Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / GridViewColumnCollectionChangedEventArgs.cs / 1 / GridViewColumnCollectionChangedEventArgs.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Controls; using System.Collections.Generic; // IListusing System.Collections.Specialized; // NotifyCollectionChangedEventArgs using System.Collections.ObjectModel; // Collection, ReadOnlyCollection using System.Diagnostics; // Assert namespace System.Windows.Controls { /// /// Argument for GridViewColumnCollectionChanged event /// internal class GridViewColumnCollectionChangedEventArgs : NotifyCollectionChangedEventArgs { ////// constructor (for a property of one column changed) /// /// column whose property changed /// Name of the changed property internal GridViewColumnCollectionChangedEventArgs(GridViewColumn column, string propertyName) : base(NotifyCollectionChangedAction.Reset) // NotifyCollectionChangedEventArgs doesn't have 0 parameter constructor, so pass in an arbitrary parameter. { _column = column; _propertyName = propertyName; } ////// constructor (for clear) /// /// must be NotifyCollectionChangedAction.Reset /// Columns removed in reset action internal GridViewColumnCollectionChangedEventArgs(NotifyCollectionChangedAction action, GridViewColumn[] clearedColumns) : base(action) { _clearedColumns = System.Array.AsReadOnly(clearedColumns); } /// /// Construct for one-column Add/Remove event. /// internal GridViewColumnCollectionChangedEventArgs(NotifyCollectionChangedAction action, GridViewColumn changedItem, int index, int actualIndex) : base (action, changedItem, index) { Debug.Assert(action == NotifyCollectionChangedAction.Add || action == NotifyCollectionChangedAction.Remove, "This constructor only supports Add/Remove action."); Debug.Assert(changedItem != null, "changedItem can't be null"); Debug.Assert(index >= 0, "index must >= 0"); Debug.Assert(actualIndex >= 0, "actualIndex must >= 0"); _actualIndex = actualIndex; } ////// Construct for a one-column Replace event. /// internal GridViewColumnCollectionChangedEventArgs(NotifyCollectionChangedAction action, GridViewColumn newItem, GridViewColumn oldItem, int index, int actualIndex) : base(action, newItem, oldItem, index) { Debug.Assert(newItem != null, "newItem can't be null"); Debug.Assert(oldItem != null, "oldItem can't be null"); Debug.Assert(index >= 0, "index must >= 0"); Debug.Assert(actualIndex >= 0, "actualIndex must >= 0"); _actualIndex = actualIndex; } ////// Construct for a one-column Move event. /// internal GridViewColumnCollectionChangedEventArgs(NotifyCollectionChangedAction action, GridViewColumn changedItem, int index, int oldIndex, int actualIndex) : base(action, changedItem, index, oldIndex) { Debug.Assert(changedItem != null, "changedItem can't be null"); Debug.Assert(index >= 0, "index must >= 0"); Debug.Assert(oldIndex >= 0, "oldIndex must >= 0"); Debug.Assert(actualIndex >= 0, "actualIndex must >= 0"); _actualIndex = actualIndex; } ////// index of the changed column in the internal column list. /// internal int ActualIndex { get { return _actualIndex; } } private int _actualIndex = -1; ////// Columns removed in reset action. /// internal ReadOnlyCollectionClearedColumns { get { return _clearedColumns; } } private ReadOnlyCollection _clearedColumns; // The following two properties are used to store information of GridViewColumns.PropertyChanged