Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / HttpRequestMessageProperty.cs / 2 / HttpRequestMessageProperty.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Net; using System.ServiceModel.Activation; using System.Collections.Specialized; public sealed class HttpRequestMessageProperty { WebHeaderCollection headers; string method; string queryString; bool suppressEntityBody; HttpListenerRequest listenerRequest; HostedRequestContainer hostedRequestContainer; internal HttpRequestMessageProperty(HttpListenerRequest listenerRequest) : this() { this.listenerRequest = listenerRequest; } internal HttpRequestMessageProperty(HostedRequestContainer hostedRequest) : this() { this.hostedRequestContainer = hostedRequest; } public HttpRequestMessageProperty() { this.method = "POST"; this.queryString = string.Empty; this.suppressEntityBody = false; } public static string Name { get { return "httpRequest"; } } public WebHeaderCollection Headers { get { if (this.headers == null) { this.headers = new WebHeaderCollection(); if (this.listenerRequest != null) { this.headers.Add(this.listenerRequest.Headers); // MB 57988 - System.Net strips off user-agent from the headers collection if (this.listenerRequest.UserAgent != null && this.headers[HttpRequestHeader.UserAgent] == null) { this.headers.Add(HttpRequestHeader.UserAgent, this.listenerRequest.UserAgent); } this.listenerRequest = null; } else if (this.hostedRequestContainer != null) { this.hostedRequestContainer.CopyHeaders(this.headers); this.hostedRequestContainer = null; } } return this.headers; } } public string Method { get { return this.method; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } this.method = value; } } public string QueryString { get { return this.queryString; } set { if (value == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); } this.queryString = value; } } public bool SuppressEntityBody { get { return this.suppressEntityBody; } set { this.suppressEntityBody = value; } } internal void MakeRequestContainerNull() { this.hostedRequestContainer = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
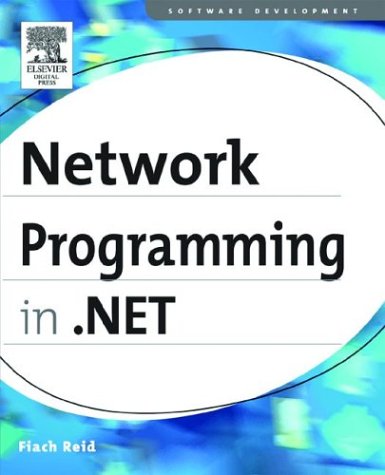
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompletedAsyncResult.cs
- CompositeCollection.cs
- FrameworkContentElementAutomationPeer.cs
- FontFamily.cs
- IEnumerable.cs
- AuthenticationService.cs
- PropertyChangingEventArgs.cs
- ScriptResourceInfo.cs
- ActionMessageFilterTable.cs
- DummyDataSource.cs
- Wizard.cs
- FixedHyperLink.cs
- MouseButtonEventArgs.cs
- FileNameEditor.cs
- XmlNodeChangedEventArgs.cs
- Pair.cs
- DataGridCellAutomationPeer.cs
- List.cs
- Parsers.cs
- ExpressionBuilderCollection.cs
- Console.cs
- PanelStyle.cs
- PeerInputChannelListener.cs
- SiteIdentityPermission.cs
- IndicFontClient.cs
- Label.cs
- BitmapEffect.cs
- TraceFilter.cs
- ScriptRegistrationManager.cs
- arc.cs
- HttpHandlerAction.cs
- CustomAssemblyResolver.cs
- FontFamilyValueSerializer.cs
- ServiceEndpointElementCollection.cs
- SimpleMailWebEventProvider.cs
- FileDataSourceCache.cs
- Model3DGroup.cs
- MouseEvent.cs
- SpeakProgressEventArgs.cs
- QuaternionKeyFrameCollection.cs
- SmiEventStream.cs
- SqlProviderManifest.cs
- NameValueFileSectionHandler.cs
- ParserExtension.cs
- Stack.cs
- RawStylusInputReport.cs
- PersistenceProviderElement.cs
- FamilyMap.cs
- TagNameToTypeMapper.cs
- TableLayoutSettingsTypeConverter.cs
- TypeConstant.cs
- EventMappingSettings.cs
- PersonalizableAttribute.cs
- SignatureDescription.cs
- XamlWriter.cs
- SynchronizationLockException.cs
- PathFigureCollection.cs
- MDIWindowDialog.cs
- MetadataItem.cs
- ObjectDisposedException.cs
- CounterSample.cs
- TriggerAction.cs
- ConnectionPoint.cs
- GenericPrincipal.cs
- UserControlBuildProvider.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- TransactionChannelFaultConverter.cs
- FormsAuthenticationEventArgs.cs
- cookiecontainer.cs
- BoundingRectTracker.cs
- MsmqIntegrationSecurityMode.cs
- CompressionTransform.cs
- ResourceProperty.cs
- DisplayClaim.cs
- ObjectQueryExecutionPlan.cs
- ISessionStateStore.cs
- EventDescriptor.cs
- FixedSOMFixedBlock.cs
- DataView.cs
- TemplatedWizardStep.cs
- InputBindingCollection.cs
- DataBindingList.cs
- _LoggingObject.cs
- WorkflowInstanceAbortedRecord.cs
- DataGridSortCommandEventArgs.cs
- UriPrefixTable.cs
- DataGrid.cs
- FontFamilyValueSerializer.cs
- TemplateField.cs
- PrintControllerWithStatusDialog.cs
- StringArrayEditor.cs
- MemberPathMap.cs
- DataSourceControlBuilder.cs
- LifetimeServices.cs
- ArgumentReference.cs
- CodeBinaryOperatorExpression.cs
- SqlDeflator.cs
- ErrorProvider.cs
- RecordBuilder.cs
- VisualStyleElement.cs