Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Ink / ExtendedProperty.cs / 1 / ExtendedProperty.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using MS.Utility; using System; using System.IO; using System.Collections; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters.Binary; using System.Text; using MS.Internal.Ink.InkSerializedFormat; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Ink { ////// Drawing Attribute Key/Value pair for specifying each attribute /// internal sealed class ExtendedProperty { ////// Create a new drawing attribute with the specified key and value /// /// Identifier of attribute /// Attribute value - not that the Type for value is tied to the id ///Value type must be compatible with attribute Id internal ExtendedProperty(Guid id, object value) { if (id == Guid.Empty) { throw new ArgumentException(SR.Get(SRID.InvalidGuid)); } _id = id; Value = value; } ///Returns a value that can be used to store and lookup /// ExtendedProperty objects in a hash table public override int GetHashCode() { return Id.GetHashCode() ^ Value.GetHashCode(); } ///Determine if two ExtendedProperty objects are equal public override bool Equals(object obj) { if (obj == null || obj.GetType() != GetType()) { return false; } ExtendedProperty that = (ExtendedProperty)obj; if (that.Id == this.Id) { Type type1 = this.Value.GetType(); Type type2 = that.Value.GetType(); if (type1.IsArray && type2.IsArray) { Type elementType1 = type1.GetElementType(); Type elementType2 = type2.GetElementType(); if (elementType1 == elementType2 && elementType1.IsValueType && type1.GetArrayRank() == 1 && elementType2.IsValueType && type2.GetArrayRank() == 1) { Array array1 = (Array)this.Value; Array array2 = (Array)that.Value; if (array1.Length == array2.Length) { for (int i = 0; i < array1.Length; i++) { if (!array1.GetValue(i).Equals(array2.GetValue(i))) { return false; } } return true; } } } else { return that.Value.Equals(this.Value); } } return false; } ///Overload of the equality operator for comparing /// two ExtendedProperty objects public static bool operator ==(ExtendedProperty first, ExtendedProperty second) { if ((object)first == null && (object)second == null) { return true; } else if ((object)first == null || (object)second == null) { return false; } else { return first.Equals(second); } } ///Compare two custom attributes for Id and value inequality ///Value comparison is performed based on Value.Equals public static bool operator !=(ExtendedProperty first, ExtendedProperty second) { return !(first == second); } ////// Returns a debugger-friendly version of the ExtendedProperty /// ///public override string ToString() { string val; if (Value == null) { val = " "; } else if (Value is string) { val = "\"" + Value.ToString() + "\""; } else { val = Value.ToString(); } return KnownIds.ConvertToString(Id) + "," + val; } /// /// Retrieve the Identifier, or key, for Drawing Attribute key/value pair /// internal Guid Id { get { return _id; } } ////// Set or retrieve the value for ExtendedProperty key/value pair /// ///Value type must be compatible with attribute Id ///Value can be null. internal object Value { get { return _value; } set { if (value == null) { throw new ArgumentNullException("value"); } // validate the type information for the id against the id ExtendedPropertySerializer.Validate(_id, value); _value = value; } } ////// Creates a copy of the Guid and Value /// ///internal ExtendedProperty Clone() { // // the only properties we accept are value