event. // // GridViewColumnCollection hookup GridViewColumns.PropertyChanged event. When GridViewColumns.PropertyChanged // event is raised, GridViewColumnCollection will raised CollectionChanged event with GridViewColumnCollectionChangedEventArgs. // In the event arg the following two properties will be set, so GridViewRowPresenter will be informed. // // GridViewRowPresenter needn't hookup PropertyChanged event of each column, which cost a lot of time in scroll operation. /// /// Column whose property changed /// internal GridViewColumn Column { get { return _column; } } private GridViewColumn _column; ////// Name of the changed property /// internal string PropertyName { get { return _propertyName; } } private string _propertyName; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
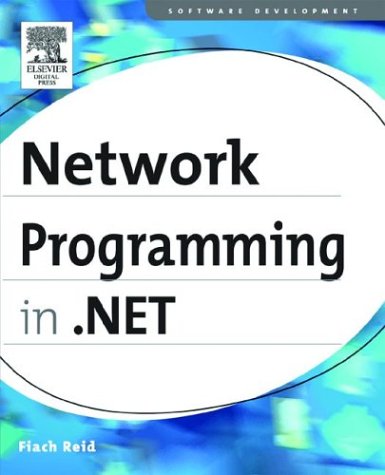
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StyleXamlTreeBuilder.cs
- InternalPermissions.cs
- DataGridItemEventArgs.cs
- SplitterPanelDesigner.cs
- ApplicationId.cs
- WriterOutput.cs
- ListenDesigner.cs
- AlgoModule.cs
- TemplateNodeContextMenu.cs
- Panel.cs
- X509Certificate.cs
- AdPostCacheSubstitution.cs
- ResourceContainer.cs
- SqlClientMetaDataCollectionNames.cs
- DataGridViewRowsRemovedEventArgs.cs
- Cursors.cs
- DataGridViewAccessibleObject.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- XmlnsDefinitionAttribute.cs
- ProgressBar.cs
- Color.cs
- ObjectView.cs
- FrameworkContextData.cs
- AssociationSet.cs
- DrawListViewSubItemEventArgs.cs
- ElementNotEnabledException.cs
- ConfigurationManagerHelper.cs
- Brush.cs
- ipaddressinformationcollection.cs
- Model3DGroup.cs
- Bidi.cs
- WebPartUtil.cs
- UnsafeNativeMethodsMilCoreApi.cs
- contentDescriptor.cs
- SurrogateDataContract.cs
- SqlProfileProvider.cs
- TriggerActionCollection.cs
- oledbconnectionstring.cs
- CompilerErrorCollection.cs
- Attachment.cs
- ActivitySurrogateSelector.cs
- SizeAnimation.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- DataListItemCollection.cs
- CollectionType.cs
- DefaultPropertyAttribute.cs
- ReflectEventDescriptor.cs
- ParameterBuilder.cs
- AnimationException.cs
- DataContractJsonSerializer.cs
- GeometryDrawing.cs
- XmlSchemaSubstitutionGroup.cs
- DrawListViewSubItemEventArgs.cs
- DashStyles.cs
- UnmanagedMemoryStream.cs
- CodeDefaultValueExpression.cs
- InfoCard.cs
- TextServicesManager.cs
- PerspectiveCamera.cs
- ControlEvent.cs
- ToolStripDropDown.cs
- EntityDescriptor.cs
- SizeIndependentAnimationStorage.cs
- SystemInformation.cs
- QueryCacheManager.cs
- XmlDataContract.cs
- GuidelineSet.cs
- WindowsPrincipal.cs
- QilIterator.cs
- ObfuscationAttribute.cs
- InfoCardRSACryptoProvider.cs
- RoutingConfiguration.cs
- DataGridViewColumnConverter.cs
- HtmlShimManager.cs
- FixedSOMContainer.cs
- ColorEditor.cs
- translator.cs
- DataControlPagerLinkButton.cs
- IdentityReference.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- TextStore.cs
- base64Transforms.cs
- AttachedAnnotationChangedEventArgs.cs
- GraphicsContext.cs
- ControlEvent.cs
- documentsequencetextcontainer.cs
- SqlNotificationRequest.cs
- DataGridItemAutomationPeer.cs
- relpropertyhelper.cs
- ScriptingSectionGroup.cs
- Event.cs
- Debug.cs
- MediaScriptCommandRoutedEventArgs.cs
- MethodSet.cs
- TypeInfo.cs
- GeneralTransform3D.cs
- PtsHelper.cs
- GPRECTF.cs
- XmlFormatExtensionPointAttribute.cs
- PanelStyle.cs