Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / TransportOutputChannel.cs / 1 / TransportOutputChannel.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel; using System.Diagnostics; using System.Xml; abstract class TransportOutputChannel : OutputChannel { bool anyHeadersToAdd; bool manualAddressing; MessageVersion messageVersion; EndpointAddress to; Uri via; ToHeader toHeader; protected TransportOutputChannel(ChannelManagerBase channelManager, EndpointAddress to, Uri via, bool manualAddressing, MessageVersion messageVersion) : base(channelManager) { this.manualAddressing = manualAddressing; this.messageVersion = messageVersion; this.to = to; this.via = via; if (!manualAddressing && to != null) { Uri toUri; if (to.IsAnonymous) { toUri = this.messageVersion.Addressing.AnonymousUri; } else if (to.IsNone) { toUri = this.messageVersion.Addressing.NoneUri; } else { toUri = to.Uri; } XmlDictionaryString dictionaryTo = new ToDictionary(toUri.AbsoluteUri).To; this.toHeader = ToHeader.Create(toUri, dictionaryTo, messageVersion.Addressing); this.anyHeadersToAdd = to.Headers.Count > 0; } } protected bool ManualAddressing { get { return this.manualAddressing; } } public MessageVersion MessageVersion { get { return this.messageVersion; } } public override EndpointAddress RemoteAddress { get { return this.to; } } public override Uri Via { get { return this.via; } } protected override void AddHeadersTo(Message message) { base.AddHeadersTo(message); if (toHeader != null) { // we don't use to.ApplyTo(message) since it's faster to cache and // use the actualheader then to call message.Headers.To = Uri... message.Headers.SetToHeader(toHeader); if (anyHeadersToAdd) { to.Headers.AddHeadersTo(message); } } } class ToDictionary : IXmlDictionary { XmlDictionaryString to; public ToDictionary(string to) { this.to = new XmlDictionaryString(this, to, 0); } public XmlDictionaryString To { get { return to; } } public bool TryLookup(string value, out XmlDictionaryString result) { if (value == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); if (value == to.Value) { result = to; return true; } result = null; return false; } public bool TryLookup(int key, out XmlDictionaryString result) { if (key == 0) { result = to; return true; } result = null; return false; } public bool TryLookup(XmlDictionaryString value, out XmlDictionaryString result) { if (value == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("value"); if (value == to) { result = to; return true; } result = null; return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
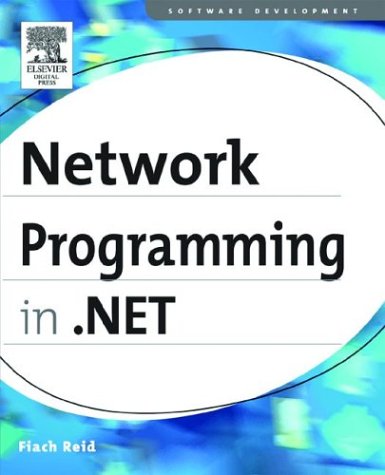
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigUtil.cs
- SmiXetterAccessMap.cs
- MeasureData.cs
- TriState.cs
- Visitor.cs
- WebReferencesBuildProvider.cs
- TabControlCancelEvent.cs
- WsatConfiguration.cs
- VectorCollection.cs
- SHA384Cng.cs
- CharEnumerator.cs
- SqlParameterCollection.cs
- WebPartManager.cs
- MessageQueueInstaller.cs
- DirectoryInfo.cs
- TraceInternal.cs
- EmulateRecognizeCompletedEventArgs.cs
- TextEditorDragDrop.cs
- TemplatedControlDesigner.cs
- StructuredTypeInfo.cs
- LocalBuilder.cs
- JsonReader.cs
- StylusDevice.cs
- HttpContextWrapper.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- _ProxyRegBlob.cs
- XsdBuildProvider.cs
- GridViewRowEventArgs.cs
- XmlSchemaSequence.cs
- SelectionEditor.cs
- DataChangedEventManager.cs
- SqlSupersetValidator.cs
- TrackingAnnotationCollection.cs
- AdRotatorDesigner.cs
- TargetConverter.cs
- WebPartExportVerb.cs
- NameTable.cs
- SqlRemoveConstantOrderBy.cs
- SkinIDTypeConverter.cs
- ReferencedCollectionType.cs
- PeerCollaboration.cs
- Module.cs
- DataControlCommands.cs
- TdsParserStaticMethods.cs
- UserThread.cs
- DataGridGeneralPage.cs
- GlyphElement.cs
- StatusBar.cs
- DataGridTableCollection.cs
- FormViewRow.cs
- oledbconnectionstring.cs
- LookupBindingPropertiesAttribute.cs
- DataRowView.cs
- Method.cs
- QilParameter.cs
- HttpEncoderUtility.cs
- TaiwanLunisolarCalendar.cs
- DynamicValidatorEventArgs.cs
- XmlSchemaType.cs
- messageonlyhwndwrapper.cs
- DataSourceIDConverter.cs
- InvalidateEvent.cs
- SoapAttributeAttribute.cs
- StorageEntityTypeMapping.cs
- XmlDigitalSignatureProcessor.cs
- ResourceProviderFactory.cs
- ExpressionSelection.cs
- DetailsViewRow.cs
- ApplicationServiceHelper.cs
- ThreadAttributes.cs
- RuntimeHandles.cs
- SystemGatewayIPAddressInformation.cs
- WeakReadOnlyCollection.cs
- XmlUtil.cs
- PropertyEmitter.cs
- ListViewDeleteEventArgs.cs
- Activator.cs
- SelectionItemPattern.cs
- OdbcPermission.cs
- SHA512Managed.cs
- LayoutEvent.cs
- AuthenticationServiceManager.cs
- EndpointAddressProcessor.cs
- ProtocolsConfigurationHandler.cs
- SqlDependencyUtils.cs
- WCFModelStrings.Designer.cs
- VectorValueSerializer.cs
- EntityDataSourceStatementEditorForm.cs
- Ops.cs
- FileAuthorizationModule.cs
- BitmapEffectRenderDataResource.cs
- PerformanceCounterPermissionEntryCollection.cs
- PrimitiveType.cs
- ObjectContextServiceProvider.cs
- SplitterEvent.cs
- ContentPlaceHolder.cs
- EdmMember.cs
- Light.cs
- HideDisabledControlAdapter.cs
- securitycriticaldataformultiplegetandset.cs