Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / FaultException.cs / 3 / FaultException.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel { using System; using System.ServiceModel.Channels; using System.Reflection; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Runtime.Serialization; using System.Security.Permissions; using System.Xml; using System.ServiceModel.Dispatcher; using System.Security; [Serializable] [KnownType(typeof(FaultException.FaultCodeData))] [KnownType(typeof(FaultException.FaultCodeData[]))] [KnownType(typeof(FaultException.FaultReasonData))] [KnownType(typeof(FaultException.FaultReasonData[]))] public class FaultException : CommunicationException { internal const string Namespace = "http://schemas.xmlsoap.org/Microsoft/WindowsCommunicationFoundation/2005/08/Faults/"; string action; FaultCode code; FaultReason reason; MessageFault fault; public FaultException() : base(SR.GetString(SR.SFxFaultReason)) { this.code = FaultException.DefaultCode; this.reason = FaultException.DefaultReason; } public FaultException(string reason) : base(reason) { this.code = FaultException.DefaultCode; this.reason = FaultException.CreateReason(reason); } public FaultException(FaultReason reason) : base(FaultException.GetSafeReasonText(reason)) { this.code = FaultException.DefaultCode; this.reason = FaultException.EnsureReason(reason); } public FaultException(string reason, FaultCode code) : base(reason) { this.code = FaultException.EnsureCode(code); this.reason = FaultException.CreateReason(reason); } public FaultException(FaultReason reason, FaultCode code) : base(FaultException.GetSafeReasonText(reason)) { this.code = FaultException.EnsureCode(code); this.reason = FaultException.EnsureReason(reason); } public FaultException(string reason, FaultCode code, string action) : base(reason) { this.code = FaultException.EnsureCode(code); this.reason = FaultException.CreateReason(reason); this.action = action; } internal FaultException(string reason, FaultCode code, string action, Exception innerException) : base(reason, innerException) { this.code = FaultException.EnsureCode(code); this.reason = FaultException.CreateReason(reason); this.action = action; } public FaultException(FaultReason reason, FaultCode code, string action) : base(FaultException.GetSafeReasonText(reason)) { this.code = FaultException.EnsureCode(code); this.reason = FaultException.EnsureReason(reason); this.action = action; } internal FaultException(FaultReason reason, FaultCode code, string action, Exception innerException) : base(FaultException.GetSafeReasonText(reason), innerException) { this.code = FaultException.EnsureCode(code); this.reason = FaultException.EnsureReason(reason); this.action = action; } public FaultException(MessageFault fault) : base(FaultException.GetSafeReasonText(GetReason(fault))) { if (fault == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("fault"); this.code = FaultException.EnsureCode(fault.Code); this.reason = FaultException.EnsureReason(fault.Reason); this.fault = fault; } public FaultException(MessageFault fault, string action) : base(FaultException.GetSafeReasonText(GetReason(fault))) { if (fault == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("fault"); this.code = fault.Code; this.reason = fault.Reason; this.fault = fault; this.action = action; } protected FaultException(SerializationInfo info, StreamingContext context) : base(info, context) { this.code = this.ReconstructFaultCode(info, "code"); this.reason = this.ReconstructFaultReason(info, "reason"); this.fault = (MessageFault)info.GetValue("messageFault", typeof(MessageFault)); this.action = (string)info.GetString("action"); } public string Action { get { return this.action; } } public FaultCode Code { get { return this.code; } } static FaultReason DefaultReason { get { return new FaultReason(SR.GetString(SR.SFxFaultReason)); } } static FaultCode DefaultCode { get { return new FaultCode("Sender"); } } public override string Message { get { return FaultException.GetSafeReasonText(this.Reason); } } public FaultReason