Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / NewArray.cs / 1305376 / NewArray.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Expressions { using System.Activities; using System.Collections.ObjectModel; using System.Reflection; using System.Runtime.Collections; using System.Windows.Markup; [ContentProperty("Bounds")] public sealed class NewArray: CodeActivity { Collection bounds; ConstructorInfo constructorInfo; public Collection Bounds { get { if (this.bounds == null) { this.bounds = new ValidatingCollection { // disallow null values OnAddValidationCallback = item => { if (item == null) { throw FxTrace.Exception.ArgumentNull("item"); } } }; } return this.bounds; } } protected override void CacheMetadata(CodeActivityMetadata metadata) { if (!typeof(TResult).IsArray) { metadata.AddValidationError(SR.NewArrayRequiresArrayTypeAsResultType); // We shortcut any further processing in this case. return; } bool foundError = false; // Loop through each argument, validate it, and if validation // passed expose it to the metadata Type[] types = new Type[this.Bounds.Count]; for (int i = 0; i < this.Bounds.Count; i++) { Argument argument = this.Bounds[i]; if (argument == null || argument.IsEmpty) { metadata.AddValidationError(SR.ArgumentRequired("Bounds", typeof(NewArray ))); foundError = true; } else { if (!isIntegralType(argument.ArgumentType)) { metadata.AddValidationError(SR.NewArrayBoundsRequiresIntegralArguments); foundError = true; } else { RuntimeArgument runtimeArgument = new RuntimeArgument("Argument" + i, this.Bounds[i].ArgumentType, this.bounds[i].Direction, true); metadata.Bind(this.Bounds[i], runtimeArgument); metadata.AddArgument(runtimeArgument); types[i] = argument.ArgumentType; } } } // If we didn't find any errors in the arguments then // we can look for an appropriate constructor. if (!foundError) { this.constructorInfo = typeof(TResult).GetConstructor(types); if (this.constructorInfo == null) { metadata.AddValidationError(SR.ConstructorInfoNotFound(typeof(TResult).Name)); } } } protected override TResult Execute(CodeActivityContext context) { object[] objects = new object[this.Bounds.Count]; int i = 0; foreach (Argument argument in this.Bounds) { objects[i] = argument.Get(context); i++; } TResult result = (TResult)this.constructorInfo.Invoke(objects); return result; } bool isIntegralType(Type type) { if (type == typeof(sbyte) || type == typeof(byte) || type == typeof(char) || type == typeof(short) || type == typeof(ushort) || type == typeof(int) || type == typeof(uint) || type == typeof(long) || type == typeof(ulong)) { return true; } else { return false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
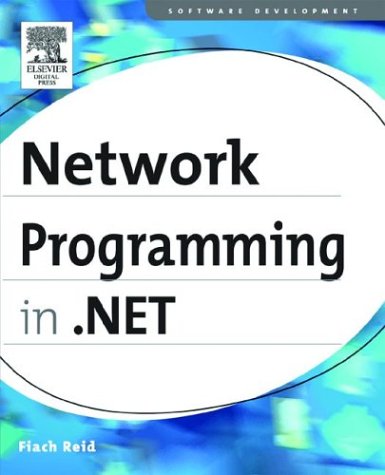
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MethodAccessException.cs
- SqlDeflator.cs
- ProfileSettingsCollection.cs
- typedescriptorpermissionattribute.cs
- MarkedHighlightComponent.cs
- SchemaImporterExtensionElement.cs
- MemberAssignmentAnalysis.cs
- Type.cs
- DesignerActionPropertyItem.cs
- PolyBezierSegment.cs
- ListBoxAutomationPeer.cs
- XmlEncodedRawTextWriter.cs
- UIElementHelper.cs
- TransformPattern.cs
- ListDictionary.cs
- SoapProtocolImporter.cs
- ColorMap.cs
- TemplateBindingExpression.cs
- glyphs.cs
- Compress.cs
- StylusPointPropertyInfo.cs
- VirtualPath.cs
- EncoderParameter.cs
- CodeNamespaceImportCollection.cs
- OracleDataReader.cs
- DataGridPagerStyle.cs
- ConfigurationStrings.cs
- ScriptingRoleServiceSection.cs
- SoapExtensionTypeElement.cs
- DrawingContextWalker.cs
- GiveFeedbackEvent.cs
- EntityContainerAssociationSet.cs
- NumberSubstitution.cs
- FrameworkObject.cs
- Timer.cs
- BooleanExpr.cs
- UpdatePanelTrigger.cs
- PointIndependentAnimationStorage.cs
- MemberPath.cs
- DataGridView.cs
- ExportException.cs
- StringValidator.cs
- RuntimeCompatibilityAttribute.cs
- CheckBoxPopupAdapter.cs
- InternalControlCollection.cs
- ColorAnimationBase.cs
- PackageRelationship.cs
- PackageRelationship.cs
- SmtpLoginAuthenticationModule.cs
- DataSourceProvider.cs
- QuotedStringWriteStateInfo.cs
- counter.cs
- UrlUtility.cs
- SecureStringHasher.cs
- MachineKey.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- DataTableClearEvent.cs
- EmptyImpersonationContext.cs
- FormViewDeleteEventArgs.cs
- Control.cs
- BeginEvent.cs
- WorkflowIdleElement.cs
- InvalidPropValue.cs
- Debugger.cs
- ButtonChrome.cs
- ProofTokenCryptoHandle.cs
- SweepDirectionValidation.cs
- XsdDataContractImporter.cs
- ModuleConfigurationInfo.cs
- RelationshipEndCollection.cs
- OdbcConnectionHandle.cs
- DataSourceXmlSerializationAttribute.cs
- Panel.cs
- CustomErrorCollection.cs
- DependencyPropertyAttribute.cs
- ListViewTableRow.cs
- DBSchemaTable.cs
- ClientType.cs
- ProfileBuildProvider.cs
- TextSearch.cs
- InternalConfigHost.cs
- ResourceCodeDomSerializer.cs
- BlurEffect.cs
- SettingsBindableAttribute.cs
- StrongNameMembershipCondition.cs
- CommonXSendMessage.cs
- ColorAnimationBase.cs
- WrappedReader.cs
- HtmlEmptyTagControlBuilder.cs
- PathSegmentCollection.cs
- AuthenticationModulesSection.cs
- HttpServerVarsCollection.cs
- CachingHintValidation.cs
- Equal.cs
- OleDbReferenceCollection.cs
- RegexRunner.cs
- NumericUpDownAccelerationCollection.cs
- FileClassifier.cs
- CodeDomSerializerException.cs
- FtpWebResponse.cs