Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / Tokens / SupportingTokenParameters.cs / 1 / SupportingTokenParameters.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Security.Tokens { using System.ServiceModel.Security; using System.Collections.ObjectModel; using System.Text; using System.Globalization; public class SupportingTokenParameters { Collectionsigned = new Collection (); Collection signedEncrypted = new Collection (); Collection endorsing = new Collection (); Collection signedEndorsing = new Collection (); SupportingTokenParameters(SupportingTokenParameters other) { if (other == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("other"); foreach (SecurityTokenParameters p in other.signed) this.signed.Add((SecurityTokenParameters)p.Clone()); foreach (SecurityTokenParameters p in other.signedEncrypted) this.signedEncrypted.Add((SecurityTokenParameters)p.Clone()); foreach (SecurityTokenParameters p in other.endorsing) this.endorsing.Add((SecurityTokenParameters)p.Clone()); foreach (SecurityTokenParameters p in other.signedEndorsing) this.signedEndorsing.Add((SecurityTokenParameters)p.Clone()); } public SupportingTokenParameters() { // empty } public Collection Endorsing { get { return this.endorsing; } } public Collection SignedEndorsing { get { return this.signedEndorsing; } } public Collection Signed { get { return this.signed; } } public Collection SignedEncrypted { get { return this.signedEncrypted; } } public void SetKeyDerivation(bool requireDerivedKeys) { foreach (SecurityTokenParameters t in this.endorsing) t.RequireDerivedKeys = requireDerivedKeys; foreach (SecurityTokenParameters t in this.signedEndorsing) t.RequireDerivedKeys = requireDerivedKeys; } internal bool IsSetKeyDerivation(bool requireDerivedKeys) { foreach (SecurityTokenParameters t in this.endorsing) if (t.RequireDerivedKeys != requireDerivedKeys) return false; foreach (SecurityTokenParameters t in this.signedEndorsing) if (t.RequireDerivedKeys != requireDerivedKeys) return false; return true; } public override string ToString() { StringBuilder sb = new StringBuilder(); int k; if (this.endorsing.Count == 0) sb.AppendLine("No endorsing tokens."); else for (k = 0; k < this.endorsing.Count; k++) { sb.AppendLine(String.Format(CultureInfo.InvariantCulture, "Endorsing[{0}]", k.ToString(CultureInfo.InvariantCulture))); sb.AppendLine(" " + this.endorsing[k].ToString().Trim().Replace("\n", "\n ")); } if (this.signed.Count == 0) sb.AppendLine("No signed tokens."); else for (k = 0; k < this.signed.Count; k++) { sb.AppendLine(String.Format(CultureInfo.InvariantCulture, "Signed[{0}]", k.ToString(CultureInfo.InvariantCulture))); sb.AppendLine(" " + this.signed[k].ToString().Trim().Replace("\n", "\n ")); } if (this.signedEncrypted.Count == 0) sb.AppendLine("No signed encrypted tokens."); else for (k = 0; k < this.signedEncrypted.Count; k++) { sb.AppendLine(String.Format(CultureInfo.InvariantCulture, "SignedEncrypted[{0}]", k.ToString(CultureInfo.InvariantCulture))); sb.AppendLine(" " + this.signedEncrypted[k].ToString().Trim().Replace("\n", "\n ")); } if (this.signedEndorsing.Count == 0) sb.AppendLine("No