Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Activation / ServiceMemoryGates.cs / 1 / ServiceMemoryGates.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System.Collections.Generic; using System.ServiceModel.Channels; using System.Runtime.InteropServices; using System.ComponentModel; using System.Threading; using System.Runtime.CompilerServices; using System.Security; static class ServiceMemoryGates { ////// Critical - uses SecurityCritical helper methods to check memory status /// - allocates unmanaged resources, can only be called with admin-specified value /// [SecurityCritical] internal static void Check(int minFreeMemoryPercentage) { // Boundary check percentage, if out of bounds Gate is turned off. // 0 is defined as disabled. Configuration defines 99 as max allowed so we disable // if we receive something out of range if (minFreeMemoryPercentage < 1 || minFreeMemoryPercentage > 99) { return; } UnsafeNativeMethods.MEMORYSTATUSEX memoryStatus = new UnsafeNativeMethods.MEMORYSTATUSEX(); GetGlobalMemoryStatus(ref memoryStatus); ulong threshold = memoryStatus.ullTotalPageFile / 100 * (ulong)minFreeMemoryPercentage; if (memoryStatus.ullAvailPageFile < threshold) { // Yield this thread -- this will also ensure that we have a full time slice when we // do our alloc/free Thread.Sleep(0); // Commit 1.5 times of the threshold. This should force the page file to grow, if // possible. (GlobalMemoryStatusEx only returns information about the current pagefile, // not about the maximum pagefile.) uint sizeToAlloc = 0; if (threshold < (ulong)int.MaxValue) { sizeToAlloc = (uint)threshold / 2 * 3; } if (sizeToAlloc > 0) { ForcePageFileToGrow(sizeToAlloc); GetGlobalMemoryStatus(ref memoryStatus); threshold = memoryStatus.ullTotalPageFile / 100 * (ulong)minFreeMemoryPercentage; } if (memoryStatus.ullAvailPageFile < threshold) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InsufficientMemoryException( SR.GetString(SR.Hosting_MemoryGatesCheckFailed, memoryStatus.ullAvailPageFile, minFreeMemoryPercentage))); } } } ////// Critical - Uses UnsafeNativeMethods, caller must guard parameter /// [SecurityCritical] static void ForcePageFileToGrow(uint sizeToAlloc) { RuntimeHelpers.PrepareConstrainedRegions(); try {} finally { // We don't care if this actually succeeds or not, we are just trying to grow the page // file. #pragma warning suppress 56523 // [....], we don't care whether this actually succeeds IntPtr ptr = UnsafeNativeMethods.VirtualAlloc(IntPtr.Zero, (UIntPtr)sizeToAlloc, UnsafeNativeMethods.MEM_COMMIT, (uint)UnsafeNativeMethods.PAGE_READWRITE); if (ptr != IntPtr.Zero) { #pragma warning suppress 56523 // [....], we don't care whether this actually succeeds UnsafeNativeMethods.VirtualFree(ptr, (UIntPtr)sizeToAlloc, UnsafeNativeMethods.MEM_DECOMMIT); } } } ////// Critical - Uses UnsafeNativeMethods to fetch memory status structure, caller must not leak it /// [SecurityCritical] static void GetGlobalMemoryStatus(ref UnsafeNativeMethods.MEMORYSTATUSEX memoryStatus) { memoryStatus.dwLength = (uint)Marshal.SizeOf(typeof(UnsafeNativeMethods.MEMORYSTATUSEX)); if (!UnsafeNativeMethods.GlobalMemoryStatusEx(ref memoryStatus)) { int error = Marshal.GetLastWin32Error(); // Treat as the worst case. