Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Serializers / XmlDocumentSerializer.cs / 1305376 / XmlDocumentSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for the Atom Service Document format. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Data.Services.Providers; using System.Diagnostics; using System.IO; using System.Text; using System.Xml; ///Provides support for serializing generic XML documents. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1001:TypesThatOwnDisposableFieldsShouldBeDisposable", Justification = "Pending review.")] [DebuggerDisplay("XmlDocumentSerializer={baseUri}")] internal abstract class XmlDocumentSerializer : IExceptionWriter { ///Base URI from which resources should be resolved. private readonly Uri baseUri; ///Data provider from which metadata should be gathered. private readonly DataServiceProviderWrapper provider; ///Writer to which output is sent. private readonly XmlWriter writer; ////// Initializes a new XmlDocumentSerializer, ready to write /// out an XML document /// /// Stream to which output should be sent. /// Base URI from which resources should be resolved. /// Data provider from which metadata should be gathered. /// Text encoding for the response. internal XmlDocumentSerializer( Stream output, Uri baseUri, DataServiceProviderWrapper provider, Encoding encoding) { Debug.Assert(output != null, "output != null"); Debug.Assert(provider != null, "provider != null"); Debug.Assert(baseUri != null, "baseUri != null"); Debug.Assert(encoding != null, "encoding != null"); Debug.Assert(baseUri.IsAbsoluteUri, "baseUri.IsAbsoluteUri(" + baseUri + ")"); Debug.Assert(baseUri.AbsoluteUri[baseUri.AbsoluteUri.Length - 1] == '/', "baseUri(" + baseUri.AbsoluteUri + ") ends with '/'"); this.writer = XmlUtil.CreateXmlWriterAndWriteProcessingInstruction(output, encoding); this.provider = provider; this.baseUri = baseUri; } ///Base URI from which resources should be resolved. protected Uri BaseUri { get { return this.baseUri; } } ///Data provider from which metadata should be gathered. protected DataServiceProviderWrapper Provider { get { return this.provider; } } ///Writer to which output is sent. protected XmlWriter Writer { get { return this.writer; } } ///Serializes exception information. /// Description of exception to serialize. public void WriteException(HandleExceptionArgs args) { ErrorHandler.SerializeXmlError(args, this.writer); } ///Writes the document for this request.. /// Data service instance. internal abstract void WriteRequest(IDataService service); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a serializer for the Atom Service Document format. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Serializers { using System; using System.Data.Services.Providers; using System.Diagnostics; using System.IO; using System.Text; using System.Xml; ///Provides support for serializing generic XML documents. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1001:TypesThatOwnDisposableFieldsShouldBeDisposable", Justification = "Pending review.")] [DebuggerDisplay("XmlDocumentSerializer={baseUri}")] internal abstract class XmlDocumentSerializer : IExceptionWriter { ///Base URI from which resources should be resolved. private readonly Uri baseUri; ///Data provider from which metadata should be gathered. private readonly DataServiceProviderWrapper provider; ///Writer to which output is sent. private readonly XmlWriter writer; ////// Initializes a new XmlDocumentSerializer, ready to write /// out an XML document /// /// Stream to which output should be sent. /// Base URI from which resources should be resolved. /// Data provider from which metadata should be gathered. /// Text encoding for the response. internal XmlDocumentSerializer( Stream output, Uri baseUri, DataServiceProviderWrapper