Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / XPath / Internal / BooleanExpr.cs / 1 / BooleanExpr.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Xml.Xsl; using System.Diagnostics; using System.Globalization; internal sealed class BooleanExpr : ValueQuery { private Query opnd1; private Query opnd2; private bool isOr; public BooleanExpr(Operator.Op op, Query opnd1, Query opnd2) { Debug.Assert(op == Operator.Op.AND || op == Operator.Op.OR); Debug.Assert(opnd1 != null && opnd2 != null); if (opnd1.StaticType != XPathResultType.Boolean) { opnd1 = new BooleanFunctions(Function.FunctionType.FuncBoolean, opnd1); } if (opnd2.StaticType != XPathResultType.Boolean) { opnd2 = new BooleanFunctions(Function.FunctionType.FuncBoolean, opnd2); } this.opnd1 = opnd1; this.opnd2 = opnd2; isOr = (op == Operator.Op.OR); } private BooleanExpr(BooleanExpr other) : base(other) { this.opnd1 = Clone(other.opnd1); this.opnd2 = Clone(other.opnd2); this.isOr = other.isOr; } public override void SetXsltContext(XsltContext context){ opnd1.SetXsltContext(context); opnd2.SetXsltContext(context); } public override object Evaluate(XPathNodeIterator nodeIterator) { object n1 = opnd1.Evaluate(nodeIterator); if (((bool) n1) == isOr) { return n1; } return opnd2.Evaluate(nodeIterator); } public override XPathNodeIterator Clone() { return new BooleanExpr(this); } public override XPathResultType StaticType { get { return XPathResultType.Boolean; } } public override void PrintQuery(XmlWriter w) { w.WriteStartElement(this.GetType().Name); w.WriteAttributeString("op", (isOr ? Operator.Op.OR : Operator.Op.AND).ToString()); opnd1.PrintQuery(w); opnd2.PrintQuery(w); w.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Xml.Xsl; using System.Diagnostics; using System.Globalization; internal sealed class BooleanExpr : ValueQuery { private Query opnd1; private Query opnd2; private bool isOr; public BooleanExpr(Operator.Op op, Query opnd1, Query opnd2) { Debug.Assert(op == Operator.Op.AND || op == Operator.Op.OR); Debug.Assert(opnd1 != null && opnd2 != null); if (opnd1.StaticType != XPathResultType.Boolean) { opnd1 = new BooleanFunctions(Function.FunctionType.FuncBoolean, opnd1); } if (opnd2.StaticType != XPathResultType.Boolean) { opnd2 = new BooleanFunctions(Function.FunctionType.FuncBoolean, opnd2); } this.opnd1 = opnd1; this.opnd2 = opnd2; isOr = (op == Operator.Op.OR); } private BooleanExpr(BooleanExpr other) : base(other) { this.opnd1 = Clone(other.opnd1); this.opnd2 = Clone(other.opnd2); this.isOr = other.isOr; } public override void SetXsltContext(XsltContext context){ opnd1.SetXsltContext(context); opnd2.SetXsltContext(context); } public override object Evaluate(XPathNodeIterator nodeIterator) { object n1 = opnd1.Evaluate(nodeIterator); if (((bool) n1) == isOr) { return n1; } return opnd2.Evaluate(nodeIterator); } public override XPathNodeIterator Clone() { return new BooleanExpr(this); } public override XPathResultType StaticType { get { return XPathResultType.Boolean; } } public override void PrintQuery(XmlWriter w) { w.WriteStartElement(this.GetType().Name); w.WriteAttributeString("op", (isOr ? Operator.Op.OR : Operator.Op.AND).ToString()); opnd1.PrintQuery(w); opnd2.PrintQuery(w); w.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
