Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RpcCryptoContext.cs / 1 / RpcCryptoContext.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.ComponentModel; using System.IO; using System.Runtime.InteropServices; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // The context class for perfoming RpcCrypto operations. // internal class RpcCryptoContext : IDisposable { string m_portName; string m_contextKey; IntPtr m_idlHandle; object m_syncRoot; bool m_open; bool m_disposed; // // Summary: // Creates a new RpcCryptoContext // // Arguments: // portName: The name of the port // contextKey: The name of the context // public RpcCryptoContext( string portName, string contextKey ) { m_portName = portName; m_contextKey = contextKey; m_syncRoot = new object(); m_disposed = false; } // // Summary: // DTOR // ~RpcCryptoContext() { if( !m_disposed ) { ((IDisposable)this).Dispose(); } } // // Summary: // Gets the ContextKye. // public string ContextKey { get{ return m_contextKey; } } // // Summary: // Gets the native IdlHandle // public IntPtr InterfaceHandle { get{ return m_idlHandle; } } // // Summary: // Indicates if the context is still open. // public bool IsOpen { get{ return m_open; } } // // Summary: // Opens the context. // public void Open() { ThrowIfDisposed(); if( !m_open ) { try { m_idlHandle = NativeMcppMethods.RpcCryptoOpen( m_portName ); } catch( Win32Exception we ) { throw IDT.ThrowHelperError( new CommunicationException( null, we ) ); } m_open = true; } } // // Summary: // Closes the context. // public void Close() { if( m_open ) { try { NativeMcppMethods.RpcCryptoClose( m_idlHandle, m_contextKey ); } catch( Win32Exception we ) { throw IDT.ThrowHelperError( new CommunicationException( null, we ) ); } m_idlHandle = IntPtr.Zero; m_open = false; } } // // Summary: // Disposes the object if not alreay done. // void IDisposable.Dispose() { if( !m_disposed ) { lock( m_syncRoot ) { if( !m_disposed ) { m_disposed = true; try { Close(); } catch( CommunicationException ie ) { // // In case the RPC interface on the agent had already shutdown, // we will get this exception from Close( ) above. Dispose is NOT // expected to throw, so catch this specific exception here. // string msg = ie.Message; IDT.TraceDebug( "Ignoring failure in RpcCryptoContext Close(): " + msg ); } m_portName = null; m_contextKey = null; m_idlHandle = IntPtr.Zero; GC.SuppressFinalize( this ); } } } } void ThrowIfDisposed() { if( m_disposed ) { throw IDT.ThrowHelperError( new ObjectDisposedException( "RpcCryptoContext" ) ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
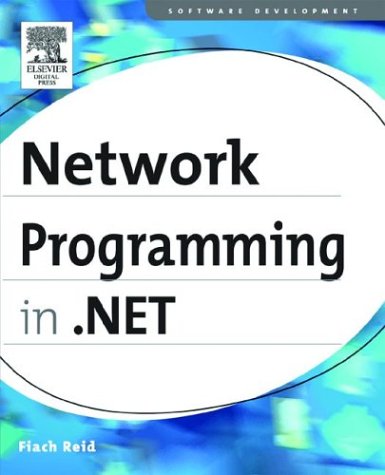
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrintingPermissionAttribute.cs
- webclient.cs
- ProfileModule.cs
- InputMethodStateChangeEventArgs.cs
- PropagatorResult.cs
- OdbcUtils.cs
- StrokeCollectionConverter.cs
- WmlCommandAdapter.cs
- SchemaNotation.cs
- X509ChainElement.cs
- ExpanderAutomationPeer.cs
- Int32Rect.cs
- Evidence.cs
- ValidationPropertyAttribute.cs
- Quad.cs
- KernelTypeValidation.cs
- ZipIOModeEnforcingStream.cs
- AutoResizedEvent.cs
- PropertyRecord.cs
- M3DUtil.cs
- TransformerTypeCollection.cs
- Bits.cs
- GridViewDeletedEventArgs.cs
- ServiceAuthorizationManager.cs
- GZipStream.cs
- CompositeControl.cs
- XmlIlVisitor.cs
- SafeCertificateStore.cs
- SchemaImporterExtensionElementCollection.cs
- ConfigXmlText.cs
- RSAOAEPKeyExchangeFormatter.cs
- XPathNavigatorKeyComparer.cs
- AssociationTypeEmitter.cs
- FreeFormPanel.cs
- PreDigestedSignedInfo.cs
- XmlChildEnumerator.cs
- CodeNamespaceImportCollection.cs
- ContainsRowNumberChecker.cs
- SqlParameterCollection.cs
- RenderTargetBitmap.cs
- NativeWrapper.cs
- ReliableMessagingHelpers.cs
- PointLight.cs
- PreservationFileWriter.cs
- FontFamilyConverter.cs
- XmlSchemaObjectTable.cs
- ConnectionInterfaceCollection.cs
- SecurityKeyIdentifierClause.cs
- BufferedGraphicsContext.cs
- TimeSpanStorage.cs
- SafePEFileHandle.cs
- GenericUriParser.cs
- DataContractSerializerElement.cs
- RadioButton.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- AutoCompleteStringCollection.cs
- MetaModel.cs
- HandleCollector.cs
- EncodingTable.cs
- PolicyUnit.cs
- QueryInterceptorAttribute.cs
- QilReplaceVisitor.cs
- RetrieveVirtualItemEventArgs.cs
- MemoryPressure.cs
- ShaderEffect.cs
- sqlnorm.cs
- HtmlInputReset.cs
- SearchForVirtualItemEventArgs.cs
- ISessionStateStore.cs
- BitStack.cs
- TimersDescriptionAttribute.cs
- PowerModeChangedEventArgs.cs
- dataprotectionpermission.cs
- EventHandlerList.cs
- ArithmeticException.cs
- FunctionQuery.cs
- Permission.cs
- ProfileGroupSettingsCollection.cs
- DatatypeImplementation.cs
- Events.cs
- odbcmetadatacolumnnames.cs
- MetadataCache.cs
- DbDataSourceEnumerator.cs
- DataRecord.cs
- SchemeSettingElementCollection.cs
- OpacityConverter.cs
- InvalidContentTypeException.cs
- WizardDesigner.cs
- LiteralControl.cs
- OleDbStruct.cs
- SystemIcmpV6Statistics.cs
- UInt16Converter.cs
- XmlUtil.cs
- LineVisual.cs
- Literal.cs
- Rules.cs
- PropertyInformationCollection.cs
- CommandDevice.cs
- SystemIcmpV6Statistics.cs
- ThreadPool.cs