Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Shared / MS / Internal / Permissions / InternalPermissions.cs / 1 / InternalPermissions.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Internal Permissions. // These are classes for permissions that will be asserted/demanded internally. // But will be granted in full-trust. // Only internal avalon code will assert these permissions. // // Using them allows the following: // We can have very specific targeted asserts. So for example instead of // a blanket assert for Unmanaged code instead we can have very granular permissiosn. // // They are still available by default in full-trust. // // Currently the only way to detect User-Initiated actions is for commands. // So by associating a custom permisison with a command we can very tightly scope // the set of operations allowed. // // History: // 02/28/05 : [....] - Created //--------------------------------------------------------------------------- using System; using System.Text; using System.Security; using System.Security.Permissions; using System.Windows; #if WINDOWS_BASE using MS.Internal.WindowsBase; #endif namespace MS.Internal.Permissions { // // derive all InternalPermissions from this. // Provides default implementations of several abstract methods on CodeAccessPermission // [FriendAccessAllowed] internal abstract class InternalPermissionBase : CodeAccessPermission, IUnrestrictedPermission { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructor public InternalPermissionBase( ) { } #endregion Constructor //------------------------------------------------------ // // Interface Methods // //----------------------------------------------------- #region Interface Methods public bool IsUnrestricted() { return true; } #endregion Interface Methods //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods public override SecurityElement ToXml() { SecurityElement element = new SecurityElement("IPermission"); Type type = this.GetType(); StringBuilder AssemblyName = new StringBuilder(type.Assembly.ToString()); AssemblyName.Replace('\"', '\''); element.AddAttribute("class", type.FullName + ", " + AssemblyName); element.AddAttribute("version", "1"); return element; } public override void FromXml( SecurityElement elem) { // from XML is easy - there is no state. } public override IPermission Intersect(IPermission target) { if(null == target) { return null; } if ( target.GetType() != this.GetType() ) { throw new ArgumentException( SR.Get(SRID.InvalidPermissionType), this.GetType().FullName); } // there is no state. The intersection of 2 permissions of the same type is the same permission. return this.Copy(); } public override bool IsSubsetOf(IPermission target) { if(null == target) { return false; } if ( target.GetType() != this.GetType() ) { throw new ArgumentException( SR.Get(SRID.InvalidPermissionType), this.GetType().FullName); } // there is no state. If you are the same type as me - you are a subset of me. return true; } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
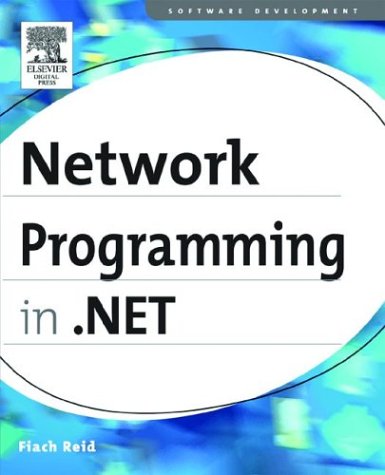
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventHandlerList.cs
- PictureBox.cs
- TextRangeProviderWrapper.cs
- SQLInt64Storage.cs
- NavigationPropertyEmitter.cs
- SqlCharStream.cs
- TextViewSelectionProcessor.cs
- AutomationEvent.cs
- ManipulationLogic.cs
- login.cs
- Container.cs
- WindowProviderWrapper.cs
- VolatileEnlistmentMultiplexing.cs
- CodeParameterDeclarationExpression.cs
- WriteFileContext.cs
- RegionIterator.cs
- MethodCallExpression.cs
- ExtendedPropertyCollection.cs
- MILUtilities.cs
- PageVisual.cs
- FlowDocumentPaginator.cs
- ClipboardData.cs
- RSAPKCS1SignatureDeformatter.cs
- Vector3DValueSerializer.cs
- ApplyHostConfigurationBehavior.cs
- WebFormDesignerActionService.cs
- PersonalizableTypeEntry.cs
- EastAsianLunisolarCalendar.cs
- XmlDataProvider.cs
- TrustLevelCollection.cs
- OracleTimeSpan.cs
- QueueProcessor.cs
- NavigationHelper.cs
- Trace.cs
- OleDbDataReader.cs
- linebase.cs
- SqlNodeAnnotation.cs
- Operator.cs
- CodeDesigner.cs
- Trigger.cs
- ButtonChrome.cs
- CodeDefaultValueExpression.cs
- XmlQueryCardinality.cs
- BrowserDefinition.cs
- XmlSchemaCollection.cs
- SettingsAttributes.cs
- DataColumnCollection.cs
- Maps.cs
- UriExt.cs
- ServiceDescriptionSerializer.cs
- OdbcConnectionStringbuilder.cs
- EventMemberCodeDomSerializer.cs
- TreeViewImageGenerator.cs
- WindowsServiceCredential.cs
- BaseTemplateCodeDomTreeGenerator.cs
- EditorReuseAttribute.cs
- httpapplicationstate.cs
- XhtmlConformanceSection.cs
- SchemaElementDecl.cs
- RetrieveVirtualItemEventArgs.cs
- ContainerUtilities.cs
- ThreadStaticAttribute.cs
- SeverityFilter.cs
- Int32CollectionValueSerializer.cs
- HtmlLink.cs
- Process.cs
- IntPtr.cs
- SqlDataSourceTableQuery.cs
- ExpressionPrefixAttribute.cs
- sqlcontext.cs
- FastEncoderWindow.cs
- AbandonedMutexException.cs
- AxisAngleRotation3D.cs
- JoinGraph.cs
- ISAPIApplicationHost.cs
- XmlDocumentSerializer.cs
- Int64Animation.cs
- SizeFConverter.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- Reference.cs
- ApplicationManager.cs
- QualificationDataItem.cs
- StringValueSerializer.cs
- CacheRequest.cs
- VisualProxy.cs
- RectangleGeometry.cs
- XD.cs
- TimeEnumHelper.cs
- Byte.cs
- BasePropertyDescriptor.cs
- loginstatus.cs
- TreeViewItemAutomationPeer.cs
- xmlsaver.cs
- DynamicDataRoute.cs
- XPathDescendantIterator.cs
- NameValueConfigurationElement.cs
- ClientBuildManagerTypeDescriptionProviderBridge.cs
- CodeTypeReferenceSerializer.cs
- RecommendedAsConfigurableAttribute.cs
- BindingElementCollection.cs