Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / DynamicDataRoute.cs / 1305376 / DynamicDataRoute.cs
using System.Web.Routing; using System.Diagnostics; namespace System.Web.DynamicData { ////// Route used by Dynamic Data /// public class DynamicDataRoute : Route { internal const string ActionToken = "Action"; internal const string TableToken = "Table"; internal const string ModelToken = "__Model"; private MetaModel _model; private bool _initialized; private object _initializationLock = new object(); ////// Construct a DynamicDataRoute /// /// url passed to the base ctor [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1054:UriParametersShouldNotBeStrings", Justification = "This is a URL template with special characters, not just a regular valid URL.")] public DynamicDataRoute(string url) : base(url, new DynamicDataRouteHandler()) { } ////// Name of the table that this route applies to. Can be omitted. /// public string Table { get; set; } ////// Action that this route applies to. Can be omitted. /// public string Action { get; set; } ////// The ViewName is the name of the page used to handle the request. If omitted, it defaults to the Action name. /// public string ViewName { get; set; } ////// The MetaModel that this route applies to /// public MetaModel Model { get { return _model ?? MetaModel.Default; } set { _model = value; } } // Make sure that if the Table or Action properties were used, they get added to // the Defaults dictionary private void EnsureRouteInitialize() { if (!_initialized) { lock (_initializationLock) { if (!_initialized) { _initialized = true; // Give the model to the handler Debug.Assert(Model != null); RouteHandler.Model = Model; // If neither was specified, we don't need to do anything if (Table == null && Action == null) return; // If we don't already have a Defaults dictionary, create one if (Defaults == null) Defaults = new RouteValueDictionary(); if (Table != null) { // Try to get the table just to cause a failure if it doesn't exist var metaTable = Model.GetTable(Table); Defaults[TableToken] = Table; } if (Action != null) Defaults[ActionToken] = Action; } } } } ////// See base class documentation /// public override RouteData GetRouteData(HttpContextBase httpContext) { EnsureRouteInitialize(); // Try to get the route data for this route RouteData routeData = base.GetRouteData(httpContext); // If not, we're done if (routeData == null) { return null; } // Add all the query string values to the RouteData // AddQueryStringParamsToRouteData(httpContext, routeData); // Check if the route values match an existing table and if they can be served by a scaffolded or custom page if (!VerifyRouteValues(routeData.Values)) return null; return routeData; } internal static void AddQueryStringParamsToRouteData(HttpContextBase httpContext, RouteData routeData) { foreach (string key in httpContext.Request.QueryString) { // Don't overwrite existing items if (!routeData.Values.ContainsKey(key)) { routeData.Values[key] = httpContext.Request.QueryString[key]; } } } ////// See base class documentation /// public override VirtualPathData GetVirtualPath(RequestContext requestContext, RouteValueDictionary values) { EnsureRouteInitialize(); // Check if the route values include a MetaModel object modelObject; if (values.TryGetValue(ModelToken, out modelObject)) { var model = modelObject as MetaModel; if (model != null) { // If it's different from the one for this route, fail the route matching if (modelObject != Model) return null; // It has the right model, so we want to continue. But we first need to // remove this token so it doesn't affect the path values.Remove(ModelToken); } } // Call the base to try to generate a path from this route VirtualPathData virtualPathData = base.GetVirtualPath(requestContext, values); // If not, we're done if (virtualPathData == null) return null; // Check if the route values match an existing table and if they can be served by a scaffolded or custom page if (VerifyRouteValues(values)) { return virtualPathData; } else { return