provider, Encoding encoding) { Debug.Assert(output != null, "output != null"); Debug.Assert(provider != null, "provider != null"); Debug.Assert(baseUri != null, "baseUri != null"); Debug.Assert(encoding != null, "encoding != null"); Debug.Assert(baseUri.IsAbsoluteUri, "baseUri.IsAbsoluteUri(" + baseUri + ")"); Debug.Assert(baseUri.AbsoluteUri[baseUri.AbsoluteUri.Length - 1] == '/', "baseUri(" + baseUri.AbsoluteUri + ") ends with '/'"); this.writer = XmlUtil.CreateXmlWriterAndWriteProcessingInstruction(output, encoding); this.provider = provider; this.baseUri = baseUri; } ///Base URI from which resources should be resolved. protected Uri BaseUri { get { return this.baseUri; } } ///Data provider from which metadata should be gathered. protected DataServiceProviderWrapper Provider { get { return this.provider; } } ///Writer to which output is sent. protected XmlWriter Writer { get { return this.writer; } } ///Serializes exception information. /// Description of exception to serialize. public void WriteException(HandleExceptionArgs args) { ErrorHandler.SerializeXmlError(args, this.writer); } ///Writes the document for this request.. /// Data service instance. internal abstract void WriteRequest(IDataService service); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
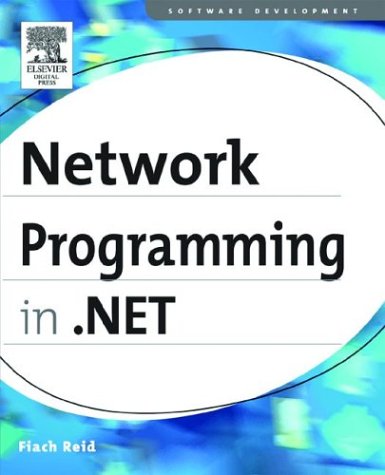
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SharedPersonalizationStateInfo.cs
- WorkflowDataContext.cs
- TypeSystem.cs
- WebBrowserContainer.cs
- ScriptingWebServicesSectionGroup.cs
- UnsafeNativeMethodsCLR.cs
- EventToken.cs
- DiffuseMaterial.cs
- CodeParameterDeclarationExpressionCollection.cs
- StringBuilder.cs
- EmbeddedMailObjectsCollection.cs
- BaseCodeDomTreeGenerator.cs
- EnumMember.cs
- DynamicVirtualDiscoSearcher.cs
- CatalogZone.cs
- PerspectiveCamera.cs
- SimpleRecyclingCache.cs
- TypeUtil.cs
- CfgSemanticTag.cs
- FontWeightConverter.cs
- WindowsGraphics2.cs
- KeyValueConfigurationCollection.cs
- WebBodyFormatMessageProperty.cs
- Stack.cs
- SelectingProviderEventArgs.cs
- SoapFault.cs
- XhtmlBasicLiteralTextAdapter.cs
- DetailsView.cs
- PathFigureCollectionValueSerializer.cs
- RelationshipDetailsRow.cs
- XmlSchemaRedefine.cs
- Label.cs
- ListItemViewControl.cs
- DataBinder.cs
- _ServiceNameStore.cs
- BinaryObjectInfo.cs
- UpdateTranslator.cs
- MsmqMessage.cs
- SystemInformation.cs
- RoutedEventArgs.cs
- FontUnit.cs
- ProfileBuildProvider.cs
- RouteValueExpressionBuilder.cs
- SystemTcpStatistics.cs
- IndicCharClassifier.cs
- XmlSchemaComplexContentRestriction.cs
- SharedPerformanceCounter.cs
- Rect.cs
- mactripleDES.cs
- WebReference.cs
- Help.cs
- DataSvcMapFile.cs
- AbandonedMutexException.cs
- PixelFormats.cs
- ExpressionStringBuilder.cs
- XmlReflectionMember.cs
- TrackingQuery.cs
- DBSqlParserColumnCollection.cs
- QuadTree.cs
- RuleSettings.cs
- DispatchChannelSink.cs
- ConnectionModeReader.cs
- GetRecipientListRequest.cs
- TrackBarRenderer.cs
- PropertyKey.cs
- CodePageUtils.cs
- StreamWriter.cs
- DataSetMappper.cs
- CodeCommentStatement.cs
- FixedSOMPageConstructor.cs
- Faults.cs
- ChildrenQuery.cs
- ColumnCollection.cs
- OracleRowUpdatingEventArgs.cs
- XmlILAnnotation.cs
- CriticalFinalizerObject.cs
- CmsInterop.cs
- DesigntimeLicenseContext.cs
- MenuItemBindingCollection.cs
- CustomLineCap.cs
- StorageMappingFragment.cs
- ObjectSecurity.cs
- CqlLexerHelpers.cs
- EmptyQuery.cs
- DesignerActionItemCollection.cs
- WebProxyScriptElement.cs
- TreeView.cs
- AmbiguousMatchException.cs
- XmlQualifiedName.cs
- OdbcEnvironmentHandle.cs
- ExpandSegmentCollection.cs
- GridViewHeaderRowPresenter.cs
- XmlSchemaComplexContent.cs
- RouteValueExpressionBuilder.cs
- RuntimeConfigLKG.cs
- LinqDataSourceInsertEventArgs.cs
- Image.cs
- DataViewManagerListItemTypeDescriptor.cs
- AesManaged.cs
- LogicalExpr.cs