Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / XPath / Internal / NodeFunctions.cs / 1 / NodeFunctions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Globalization; using System.Xml.Xsl; using FT = MS.Internal.Xml.XPath.Function.FunctionType; internal sealed class NodeFunctions : ValueQuery { Query arg = null; FT funcType; XsltContext xsltContext; public NodeFunctions(FT funcType, Query arg) { this.funcType = funcType; this.arg = arg; } public override void SetXsltContext(XsltContext context){ this.xsltContext = context.Whitespace ? context : null; if (arg != null) { arg.SetXsltContext(context); } } private XPathNavigator EvaluateArg(XPathNodeIterator context) { if (arg == null) { return context.Current; } arg.Evaluate(context); return arg.Advance(); } public override object Evaluate(XPathNodeIterator context) { XPathNavigator argVal; switch (funcType) { case FT.FuncPosition: return (double)context.CurrentPosition; case FT.FuncLast: return (double)context.Count; case FT.FuncNameSpaceUri: argVal = EvaluateArg(context); if (argVal != null) { return argVal.NamespaceURI; } break; case FT.FuncLocalName: argVal = EvaluateArg(context); if (argVal != null) { return argVal.LocalName; } break; case FT.FuncName : argVal = EvaluateArg(context); if (argVal != null) { return argVal.Name; } break; case FT.FuncCount: arg.Evaluate(context); int count = 0; if (xsltContext != null) { XPathNavigator nav; while ((nav = arg.Advance()) != null) { if (nav.NodeType != XPathNodeType.Whitespace || xsltContext.PreserveWhitespace(nav)) { count++; } } } else { while (arg.Advance() != null) { count++; } } return (double) count; } return string.Empty; } public override XPathResultType StaticType { get { return Function.ReturnTypes[(int)funcType]; } } public override XPathNodeIterator Clone() { NodeFunctions method = new NodeFunctions(funcType, Clone(arg)); method.xsltContext = this.xsltContext; return method; } public override void PrintQuery(XmlWriter w) { w.WriteStartElement(this.GetType().Name); w.WriteAttributeString("name", funcType.ToString()); if (arg != null) { arg.PrintQuery(w); } w.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
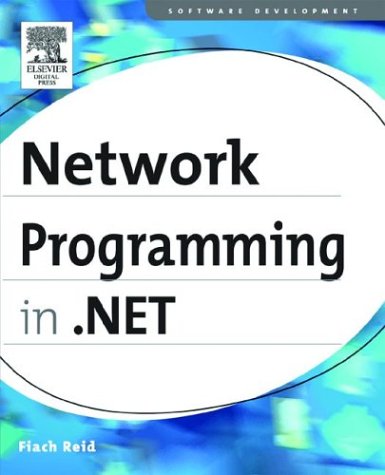
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListCollectionView.cs
- NamespaceEmitter.cs
- PointCollection.cs
- SafeFindHandle.cs
- SizeAnimationClockResource.cs
- ConstraintCollection.cs
- DesignRelationCollection.cs
- ElementAtQueryOperator.cs
- DeclarationUpdate.cs
- ToolStripScrollButton.cs
- TdsEnums.cs
- OdbcDataReader.cs
- ChangeBlockUndoRecord.cs
- DecoderNLS.cs
- DoubleUtil.cs
- SmiXetterAccessMap.cs
- LingerOption.cs
- AlignmentXValidation.cs
- ValueUtilsSmi.cs
- SchemaImporterExtensionsSection.cs
- PointConverter.cs
- PrincipalPermission.cs
- DispatchChannelSink.cs
- WaitingCursor.cs
- ButtonRenderer.cs
- ControlBuilder.cs
- TextBox.cs
- WindowsRichEdit.cs
- InertiaTranslationBehavior.cs
- sqlmetadatafactory.cs
- SessionEndedEventArgs.cs
- XsltLibrary.cs
- ValidationErrorEventArgs.cs
- StructuralType.cs
- WindowsAltTab.cs
- TreeViewHitTestInfo.cs
- UnsafeNativeMethods.cs
- AmbientLight.cs
- HtmlTableCell.cs
- ProxyGenerationError.cs
- JavascriptCallbackResponseProperty.cs
- COM2ComponentEditor.cs
- WebPartDisplayModeCancelEventArgs.cs
- SystemIPGlobalProperties.cs
- TextElement.cs
- precedingquery.cs
- RoleManagerSection.cs
- OutputCacheProfile.cs
- PeerTransportSecuritySettings.cs
- ServicePoint.cs
- CompiledQueryCacheKey.cs
- ExcludeFromCodeCoverageAttribute.cs
- CriticalFinalizerObject.cs
- UpdateInfo.cs
- NonSerializedAttribute.cs
- AdPostCacheSubstitution.cs
- CodeRemoveEventStatement.cs
- MultiView.cs
- Helpers.cs
- ClientRoleProvider.cs
- NamedObject.cs
- WinCategoryAttribute.cs
- Compilation.cs
- ManipulationStartingEventArgs.cs
- Win32Exception.cs
- ReadContentAsBinaryHelper.cs
- FactoryId.cs
- XmlSchemaGroup.cs
- DataGridViewMethods.cs
- HtmlInputReset.cs
- OracleParameterBinding.cs
- TrackingRecord.cs
- OdbcReferenceCollection.cs
- TextEffectResolver.cs
- CommonRemoteMemoryBlock.cs
- StreamResourceInfo.cs
- ThrowHelper.cs
- LinkButton.cs
- Int16Storage.cs
- EventManager.cs
- PerformanceCounter.cs
- HttpMethodAttribute.cs
- BitmapEffectDrawingContextState.cs
- BinaryReader.cs
- BevelBitmapEffect.cs
- Emitter.cs
- IdnElement.cs
- CookieHandler.cs
- EvidenceBase.cs
- WindowsGraphicsWrapper.cs
- XmlElementCollection.cs
- CmsInterop.cs
- TriState.cs
- ChannelDemuxer.cs
- Crypto.cs
- BevelBitmapEffect.cs
- TextInfo.cs
- StylusPoint.cs
- SQLInt32Storage.cs
- PreProcessInputEventArgs.cs