signed endorsing tokens."); else for (k = 0; k < this.signedEndorsing.Count; k++) { sb.AppendLine(String.Format(CultureInfo.InvariantCulture, "SignedEndorsing[{0}]", k.ToString(CultureInfo.InvariantCulture))); sb.AppendLine(" " + this.signedEndorsing[k].ToString().Trim().Replace("\n", "\n ")); } return sb.ToString().Trim(); } public SupportingTokenParameters Clone() { return new SupportingTokenParameters(this); } internal bool IsEmpty() { return signed.Count == 0 && signedEncrypted.Count == 0 && endorsing.Count == 0 && signedEndorsing.Count == 0; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
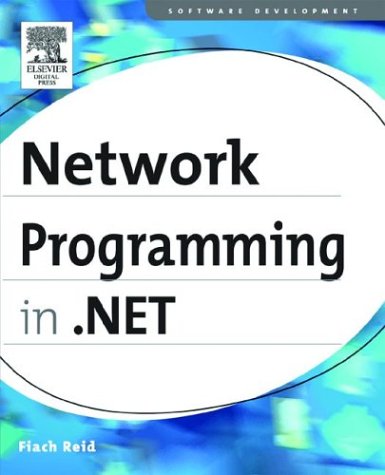
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThreadInterruptedException.cs
- Int64Converter.cs
- ToolStripOverflow.cs
- OracleRowUpdatingEventArgs.cs
- HtmlAnchor.cs
- LazyTextWriterCreator.cs
- XmlLinkedNode.cs
- MethodToken.cs
- SqlClientPermission.cs
- SafeReversePInvokeHandle.cs
- _Rfc2616CacheValidators.cs
- PersonalizationAdministration.cs
- GroupBoxDesigner.cs
- InputReportEventArgs.cs
- ProjectionCamera.cs
- DataSourceXmlSubItemAttribute.cs
- ResXResourceWriter.cs
- UICuesEvent.cs
- PropertyCondition.cs
- KeyValueSerializer.cs
- HtmlTableRow.cs
- GridItemCollection.cs
- WebPartCloseVerb.cs
- WSTrustFeb2005.cs
- ToolStripSeparatorRenderEventArgs.cs
- ProtocolsConfiguration.cs
- safemediahandle.cs
- HTMLTagNameToTypeMapper.cs
- SecUtil.cs
- EventLogPermissionAttribute.cs
- XmlStringTable.cs
- Configuration.cs
- TagPrefixCollection.cs
- AutomationAttributeInfo.cs
- Utility.cs
- RequestCachePolicyConverter.cs
- KeyPullup.cs
- BaseTemplatedMobileComponentEditor.cs
- SymbolEqualComparer.cs
- XmlnsCompatibleWithAttribute.cs
- pingexception.cs
- ScriptManagerProxy.cs
- GuidTagList.cs
- JournalEntryListConverter.cs
- PropertyGrid.cs
- TlsnegoTokenAuthenticator.cs
- ImageConverter.cs
- TokenFactoryCredential.cs
- SemaphoreSecurity.cs
- BitmapDecoder.cs
- WindowsFormsSynchronizationContext.cs
- _Connection.cs
- XmlBindingWorker.cs
- COM2IDispatchConverter.cs
- OnOperation.cs
- DrawListViewColumnHeaderEventArgs.cs
- Sentence.cs
- SQLInt16Storage.cs
- DataSourceSelectArguments.cs
- MemberPath.cs
- CustomSignedXml.cs
- PropertyDescriptorCollection.cs
- ClaimTypes.cs
- PageThemeParser.cs
- UseAttributeSetsAction.cs
- EncryptedPackageFilter.cs
- InstanceLockTracking.cs
- DataKey.cs
- DrawListViewItemEventArgs.cs
- DocumentsTrace.cs
- StylusPointPropertyUnit.cs
- TemplateFactory.cs
- OAVariantLib.cs
- NavigationWindowAutomationPeer.cs
- CodeArrayCreateExpression.cs
- DescendantBaseQuery.cs
- PaintValueEventArgs.cs
- PauseStoryboard.cs
- DirectoryInfo.cs
- OdbcErrorCollection.cs
- ReadWriteControlDesigner.cs
- RawStylusInputCustomDataList.cs
- WsatConfiguration.cs
- PropertyCollection.cs
- ComplexPropertyEntry.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- EUCJPEncoding.cs
- ToolStripLabel.cs
- TreePrinter.cs
- Publisher.cs
- StyleSelector.cs
- ForEachAction.cs
- ConstNode.cs
- WebBrowserPermission.cs
- BindingSource.cs
- ExclusiveNamedPipeTransportManager.cs
- ItemContainerProviderWrapper.cs
- Composition.cs
- ReturnType.cs
- ObjectDataProvider.cs