Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Wsat / StateMachines / TransactionContext.cs / 1 / TransactionContext.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // This file contains the the implementations of the various states used by // the transaction context state machine. // // This state machine exists to solve the rather unpleasant problems associated // with receiving CreateCoordinationContext w/ context messages in various circumstances. // // Most notably, we can't send a CCCR message to anyone before we know that we're // part of the distributed transaction. So if we're subordinate to a foreign TM, we can't // send anyone a CCCR until we have successfully registered for durable 2PC with our superior. // Since there's no such thing as a PPL EnlistPrepare, we have to handle the queueing of // requests ourselves. // // This state machine is driven by either a Coordinator state machine, a Subordinate state machine // or a Completion state machine. When our 'parent' state machine enters a terminal state, its // associated enlistment will send us a TransactionContextTransactionDoneEvent. That event will // make us transition to our own finished state, which will trigger the removal of our context manager // from the global lookup table. using Microsoft.Transactions.Wsat.Messaging; using Microsoft.Transactions.Wsat.Protocol; namespace Microsoft.Transactions.Wsat.StateMachines { class TransactionContextInitializing : InactiveState { public TransactionContextInitializing(ProtocolState state) : base (state) {} // Delivered when we receive a CCC w/ context message // and there's nothing in the transaction context lookup table public override void OnEvent(TransactionContextEnlistTransactionEvent e) { // Add the event to the queue and change states e.ContextManager.Requests.Enqueue(e); e.StateMachine.ChangeState(state.States.TransactionContextInitializingCoordinator); // Forward the event to a new coordinator state machine // It will eventually call us back when it decides that we're ready to answer CoordinatorEnlistment coordinator = new CoordinatorEnlistment(state, e.ContextManager, e.Body.CurrentContext, e.Body.IssuedToken); CreateCoordinationContext body = e.Body; MsgEnlistTransactionEvent newEvent = new MsgEnlistTransactionEvent(coordinator, ref body, e.Result); coordinator.StateMachine.Enqueue(newEvent); } // Delivered by the completion state machine public override void OnEvent(TransactionContextCreatedEvent e) { e.ContextManager.TransactionContext = e.TransactionContext; e.StateMachine.ChangeState(state.States.TransactionContextActive); } } class TransactionContextInitializingCoordinator : InactiveState { public TransactionContextInitializingCoordinator(ProtocolState state) : base(state) { } public override void OnEvent(TransactionContextEnlistTransactionEvent e) { e.ContextManager.Requests.Enqueue(e); } public override void OnEvent(TransactionContextCreatedEvent e) { e.ContextManager.TransactionContext = e.TransactionContext; e.StateMachine.ChangeState(state.States.TransactionContextActive); } public override void OnEvent(TransactionContextTransactionDoneEvent e) { e.StateMachine.ChangeState(state.States.TransactionContextFinished); } } class TransactionContextActive : InactiveState { public TransactionContextActive(ProtocolState state) : base(state) { } public override void Enter(StateMachine stateMachine) { base.Enter(stateMachine); TransactionContextStateMachine contextStateMachine = (TransactionContextStateMachine)stateMachine; TransactionContextManager contextManager = contextStateMachine.ContextManager; foreach (TransactionContextEnlistTransactionEvent e in contextManager.Requests) { state.ActivationCoordinator.SendCreateCoordinationContextResponse(contextManager.TransactionContext, e.Result); } contextManager.Requests.Clear(); } public