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / ServiceBuildProvider.cs / 1 / ServiceBuildProvider.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.CodeDom; using System.CodeDom.Compiler; using System.Collections; using System.Diagnostics; using System.IO; using System.Reflection; using System.Web.Compilation; using System.Security; using System.Web; using System.Security.Permissions; using System.Threading; ////// Critical - entry-point from asp.net, called outside PermitOnly context /// also needs to elevate in order to inherit from BuildProvider and call methods on the base class /// [SecurityCritical(SecurityCriticalScope.Everything)] [BuildProviderAppliesTo(BuildProviderAppliesTo.Web)] [ServiceActivationBuildProvider] class ServiceBuildProvider : BuildProvider { ServiceParser parser; public override CompilerType CodeCompilerType { get { return GetCodeCompilerType(); } } CompilerType GetCodeCompilerType() { EnsureParsed(); return parser.CompilerType; } protected override CodeCompileUnit GetCodeCompileUnit(out IDictionary linePragmasTable) { CodeSnippetCompileUnit ccu = parser.GetCodeModel() as CodeSnippetCompileUnit; linePragmasTable = parser.GetLinePragmasTable(); return ccu; } void EnsureParsed() { if (parser == null) { parser = new ServiceParser(VirtualPath, this); parser.Parse(ReferencedAssemblies); } } public override BuildProviderResultFlags GetResultFlags(CompilerResults results) { return BuildProviderResultFlags.ShutdownAppDomainOnChange; } public override void GenerateCode(AssemblyBuilder assemblyBuilder) { GenerateCodeCore(assemblyBuilder); } void GenerateCodeCore(AssemblyBuilder assemblyBuilder) { if (assemblyBuilder == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("assemblyBuilder"); } CodeCompileUnit codeCompileUnit = parser.GetCodeModel(); // Bail if we have nothing we need to compile // if (codeCompileUnit == null) return; // Add the code unit and then add all the assemblies // assemblyBuilder.AddCodeCompileUnit(this, codeCompileUnit); if (parser.AssemblyDependencies != null) { foreach (Assembly assembly in parser.AssemblyDependencies) { assemblyBuilder.AddAssemblyReference(assembly); } } } public override string GetCustomString(CompilerResults results) { return GetCustomStringCore(results); } string GetCustomStringCore(CompilerResults results) { return parser.CreateParseString((results == null) ? null : results.CompiledAssembly); } public override System.Collections.ICollection VirtualPathDependencies { get { return parser.SourceDependencies; } } internal CompilerType GetDefaultCompilerTypeForLanguageInternal(string language) { return GetDefaultCompilerTypeForLanguage(language); } internal CompilerType GetDefaultCompilerTypeInternal() { return GetDefaultCompilerType(); } internal TextReader OpenReaderInternal() { return OpenReader(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
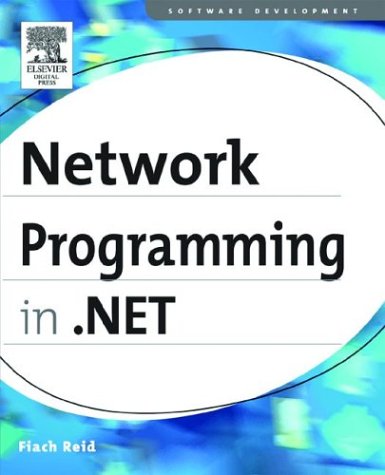
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestCache.cs
- SHA512Managed.cs
- SafeTokenHandle.cs
- HttpHandlerActionCollection.cs
- Helper.cs
- GeneralTransform.cs
- BinaryOperationBinder.cs
- DebugController.cs
- WmlTextViewAdapter.cs
- RuntimeArgumentHandle.cs
- CheckBox.cs
- WebPartExportVerb.cs
- ListViewInsertionMark.cs
- invalidudtexception.cs
- WebContentFormatHelper.cs
- XhtmlBasicCalendarAdapter.cs
- ZoneButton.cs
- DataRecordInternal.cs
- PlatformNotSupportedException.cs
- TransformerTypeCollection.cs
- PagePropertiesChangingEventArgs.cs
- DataViewListener.cs
- UInt64.cs
- Rect3DConverter.cs
- CommonGetThemePartSize.cs
- SourceElementsCollection.cs
- InnerItemCollectionView.cs
- DbConnectionFactory.cs
- WindowsGraphicsWrapper.cs
- TextDpi.cs
- IBuiltInEvidence.cs
- BookmarkTable.cs
- WebPartsPersonalization.cs
- EraserBehavior.cs
- CodeDomLocalizationProvider.cs
- PageContentAsyncResult.cs
- MinimizableAttributeTypeConverter.cs
- RequestCacheManager.cs
- InlineObject.cs
- DeleteIndexBinder.cs
- MulticastIPAddressInformationCollection.cs
- XmlReader.cs
- DbConnectionOptions.cs
- ManagedCodeMarkers.cs
- PeerNameRecord.cs
- MediaContext.cs
- ExtensionQuery.cs
- MDIControlStrip.cs
- UserControl.cs
- OutputCacheSettingsSection.cs
- AdornerDecorator.cs
- PointCollectionValueSerializer.cs
- KeyValueSerializer.cs
- PropertySourceInfo.cs
- MouseActionConverter.cs
- DefaultValidator.cs
- TripleDESCryptoServiceProvider.cs
- RadioButtonAutomationPeer.cs
- RuntimeComponentFilter.cs
- coordinatorscratchpad.cs
- TCPClient.cs
- IgnoreSectionHandler.cs
- Style.cs
- DragDeltaEventArgs.cs
- AttributeEmitter.cs
- ColorConverter.cs
- Constraint.cs
- DynamicObject.cs
- SchemaImporterExtension.cs
- ShaperBuffers.cs
- Point3DAnimation.cs
- SqlServer2KCompatibilityCheck.cs
- PartialArray.cs
- BrowserCapabilitiesFactoryBase.cs
- ConfigurationFileMap.cs
- ExpressionEditorSheet.cs
- ParserOptions.cs
- TemplateNodeContextMenu.cs
- TextParagraph.cs
- ModelVisual3D.cs
- OrderByQueryOptionExpression.cs
- StaticResourceExtension.cs
- ListView.cs
- TdsParserStateObject.cs
- PasswordBoxAutomationPeer.cs
- VisualCollection.cs
- ArgumentException.cs
- DifferencingCollection.cs
- Inline.cs
- SqlRecordBuffer.cs
- SiblingIterators.cs
- QueryLifecycle.cs
- BitmapImage.cs
- DesignUtil.cs
- ContentElement.cs
- ValidationPropertyAttribute.cs
- ConstantProjectedSlot.cs
- RuntimeWrappedException.cs
- ComponentResourceKeyConverter.cs
- DrawingBrush.cs