Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / Metadata / AttributeCallbackBuilder.cs / 1305376 / AttributeCallbackBuilder.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Metadata { using System.Runtime; using System.Activities.Presentation.Internal.Metadata; using System.Activities.Presentation.Internal.Properties; using System; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.Windows; using System.Activities.Presentation; //// An instance of this class is passed to callback delegates to lazily // populate the attributes for a type. // [Fx.Tag.XamlVisible(false)] public sealed class AttributeCallbackBuilder { private MutableAttributeTable _table; private Type _callbackType; internal AttributeCallbackBuilder(MutableAttributeTable table, Type callbackType) { _table = table; _callbackType = callbackType; } //// The type this callback is being invoked for. // public Type CallbackType { get { return _callbackType; } } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // // The new attributes to add. // //if type or attributes is null public void AddCustomAttributes(params Attribute[] attributes) { if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, attributes); } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // An event or property descriptor to add attributes to. // // The new attributes to add. // //if descriptor or attributes is null public void AddCustomAttributes(MemberDescriptor descriptor, params Attribute[] attributes) { if (descriptor == null) { throw FxTrace.Exception.ArgumentNull("descriptor"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, descriptor, attributes); } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // An event or property info to add attributes to. // // The new attributes to add. // //if member or attributes is null public void AddCustomAttributes(MemberInfo member, params Attribute[] attributes) { if (member == null) { throw FxTrace.Exception.ArgumentNull("member"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, member, attributes); } //// Adds attributes to the member with the given name. The member can be a property // or an event. The member is evaluated on demand when the user queries // attributes on a given property or event. // // // The member to add attributes for. Only property and event members are supported; // all others will be ignored. // // // The new attributes to add. // public void AddCustomAttributes(string memberName, params Attribute[] attributes) { if (memberName == null) { throw FxTrace.Exception.ArgumentNull("memberName"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, memberName, attributes); } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // A dependency property to add attributes to. // // The new attributes to add. // //if dp or attributes is null public void AddCustomAttributes(DependencyProperty dp, params Attribute[] attributes) { if (dp == null) { throw FxTrace.Exception.ArgumentNull("dp"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, dp, attributes); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Metadata { using System.Runtime; using System.Activities.Presentation.Internal.Metadata; using System.Activities.Presentation.Internal.Properties; using System; using System.ComponentModel; using System.Globalization; using System.Reflection; using System.Windows; using System.Activities.Presentation; //// An instance of this class is passed to callback delegates to lazily // populate the attributes for a type. // [Fx.Tag.XamlVisible(false)] public sealed class AttributeCallbackBuilder { private MutableAttributeTable _table; private Type _callbackType; internal AttributeCallbackBuilder(MutableAttributeTable table, Type callbackType) { _table = table; _callbackType = callbackType; } //// The type this callback is being invoked for. // public Type CallbackType { get { return _callbackType; } } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // // The new attributes to add. // //if type or attributes is null public void AddCustomAttributes(params Attribute[] attributes) { if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, attributes); } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // An event or property descriptor to add attributes to. // // The new attributes