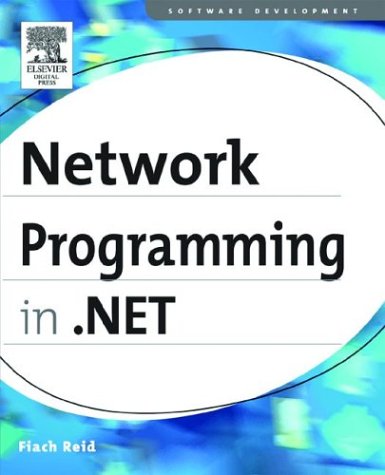
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpWebResponse.cs
- Route.cs
- DynamicDocumentPaginator.cs
- KeyGestureValueSerializer.cs
- WebPartCloseVerb.cs
- AppearanceEditorPart.cs
- SelectionPattern.cs
- FreezableCollection.cs
- PtsPage.cs
- EventDescriptorCollection.cs
- Cursors.cs
- Geometry3D.cs
- CompiledXpathExpr.cs
- DispatcherEventArgs.cs
- SourceChangedEventArgs.cs
- WindowsStartMenu.cs
- SerializationSectionGroup.cs
- HitTestWithPointDrawingContextWalker.cs
- XmlSigningNodeWriter.cs
- InfoCardProofToken.cs
- SimpleRecyclingCache.cs
- UriTemplateClientFormatter.cs
- SerializationAttributes.cs
- LookupBindingPropertiesAttribute.cs
- TypeNameConverter.cs
- InputLangChangeRequestEvent.cs
- PeerCredentialElement.cs
- WsrmMessageInfo.cs
- SimpleHandlerFactory.cs
- _AutoWebProxyScriptHelper.cs
- CriticalHandle.cs
- BindableTemplateBuilder.cs
- Point4DConverter.cs
- SegmentInfo.cs
- ParserStreamGeometryContext.cs
- BoolLiteral.cs
- Line.cs
- FormatterConverter.cs
- HttpHandlerActionCollection.cs
- XsdDataContractExporter.cs
- LinqDataSourceUpdateEventArgs.cs
- DiscreteKeyFrames.cs
- DecimalKeyFrameCollection.cs
- KeyEventArgs.cs
- CookielessHelper.cs
- ResourcePool.cs
- ArrayWithOffset.cs
- CngUIPolicy.cs
- ActivityExecutionContext.cs
- EventEntry.cs
- newitemfactory.cs
- EventHandlersStore.cs
- BaseAddressPrefixFilterElement.cs
- ProfessionalColorTable.cs
- Table.cs
- StringToken.cs
- RuntimeHelpers.cs
- RelatedEnd.cs
- LiteralControl.cs
- ClientEndpointLoader.cs
- TextOutput.cs
- DataBindEngine.cs
- PointCollectionConverter.cs
- ToggleButton.cs
- TablePatternIdentifiers.cs
- MobileCategoryAttribute.cs
- SignatureDescription.cs
- TreeNodeCollection.cs
- PersonalizationProviderHelper.cs
- And.cs
- RtfControlWordInfo.cs
- XPathDocumentBuilder.cs
- StoryFragments.cs
- ProcessThreadCollection.cs
- path.cs
- GridViewColumn.cs
- TextReturnReader.cs
- EntityDataSourceDataSelection.cs
- ICspAsymmetricAlgorithm.cs
- Helpers.cs
- TcpClientChannel.cs
- MethodBuilder.cs
- DragAssistanceManager.cs
- ArglessEventHandlerProxy.cs
- LambdaCompiler.ControlFlow.cs
- HandlerFactoryCache.cs
- HttpWriter.cs
- AnnotationComponentChooser.cs
- AmbientLight.cs
- OlePropertyStructs.cs
- PlanCompilerUtil.cs
- COM2EnumConverter.cs
- XomlCompilerHelpers.cs
- TableProviderWrapper.cs
- TransformValueSerializer.cs
- SqlUdtInfo.cs
- RegexMatchCollection.cs
- OracleInternalConnection.cs
- EntitySqlQueryBuilder.cs
- BindingWorker.cs