Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / ButtonRenderer.cs / 1 / ButtonRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class ButtonRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement ButtonElement = VisualStyleElement.Button.PushButton.Normal; private static bool renderMatchingApplicationState = true; //cannot instantiate private ButtonRenderer() { } ////// This is a rendering class for the Button control. It works downlevel too (obviously /// without visual styles applied.) /// ////// /// public static bool RenderMatchingApplicationState { get { return renderMatchingApplicationState; } set { renderMatchingApplicationState = value; } } private static bool RenderWithVisualStyles { get { return (!renderMatchingApplicationState || Application.RenderWithVisualStyles); } } ////// If this property is true, then the renderer will use the setting from Application.RenderWithVisualStyles to /// determine how to render. /// If this property is false, the renderer will always render with visualstyles. /// ////// /// public static bool IsBackgroundPartiallyTransparent(PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); return visualStyleRenderer.IsBackgroundPartiallyTransparent(); } else { return false; //for downlevel, this is false } } ////// Returns true if the background corresponding to the given state is partially transparent, else false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] public static void DrawParentBackground(Graphics g, Rectangle bounds, Control childControl) { if (RenderWithVisualStyles) { InitializeRenderer(0); visualStyleRenderer.DrawParentBackground(g, bounds, childControl); } } ////// This is just a convenience wrapper for VisualStyleRenderer.DrawThemeParentBackground. For downlevel, /// this isn't required and does nothing. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageBounds, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } internal static ButtonState ConvertToButtonState(PushButtonState state) { switch (state) { case PushButtonState.Pressed: return ButtonState.Pushed; case PushButtonState.Disabled: return ButtonState.Inactive; default: return ButtonState.Normal; } } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(ButtonElement.ClassName, ButtonElement.Part, state); } else { visualStyleRenderer.SetParameters(ButtonElement.ClassName, ButtonElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Renders a Button control. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class ButtonRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement ButtonElement = VisualStyleElement.Button.PushButton.Normal; private static bool renderMatchingApplicationState = true; //cannot instantiate private ButtonRenderer() { } ////// This is a rendering class for the Button control. It works downlevel too (obviously /// without visual styles applied.) /// ////// /// public static bool RenderMatchingApplicationState { get { return renderMatchingApplicationState; } set { renderMatchingApplicationState = value; } } private static bool RenderWithVisualStyles { get { return (!renderMatchingApplicationState || Application.RenderWithVisualStyles); } } ////// If this property is true, then the renderer will use the setting from Application.RenderWithVisualStyles to /// determine how to render. /// If this property is false, the renderer will always render with visualstyles. /// ////// /// public static bool IsBackgroundPartiallyTransparent(PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); return visualStyleRenderer.IsBackgroundPartiallyTransparent(); } else { return false; //for downlevel, this is false } } ////// Returns true if the background corresponding to the given state is partially transparent, else false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] public static void DrawParentBackground(Graphics g, Rectangle bounds, Control childControl) { if (RenderWithVisualStyles) { InitializeRenderer(0); visualStyleRenderer.DrawParentBackground(g, bounds, childControl); } } ////// This is just a convenience wrapper for VisualStyleRenderer.DrawThemeParentBackground. For downlevel, /// this isn't required and does nothing. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, PushButtonState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); } if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { DrawButton(g, bounds, buttonText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageBounds, focused, state); } ////// Renders a Button control. /// ////// /// public static void DrawButton(Graphics g, Rectangle bounds, string buttonText, Font font, TextFormatFlags flags, Image image, Rectangle imageBounds, bool focused, PushButtonState state) { Rectangle contentBounds; Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, bounds); visualStyleRenderer.DrawImage(g, imageBounds, image); contentBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { ControlPaint.DrawButton(g, bounds, ConvertToButtonState(state)); g.DrawImage(image, imageBounds); contentBounds = Rectangle.Inflate(bounds, -3, -3); textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, buttonText, font, contentBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, contentBounds); } } internal static ButtonState ConvertToButtonState(PushButtonState state) { switch (state) { case PushButtonState.Pressed: return ButtonState.Pushed; case PushButtonState.Disabled: return ButtonState.Inactive; default: return ButtonState.Normal; } } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(ButtonElement.ClassName, ButtonElement.Part, state); } else { visualStyleRenderer.SetParameters(ButtonElement.ClassName, ButtonElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Renders a Button control. /// ///
Link Menu
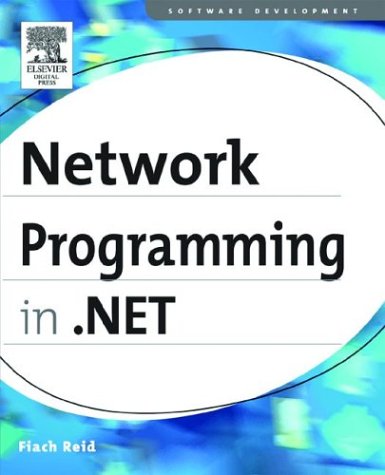
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataCache.cs
- PolicyDesigner.cs
- XamlClipboardData.cs
- PageParserFilter.cs
- CaseCqlBlock.cs
- XmlValidatingReaderImpl.cs
- BuildManagerHost.cs
- ImmutableDispatchRuntime.cs
- SHA1CryptoServiceProvider.cs
- RichTextBoxAutomationPeer.cs
- TraceInternal.cs
- MarkupCompilePass2.cs
- SingleAnimationBase.cs
- DataColumnPropertyDescriptor.cs
- Expander.cs
- TlsSspiNegotiation.cs
- Suspend.cs
- TextRangeEditLists.cs
- WebColorConverter.cs
- PlanCompilerUtil.cs
- DirectoryNotFoundException.cs
- Configuration.cs
- StaticResourceExtension.cs
- SqlStatistics.cs
- FontInfo.cs
- XmlTypeMapping.cs
- WebPartDescriptionCollection.cs
- SiteOfOriginPart.cs
- PrefixQName.cs
- StylusEventArgs.cs
- SettingsPropertyNotFoundException.cs
- LayoutEditorPart.cs
- MembershipSection.cs
- SecureStringHasher.cs
- _NetworkingPerfCounters.cs
- PaperSize.cs
- ProgramPublisher.cs
- XmlSignatureManifest.cs
- BoundField.cs
- HttpVersion.cs
- BaseDataBoundControl.cs
- TokenBasedSetEnumerator.cs
- StrongNameIdentityPermission.cs
- DockingAttribute.cs
- MethodToken.cs
- PrintPreviewGraphics.cs
- TableLayoutStyle.cs
- StringPropertyBuilder.cs
- HttpClientCertificate.cs
- DesignerLinkAdapter.cs
- RegisteredDisposeScript.cs
- EnvironmentPermission.cs
- _BaseOverlappedAsyncResult.cs
- RowToParametersTransformer.cs
- HttpVersion.cs
- _NestedSingleAsyncResult.cs
- ContentHostHelper.cs
- ExeConfigurationFileMap.cs
- HttpListenerException.cs
- DesignTimeParseData.cs
- XmlSchemaChoice.cs
- CreateUserWizardStep.cs
- ReferenceConverter.cs
- AttachInfo.cs
- FixedFlowMap.cs
- DataViewListener.cs
- FormViewPageEventArgs.cs
- ScrollBarRenderer.cs
- IncrementalHitTester.cs
- XmlNodeComparer.cs
- StringDictionaryCodeDomSerializer.cs
- TitleStyle.cs
- DriveInfo.cs
- XmlUtil.cs
- ListBox.cs
- ServiceContractViewControl.cs
- PersonalizationProvider.cs
- ParenthesizePropertyNameAttribute.cs
- WindowsFormsHostAutomationPeer.cs
- RoleBoolean.cs
- LifetimeMonitor.cs
- IndexObject.cs
- PeerNameRecord.cs
- UdpConstants.cs
- AvTraceFormat.cs
- AuthorizationSection.cs
- DataViewListener.cs
- SafeTokenHandle.cs
- HTMLTagNameToTypeMapper.cs
- PersistenceTypeAttribute.cs
- DrawingImage.cs
- TextDecoration.cs
- ToolStripItemTextRenderEventArgs.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- HttpListener.cs
- SharedTcpTransportManager.cs
- IIS7WorkerRequest.cs
- CompositeActivityValidator.cs
- MetadataArtifactLoaderComposite.cs
- XmlSchemaComplexContent.cs