types or arrays of // value types with the exception of string. // Guid guid = _id; //Guid is a ValueType that copies on assignment Type type = _value.GetType(); // // check for the very common, copy on assignment // types (ValueType or string) // if (type.IsValueType || type == typeof(string)) { // // either ValueType or string is passed by value // return new ExtendedProperty(guid, _value); } else if (type.IsArray) { Type elementType = type.GetElementType(); if (elementType.IsValueType && type.GetArrayRank() == 1) { // // copy the array memebers, which we know are copy // on assignment value types // Array newArray = Array.CreateInstance(elementType, ((Array)_value).Length); Array.Copy((Array)_value, newArray, ((Array)_value).Length); return new ExtendedProperty(guid, newArray); } } // // we didn't find a type we expect, throw // throw new InvalidOperationException(SR.Get(SRID.InvalidDataTypeForExtendedProperty)); } private Guid _id; // id of attribute private object _value; // data in attribute } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
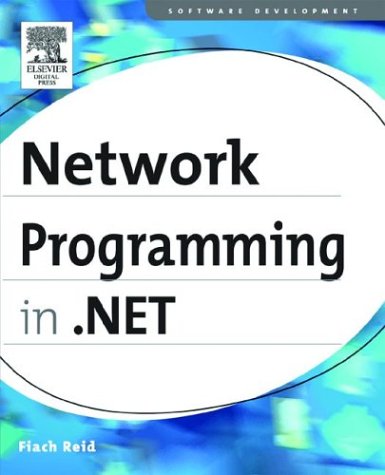
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FillRuleValidation.cs
- WebConfigurationManager.cs
- VisualCollection.cs
- SimplePropertyEntry.cs
- WebPartsPersonalization.cs
- Barrier.cs
- SafeWaitHandle.cs
- WorkflowRuntimeElement.cs
- Image.cs
- UrlMapping.cs
- DBSchemaRow.cs
- ScrollViewerAutomationPeer.cs
- parserscommon.cs
- SspiSecurityToken.cs
- CookieParameter.cs
- HtmlForm.cs
- XsdDateTime.cs
- Row.cs
- RepeaterItemEventArgs.cs
- BaseDataListActionList.cs
- SchemaCollectionPreprocessor.cs
- DataGridViewTextBoxEditingControl.cs
- ObjectIDGenerator.cs
- PathParser.cs
- AssemblyCache.cs
- rsa.cs
- SqlDataReaderSmi.cs
- ErrorHandler.cs
- TextStore.cs
- SQLBoolean.cs
- XmlSchemaSubstitutionGroup.cs
- GCHandleCookieTable.cs
- LinkTarget.cs
- DispatcherObject.cs
- CodeSnippetStatement.cs
- SQLResource.cs
- WindowsIdentity.cs
- MemberListBinding.cs
- EntityCollectionChangedParams.cs
- XmlSchemaFacet.cs
- ChtmlPhoneCallAdapter.cs
- UIElement3D.cs
- ResXFileRef.cs
- DocComment.cs
- DefaultAuthorizationContext.cs
- DataGridViewCellStyle.cs
- ComboBoxAutomationPeer.cs
- XmlNamespaceMapping.cs
- DesignerActionVerbItem.cs
- ExcCanonicalXml.cs
- UIInitializationException.cs
- WebBrowserUriTypeConverter.cs
- EntityContainerEmitter.cs
- ColorAnimationUsingKeyFrames.cs
- SortDescription.cs
- SqlMethodAttribute.cs
- BuildProviderUtils.cs
- ReadOnlyHierarchicalDataSource.cs
- ImmComposition.cs
- DuplexChannel.cs
- ListenerServiceInstallComponent.cs
- SoapFormatExtensions.cs
- HealthMonitoringSectionHelper.cs
- PageRouteHandler.cs
- BinaryParser.cs
- CheckedPointers.cs
- CompositeKey.cs
- SubclassTypeValidator.cs
- SrgsToken.cs
- VirtualPathUtility.cs
- RuntimeResourceSet.cs
- LogSwitch.cs
- XmlSchemaSimpleType.cs
- XPathDocument.cs
- XpsException.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- TextDocumentView.cs
- JavaScriptSerializer.cs
- MaterialGroup.cs
- sqlser.cs
- DocumentApplicationJournalEntry.cs
- SerialPort.cs
- Hash.cs
- DataGridViewRowsRemovedEventArgs.cs
- Processor.cs
- BamlRecordReader.cs
- SqlCachedBuffer.cs
- IfAction.cs
- RegistrySecurity.cs
- GenericAuthenticationEventArgs.cs
- ColumnTypeConverter.cs
- PreviewPrintController.cs
- MimeTypeAttribute.cs
- SqlErrorCollection.cs
- WmfPlaceableFileHeader.cs
- VirtualPathProvider.cs
- ThousandthOfEmRealDoubles.cs
- HtmlWindowCollection.cs
- X509IssuerSerialKeyIdentifierClause.cs
- MailDefinition.cs