Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / UserNamePasswordServiceCredential.cs / 2 / UserNamePasswordServiceCredential.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Security { using System.Collections.Generic; using System.ServiceModel; using System.Runtime.Serialization; using System.IdentityModel.Selectors; using System.Net; using System.Security.Principal; using System.ServiceModel.Security.Tokens; using System.Security.Cryptography.X509Certificates; using System.Web.Security; using System.Runtime.CompilerServices; public sealed class UserNamePasswordServiceCredential { internal const UserNamePasswordValidationMode DefaultUserNamePasswordValidationMode = UserNamePasswordValidationMode.Windows; internal const bool DefaultCacheLogonTokens = false; internal const int DefaultMaxCachedLogonTokens = 128; internal const string DefaultCachedLogonTokenLifetimeString = "00:15:00"; internal static readonly TimeSpan DefaultCachedLogonTokenLifetime = TimeSpan.Parse(DefaultCachedLogonTokenLifetimeString); UserNamePasswordValidationMode validationMode = DefaultUserNamePasswordValidationMode; UserNamePasswordValidator validator; object membershipProvider; bool includeWindowsGroups = SspiSecurityTokenProvider.DefaultExtractWindowsGroupClaims; bool cacheLogonTokens = DefaultCacheLogonTokens; int maxCachedLogonTokens = DefaultMaxCachedLogonTokens; TimeSpan cachedLogonTokenLifetime = DefaultCachedLogonTokenLifetime; bool isReadOnly; internal UserNamePasswordServiceCredential() { // empty } internal UserNamePasswordServiceCredential(UserNamePasswordServiceCredential other) { this.includeWindowsGroups = other.includeWindowsGroups; this.membershipProvider = other.membershipProvider; this.validationMode = other.validationMode; this.validator = other.validator; this.cacheLogonTokens = other.cacheLogonTokens; this.maxCachedLogonTokens = other.maxCachedLogonTokens; this.cachedLogonTokenLifetime = other.cachedLogonTokenLifetime; this.isReadOnly = other.isReadOnly; } public UserNamePasswordValidationMode UserNamePasswordValidationMode { get { return this.validationMode; } set { UserNamePasswordValidationModeHelper.Validate(value); ThrowIfImmutable(); this.validationMode = value; } } public UserNamePasswordValidator CustomUserNamePasswordValidator { get { return this.validator; } set { ThrowIfImmutable(); this.validator = value; } } public MembershipProvider MembershipProvider { get { return (MembershipProvider)this.membershipProvider; } set { ThrowIfImmutable(); this.membershipProvider = value; } } public bool IncludeWindowsGroups { get { return this.includeWindowsGroups; } set { ThrowIfImmutable(); this.includeWindowsGroups = value; } } public bool CacheLogonTokens { get { return this.cacheLogonTokens; } set { ThrowIfImmutable(); this.cacheLogonTokens = value; } } public int MaxCachedLogonTokens { get { return this.maxCachedLogonTokens; } set { if (value <= 0) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", SR.GetString(SR.ValueMustBeGreaterThanZero))); } ThrowIfImmutable(); this.maxCachedLogonTokens = value; } } public TimeSpan CachedLogonTokenLifetime { get { return this.cachedLogonTokenLifetime; } set { if (value <= TimeSpan.Zero) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", SR.GetString(SR.TimeSpanMustbeGreaterThanTimeSpanZero))); } if (TimeoutHelper.IsTooLarge(value)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value", value, SR.GetString(SR.SFxTimeoutOutOfRangeTooBig))); } ThrowIfImmutable(); this.cachedLogonTokenLifetime = value; } } internal