Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / AddressHeaderCollection.cs / 1 / AddressHeaderCollection.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel; using System.Collections.ObjectModel; using System.Diagnostics; using System.Runtime.Serialization; using System.Text; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; using System.ServiceModel.Security; using System.IdentityModel.Claims; using System.IdentityModel.Policy; public sealed class AddressHeaderCollection : ReadOnlyCollection{ static AddressHeaderCollection emptyHeaderCollection = new AddressHeaderCollection(); public AddressHeaderCollection() : base(new List ()) { } public AddressHeaderCollection(IEnumerable addressHeaders) : base(new List (addressHeaders)) { // avoid allocating an enumerator when possible IList collection = addressHeaders as IList ; if (collection != null) { for (int i = 0; i < collection.Count; i++) { if (collection[i] == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.MessageHeaderIsNull0))); } } else { foreach (AddressHeader addressHeader in addressHeaders) { if (addressHeaders == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.MessageHeaderIsNull0))); } } } internal static AddressHeaderCollection EmptyHeaderCollection { get { return emptyHeaderCollection; } } int InternalCount { get { if (this == (object)emptyHeaderCollection) return 0; return Count; } } public void AddHeadersTo(Message message) { if (message == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("message"); for (int i = 0; i < InternalCount; i++) { #pragma warning suppress 56506 // [....], Message.Headers can never be null message.Headers.Add(this[i].ToMessageHeader()); } } public AddressHeader[] FindAll(string name, string ns) { if (name == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("name")); if (ns == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("ns")); List results = new List (); for (int i = 0; i < Count; i++) { AddressHeader header = this[i]; if (header.Name == name && header.Namespace == ns) { results.Add(header); } } return results.ToArray(); } public AddressHeader FindHeader(string name, string ns) { if (name == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("name")); if (ns == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("ns")); AddressHeader matchingHeader = null; for (int i = 0; i < Count; i++) { AddressHeader header = this[i]; if (header.Name == name && header.Namespace == ns) { if (matchingHeader != null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentException(SR.GetString(SR.MultipleMessageHeaders, name, ns))); matchingHeader = header; } } return matchingHeader; } internal bool IsEquivalent(AddressHeaderCollection col) { if (InternalCount != col.InternalCount) return false; StringBuilder builder = new StringBuilder(); Dictionary myHeaders = new Dictionary (); PopulateHeaderDictionary(builder, myHeaders); Dictionary otherHeaders = new Dictionary (); col.PopulateHeaderDictionary(builder, otherHeaders); if (myHeaders.Count != otherHeaders.Count) return false; foreach (KeyValuePair pair in myHeaders) { int count; if (otherHeaders.TryGetValue(pair.Key, out count)) { if (count != pair.Value) return false; } else { return false; } } return true; } internal void PopulateHeaderDictionary(StringBuilder builder, Dictionary headers) { string key; for (int i = 0; i < InternalCount; ++i) { builder.Remove(0, builder.Length); key = this[i].GetComparableForm(builder); if (headers.ContainsKey(key)) { headers[key] = headers[key] + 1; } else { headers.Add(key, 1); } } } internal static AddressHeaderCollection ReadServiceParameters(XmlDictionaryReader reader) { return