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new InvalidOperationException(SR.GetString(SR.Hosting_GetGlobalMemoryFailed), new Win32Exception(error))); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
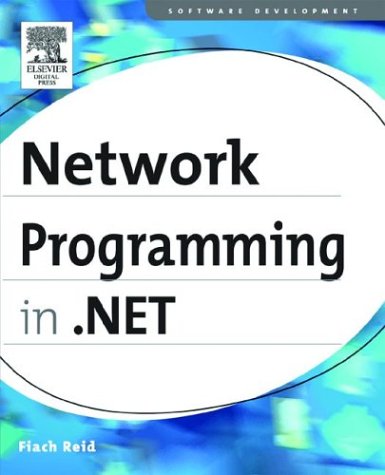
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusPointProperties.cs
- RotateTransform3D.cs
- PageCodeDomTreeGenerator.cs
- NavigationExpr.cs
- IndentTextWriter.cs
- StorageTypeMapping.cs
- BasicAsyncResult.cs
- ToolStripRenderEventArgs.cs
- CaretElement.cs
- InvalidateEvent.cs
- SqlCharStream.cs
- ReadingWritingEntityEventArgs.cs
- IriParsingElement.cs
- HashSetEqualityComparer.cs
- ApplicationId.cs
- TextStore.cs
- NumberSubstitution.cs
- ConstraintEnumerator.cs
- ProfileManager.cs
- PcmConverter.cs
- ObjectDataSourceFilteringEventArgs.cs
- DbMetaDataCollectionNames.cs
- UnicastIPAddressInformationCollection.cs
- RequiredAttributeAttribute.cs
- Scheduler.cs
- ControlCollection.cs
- WeakReferenceEnumerator.cs
- FixedBufferAttribute.cs
- IBuiltInEvidence.cs
- DataGridRowHeaderAutomationPeer.cs
- OracleFactory.cs
- WeakReadOnlyCollection.cs
- WindowPattern.cs
- TextProviderWrapper.cs
- SecurityChannelListener.cs
- Convert.cs
- ConfigXmlElement.cs
- RowUpdatingEventArgs.cs
- EntityChangedParams.cs
- MemberNameValidator.cs
- ExpressionParser.cs
- NestedContainer.cs
- ToolStripScrollButton.cs
- AgileSafeNativeMemoryHandle.cs
- EventLogEntry.cs
- ActivatedMessageQueue.cs
- ImageCodecInfoPrivate.cs
- SortDescription.cs
- Int64AnimationUsingKeyFrames.cs
- MediaScriptCommandRoutedEventArgs.cs
- FontCacheLogic.cs
- FunctionDetailsReader.cs
- PointCollection.cs
- XmlSchemaType.cs
- ImageListStreamer.cs
- DependencyPropertyHelper.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- DocumentScope.cs
- ClassData.cs
- RuleSettingsCollection.cs
- SafeNativeMemoryHandle.cs
- XmlSchemaNotation.cs
- DocumentViewer.cs
- FreeIndexList.cs
- Point4DConverter.cs
- SHA512.cs
- ContactManager.cs
- ImageAutomationPeer.cs
- EncoderNLS.cs
- QueryStatement.cs
- WebSysDefaultValueAttribute.cs
- InvokePatternIdentifiers.cs
- CachedPathData.cs
- WebScriptMetadataMessage.cs
- WorkflowExecutor.cs
- RegexMatch.cs
- Scripts.cs
- MinMaxParagraphWidth.cs
- FixedSOMTableRow.cs
- MetadataSection.cs
- DataGridCaption.cs
- StylusShape.cs
- DocumentPageView.cs
- CodeTypeConstructor.cs
- ImageUrlEditor.cs
- ItemCheckedEvent.cs
- BufferModesCollection.cs
- Rect3D.cs
- TextEditor.cs
- CatalogZoneBase.cs
- RenderData.cs
- ConnectionConsumerAttribute.cs
- StoreContentChangedEventArgs.cs
- MgmtConfigurationRecord.cs
- DataRowCollection.cs
- BinaryEditor.cs
- XmlNamespaceManager.cs
- DataGridViewCellStyleConverter.cs
- URL.cs
- MediaTimeline.cs