Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / ComIntegration / ComPlusInstanceProvider.cs / 1 / ComPlusInstanceProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.ComIntegration { using System; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.Diagnostics; using System.EnterpriseServices; using System.Runtime.InteropServices; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.Security.Principal; using System.ServiceModel; using System.ServiceModel.Diagnostics; using SafeCloseHandle = System.IdentityModel.SafeCloseHandle; class ComPlusInstanceProvider : IInstanceProvider { ServiceInfo info; static readonly Guid IID_IUnknown = new Guid("00000000-0000-0000-C000-000000000046"); public ComPlusInstanceProvider(ServiceInfo info) { this.info = info; } public object GetInstance(InstanceContext instanceContext) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ComPlusInstanceProviderRequiresMessage0))); } public object GetInstance(InstanceContext instanceContext, Message message) { object result = null; Guid incomingTransactionID = Guid.Empty; if (ContextUtil.IsInTransaction) incomingTransactionID = ContextUtil.TransactionId; ComPlusInstanceCreationTrace.Trace(TraceEventType.Verbose, TraceCode.ComIntegrationInstanceCreationRequest, SR.TraceCodeComIntegrationInstanceCreationRequest, this.info, message, incomingTransactionID); WindowsIdentity callerIdentity = null; callerIdentity = MessageUtil.GetMessageIdentity(message); WindowsImpersonationContext impersonateContext = null; try { try { if (this.info.HostingMode == HostingMode.WebHostOutOfProcess) { if (SecurityUtils.IsAtleastImpersonationToken(new SafeCloseHandle(callerIdentity.Token, false))) impersonateContext = callerIdentity.Impersonate(); else throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new COMException (SR.GetString(SR.BadImpersonationLevelForOutOfProcWas), HR.ERROR_BAD_IMPERSONATION_LEVEL)); } CLSCTX clsctx = CLSCTX.SERVER; if (PlatformSupportsBitness && (this.info.HostingMode == HostingMode.WebHostOutOfProcess)) { if (this.info.Bitness == Bitness.Bitness32) { clsctx |= CLSCTX.ACTIVATE_32_BIT_SERVER; } else { clsctx |= CLSCTX.ACTIVATE_64_BIT_SERVER; } } result = SafeNativeMethods.CoCreateInstance( info.Clsid, null, clsctx, IID_IUnknown); } finally { if (impersonateContext != null) impersonateContext.Undo(); } } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) throw; Uri from = null; if (message.Headers.From != null) from = message.Headers.From.Uri; DiagnosticUtility.EventLog.LogEvent(TraceEventType.Error, EventLogCategory.ComPlus, EventLogEventId.ComPlusInstanceCreationError, from == null ? string.Empty : from.ToString(), this.info.AppID.ToString(), this.info.Clsid.ToString(), incomingTransactionID.ToString(), callerIdentity.Name, e.ToString()); throw TraceUtility.ThrowHelperError(e, message); } TransactionProxy proxy = instanceContext.Extensions.Find(); if (proxy != null) { proxy.InstanceID = result.GetHashCode(); } ComPlusInstanceCreationTrace.Trace(TraceEventType.Verbose, TraceCode.ComIntegrationInstanceCreationSuccess, SR.TraceCodeComIntegrationInstanceCreationSuccess, this.info, message, result.GetHashCode(), incomingTransactionID); return result; } public void ReleaseInstance(InstanceContext instanceContext, object instance) { int instanceID = instance.GetHashCode(); IDisposable disposable = instance as IDisposable; if (disposable != null) { disposable.Dispose(); } else { // All ServicedComponents are disposable, so we don't // have to worry about getting a ServicedComponent // here. // Marshal.ReleaseComObject(instance); } ComPlusInstanceCreationTrace.Trace(TraceEventType.Verbose, TraceCode.ComIntegrationInstanceReleased, SR.TraceCodeComIntegrationInstanceReleased, this.info, instanceContext, instanceID); } static bool platformSupportsBitness; static bool platformSupportsBitnessSet; static bool PlatformSupportsBitness { get { if (!platformSupportsBitnessSet) { // Bitness is supported on Windows 2003 Server SP1 or // greater. // if (Environment.OSVersion.Version.Major > 5) platformSupportsBitness = true; else if (Environment.OSVersion.Version.Major == 5) { if (Environment.OSVersion.Version.Minor > 2) platformSupportsBitness = true; else if (Environment.OSVersion.Version.Minor == 2) { if (!string.IsNullOrEmpty(Environment.OSVersion.ServicePack)) platformSupportsBitness = true; } } platformSupportsBitnessSet = true; } return platformSupportsBitness; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
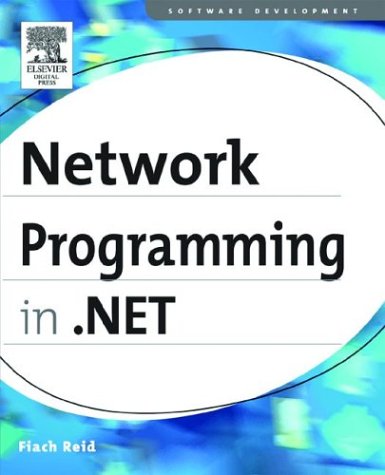
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaManager.cs
- TypeSystemProvider.cs
- ExeContext.cs
- LockedAssemblyCache.cs
- GridEntry.cs
- DataControlFieldCell.cs
- ArithmeticException.cs
- SafeSecurityHelper.cs
- Merger.cs
- codemethodreferenceexpression.cs
- DataServiceRequestOfT.cs
- ListViewContainer.cs
- X509Certificate2.cs
- InOutArgumentConverter.cs
- UrlRoutingHandler.cs
- NullableFloatMinMaxAggregationOperator.cs
- ExpressionBuilder.cs
- FormViewInsertedEventArgs.cs
- DesignerDataTable.cs
- DataGridViewTextBoxEditingControl.cs
- ValueChangedEventManager.cs
- Component.cs
- ApplicationManager.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- SafeViewOfFileHandle.cs
- DataServiceQueryException.cs
- PreApplicationStartMethodAttribute.cs
- TableItemStyle.cs
- HttpDictionary.cs
- SelectedDatesCollection.cs
- CodeArgumentReferenceExpression.cs
- RequestCachePolicy.cs
- PropertyEmitterBase.cs
- ClientScriptManager.cs
- DirectoryObjectSecurity.cs
- NavigatorInput.cs
- InputReportEventArgs.cs
- cryptoapiTransform.cs
- MultiSelector.cs
- TreeNode.cs
- ThicknessAnimation.cs
- SimpleColumnProvider.cs
- SamlAuthorizationDecisionClaimResource.cs
- HandlerFactoryCache.cs
- MimeMapping.cs
- BindingContext.cs
- BitmapSourceSafeMILHandle.cs
- CollectionBase.cs
- OverflowException.cs
- DataProtection.cs
- MessageSecurityOverMsmqElement.cs
- IconHelper.cs
- AgileSafeNativeMemoryHandle.cs
- Evidence.cs
- DrawingCollection.cs
- RenameRuleObjectDialog.Designer.cs
- TableCellAutomationPeer.cs
- DisplayNameAttribute.cs
- TableLayoutRowStyleCollection.cs
- httpapplicationstate.cs
- StylusPlugin.cs
- ExceptionHandler.cs
- TextServicesCompartment.cs
- RangeValidator.cs
- WebServicesDescriptionAttribute.cs
- Axis.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- WebRequest.cs
- BindingNavigator.cs
- StrokeNode.cs
- XPathSelectionIterator.cs
- ObjectStateEntry.cs
- MobileTemplatedControlDesigner.cs
- IntegerCollectionEditor.cs
- XmlDomTextWriter.cs
- XmlObjectSerializerReadContextComplexJson.cs
- TextureBrush.cs
- EditorZoneDesigner.cs
- GridSplitter.cs
- NamedPipeHostedTransportConfiguration.cs
- DetailsViewPagerRow.cs
- base64Transforms.cs
- ResourceBinder.cs
- Shared.cs
- MatrixAnimationBase.cs
- VBIdentifierDesigner.xaml.cs
- XmlAtomicValue.cs
- DoubleLinkListEnumerator.cs
- BigInt.cs
- MsmqInputSessionChannelListener.cs
- XmlWellformedWriter.cs
- RewritingSimplifier.cs
- FullTrustAssembly.cs
- AppDomainManager.cs
- PageStatePersister.cs
- TransferRequestHandler.cs
- DesignerToolStripControlHost.cs
- FixedTextSelectionProcessor.cs
- Stroke2.cs
- IssuanceLicense.cs