Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / GetRecipientListRequest.cs / 1 / GetRecipientListRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Collections; using System.Collections.Generic; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // This class handles a UI request for recipient information // class GetRecipientListRequest : UIAgentRequest { IListm_recipientList; // // Summary // Create a new request to return the list of recipients // public GetRecipientListRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } protected override void OnMarshalInArgs() { } // // Summary // Retrive the recipient list // protected override void OnProcess() { StoreConnection connection = StoreConnection.GetConnection(); try { // // Retrieve the recipient objects from the store // IList rows = ( IList )connection.Query( QueryDetails.FullRow, new QueryParameter( SecondaryIndexDefinition.ObjectTypeIndex, ( Int32 )StorableObjectType.Recipient ) ); if( null != rows ) { IList recipientList = new List ( rows.Count ); foreach( DataRow row in rows ) { recipientList.Add( new Recipient( new MemoryStream( row.GetDataField() ) ) ); } m_recipientList = recipientList; } } finally { connection.Close(); } } // // Summary // Serialize the output in the following order // Count of objects // Recipient objects // // protected override void OnMarshalOutArgs() { BinaryWriter writer = new BinaryWriter( OutArgs, System.Text.Encoding.Unicode ); UInt32 count = 0; IDT.TraceDebug( "Serialize the recipient List" ); if( null != m_recipientList ) { count = ( UInt32 )m_recipientList.Count; writer.Write( count ); foreach( Recipient rec in m_recipientList ) { rec.Serialize( writer ); } } else { writer.Write( count ); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
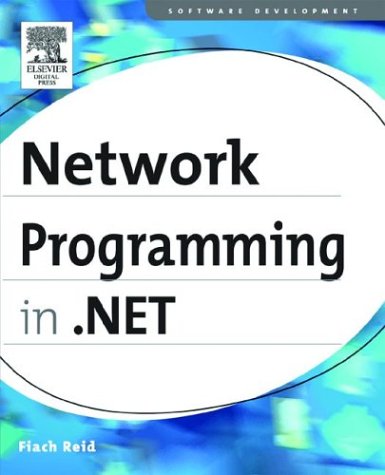
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExceptionTranslationTable.cs
- FontConverter.cs
- EntityDataSourceWizardForm.cs
- SoapAttributeAttribute.cs
- MsmqIntegrationValidationBehavior.cs
- Int64AnimationUsingKeyFrames.cs
- X509RecipientCertificateServiceElement.cs
- HtmlInputCheckBox.cs
- EntityTransaction.cs
- PackageRelationshipCollection.cs
- LingerOption.cs
- SafeThemeHandle.cs
- DataServiceResponse.cs
- ComponentDispatcher.cs
- DbFunctionCommandTree.cs
- GraphicsContext.cs
- GlyphsSerializer.cs
- SEHException.cs
- ManagedIStream.cs
- _DigestClient.cs
- ScalarOps.cs
- XmlAnyAttributeAttribute.cs
- cookiecollection.cs
- BevelBitmapEffect.cs
- RemotingServices.cs
- SHA512Managed.cs
- ValidationPropertyAttribute.cs
- SpnEndpointIdentityExtension.cs
- ServiceRoute.cs
- PenThread.cs
- ServicePointManagerElement.cs
- StackSpiller.Generated.cs
- UrlMappingsSection.cs
- DataTableReader.cs
- URLIdentityPermission.cs
- CheckBox.cs
- CodeAttributeArgument.cs
- TextBreakpoint.cs
- ArgumentsParser.cs
- GridViewColumnCollectionChangedEventArgs.cs
- QilChoice.cs
- Cursors.cs
- ToolStripSplitButton.cs
- EllipseGeometry.cs
- FontCollection.cs
- ClientType.cs
- FilteredDataSetHelper.cs
- DocumentViewerConstants.cs
- ProfileService.cs
- SQLDecimal.cs
- AlphabetConverter.cs
- ConfigXmlAttribute.cs
- WsatServiceCertificate.cs
- EventLogPermission.cs
- updateconfighost.cs
- HttpDictionary.cs
- PolyBezierSegment.cs
- MetaType.cs
- EdmItemError.cs
- NullableDecimalSumAggregationOperator.cs
- ObjectDataSourceFilteringEventArgs.cs
- XmlAnyAttributeAttribute.cs
- ServiceOperation.cs
- RsaKeyIdentifierClause.cs
- ClockController.cs
- ThreadAbortException.cs
- BlurBitmapEffect.cs
- UnsafeMethods.cs
- BindableTemplateBuilder.cs
- DirectoryInfo.cs
- WebPartVerb.cs
- ImpersonateTokenRef.cs
- FamilyMap.cs
- WeakReference.cs
- InternalSafeNativeMethods.cs
- RegistrySecurity.cs
- ReflectTypeDescriptionProvider.cs
- errorpatternmatcher.cs
- XmlUtf8RawTextWriter.cs
- AddInStore.cs
- EventLog.cs
- FormViewPageEventArgs.cs
- DecoderReplacementFallback.cs
- PassportAuthenticationModule.cs
- ImageField.cs
- MenuTracker.cs
- ClientFactory.cs
- FindCriteriaElement.cs
- CanonicalFormWriter.cs
- DataBindEngine.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- LowerCaseStringConverter.cs
- SafeCryptoHandles.cs
- CodeTypeParameterCollection.cs
- SiteOfOriginPart.cs
- StoreItemCollection.cs
- RequestCacheValidator.cs
- DecoderFallbackWithFailureFlag.cs
- ItemCheckedEvent.cs
- MonitoringDescriptionAttribute.cs