to add. // //if descriptor or attributes is null public void AddCustomAttributes(MemberDescriptor descriptor, params Attribute[] attributes) { if (descriptor == null) { throw FxTrace.Exception.ArgumentNull("descriptor"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, descriptor, attributes); } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // An event or property info to add attributes to. // // The new attributes to add. // //if member or attributes is null public void AddCustomAttributes(MemberInfo member, params Attribute[] attributes) { if (member == null) { throw FxTrace.Exception.ArgumentNull("member"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, member, attributes); } //// Adds attributes to the member with the given name. The member can be a property // or an event. The member is evaluated on demand when the user queries // attributes on a given property or event. // // // The member to add attributes for. Only property and event members are supported; // all others will be ignored. // // // The new attributes to add. // public void AddCustomAttributes(string memberName, params Attribute[] attributes) { if (memberName == null) { throw FxTrace.Exception.ArgumentNull("memberName"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, memberName, attributes); } //// Adds the contents of the provided attributes to this builder. // Conflicts are resolved with a last-in-wins strategy. // // A dependency property to add attributes to. // // The new attributes to add. // //if dp or attributes is null public void AddCustomAttributes(DependencyProperty dp, params Attribute[] attributes) { if (dp == null) { throw FxTrace.Exception.ArgumentNull("dp"); } if (attributes == null) { throw FxTrace.Exception.ArgumentNull("attributes"); } _table.AddCustomAttributes(_callbackType, dp, attributes); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
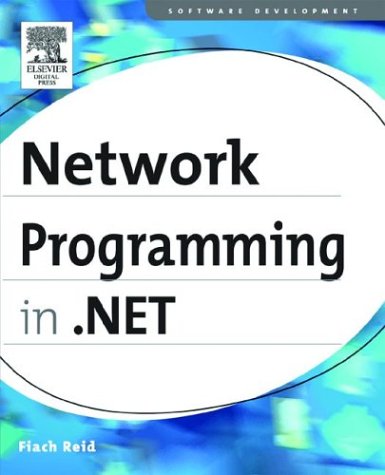
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DBConcurrencyException.cs
- filewebresponse.cs
- SpeechAudioFormatInfo.cs
- SqlCommandSet.cs
- ShellProvider.cs
- NotificationContext.cs
- ErrorHandlerModule.cs
- LocatorPartList.cs
- FixedDocument.cs
- Helpers.cs
- ButtonChrome.cs
- safex509handles.cs
- Italic.cs
- ObjectStorage.cs
- MeshGeometry3D.cs
- CanonicalXml.cs
- FunctionMappingTranslator.cs
- SqlProfileProvider.cs
- Menu.cs
- InvalidOperationException.cs
- UnSafeCharBuffer.cs
- HtmlContainerControl.cs
- AccessKeyManager.cs
- JavaScriptObjectDeserializer.cs
- Italic.cs
- EditorPartCollection.cs
- XmlImplementation.cs
- Funcletizer.cs
- VersionValidator.cs
- CustomError.cs
- TextSelectionProcessor.cs
- CounterSetInstanceCounterDataSet.cs
- SortExpressionBuilder.cs
- MouseEvent.cs
- ResourcesBuildProvider.cs
- ReadOnlyCollection.cs
- WindowsAuthenticationModule.cs
- WarningException.cs
- DisableDpiAwarenessAttribute.cs
- PointHitTestParameters.cs
- HwndProxyElementProvider.cs
- InvokePattern.cs
- InstanceDescriptor.cs
- RuleElement.cs
- DoubleCollectionValueSerializer.cs
- RowUpdatedEventArgs.cs
- EditModeSwitchButton.cs
- ResXBuildProvider.cs
- TypedTableBase.cs
- RectAnimationBase.cs
- PropertyMap.cs
- Pen.cs
- SafeNativeMethodsMilCoreApi.cs
- WorkflowElementDialog.cs
- ProcessInfo.cs
- OleDbPermission.cs
- PropertyToken.cs
- COM2PictureConverter.cs
- PerformanceCounter.cs
- PropVariant.cs
- MsmqEncryptionAlgorithm.cs
- DataControlImageButton.cs
- SurrogateEncoder.cs
- SafeNativeMethods.cs
- ComAwareEventInfo.cs
- EditingCoordinator.cs
- GenericNameHandler.cs
- BaseHashHelper.cs
- CodeMemberEvent.cs
- WebExceptionStatus.cs
- ViewStateException.cs
- WebUtil.cs
- DataGridViewAccessibleObject.cs
- OleDbSchemaGuid.cs
- Transform3D.cs
- ChangeProcessor.cs
- CompareValidator.cs
- RSAPKCS1SignatureFormatter.cs
- TableRowsCollectionEditor.cs
- CheckBoxFlatAdapter.cs
- TabPageDesigner.cs
- FormViewPagerRow.cs
- DataSourceProvider.cs
- TreeViewHitTestInfo.cs
- DataServiceRequestOfT.cs
- DataServiceException.cs
- AccessDataSourceWizardForm.cs
- SHA512.cs
- XmlMapping.cs
- SafeFindHandle.cs
- HttpWriter.cs
- CompilationUtil.cs
- ForceCopyBuildProvider.cs
- PhoneCall.cs
- InputReferenceExpression.cs
- CultureTableRecord.cs
- BitmapEffectInput.cs
- SortKey.cs
- Contracts.cs
- EncodingDataItem.cs