override void OnEvent(TransactionContextEnlistTransactionEvent e) { state.ActivationCoordinator.SendCreateCoordinationContextResponse(e.ContextManager.TransactionContext, e.Result); } public override void OnEvent(TransactionContextTransactionDoneEvent e) { e.StateMachine.ChangeState(state.States.TransactionContextFinished); } } class TransactionContextFinished : TerminalState { public TransactionContextFinished(ProtocolState state) : base(state) { } public override void Enter(StateMachine stateMachine) { base.Enter(stateMachine); TransactionContextStateMachine contextStateMachine = (TransactionContextStateMachine)stateMachine; TransactionContextManager contextManager = contextStateMachine.ContextManager; Fault fault = contextManager.Fault; foreach (TransactionContextEnlistTransactionEvent e in contextManager.Requests) { state.ActivationCoordinator.SendFault(e.Result, fault); } contextManager.Requests.Clear(); } public override void OnEvent(TransactionContextEnlistTransactionEvent e) { state.ActivationCoordinator.SendFault(e.Result, e.ContextManager.Fault); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
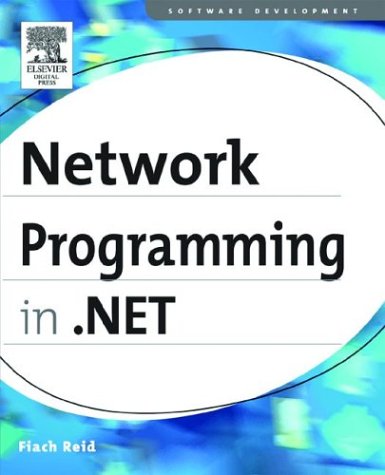
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LightweightCodeGenerator.cs
- PageFunction.cs
- WebPartConnectionsConnectVerb.cs
- HeaderUtility.cs
- LineGeometry.cs
- storepermission.cs
- DetailsViewCommandEventArgs.cs
- ChangePassword.cs
- RadioButtonList.cs
- QueryInterceptorAttribute.cs
- WaitHandleCannotBeOpenedException.cs
- SplineKeyFrames.cs
- ElementAction.cs
- ExplicitDiscriminatorMap.cs
- ResizeBehavior.cs
- DetailsViewInsertedEventArgs.cs
- IIS7UserPrincipal.cs
- TreeNodeBinding.cs
- RecognizedAudio.cs
- ForwardPositionQuery.cs
- HttpCachePolicyElement.cs
- ChangePassword.cs
- NumericUpDown.cs
- UrlMappingsModule.cs
- HttpListenerResponse.cs
- WindowsStartMenu.cs
- _HTTPDateParse.cs
- Listbox.cs
- WindowVisualStateTracker.cs
- MissingMemberException.cs
- TextDecoration.cs
- Marshal.cs
- altserialization.cs
- XmlSerializerOperationFormatter.cs
- ProviderConnectionPointCollection.cs
- BasicHttpSecurity.cs
- IsolationInterop.cs
- CalendarDay.cs
- IEnumerable.cs
- DataSourceGroupCollection.cs
- diagnosticsswitches.cs
- ProcessInputEventArgs.cs
- ThrowHelper.cs
- ProtocolViolationException.cs
- SafeBitVector32.cs
- DelegateSerializationHolder.cs
- DataSourceXmlSubItemAttribute.cs
- ConsumerConnectionPoint.cs
- ClientScriptManagerWrapper.cs
- NetworkInformationException.cs
- SafeEventLogWriteHandle.cs
- ApplicationProxyInternal.cs
- oledbconnectionstring.cs
- SystemWebCachingSectionGroup.cs
- PointAnimationBase.cs
- TextEffectCollection.cs
- SqlTriggerContext.cs
- DetailsViewDesigner.cs
- StorageScalarPropertyMapping.cs
- dsa.cs
- Substitution.cs
- FullTextState.cs
- SortFieldComparer.cs
- TemplatedMailWebEventProvider.cs
- PasswordRecovery.cs
- CapabilitiesSection.cs
- DecoderFallbackWithFailureFlag.cs
- _PooledStream.cs
- XmlSchemaComplexContentRestriction.cs
- AggregateNode.cs
- GiveFeedbackEventArgs.cs
- FontWeights.cs
- MenuAutoFormat.cs
- ShutDownListener.cs
- SqlTypesSchemaImporter.cs
- HtmlHistory.cs
- MultiDataTrigger.cs
- CapabilitiesAssignment.cs
- QueryExpr.cs
- DirectoryInfo.cs
- RectAnimationUsingKeyFrames.cs
- SqlCharStream.cs
- mda.cs
- OleDbParameterCollection.cs
- StrokeCollection.cs
- HMACSHA512.cs
- HwndKeyboardInputProvider.cs
- XmlSchemaComplexContentExtension.cs
- EncoderNLS.cs
- DocumentGridContextMenu.cs
- ParseNumbers.cs
- SQLInt32.cs
- SafeArrayRankMismatchException.cs
- ServiceOperationListItemList.cs
- SourceChangedEventArgs.cs
- DirectoryInfo.cs
- RuleInfoComparer.cs
- ListMarkerSourceInfo.cs
- Inline.cs
- ArrangedElementCollection.cs