Reason { get { return this.reason; } } internal MessageFault Fault { get { return this.fault; } } internal void AddFaultCodeObjectData(SerializationInfo info, string key, FaultCode code) { info.AddValue(key, FaultCodeData.GetObjectData(code)); } internal void AddFaultReasonObjectData(SerializationInfo info, string key, FaultReason reason) { info.AddValue(key, FaultReasonData.GetObjectData(reason)); } static FaultCode CreateCode(string code) { return (code != null) ? new FaultCode(code) : DefaultCode; } public static FaultException CreateFault(MessageFault messageFault, params Type[] faultDetailTypes) { return CreateFault(messageFault, null, faultDetailTypes); } public static FaultException CreateFault(MessageFault messageFault, string action, params Type[] faultDetailTypes) { if (messageFault == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("messageFault"); } if (faultDetailTypes == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("faultDetailTypes"); } DataContractSerializerFaultFormatter faultFormatter = new DataContractSerializerFaultFormatter(faultDetailTypes); return faultFormatter.Deserialize(messageFault, action); } public virtual MessageFault CreateMessageFault() { if (this.fault != null) { return this.fault; } else { return MessageFault.CreateFault(this.code, this.reason); } } static FaultReason CreateReason(string reason) { return (reason != null) ? new FaultReason(reason) : DefaultReason; } ////// Critical - calls base.GetObjectData which is protected by a LinkDemand /// Safe - replicates the LinkDemand /// [SecurityCritical] [SecurityPermissionAttribute(SecurityAction.LinkDemand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); this.AddFaultCodeObjectData(info, "code", this.code); this.AddFaultReasonObjectData(info, "reason", this.reason); info.AddValue("messageFault", this.fault); info.AddValue("action", this.action); } static FaultReason GetReason(MessageFault fault) { if (fault == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("fault"); } return fault.Reason; } internal static string GetSafeReasonText(MessageFault messageFault) { if (messageFault == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("messageFault"); return GetSafeReasonText(messageFault.Reason); } internal static string GetSafeReasonText(FaultReason reason) { if (reason == null) return SR.GetString(SR.SFxUnknownFaultNullReason0); try { return reason.GetMatchingTranslation(System.Globalization.CultureInfo.CurrentCulture).Text; } catch (ArgumentException) { if (reason.Translations.Count == 0) { return SR.GetString(SR.SFxUnknownFaultZeroReasons0); } else { return SR.GetString(SR.SFxUnknownFaultNoMatchingTranslation1, reason.Translations[0].Text); } } } static FaultCode EnsureCode(FaultCode code) { return (code != null) ? code : DefaultCode; } static FaultReason EnsureReason(FaultReason reason) { return (reason != null) ? reason : DefaultReason; } internal FaultCode ReconstructFaultCode(SerializationInfo info, string key) { FaultCodeData[] data = (FaultCodeData[])info.GetValue(key, typeof(FaultCodeData[])); return FaultCodeData.Construct(data); } internal FaultReason ReconstructFaultReason(SerializationInfo info, string key) { FaultReasonData[] data = (FaultReasonData[])info.GetValue(key, typeof(FaultReasonData[])); return FaultReasonData.Construct(data); } [Serializable] internal class FaultCodeData { string name; string ns; internal static FaultCode Construct(FaultCodeData[] nodes) { FaultCode code = null; for (int i=nodes.Length-1; i>=0; i--) { code = new FaultCode(nodes[i].name, nodes[i].ns, code); } return code; } internal static FaultCodeData[] GetObjectData(FaultCode code) { FaultCodeData[] array = new FaultCodeData[FaultCodeData.GetDepth(code)]; for (int i=0; itranslations = reason.Translations; FaultReasonData[] array = new FaultReasonData[translations.Count]; for (int i=0; i : FaultException { TDetail detail; public FaultException(TDetail detail) : base() { this.detail = detail; } public FaultException(TDetail detail, string reason) : base(reason) { this.detail = detail; } public