Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / MaxMessageSizeStream.cs / 1 / MaxMessageSizeStream.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.IO; using System.ServiceModel; using System.Diagnostics; class MaxMessageSizeStream : DelegatingStream { long maxMessageSize; long totalBytesRead; long bytesWritten; public MaxMessageSizeStream(Stream stream, long maxMessageSize) : base(stream) { this.maxMessageSize = maxMessageSize; } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { count = PrepareRead(count); return base.BeginRead(buffer, offset, count, callback, state); } public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { PrepareWrite(count); return base.BeginWrite(buffer, offset, count, callback, state); } public override int EndRead(IAsyncResult result) { return FinishRead(base.EndRead(result)); } public override int Read(byte[] buffer, int offset, int count) { count = PrepareRead(count); return FinishRead(base.Read(buffer, offset, count)); } public override int ReadByte() { PrepareRead(1); int i = base.ReadByte(); if (i != -1) FinishRead(1); return i; } public override void Write(byte[] buffer, int offset, int count) { PrepareWrite(count); base.Write(buffer, offset, count); } public override void WriteByte(byte value) { PrepareWrite(1); base.WriteByte(value); } internal static Exception CreateMaxReceivedMessageSizeExceededException(long maxMessageSize) { string message = SR.GetString(SR.MaxReceivedMessageSizeExceeded, maxMessageSize); Exception inner = new QuotaExceededException(message); return new CommunicationException(message, inner); } internal static Exception CreateMaxSentMessageSizeExceededException(long maxMessageSize) { string message = SR.GetString(SR.MaxSentMessageSizeExceeded, maxMessageSize); Exception inner = new QuotaExceededException(message); return new CommunicationException(message, inner); } int PrepareRead(int bytesToRead) { if (totalBytesRead >= maxMessageSize) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(CreateMaxReceivedMessageSizeExceededException(maxMessageSize)); } long bytesRemaining = maxMessageSize - totalBytesRead; if (bytesRemaining > int.MaxValue) { return bytesToRead; } else { return Math.Min(bytesToRead, (int)(maxMessageSize - totalBytesRead)); } } int FinishRead(int bytesRead) { totalBytesRead += bytesRead; return bytesRead; } void PrepareWrite(int bytesToWrite) { if (bytesWritten + bytesToWrite > maxMessageSize) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(CreateMaxSentMessageSizeExceededException(maxMessageSize)); } bytesWritten += bytesToWrite; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
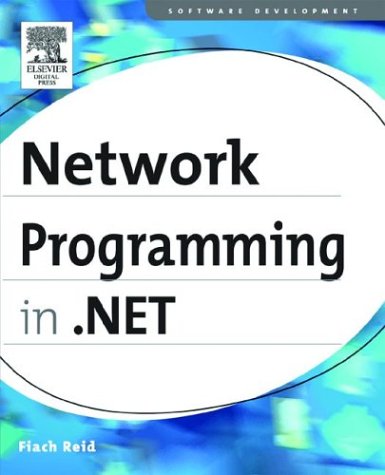
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FileDialog_Vista_Interop.cs
- GradientBrush.cs
- TextServicesCompartmentContext.cs
- ImageFormat.cs
- DrawingCollection.cs
- PropertyGrid.cs
- Geometry3D.cs
- TakeQueryOptionExpression.cs
- HtmlDocument.cs
- JoinCqlBlock.cs
- TrackingValidationObjectDictionary.cs
- NavigationHelper.cs
- recordstatescratchpad.cs
- RegistrationServices.cs
- SqlHelper.cs
- SequentialWorkflowRootDesigner.cs
- Encoder.cs
- XslAst.cs
- StringValidatorAttribute.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- CrossSiteScriptingValidation.cs
- TextSimpleMarkerProperties.cs
- PropertyIDSet.cs
- MessageHeader.cs
- UniqueIdentifierService.cs
- ZoneIdentityPermission.cs
- AesManaged.cs
- DefaultTraceListener.cs
- ArrayWithOffset.cs
- ResourceManager.cs
- LinkUtilities.cs
- CheckBoxPopupAdapter.cs
- SerialPinChanges.cs
- ReturnValue.cs
- ServiceMemoryGates.cs
- HideDisabledControlAdapter.cs
- SqlConnectionManager.cs
- DataMemberConverter.cs
- DrawingVisual.cs
- ExpressionWriter.cs
- SessionIDManager.cs
- Menu.cs
- IdentitySection.cs
- NamespaceInfo.cs
- SrgsElement.cs
- NotCondition.cs
- DataControlCommands.cs
- XmlSchemaValidator.cs
- ProvidersHelper.cs
- TextClipboardData.cs
- SystemIcons.cs
- DoubleCollection.cs
- ArgumentElement.cs
- SoapElementAttribute.cs
- FlowDocumentReaderAutomationPeer.cs
- XmlILStorageConverter.cs
- EventPropertyMap.cs
- TreeNodeBindingCollection.cs
- HMACSHA384.cs
- X509ChainElement.cs
- ProfileService.cs
- TreeViewHitTestInfo.cs
- MulticastOption.cs
- DesignTimeParseData.cs
- TextHintingModeValidation.cs
- ApplicationFileCodeDomTreeGenerator.cs
- BitmapInitialize.cs
- DiscoveryOperationContextExtension.cs
- FileDataSourceCache.cs
- BindingValueChangedEventArgs.cs
- StringFormat.cs
- CompModSwitches.cs
- SynchronizingStream.cs
- _NestedSingleAsyncResult.cs
- AuthorizationRule.cs
- SqlDataSourceCache.cs
- ProfilePropertyMetadata.cs
- SQlBooleanStorage.cs
- ConstantExpression.cs
- SrgsGrammarCompiler.cs
- RadioButtonList.cs
- ErrorLog.cs
- _TimerThread.cs
- DataViewManager.cs
- ClientSettingsProvider.cs
- SmiSettersStream.cs
- Stackframe.cs
- CreateUserWizardStep.cs
- SQLUtility.cs
- EntityRecordInfo.cs
- HyperLinkColumn.cs
- TreeNodeConverter.cs
- MsmqIntegrationProcessProtocolHandler.cs
- MemberAccessException.cs
- EnumerableRowCollection.cs
- DesignerGeometryHelper.cs
- SmiRequestExecutor.cs
- TagPrefixInfo.cs
- AbandonedMutexException.cs
- BindingNavigator.cs