Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Helpers.cs / 1 / Helpers.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.IO; using System.Globalization; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal { internal static class Helpers { //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods // Disable parameter validation check #pragma warning disable 56507 // Throws exception if the specified Rule does not have a valid Id. static internal void ThrowIfEmptyOrNull (string s, string paramName) { if (string.IsNullOrEmpty (s)) { if (s == null) { throw new ArgumentNullException (paramName); } else { throw new ArgumentException (SR.Get (SRID.StringCanNotBeEmpty, paramName), paramName); } } } #pragma warning restore 56507 // Throws exception if the specified Rule does not have a valid Id. static internal void ThrowIfNull (object value, string paramName) { if (value == null) { throw new ArgumentNullException (paramName); } } static internal bool CompareInvariantCulture (CultureInfo culture1, CultureInfo culture2) { // If perfect match easy if (culture1.Equals (culture2)) { return true; } // Compare the Neutral culture while (!culture1.IsNeutralCulture) { culture1 = culture1.Parent; } while (!culture2.IsNeutralCulture) { culture2 = culture2.Parent; } return culture1.Equals (culture2); } // Copy the input cfg to the output. // Streams point to the start of the data on entry and to the end on exit static internal void CopyStream (Stream inputStream, Stream outputStream, int bytesToCopy) { // Copy using an intermediate buffer of a reasonable size. int bufferSize = bytesToCopy > 4096 ? 4096 : bytesToCopy; byte [] buffer = new byte [bufferSize]; int bytesRead; while (bytesToCopy > 0) { bytesRead = inputStream.Read (buffer, 0, bufferSize); if (bytesRead <= 0) { throw new EndOfStreamException (SR.Get (SRID.StreamEndedUnexpectedly)); } outputStream.Write (buffer, 0, bytesRead); bytesToCopy -= bytesRead; } } // Copy the input cfg to the output. // inputStream points to the start of the data on entry and to the end on exit static internal byte [] ReadStreamToByteArray (Stream inputStream, int bytesToCopy) { byte [] outputArray = new byte [bytesToCopy]; BlockingRead (inputStream, outputArray, 0, bytesToCopy); return outputArray; } static internal void BlockingRead (Stream stream, byte [] buffer, int offset, int count) { // Stream is not like IStream - it will block until some data is available but not necessarily all of it. while (count > 0) { int read = stream.Read (buffer, offset, count); if (read <= 0) // End of stream { throw new EndOfStreamException (); } count -= read; offset += read; } } ////// Combine 2 cultures into in a composite one and assign a keyboardLayoutId /// Language /// ---- /// /// /// static internal void CombineCulture (string language, string location, CultureInfo parentCulture, int layoutId) { CultureInfo ciLanguage = new CultureInfo (language); RegionInfo riLocation = new RegionInfo (location); String strNewCulture = ciLanguage.TwoLetterISOLanguageName + "-" + riLocation.TwoLetterISORegionName; CultureAndRegionInfoBuilder cb = new CultureAndRegionInfoBuilder (strNewCulture, CultureAndRegionModifiers.None); cb.LoadDataFromRegionInfo (riLocation); cb.LoadDataFromCultureInfo (ciLanguage); // Fix stuff cb.IetfLanguageTag = strNewCulture; cb.Parent = parentCulture; // Need to fix the language names cb.ThreeLetterISOLanguageName = ciLanguage.ThreeLetterISOLanguageName; cb.TwoLetterISOLanguageName = ciLanguage.TwoLetterISOLanguageName; cb.ThreeLetterWindowsLanguageName = ciLanguage.ThreeLetterWindowsLanguageName; // Need the Region Name in the current Language cb.RegionNativeName = riLocation.NativeName; // Fix the culture name(s) string strEnglishLanguageName = ciLanguage.EnglishName.Substring (0, ciLanguage.EnglishName.IndexOf ("(", StringComparison.Ordinal) - 1); string strNativeLanguageName = ciLanguage.NativeName.Substring (0, ciLanguage.NativeName.IndexOf ("(", StringComparison.Ordinal) - 1); ; cb.CultureEnglishName = strEnglishLanguageName + " (" + cb.RegionEnglishName + ")"; cb.CultureNativeName = strNativeLanguageName + " (" + cb.RegionNativeName + ")"; // Currency Name cb.CurrencyNativeName = riLocation.CurrencyNativeName; // Numbers cb.NumberFormat.PositiveInfinitySymbol = ciLanguage.NumberFormat.PositiveInfinitySymbol; cb.NumberFormat.NegativeInfinitySymbol = ciLanguage.NumberFormat.NegativeInfinitySymbol; cb.NumberFormat.NaNSymbol = ciLanguage.NumberFormat.NaNSymbol; // Copy Calendar Strings DateTimeFormatInfo dtfi = cb.GregorianDateTimeFormat; DateTimeFormatInfo dtfiLanguage = ciLanguage.DateTimeFormat; dtfiLanguage.Calendar = new GregorianCalendar (); // Set the layoutId cb.KeyboardLayoutId = layoutId; // Copy calendar data from that gregorian localized calendar dtfi.AbbreviatedDayNames = dtfiLanguage.AbbreviatedDayNames; dtfi.AbbreviatedMonthGenitiveNames = dtfiLanguage.AbbreviatedMonthGenitiveNames; dtfi.AbbreviatedMonthNames = dtfiLanguage.AbbreviatedMonthNames; dtfi.DayNames = dtfiLanguage.DayNames; dtfi.MonthGenitiveNames = dtfiLanguage.MonthGenitiveNames; dtfi.MonthNames = dtfiLanguage.MonthNames; dtfi.ShortestDayNames = dtfiLanguage.ShortestDayNames; // Save it cb.Register (); } #endregion //******************************************************************** // // Internal fields // //******************************************************************* #region Internal fields internal static readonly char [] _achTrimChars = new char [] { ' ', '\t', '\n', '\r' }; // Size of a char (avoid to use the marshal class internal const int _sizeOfChar = 2; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.IO; using System.Globalization; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. namespace System.Speech.Internal { internal static class Helpers { //******************************************************************* // // Internal Methods // //******************************************************************* #region Internal Methods // Disable parameter validation check #pragma warning disable 56507 // Throws exception if the specified Rule does not have a valid Id. static internal void ThrowIfEmptyOrNull (string s, string paramName) { if (string.IsNullOrEmpty (s)) { if (s == null) { throw new ArgumentNullException (paramName); } else { throw new ArgumentException (SR.Get (SRID.StringCanNotBeEmpty, paramName), paramName); } } } #pragma warning restore 56507 // Throws exception if the specified Rule does not have a valid Id. static internal void ThrowIfNull (object value, string paramName) { if (value == null) { throw new ArgumentNullException (paramName); } } static internal bool CompareInvariantCulture (CultureInfo culture1, CultureInfo culture2) { // If perfect match easy if (culture1.Equals (culture2)) { return true; } // Compare the Neutral culture while (!culture1.IsNeutralCulture) { culture1 = culture1.Parent; } while (!culture2.IsNeutralCulture) { culture2 = culture2.Parent; } return culture1.Equals (culture2); } // Copy the input cfg to the output. // Streams point to the start of the data on entry and to the end on exit static internal void CopyStream (Stream inputStream, Stream outputStream, int bytesToCopy) { // Copy using an intermediate buffer of a reasonable size. int bufferSize = bytesToCopy > 4096 ? 