Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RecipientIdentity.cs / 2 / RecipientIdentity.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Diagnostics; using System.Globalization; using System.ServiceModel; using System.Security.Cryptography.X509Certificates; using System.IdentityModel.Tokens; using System.Security.Cryptography; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // This class is the base class for a recipient identity. The base identity is DNS. // internal class RecipientIdentity { EndpointAddress m_address; string m_identifier = string.Empty; string m_organizationIdentifier = string.Empty; string m_organizationPPIDIdentifier = string.Empty; bool m_hasBeenValidated = false; public static RecipientIdentity CreateIdentity( EndpointAddress epr, bool validate ) { if( epr.Identity is X509CertificateEndpointIdentity ) { if( null == ( (X509CertificateEndpointIdentity)epr.Identity ).Certificates || ( (X509CertificateEndpointIdentity)epr.Identity ).Certificates.Count < 1 ) { throw IDT.ThrowHelperError( new PolicyValidationException() ); } RecipientIdentity id = new X509RecipientIdentity( epr, ( (X509CertificateEndpointIdentity)epr.Identity ).Certificates ); if( validate ) { id.Validate(); } return id; } else if( null == epr.Identity ) { RecipientIdentity id = new RecipientIdentity( epr ); if( validate ) { id.Validate(); } return id; } else { throw IDT.ThrowHelperError( new IdentityValidationException( SR.GetString( SR.UnsupportedIdentityType ) ) ); } } public RecipientIdentity( EndpointAddress epr ) { m_address = epr; } public EndpointAddress Address { get { return m_address; } } protected bool HasBeenValidated { set { m_hasBeenValidated = value; } get { return m_hasBeenValidated; } } protected string Identifier { set { m_identifier = value; } } protected string OrganizationIdentifier { set { m_organizationIdentifier = value; } } protected string OrganizationPPIDIdentifier { set { m_organizationPPIDIdentifier = value; } } public virtual void Validate() { if( !m_hasBeenValidated ) { using( SHA256 sha = new SHA256Managed() ) { m_identifier = Convert.ToBase64String( sha.ComputeHash( Encoding.Unicode.GetBytes( m_address.Uri.Host ) ) ); m_organizationIdentifier = m_identifier; m_organizationPPIDIdentifier = m_identifier; } m_hasBeenValidated = true; } } public virtual string GetIdentifier() { IDT.Assert( HasBeenValidated, "Identity has not been validated" ); return m_identifier; } public virtual string GetOrganizationIdentifier() { IDT.Assert( HasBeenValidated, "Identity has not been validated" ); return m_organizationIdentifier; } public virtual string GetOrganizationPPIDIdentifier() { IDT.Assert( HasBeenValidated, "Identity has not been validated" ); return m_organizationPPIDIdentifier; } public virtual string GetName() { return m_address.Uri.Host; } } // // Summary // This class represents an X509 based recipient identity. // internal class X509RecipientIdentity : RecipientIdentity { X509Certificate2Collection m_certificates; Recipient.RecipientCertParameters m_recipientParams; bool isChainTrusted = false; public Recipient.RecipientCertParameters RecipientParams { get { return m_recipientParams; } } public X509Certificate2 LeafCertificate { get { return m_certificates[ 0 ]; } } public X509RecipientIdentity( EndpointAddress epr, X509Certificate2Collection certificates ) : base( epr ) { m_certificates = certificates; } public override void Validate() { if( !HasBeenValidated ) { isChainTrusted = ValidateCertificate(); Identifier = Microsoft.InfoCards.Recipient.CertGetRecipientIdHash( LeafCertificate, m_certificates, isChainTrusted, out m_recipientParams ); OrganizationIdentifier = Microsoft.InfoCards.Recipient.CertGetRecipientOrganizationIdHash( LeafCertificate, m_certificates, ValidateCertificate() ); OrganizationPPIDIdentifier = Microsoft.InfoCards.Recipient.CertGetRecipientOrganizationPPIDSeedHash( LeafCertificate, m_certificates, ValidateCertificate() ); HasBeenValidated = true; } } public override string GetName() { string name = LeafCertificate.FriendlyName; if( string.IsNullOrEmpty( name ) ) { name = LeafCertificate.GetNameInfo( X509NameType.SimpleName, false ); } return name; } // // Summary: // Verify that the chain for the RP certificate is trusted. // Either machine or user roots are ok. // If not, try peer trust. // // Returns // True if the recipient is chain trusted // private bool ValidateCertificate() { bool isCertChainTrusted = false; try { InfoCardX509Validator.ValidateChainOrPeer( LeafCertificate, m_certificates, out isCertChainTrusted ); } catch( SecurityTokenValidationException validationE ) { throw IDT.ThrowHelperError( new IdentityValidationException( SR.GetString( SR.RecipientCertificateNotValid ), validationE ) ); } return isCertChainTrusted; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
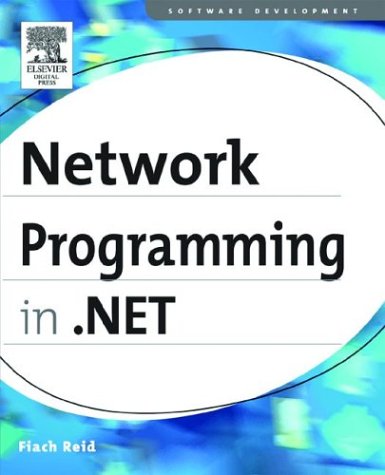
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DetailsViewUpdatedEventArgs.cs
- ItemList.cs
- SocketElement.cs
- TextRange.cs
- ToolBarDesigner.cs
- BindingsCollection.cs
- TabControlCancelEvent.cs
- GlyphCollection.cs
- DataRowComparer.cs
- SpnegoTokenAuthenticator.cs
- DbConnectionClosed.cs
- InvalidDataException.cs
- UidManager.cs
- BrowserCapabilitiesFactory.cs
- ProxyManager.cs
- securitycriticaldataClass.cs
- TextParagraph.cs
- Vector3D.cs
- Rijndael.cs
- RC2.cs
- CodeExporter.cs
- Compiler.cs
- EpmCustomContentWriterNodeData.cs
- CqlLexer.cs
- GlobalEventManager.cs
- GenerateDerivedKeyRequest.cs
- QueueAccessMode.cs
- SettingsPropertyNotFoundException.cs
- ToolTipService.cs
- RefreshEventArgs.cs
- BaseDataList.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- BehaviorEditorPart.cs
- DynamicScriptObject.cs
- WebBrowsableAttribute.cs
- LocalizationParserHooks.cs
- SecuritySessionFilter.cs
- MatrixAnimationUsingPath.cs
- _AutoWebProxyScriptEngine.cs
- SmtpException.cs
- CreateParams.cs
- SqlDependency.cs
- DuplicateMessageDetector.cs
- XmlKeywords.cs
- FastEncoder.cs
- SqlFileStream.cs
- PropertyBuilder.cs
- TextFragmentEngine.cs
- XmlDocumentType.cs
- SelectionWordBreaker.cs
- isolationinterop.cs
- ResponseStream.cs
- RijndaelManagedTransform.cs
- RowBinding.cs
- ExceptionRoutedEventArgs.cs
- ToolStripDropDownMenu.cs
- StringArrayConverter.cs
- WindowsSlider.cs
- SelectionItemPatternIdentifiers.cs
- CollectionEditorDialog.cs
- DateTimeSerializationSection.cs
- CompoundFileStreamReference.cs
- CustomAttribute.cs
- MeshGeometry3D.cs
- FlowSwitch.cs
- StringPropertyBuilder.cs
- InheritablePropertyChangeInfo.cs
- XmlSchemaResource.cs
- DataBindingHandlerAttribute.cs
- Ports.cs
- ColorIndependentAnimationStorage.cs
- TimeSpanMinutesConverter.cs
- NameValueCollection.cs
- SizeAnimationBase.cs
- UnsignedPublishLicense.cs
- ClientOptions.cs
- ContentPropertyAttribute.cs
- PassportAuthenticationModule.cs
- PngBitmapEncoder.cs
- IBuiltInEvidence.cs
- WebPartZoneCollection.cs
- SqlWebEventProvider.cs
- PassportAuthenticationEventArgs.cs
- CatalogPartChrome.cs
- Exceptions.cs
- FontWeightConverter.cs
- WriteableOnDemandStream.cs
- DependencyProperty.cs
- CreatingCookieEventArgs.cs
- Sentence.cs
- PrintingPermission.cs
- SecUtil.cs
- RelatedPropertyManager.cs
- Peer.cs
- ClientBuildManager.cs
- ThrowHelper.cs
- AncestorChangedEventArgs.cs
- XmlEncoding.cs
- Privilege.cs
- StorageComplexTypeMapping.cs