UserNamePasswordValidator GetUserNamePasswordValidator() { if (this.validationMode == UserNamePasswordValidationMode.MembershipProvider) { return this.GetMembershipProviderValidator(); } else if (this.validationMode == UserNamePasswordValidationMode.Custom) { if (this.validator == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.MissingCustomUserNamePasswordValidator))); } return this.validator; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } [MethodImpl(MethodImplOptions.NoInlining)] UserNamePasswordValidator GetMembershipProviderValidator() { MembershipProvider provider; if (this.membershipProvider != null) { provider = (MembershipProvider)this.membershipProvider; } else { provider = Membership.Provider; } if (provider == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.MissingMembershipProvider))); } return UserNamePasswordValidator.CreateMembershipProviderValidator(provider); } internal void MakeReadOnly() { this.isReadOnly = true; } void ThrowIfImmutable() { if (this.isReadOnly) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ObjectIsReadOnly))); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
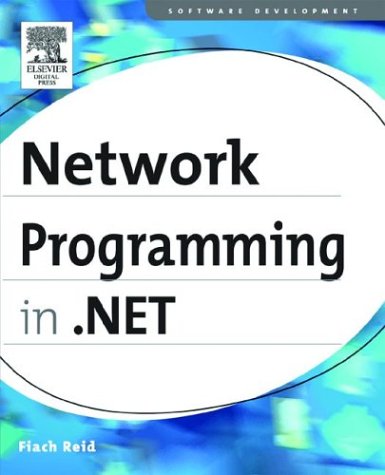
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Version.cs
- XmlWellformedWriter.cs
- NavigationCommands.cs
- DiscardableAttribute.cs
- ServicesUtilities.cs
- RegexTypeEditor.cs
- wgx_commands.cs
- XmlSerializationReader.cs
- ConnectionPointCookie.cs
- DesignerTransaction.cs
- ToolStripSplitStackLayout.cs
- MenuItemCollection.cs
- TreeViewHitTestInfo.cs
- LineGeometry.cs
- nulltextcontainer.cs
- iisPickupDirectory.cs
- StyleModeStack.cs
- DataBoundControl.cs
- PkcsUtils.cs
- XamlVector3DCollectionSerializer.cs
- BooleanFacetDescriptionElement.cs
- BooleanAnimationBase.cs
- PageRequestManager.cs
- LineSegment.cs
- SoapObjectWriter.cs
- SpeakProgressEventArgs.cs
- Pts.cs
- Keywords.cs
- TypeForwardedToAttribute.cs
- ActiveXSite.cs
- WebPartVerbCollection.cs
- XmlDataDocument.cs
- ContextInformation.cs
- ImageMetadata.cs
- EntityWithChangeTrackerStrategy.cs
- SRGSCompiler.cs
- QualifiedCellIdBoolean.cs
- QilSortKey.cs
- XmlException.cs
- MouseActionConverter.cs
- DataSourceXmlClassAttribute.cs
- XmlKeywords.cs
- FloatUtil.cs
- _ScatterGatherBuffers.cs
- xmlsaver.cs
- ZipIOLocalFileHeader.cs
- ColorMap.cs
- RuleValidation.cs
- ScriptComponentDescriptor.cs
- WindowInteropHelper.cs
- EditorBrowsableAttribute.cs
- ReaderWriterLockWrapper.cs
- XamlVector3DCollectionSerializer.cs
- RelationshipSet.cs
- LocalizationParserHooks.cs
- UInt32Converter.cs
- XMLDiffLoader.cs
- TagPrefixInfo.cs
- DataGridItemAttachedStorage.cs
- Win32SafeHandles.cs
- WebPartEditorApplyVerb.cs
- FreeFormPanel.cs
- EditorPart.cs
- CodeObject.cs
- ModelUIElement3D.cs
- LZCodec.cs
- XamlPoint3DCollectionSerializer.cs
- RuleSettings.cs
- SafeBitVector32.cs
- GridViewPageEventArgs.cs
- ProfileParameter.cs
- SessionPageStatePersister.cs
- FigureParagraph.cs
- Point3DCollection.cs
- EventsTab.cs
- TemplateKey.cs
- iisPickupDirectory.cs
- ImagingCache.cs
- KeyEvent.cs
- CompensationHandlingFilter.cs
- UnionExpr.cs
- Trigger.cs
- DispatcherOperation.cs
- TypeConverterHelper.cs
- WsatAdminException.cs
- BitmapEffectGeneralTransform.cs
- PropertyKey.cs
- ResetableIterator.cs
- BaseTemplateCodeDomTreeGenerator.cs
- EntitySqlException.cs
- DocumentPageHost.cs
- OptionalColumn.cs
- BorderGapMaskConverter.cs
- SmtpNetworkElement.cs
- StylusPointProperty.cs
- Lease.cs
- GeometryGroup.cs
- XamlVector3DCollectionSerializer.cs
- XmlNodeReader.cs
- Site.cs