ReadServiceParameters(reader, false); } internal static AddressHeaderCollection ReadServiceParameters(XmlDictionaryReader reader, bool isReferenceProperty) { reader.MoveToContent(); if (reader.IsEmptyElement) { reader.Skip(); return null; } else { reader.ReadStartElement(); List headerList = new List (); while (reader.IsStartElement()) { headerList.Add(new BufferedAddressHeader(reader, isReferenceProperty)); } reader.ReadEndElement(); return new AddressHeaderCollection(headerList); } } internal bool HasReferenceProperties { get { for (int i = 0; i < InternalCount; i++) if (this[i].IsReferenceProperty) return true; return false; } } internal bool HasNonReferenceProperties { get { for (int i = 0; i < InternalCount; i++) if (!this[i].IsReferenceProperty) return true; return false; } } internal void WriteReferencePropertyContentsTo(XmlDictionaryWriter writer) { for (int i = 0; i < InternalCount; i++) if (this[i].IsReferenceProperty) this[i].WriteAddressHeader(writer); } internal void WriteNonReferencePropertyContentsTo(XmlDictionaryWriter writer) { for (int i = 0; i < InternalCount; i++) if (!this[i].IsReferenceProperty) this[i].WriteAddressHeader(writer); } internal void WriteContentsTo(XmlDictionaryWriter writer) { for (int i = 0; i < InternalCount; i++) this[i].WriteAddressHeader(writer); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
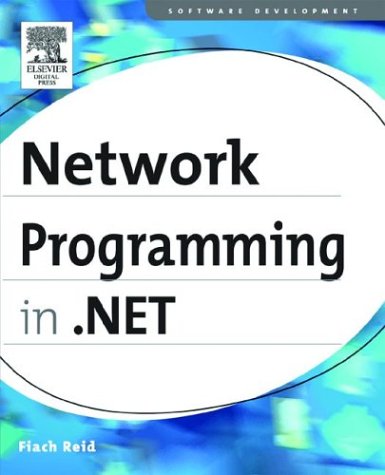
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeConstant.cs
- ActivityCodeGenerator.cs
- TaskFormBase.cs
- Path.cs
- NavigationService.cs
- RangeContentEnumerator.cs
- ThreadNeutralSemaphore.cs
- PTConverter.cs
- SafeHandles.cs
- Point4DValueSerializer.cs
- WebCategoryAttribute.cs
- ImageMetadata.cs
- SqlFlattener.cs
- ZoomPercentageConverter.cs
- AlphabetConverter.cs
- Stylus.cs
- ExpressionVisitorHelpers.cs
- assertwrapper.cs
- Interfaces.cs
- UrlMappingsSection.cs
- input.cs
- GrammarBuilderDictation.cs
- Wildcard.cs
- formatter.cs
- FormsAuthenticationUser.cs
- ObjectIDGenerator.cs
- XmlSequenceWriter.cs
- ListViewGroupConverter.cs
- CheckBoxList.cs
- LinearQuaternionKeyFrame.cs
- PropertyGrid.cs
- UInt32Converter.cs
- WebRequestModuleElementCollection.cs
- Light.cs
- CollectionView.cs
- SqlInternalConnectionSmi.cs
- ProcessInputEventArgs.cs
- AsyncWaitHandle.cs
- InstancePersistenceCommandException.cs
- MenuScrollingVisibilityConverter.cs
- FontCacheLogic.cs
- LongValidator.cs
- SqlDataSource.cs
- BindingExpression.cs
- ServiceProviders.cs
- Control.cs
- SchemaCollectionPreprocessor.cs
- DriveInfo.cs
- ProviderConnectionPointCollection.cs
- HebrewCalendar.cs
- GZipObjectSerializer.cs
- XmlIgnoreAttribute.cs
- XamlSerializationHelper.cs
- URL.cs
- Update.cs
- XmlSchemaSimpleContentExtension.cs
- Helper.cs
- Expression.cs
- ClientRuntimeConfig.cs
- ObjectStorage.cs
- ThaiBuddhistCalendar.cs
- SchemaMerger.cs
- RefExpr.cs
- AdapterDictionary.cs
- RealizationContext.cs
- ChildDocumentBlock.cs
- FileUpload.cs
- PagesChangedEventArgs.cs
- PeerCollaborationPermission.cs
- FormattedTextSymbols.cs
- StoreAnnotationsMap.cs
- Int64AnimationUsingKeyFrames.cs
- TextDecoration.cs
- RotationValidation.cs
- QueryParameter.cs
- DiscoveryReferences.cs
- Validator.cs
- CompilationLock.cs
- TryExpression.cs
- MenuItemBinding.cs
- Pair.cs
- EntityCommandDefinition.cs
- CoTaskMemHandle.cs
- CodeExporter.cs
- OrderingQueryOperator.cs
- TraceLevelStore.cs
- LineBreakRecord.cs
- Renderer.cs
- ChtmlTextWriter.cs
- InvalidFilterCriteriaException.cs
- DrawingImage.cs
- InfoCardXmlSerializer.cs
- PathGeometry.cs
- DataTableMappingCollection.cs
- DataBinding.cs
- EnterpriseServicesHelper.cs
- SrgsItemList.cs
- ETagAttribute.cs
- Keywords.cs
- TabOrder.cs