4096 : bytesToCopy; byte [] buffer = new byte [bufferSize]; int bytesRead; while (bytesToCopy > 0) { bytesRead = inputStream.Read (buffer, 0, bufferSize); if (bytesRead <= 0) { throw new EndOfStreamException (SR.Get (SRID.StreamEndedUnexpectedly)); } outputStream.Write (buffer, 0, bytesRead); bytesToCopy -= bytesRead; } } // Copy the input cfg to the output. // inputStream points to the start of the data on entry and to the end on exit static internal byte [] ReadStreamToByteArray (Stream inputStream, int bytesToCopy) { byte [] outputArray = new byte [bytesToCopy]; BlockingRead (inputStream, outputArray, 0, bytesToCopy); return outputArray; } static internal void BlockingRead (Stream stream, byte [] buffer, int offset, int count) { // Stream is not like IStream - it will block until some data is available but not necessarily all of it. while (count > 0) { int read = stream.Read (buffer, offset, count); if (read <= 0) // End of stream { throw new EndOfStreamException (); } count -= read; offset += read; } } ////// Combine 2 cultures into in a composite one and assign a keyboardLayoutId /// Language /// ---- /// /// /// static internal void CombineCulture (string language, string location, CultureInfo parentCulture, int layoutId) { CultureInfo ciLanguage = new CultureInfo (language); RegionInfo riLocation = new RegionInfo (location); String strNewCulture = ciLanguage.TwoLetterISOLanguageName + "-" + riLocation.TwoLetterISORegionName; CultureAndRegionInfoBuilder cb = new CultureAndRegionInfoBuilder (strNewCulture, CultureAndRegionModifiers.None); cb.LoadDataFromRegionInfo (riLocation); cb.LoadDataFromCultureInfo (ciLanguage); // Fix stuff cb.IetfLanguageTag = strNewCulture; cb.Parent = parentCulture; // Need to fix the language names cb.ThreeLetterISOLanguageName = ciLanguage.ThreeLetterISOLanguageName; cb.TwoLetterISOLanguageName = ciLanguage.TwoLetterISOLanguageName; cb.ThreeLetterWindowsLanguageName = ciLanguage.ThreeLetterWindowsLanguageName; // Need the Region Name in the current Language cb.RegionNativeName = riLocation.NativeName; // Fix the culture name(s) string strEnglishLanguageName = ciLanguage.EnglishName.Substring (0, ciLanguage.EnglishName.IndexOf ("(", StringComparison.Ordinal) - 1); string strNativeLanguageName = ciLanguage.NativeName.Substring (0, ciLanguage.NativeName.IndexOf ("(", StringComparison.Ordinal) - 1); ; cb.CultureEnglishName = strEnglishLanguageName + " (" + cb.RegionEnglishName + ")"; cb.CultureNativeName = strNativeLanguageName + " (" + cb.RegionNativeName + ")"; // Currency Name cb.CurrencyNativeName = riLocation.CurrencyNativeName; // Numbers cb.NumberFormat.PositiveInfinitySymbol = ciLanguage.NumberFormat.PositiveInfinitySymbol; cb.NumberFormat.NegativeInfinitySymbol = ciLanguage.NumberFormat.NegativeInfinitySymbol; cb.NumberFormat.NaNSymbol = ciLanguage.NumberFormat.NaNSymbol; // Copy Calendar Strings DateTimeFormatInfo dtfi = cb.GregorianDateTimeFormat; DateTimeFormatInfo dtfiLanguage = ciLanguage.DateTimeFormat; dtfiLanguage.Calendar = new GregorianCalendar (); // Set the layoutId cb.KeyboardLayoutId = layoutId; // Copy calendar data from that gregorian localized calendar dtfi.AbbreviatedDayNames = dtfiLanguage.AbbreviatedDayNames; dtfi.AbbreviatedMonthGenitiveNames = dtfiLanguage.AbbreviatedMonthGenitiveNames; dtfi.AbbreviatedMonthNames = dtfiLanguage.AbbreviatedMonthNames; dtfi.DayNames = dtfiLanguage.DayNames; dtfi.MonthGenitiveNames = dtfiLanguage.MonthGenitiveNames; dtfi.MonthNames = dtfiLanguage.MonthNames; dtfi.ShortestDayNames = dtfiLanguage.ShortestDayNames; // Save it cb.Register (); } #endregion //******************************************************************** // // Internal fields // //******************************************************************* #region Internal fields internal static readonly char [] _achTrimChars = new char [] { ' ', '\t', '\n', '\r' }; // Size of a char (avoid to use the marshal class internal const int _sizeOfChar = 2; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
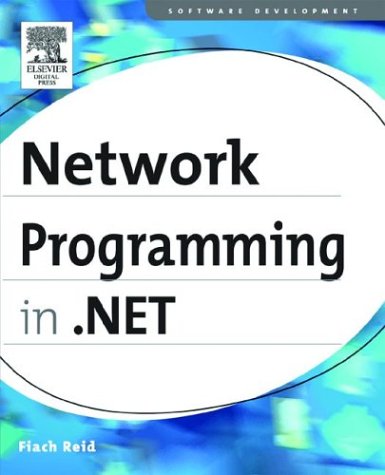
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextEditor.cs
- DecryptedHeader.cs
- MouseWheelEventArgs.cs
- XmlSerializerVersionAttribute.cs
- SelectingProviderEventArgs.cs
- BlobPersonalizationState.cs
- VariantWrapper.cs
- MonitorWrapper.cs
- XmlHierarchicalDataSourceView.cs
- CompoundFileIOPermission.cs
- ControlTemplate.cs
- DataRowCollection.cs
- ContentHostHelper.cs
- TableCellCollection.cs
- PassportPrincipal.cs
- RecommendedAsConfigurableAttribute.cs
- IERequestCache.cs
- DllNotFoundException.cs
- Accessible.cs
- WebPermission.cs
- RootProfilePropertySettingsCollection.cs
- listitem.cs
- XPathParser.cs
- VisualBrush.cs
- CompositionCommandSet.cs
- PropertyIdentifier.cs
- SqlNotificationRequest.cs
- TrustLevelCollection.cs
- _SafeNetHandles.cs
- ColorConvertedBitmap.cs
- ResponseBodyWriter.cs
- DrawingImage.cs
- DesignTimeTemplateParser.cs
- CopyNamespacesAction.cs
- PassportAuthenticationEventArgs.cs
- DataGridViewControlCollection.cs
- EventManager.cs
- DispatcherSynchronizationContext.cs
- NamespaceImport.cs
- TargetInvocationException.cs
- DataGridPageChangedEventArgs.cs
- SizeValueSerializer.cs
- ConnectionsZone.cs
- PreservationFileReader.cs
- ConfigErrorGlyph.cs
- VirtualizingPanel.cs
- InvokerUtil.cs
- MatrixTransform.cs
- Random.cs
- CLSCompliantAttribute.cs
- XmlDocumentSerializer.cs
- lengthconverter.cs
- ConnectionPool.cs
- RoleProviderPrincipal.cs
- SetterBase.cs
- WebConfigurationHostFileChange.cs
- SchemaTableColumn.cs
- EventProviderWriter.cs
- SelectionItemProviderWrapper.cs
- DataObjectPastingEventArgs.cs
- PhonemeEventArgs.cs
- SettingsBindableAttribute.cs
- Queue.cs
- DataGridViewSelectedCellCollection.cs
- FileEnumerator.cs
- WindowsSolidBrush.cs
- ReflectPropertyDescriptor.cs
- ServiceBuildProvider.cs
- ProvideValueServiceProvider.cs
- WS2007HttpBindingCollectionElement.cs
- MouseEvent.cs
- ForceCopyBuildProvider.cs
- ItemPager.cs
- CallContext.cs
- EdmSchemaAttribute.cs
- QueryResult.cs
- ReflectPropertyDescriptor.cs
- DockPatternIdentifiers.cs
- StreamHelper.cs
- WebServiceResponseDesigner.cs
- BindingElementCollection.cs
- BitmapPalettes.cs
- HtmlElement.cs
- PresentationSource.cs
- ListSortDescriptionCollection.cs
- GCHandleCookieTable.cs
- XmlDigitalSignatureProcessor.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- ColumnBinding.cs
- ConnectionStringsSection.cs
- DecimalSumAggregationOperator.cs
- ByteStreamGeometryContext.cs
- RSAPKCS1KeyExchangeFormatter.cs
- FixedHyperLink.cs
- RIPEMD160.cs
- SectionRecord.cs
- IntegerFacetDescriptionElement.cs
- LookupBindingPropertiesAttribute.cs
- DateTimeConverter.cs
- ConvertersCollection.cs