Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / ImportRequest.cs / 1 / ImportRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Security.Principal; using System.Diagnostics; using System.Threading; //ManualResetEvent using System.ComponentModel; //Win32Exception using System.IO; //Stream using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using Microsoft.InfoCards.Diagnostics; // // This class is an instance of a request to import a card from a file. // It starts up the UI in the 'Import' mode. // class ImportRequest : ClientUIRequest { FileStream m_importFile; //keep a read pointer to the file string m_filename; public ImportRequest( Process callingProcess, WindowsIdentity callingIdentity, InfoCardUIAgent uiAgent, IntPtr rpcHandle, Stream inArgs, Stream outArgs ) : base( callingProcess, callingIdentity, uiAgent, rpcHandle, inArgs, outArgs, InfoCardUIAgent.CallMode.Import, ExceptionList.AllNonFatal ) { } public string ImportedFile { get{ return m_filename; } } // // No input args // protected override void OnMarshalInArgs() { BinaryReader breader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); string filename = Utility.DeserializeString( breader ); if (String.IsNullOrEmpty(filename) || filename.Length > Constants.Maxima.FileNameLength) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFileName ) ) ); } if( !Path.IsPathRooted( filename ) ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.InvalidImportFileName) ) ); } try { m_filename = filename; m_importFile = new FileStream( m_filename, FileMode.Open, FileAccess.Read, FileShare.Read ); } catch( ArgumentException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( IOException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( NotSupportedException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } catch( UnauthorizedAccessException e ) { throw IDT.ThrowHelperError( new ImportException( SR.GetString( SR.CannotOpenImportFile ), e ) ); } } protected override void OnProcess() { // // ClientUiRequest's member function // StartAndWaitForUIAgent(); } // // No Output args. // protected override void OnMarshalOutArgs() { } protected override void OnDisposeAsUser() { base.OnDisposeAsUser(); if( null != m_importFile ) { m_importFile.Dispose(); m_importFile = null; } } // // Summary // Gets called a) if there was an exception in client processing // or b) if there was an exception in the child's request processing // protected override bool OnHandleException( Exception e, out int errorCode ) { errorCode = 0; bool handled = false; if( e is UserCancelledException ) { errorCode = (e as UserCancelledException).NativeHResult; handled = true; } return handled; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
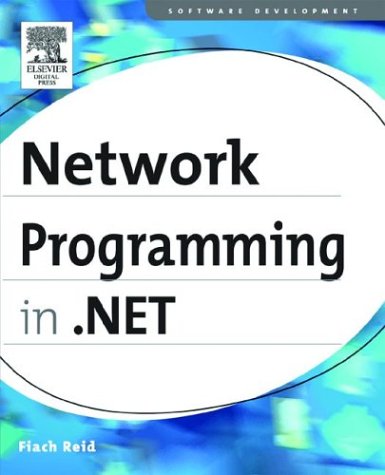
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeAttributeDeclaration.cs
- DataGridViewColumn.cs
- GridViewSortEventArgs.cs
- ConfigurationPropertyCollection.cs
- RuntimeHandles.cs
- ObjectCacheHost.cs
- HtmlInputCheckBox.cs
- ObjectConverter.cs
- XamlSerializerUtil.cs
- SmiSettersStream.cs
- ChangePasswordAutoFormat.cs
- ConfigurationElementCollection.cs
- TableItemStyle.cs
- FieldCollectionEditor.cs
- Rect3D.cs
- CompoundFileIOPermission.cs
- UpDownBaseDesigner.cs
- DescendantOverDescendantQuery.cs
- ClientCredentialsElement.cs
- SqlNode.cs
- OpenTypeCommon.cs
- Connector.cs
- DecimalAnimation.cs
- QuotaThrottle.cs
- XXXInfos.cs
- ExponentialEase.cs
- StringAttributeCollection.cs
- MarshalDirectiveException.cs
- FilteredXmlReader.cs
- XmlILTrace.cs
- WebPartDescription.cs
- ContainsRowNumberChecker.cs
- LoginCancelEventArgs.cs
- SchemaNames.cs
- WebPartEditorOkVerb.cs
- VectorCollectionValueSerializer.cs
- UserControlCodeDomTreeGenerator.cs
- SmiConnection.cs
- FlowchartStart.xaml.cs
- RegexCaptureCollection.cs
- InputLanguageEventArgs.cs
- CrossAppDomainChannel.cs
- AvTraceFormat.cs
- ScrollChrome.cs
- ThumbAutomationPeer.cs
- CompiledAction.cs
- InvalidDataException.cs
- RijndaelManagedTransform.cs
- SecurityKeyUsage.cs
- CheckBoxFlatAdapter.cs
- XmlCharacterData.cs
- SpeechRecognitionEngine.cs
- NestedContainer.cs
- Splitter.cs
- SmiMetaDataProperty.cs
- WinEventTracker.cs
- SocketException.cs
- XmlChildNodes.cs
- SqlConnectionManager.cs
- OracleInfoMessageEventArgs.cs
- EditingMode.cs
- CopyCodeAction.cs
- keycontainerpermission.cs
- DocumentSequenceHighlightLayer.cs
- ImageAnimator.cs
- TextDecoration.cs
- ReadOnlyNameValueCollection.cs
- HttpProfileGroupBase.cs
- Control.cs
- SimpleType.cs
- GifBitmapEncoder.cs
- SmiRequestExecutor.cs
- EdmToObjectNamespaceMap.cs
- _SpnDictionary.cs
- ObjectListItemCollection.cs
- Mapping.cs
- GreenMethods.cs
- InvalidateEvent.cs
- ClockGroup.cs
- updateconfighost.cs
- Trace.cs
- ListViewContainer.cs
- SettingsAttributes.cs
- XpsPackagingException.cs
- XmlValidatingReaderImpl.cs
- SQLInt32Storage.cs
- BackgroundFormatInfo.cs
- ReaderWriterLockWrapper.cs
- HttpHandler.cs
- QueryOperationResponseOfT.cs
- RoutedEvent.cs
- XmlWrappingWriter.cs
- CacheOutputQuery.cs
- SpeechSynthesizer.cs
- ActivityXRefConverter.cs
- TextLineResult.cs
- WebServiceHandlerFactory.cs
- ImageListImage.cs
- DesigntimeLicenseContextSerializer.cs
- WebAdminConfigurationHelper.cs