null; } } private bool VerifyRouteValues(RouteValueDictionary values) { // Get the MetaTable and action. If either is missing, return false to skip this route object tableNameObject, actionObject; if (!values.TryGetValue(TableToken, out tableNameObject) || !values.TryGetValue(ActionToken, out actionObject)) { return false; } MetaTable table; // If no table by such name is available, return false to move on to next route. if (!Model.TryGetTable((string)tableNameObject, out table)) { return false; } // Check if n Page can be accessed for the table/action (either scaffold or custom). // If not, return false so that this route is not used and the search goes on. return RouteHandler.CreateHandler(this, table, (string)actionObject) != null; } ////// Extract the MetaTable from the RouteData. Fails if it can't find it /// /// The route data ///The found MetaTable public MetaTable GetTableFromRouteData(RouteData routeData) { string tableName = routeData.GetRequiredString(TableToken); return Model.GetTable(tableName); } ////// Extract the Action from the RouteData. Fails if it can't find it /// /// The route data ///The found Action public string GetActionFromRouteData(RouteData routeData) { return routeData.GetRequiredString(ActionToken); } ////// Strongly typed version of Route.RouteHandler for convenience /// public new DynamicDataRouteHandler RouteHandler { get { return (DynamicDataRouteHandler)base.RouteHandler; } set { base.RouteHandler = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
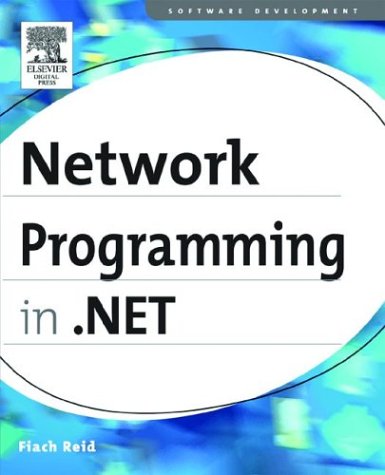
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContentPropertyAttribute.cs
- LightweightCodeGenerator.cs
- BamlStream.cs
- IsolatedStorageFileStream.cs
- IsolatedStorageFile.cs
- ADConnectionHelper.cs
- SourceExpressionException.cs
- Vector3DValueSerializer.cs
- InheritanceRules.cs
- ResXBuildProvider.cs
- XmlSchemaImport.cs
- XmlTextWriter.cs
- PeerDuplexChannelListener.cs
- FixedSOMImage.cs
- XmlIlGenerator.cs
- TabControl.cs
- HttpDictionary.cs
- ScriptingScriptResourceHandlerSection.cs
- EmptyImpersonationContext.cs
- isolationinterop.cs
- GeometryCombineModeValidation.cs
- HtmlTextArea.cs
- InstanceData.cs
- InArgumentConverter.cs
- WorkflowOperationInvoker.cs
- XmlComment.cs
- FactoryRecord.cs
- HiddenField.cs
- InfoCardBaseException.cs
- DrawingState.cs
- ObjectViewQueryResultData.cs
- SecurityRuntime.cs
- DeferredReference.cs
- UriParserTemplates.cs
- DbParameterHelper.cs
- BaseTemplateBuildProvider.cs
- WebScriptMetadataMessageEncodingBindingElement.cs
- PerfCounters.cs
- counter.cs
- CodeIdentifiers.cs
- UriScheme.cs
- TraceEventCache.cs
- GridViewAutomationPeer.cs
- ProfileEventArgs.cs
- NamedPipeProcessProtocolHandler.cs
- SslStreamSecurityBindingElement.cs
- Int32Converter.cs
- Int16AnimationBase.cs
- MenuCommand.cs
- CodeLinePragma.cs
- CodeDomConfigurationHandler.cs
- WebPartTransformer.cs
- PackageRelationshipCollection.cs
- WindowsGraphicsWrapper.cs
- JsonWriter.cs
- ParameterBuilder.cs
- StylusCaptureWithinProperty.cs
- WebPartConnectionCollection.cs
- FloaterBaseParagraph.cs
- OdbcFactory.cs
- DbConnectionFactory.cs
- DataGridColumnHeaderCollection.cs
- OdbcHandle.cs
- HttpApplicationFactory.cs
- SqlLiftWhereClauses.cs
- CssClassPropertyAttribute.cs
- InternalRelationshipCollection.cs
- ValidationErrorCollection.cs
- _NTAuthentication.cs
- PolicyManager.cs
- PropertyValueChangedEvent.cs
- DoubleLinkListEnumerator.cs
- AutomationPattern.cs
- ExpressionVisitorHelpers.cs
- TraceData.cs
- XmlEntity.cs
- CodeCatchClause.cs
- CodeBlockBuilder.cs
- DispatcherObject.cs
- GridViewColumnHeader.cs
- EntityDataSourceColumn.cs
- ExceptionHandlers.cs
- PropertyManager.cs
- EffectiveValueEntry.cs
- Win32Exception.cs
- CustomCredentialPolicy.cs
- Quaternion.cs
- TextPointer.cs
- TrustSection.cs
- SmtpAuthenticationManager.cs
- WebRequestModulesSection.cs
- DesignerProperties.cs
- ReflectionTypeLoadException.cs
- DateTimeUtil.cs
- ADMembershipProvider.cs
- TextMarkerSource.cs
- ImageButton.cs
- unsafenativemethodsother.cs
- FileClassifier.cs
- Transform3D.cs