FaultException(TDetail detail, FaultReason reason) : base(reason) { this.detail = detail; } public FaultException(TDetail detail, string reason, FaultCode code) : base(reason, code) { this.detail = detail; } public FaultException(TDetail detail, FaultReason reason, FaultCode code) : base(reason, code) { this.detail = detail; } public FaultException(TDetail detail, string reason, FaultCode code, string action) : base(reason, code, action) { this.detail = detail; } public FaultException(TDetail detail, FaultReason reason, FaultCode code, string action) : base(reason, code, action) { this.detail = detail; } protected FaultException(SerializationInfo info, StreamingContext context) : base(info, context) { this.detail = (TDetail)info.GetValue("detail", typeof(TDetail)); } public TDetail Detail { get { return this.detail; } } public override MessageFault CreateMessageFault() { return MessageFault.CreateFault(this.Code, this.Reason, this.detail); } /// /// Critical - calls base.GetObjectData which is protected by a LinkDemand /// Safe - replicates the LinkDemand /// [SecurityCritical] // SecurityTreatAsSafe: protected by LinkDemand [SecurityPermissionAttribute(SecurityAction.LinkDemand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { base.GetObjectData(info, context); info.AddValue("detail", this.detail); } public override string ToString() { return SR.GetString(SR.SFxFaultExceptionToString3, this.GetType(), this.Message, this.detail.ToString()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
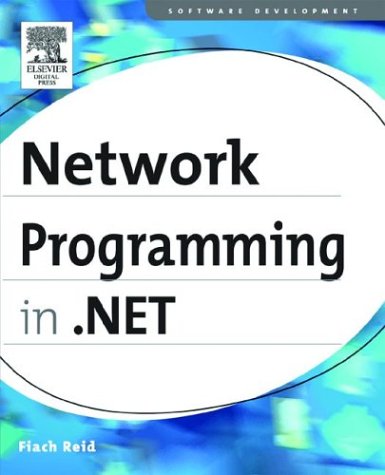
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridCellEditEndingEventArgs.cs
- x509store.cs
- DrawingGroup.cs
- wmiprovider.cs
- LocalBuilder.cs
- ModifierKeysValueSerializer.cs
- ReadOnlyCollectionBase.cs
- HtmlInputRadioButton.cs
- WebPartDescription.cs
- Cell.cs
- ServiceMemoryGates.cs
- IndependentAnimationStorage.cs
- SamlAttribute.cs
- UInt32Converter.cs
- XmlSchemaComplexContentRestriction.cs
- SamlAudienceRestrictionCondition.cs
- ListBoxItem.cs
- RSAPKCS1SignatureFormatter.cs
- ParserStreamGeometryContext.cs
- ObjectSet.cs
- LongTypeConverter.cs
- MethodBody.cs
- ProxyGenerator.cs
- FullTextBreakpoint.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- SessionStateItemCollection.cs
- TimeSpanValidatorAttribute.cs
- MenuRenderer.cs
- NodeLabelEditEvent.cs
- DecimalAverageAggregationOperator.cs
- X509CertificateChain.cs
- PageCatalogPart.cs
- CodeNamespaceCollection.cs
- GridSplitterAutomationPeer.cs
- Canvas.cs
- QueueProcessor.cs
- DateTimePicker.cs
- ScrollViewerAutomationPeer.cs
- GridSplitter.cs
- TrackingParticipant.cs
- ByeOperationCD1AsyncResult.cs
- ManualResetEventSlim.cs
- HwndAppCommandInputProvider.cs
- XpsInterleavingPolicy.cs
- OutputScope.cs
- DnsPermission.cs
- QuestionEventArgs.cs
- HostingEnvironmentSection.cs
- EntityChangedParams.cs
- ClientSettingsProvider.cs
- GPPOINT.cs
- WebPartCloseVerb.cs
- AxDesigner.cs
- UxThemeWrapper.cs
- X500Name.cs
- XmlSchemaIdentityConstraint.cs
- ByteStorage.cs
- FixedSOMTableCell.cs
- CmsInterop.cs
- ResolveNameEventArgs.cs
- SecurityManager.cs
- DataGridViewIntLinkedList.cs
- HtmlInputCheckBox.cs
- AnnotationAuthorChangedEventArgs.cs
- ConnectionPoint.cs
- _NestedMultipleAsyncResult.cs
- OleDbSchemaGuid.cs
- Label.cs
- CatalogZoneBase.cs
- ProxyHwnd.cs
- HashHelper.cs
- nulltextnavigator.cs
- TextSelectionHelper.cs
- NotSupportedException.cs
- EllipseGeometry.cs
- SqlStream.cs
- HttpCookiesSection.cs
- SqlIdentifier.cs
- baseaxisquery.cs
- FactoryGenerator.cs
- MonikerProxyAttribute.cs
- ScriptIgnoreAttribute.cs
- PersianCalendar.cs
- PrePostDescendentsWalker.cs
- AutomationPropertyInfo.cs
- EncodingInfo.cs
- IPPacketInformation.cs
- ComplexObject.cs
- PrintingPermission.cs
- SHA1CryptoServiceProvider.cs
- InputLanguageEventArgs.cs
- DoubleAnimationBase.cs
- BrowserCapabilitiesCompiler.cs
- DesignerRegionCollection.cs
- EntityDataSourceWrapperCollection.cs
- MasterPageParser.cs
- DbXmlEnabledProviderManifest.cs
- UserControlCodeDomTreeGenerator.cs
- WebPartPersonalization.cs
- Models.cs