Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / Messaging / ActivationProxy.cs / 1 / ActivationProxy.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // Define the interfaces and infrastructure needed to send activation messages using System; using System.ServiceModel.Channels; using System.Diagnostics; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.ServiceModel; using System.Transactions; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.Messaging { abstract class ActivationProxy : RequestReplyProxy { public ActivationProxy(CoordinationService coordination, EndpointAddress to) : base (coordination, to) { } CreateCoordinationContextMessage CreateCreateCoordinationContextMessage(ref CreateCoordinationContext create) { CreateCoordinationContextMessage message = new CreateCoordinationContextMessage(this.messageVersion, ref create); if (create.IssuedToken != null) { CoordinationServiceSecurity.AddIssuedToken(message, create.IssuedToken); } return message; } public CreateCoordinationContextResponse SendCreateCoordinationContext (ref CreateCoordinationContext create) { if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Sending CreateCoordinationContext to {0}", this.to.Uri); } Message message = CreateCreateCoordinationContextMessage(ref create); Message reply = SendRequest(message, this.coordinationStrings.CreateCoordinationContextResponseAction); using (reply) { if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Dispatching CreateCoordinationContextResponse reply"); if (DebugTrace.Pii) DebugTrace.TracePii(TraceLevel.Verbose, "Sender is {0}", CoordinationServiceSecurity.GetSenderName(reply)); } return new CreateCoordinationContextResponse(reply, this.protocolVersion); } } public IAsyncResult BeginSendCreateCoordinationContext (ref CreateCoordinationContext create, AsyncCallback callback, object state) { if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Sending CreateCoordinationContext to {0}", this.to.Uri); } Message message = CreateCreateCoordinationContextMessage(ref create); return BeginSendRequest (message, callback, state); } public CreateCoordinationContextResponse EndSendCreateCoordinationContext (IAsyncResult ar) { try { Message reply = EndSendRequest(ar, this.coordinationStrings.CreateCoordinationContextResponseAction); using (reply) { if (DebugTrace.Verbose) { DebugTrace.Trace(TraceLevel.Verbose, "Dispatching CreateCoordinationContextResponse reply"); if (DebugTrace.Pii) DebugTrace.TracePii(TraceLevel.Verbose, "Sender is {0}", CoordinationServiceSecurity.GetSenderName(reply)); } return new CreateCoordinationContextResponse(reply, this.protocolVersion); } } catch (CommunicationException e) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new WsatReceiveFailureException(e)); } } public static void SendCreateCoordinationContextResponse (RequestAsyncResult result, ref CreateCoordinationContextResponse response) { Message message = new CreateCoordinationContextResponseMessage(result.MessageVersion, ref response); if (response.IssuedToken != null) { CoordinationServiceSecurity.AddIssuedToken(message, response.IssuedToken); } result.Finished (message); } public static void SendFaultResponse (RequestAsyncResult result, Fault fault) { Library.SendFaultResponse(result, fault); } } class InteropActivationProxy : ActivationProxy { public InteropActivationProxy(CoordinationService coordination, EndpointAddress to) : base (coordination, to) { } protected override IChannelFactorySelectChannelFactory(out MessageVersion messageVersion) { this.interoperating = true; messageVersion = this.coordinationService.InteropActivationBinding.MessageVersion; return this.coordinationService.InteropActivationChannelFactory; } } class WindowsActivationProxy : ActivationProxy { public WindowsActivationProxy(CoordinationService coordination, EndpointAddress to) : base(coordination, to) { } protected override IChannelFactory SelectChannelFactory(out MessageVersion messageVersion) { this.interoperating = false; EndpointIdentity identity = this.to.Identity; if (identity != null) { string claimType = identity.IdentityClaim.ClaimType; if (claimType != ClaimTypes.Spn && claimType != ClaimTypes.Upn) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new CreateChannelFailureException(SR.GetString(SR.InvalidTrustIdentityType, claimType))); } } string scheme = this.to.Uri.Scheme; if (string.Compare(scheme, Uri.UriSchemeNetPipe, StringComparison.OrdinalIgnoreCase) == 0) { messageVersion = this.coordinationService.NamedPipeActivationBinding.MessageVersion; return this.coordinationService.NamedPipeActivationChannelFactory; } else if (this.coordinationService.Config.RemoteClientsEnabled && string.Compare(scheme, Uri.UriSchemeHttps, StringComparison.OrdinalIgnoreCase) == 0) { messageVersion = this.coordinationService.WindowsActivationBinding.MessageVersion; return this.coordinationService.WindowsActivationChannelFactory; } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError( new CreateChannelFailureException(SR.GetString(SR.InvalidSchemeWithTrustIdentity, scheme))); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
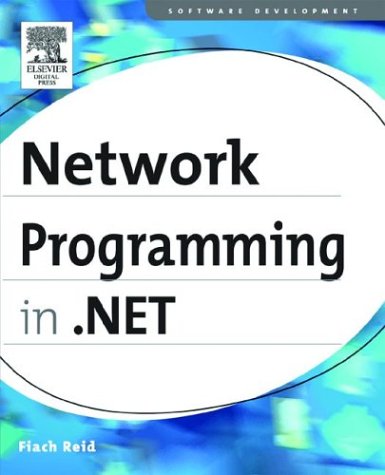
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NamedPermissionSet.cs
- ClipboardData.cs
- sqlstateclientmanager.cs
- FormViewAutoFormat.cs
- WebPartCollection.cs
- BuildResultCache.cs
- EventItfInfo.cs
- RegistryHandle.cs
- DataRowChangeEvent.cs
- UnSafeCharBuffer.cs
- DynamicValueConverter.cs
- AssemblyResourceLoader.cs
- SQLGuid.cs
- RectangleF.cs
- QilNode.cs
- ImageListStreamer.cs
- CTreeGenerator.cs
- PixelFormats.cs
- Cell.cs
- FlowLayoutPanelDesigner.cs
- RealProxy.cs
- Operators.cs
- NotifyCollectionChangedEventArgs.cs
- EtwTrace.cs
- TextLineResult.cs
- Rss20ItemFormatter.cs
- CreatingCookieEventArgs.cs
- BitSet.cs
- SqlParameterCollection.cs
- TextSegment.cs
- HandlerMappingMemo.cs
- CodeVariableDeclarationStatement.cs
- FileLoadException.cs
- CodeIdentifiers.cs
- FullTextLine.cs
- DrawingCollection.cs
- Registry.cs
- Calendar.cs
- CounterCreationDataCollection.cs
- DbConnectionHelper.cs
- WindowProviderWrapper.cs
- RequiredFieldValidator.cs
- MultiView.cs
- WebPartConnectionsConnectVerb.cs
- DataGridRowEventArgs.cs
- WebPartTransformerAttribute.cs
- CustomPopupPlacement.cs
- Part.cs
- PrintPreviewControl.cs
- BufferModesCollection.cs
- FieldInfo.cs
- LayoutEditorPart.cs
- DoubleConverter.cs
- AndAlso.cs
- DesignOnlyAttribute.cs
- ConfigXmlCDataSection.cs
- DefaultBinder.cs
- Tablet.cs
- MenuStrip.cs
- TreeNodeStyleCollection.cs
- TemplateKeyConverter.cs
- SqlBulkCopy.cs
- DesignerLoader.cs
- OdbcParameter.cs
- AudioFormatConverter.cs
- CommandCollectionEditor.cs
- CompensationExtension.cs
- Root.cs
- MenuItemCollectionEditor.cs
- MailAddress.cs
- EventSource.cs
- EmptyQuery.cs
- MaterialGroup.cs
- MdImport.cs
- NodeFunctions.cs
- FrameworkContentElement.cs
- ListViewDataItem.cs
- AnonymousIdentificationModule.cs
- configsystem.cs
- GeneralTransform3DGroup.cs
- SessionPageStateSection.cs
- LinqDataSourceDeleteEventArgs.cs
- Method.cs
- ProcessModuleCollection.cs
- UserControlBuildProvider.cs
- HttpHeaderCollection.cs
- ITextView.cs
- NamedPipeWorkerProcess.cs
- NativeActivityContext.cs
- PrimitiveXmlSerializers.cs
- LinqDataView.cs
- ToolbarAUtomationPeer.cs
- PropertyToken.cs
- BitmapFrameDecode.cs
- LoginNameDesigner.cs
- NeutralResourcesLanguageAttribute.cs
- XamlPoint3DCollectionSerializer.cs
- RayMeshGeometry3DHitTestResult.cs
- DBPropSet.cs
- NullableDecimalAverageAggregationOperator.cs