Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / XmlSerializerObjectSerializer.cs / 2 / XmlSerializerObjectSerializer.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.Xml; using System.ServiceModel; using System.Xml.Serialization; using System.Collections.Generic; using System.Runtime.Serialization; using System.ServiceModel.Description; internal class XmlSerializerObjectSerializer : XmlObjectSerializer { XmlSerializer serializer; Type rootType; string rootName; string rootNamespace; bool isSerializerSetExplicit = false; internal XmlSerializerObjectSerializer(Type type) { Initialize(type, null /*rootName*/, null /*rootNamespace*/, null /*xmlSerializer*/); } internal XmlSerializerObjectSerializer(Type type, XmlQualifiedName qualifiedName, XmlSerializer xmlSerializer) { if (qualifiedName == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("qualifiedName"); } Initialize(type, qualifiedName.Name, qualifiedName.Namespace, xmlSerializer); } void Initialize(Type type, string rootName, string rootNamespace, XmlSerializer xmlSerializer) { if (type == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("type"); } this.rootType = type; this.rootName = rootName; this.rootNamespace = rootNamespace == null ? string.Empty : rootNamespace; this.serializer = xmlSerializer; if (this.serializer == null) { if (this.rootName == null) this.serializer = new XmlSerializer(type); else { XmlRootAttribute xmlRoot = new XmlRootAttribute(); xmlRoot.ElementName = this.rootName; xmlRoot.Namespace = this.rootNamespace; this.serializer = new XmlSerializer(type, xmlRoot); } } else isSerializerSetExplicit = true; //try to get rootName and rootNamespace from type since root name not set explicitly if (this.rootName == null) { XmlTypeMapping mapping = new XmlReflectionImporter().ImportTypeMapping(this.rootType); this.rootName = mapping.ElementName; this.rootNamespace = mapping.Namespace; } } public override void WriteObject(XmlDictionaryWriter writer, object graph) { if (this.isSerializerSetExplicit) this.serializer.Serialize(writer, new object[] { graph }); else this.serializer.Serialize(writer, graph); } public override void WriteStartObject(XmlDictionaryWriter writer, object graph) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public override void WriteObjectContent(XmlDictionaryWriter writer, object graph) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public override void WriteEndObject(XmlDictionaryWriter writer) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public override object ReadObject(XmlDictionaryReader reader, bool verifyObjectName) { if (this.isSerializerSetExplicit) { object [] deserializedObjects = (object[])this.serializer.Deserialize(reader); if (deserializedObjects != null && deserializedObjects.Length > 0) return deserializedObjects[0]; else return null; } else return this.serializer.Deserialize(reader); } public override bool IsStartObject(XmlDictionaryReader reader) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); reader.MoveToElement(); if (this.rootName != null) { return reader.IsStartElement(this.rootName, this.rootNamespace); } else { return reader.IsStartElement(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
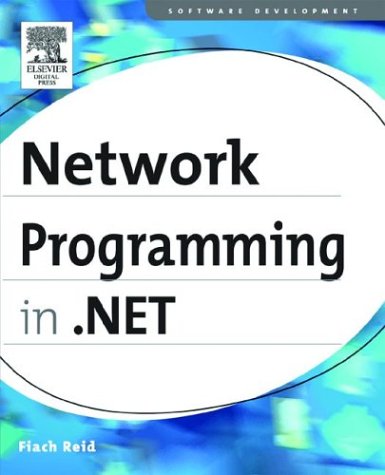
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewCommandEventArgs.cs
- fixedPageContentExtractor.cs
- GlyphRunDrawing.cs
- ExplicitDiscriminatorMap.cs
- ReadOnlyAttribute.cs
- Journal.cs
- PartialCachingControl.cs
- PageThemeParser.cs
- BindingOperations.cs
- X509ClientCertificateAuthentication.cs
- PenThreadPool.cs
- HelpProvider.cs
- ConvertBinder.cs
- RequestCachingSection.cs
- METAHEADER.cs
- RouteItem.cs
- CmsInterop.cs
- ConfigErrorGlyph.cs
- TextWriterEngine.cs
- OracleColumn.cs
- EventHandlingScope.cs
- CancellableEnumerable.cs
- DesignerTransactionCloseEvent.cs
- DependencyObjectType.cs
- Rect3DValueSerializer.cs
- ScriptResourceAttribute.cs
- HostedImpersonationContext.cs
- WebServiceAttribute.cs
- BitmapEncoder.cs
- DataTableTypeConverter.cs
- ReadOnlyCollectionBase.cs
- DefinitionBase.cs
- DesignerWebPartChrome.cs
- SqlDataSource.cs
- XmlAutoDetectWriter.cs
- VisualBrush.cs
- XPathExpr.cs
- RunClient.cs
- TabControlEvent.cs
- SQLDouble.cs
- SafeUserTokenHandle.cs
- ExpressionPrefixAttribute.cs
- EventRoute.cs
- webeventbuffer.cs
- ConstructorExpr.cs
- SqlReorderer.cs
- NativeMethods.cs
- UserControlParser.cs
- XmlCompatibilityReader.cs
- DbConnectionPoolOptions.cs
- XmlNode.cs
- AvTrace.cs
- RetrieveVirtualItemEventArgs.cs
- ReferentialConstraint.cs
- SQLByteStorage.cs
- SnapLine.cs
- StateMachineWorkflow.cs
- DictionaryContent.cs
- ListViewGroup.cs
- QueryAsyncResult.cs
- NullRuntimeConfig.cs
- ConfigurationProviderException.cs
- BitStack.cs
- RectConverter.cs
- ZipIOLocalFileBlock.cs
- ClientEventManager.cs
- Size3D.cs
- TreeViewImageKeyConverter.cs
- NonSerializedAttribute.cs
- InfoCardArgumentException.cs
- DataGridParentRows.cs
- RIPEMD160.cs
- StringUtil.cs
- PropertyGridDesigner.cs
- EncoderParameter.cs
- ToolStripSettings.cs
- AmbientProperties.cs
- XmlSchemaAll.cs
- ReaderContextStackData.cs
- WebConfigurationFileMap.cs
- HiddenField.cs
- ResourceWriter.cs
- StrokeNodeData.cs
- CurrentChangingEventManager.cs
- ValidationError.cs
- PartialArray.cs
- TypeSystem.cs
- RoutedEventHandlerInfo.cs
- State.cs
- GenericPrincipal.cs
- SafeFileMapViewHandle.cs
- DataGridViewMethods.cs
- DSASignatureDeformatter.cs
- UdpReplyToBehavior.cs
- Converter.cs
- TextViewSelectionProcessor.cs
- EdmSchemaAttribute.cs
- PopupRoot.cs
- SizeAnimationClockResource.cs
- HttpWebRequestElement.cs