Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiInterop / SapiInterop.cs / 1 / SapiInterop.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Globalization; using System.Runtime.InteropServices; using STATSTG = System.Runtime.InteropServices.ComTypes.STATSTG; using System.Speech.AudioFormat; namespace System.Speech.Internal.SapiInterop { #region Enum internal enum SAPIErrorCodes { S_OK = 0, // 0x00000000 S_FALSE = 1, // 0x00000001 SP_NO_RULE_ACTIVE = 0x00045055, SP_NO_RULES_TO_ACTIVATE = 282747, // 0x0004507B SP_NO_PARSE_FOUND = 0x0004502c, SPERR_FIRST = -2147201023, // 0x80045001 SPERR_LAST = -2147200893, // 0x80045083 //STG_E_FILENOTFOUND = -2147287038, // 0x80030002 CLASS_E_CLASSNOTAVAILABLE = -2147221231, // 0x80040111 REGDB_E_CLASSNOTREG = -2147221164, // 0x80040154 SPERR_UNSUPPORTED_FORMAT = -2147201021, // 0x80045003 //SPERR_DEVICE_BUSY = -2147201018, // 0x80045006 //SPERR_DEVICE_NOT_SUPPORTED = -2147201017, // 0x80045007 //SPERR_DEVICE_NOT_ENABLED = -2147201016, // 0x80045008 //SPERR_NO_DRIVER = -2147201015, // 0x80045009 SPERR_TOO_MANY_GRAMMARS = -2147200990, // 0x80045022 SPERR_INVALID_IMPORT = -2147200988, // 0x80045024 //SPERR_AUDIO_BUFFER_OVERFLOW = -2147200977, // 0x8004502F //SPERR_NO_AUDIO_DATA = -2147200976, // 0x80045030 //SPERR_NO_MORE_ITEMS = -2147200967, // 0x80045039 SPERR_NOT_FOUND = -2147200966, // 0x8004503A //SPERR_GENERIC_MMSYS_ERROR = -2147200964, // 0x8004503C SPERR_NOT_TOPLEVEL_RULE = -2147200940, // 0x80045054 //SPERR_NOT_ACTIVE_SESSION = -2147200925, // 0x80045063 //SPERR_SML_GENERATION_FAIL = -2147200921, // 0x80045067 SPERR_SHARED_ENGINE_DISABLED = -2147200906, // 0x80045076 SPERR_RECOGNIZER_NOT_FOUND = -2147200905, // 0x80045077 SPERR_AUDIO_NOT_FOUND = -2147200904, // 0x80045078 SPERR_NOT_SUPPORTED_FOR_INPROC_RECOGNIZER = -2147200893 // 0x80045083 } #endregion Enum #region SAPI constants internal static class SapiConstants { // SPProperties strings // internal const string SPPROP_RESOURCE_USAGE = "ResourceUsage"; // internal const string SPPROP_ACCURARY_THRESHOLD = "AccuraryThreshold"; //internal const string SPPROP_HIGH_CONFIDENCE_THRESHOLD = "HighConfidenceThreshold"; //internal const string SPPROP_NORMAL_CONFIDENCE_THRESHOLD = "NormalConfidenceThreshold"; //internal const string SPPROP_LOW_CONFIDENCE_THRESHOLD = "LowConfidenceThreshold"; internal const string SPPROP_RESPONSE_SPEED = "ResponseSpeed"; internal const string SPPROP_COMPLEX_RESPONSE_SPEED = "ComplexResponseSpeed"; //internal const string SPPROP_ADAPTATION_ON = "AdaptationOn"; internal const string SPPROP_CFG_CONFIDENCE_REJECTION_THRESHOLD = "CFGConfidenceRejectionThreshold"; // internal const UInt32 SPVALUETYPE // internal const UInt32 SPDF_PROPERTY = 0x1; // internal const UInt32 SPDF_REPLACEMENT = 0x2; // internal const UInt32 SPDF_RULE = 0x4; // internal const UInt32 SPDF_DISPLAYTEXT = 0x8; // internal const UInt32 SPDF_LEXICALFORM = 0x10; // internal const UInt32 SPDF_PRONUNCIATION = 0x20; // internal const UInt32 SPDF_AUDIO = 0x40; // internal const UInt32 SPDF_ALTERNATES = 0x80; internal const UInt32 SPDF_ALL = 0xff; // Throws exception if the specified Rule does not have a valid Id. static internal SRID SapiErrorCode2SRID (SAPIErrorCodes code) { if (code >= SAPIErrorCodes.SPERR_FIRST && code <= SAPIErrorCodes.SPERR_LAST) { return (SRID) ((int) SRID.SapiErrorUninitialized + (code - SAPIErrorCodes.SPERR_FIRST)); } else { switch (code) { case SAPIErrorCodes.SP_NO_RULE_ACTIVE: return SRID.SapiErrorNoRuleActive; case SAPIErrorCodes.SP_NO_RULES_TO_ACTIVATE: return SRID.SapiErrorNoRulesToActivate; case SAPIErrorCodes.SP_NO_PARSE_FOUND: return SRID.NoParseFound; case SAPIErrorCodes.S_FALSE: return SRID.UnexpectedError; default: return (SRID) unchecked (-1); } } } } #endregion #region Utility Class internal static class SAPIGuids { static internal readonly Guid SPDFID_WaveFormatEx = new Guid ("C31ADBAE-527F-4ff5-A230-F62BB61FF70C"); //static internal readonly Guid SPDFID_Text = new Guid ("7CEEF9F9-3D13-11d2-9EE7-00C04F797396"); } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.Globalization; using System.Runtime.InteropServices; using STATSTG = System.Runtime.InteropServices.ComTypes.STATSTG; using System.Speech.AudioFormat; namespace System.Speech.Internal.SapiInterop { #region Enum internal enum SAPIErrorCodes { S_OK = 0, // 0x00000000 S_FALSE = 1, // 0x00000001 SP_NO_RULE_ACTIVE = 0x00045055, SP_NO_RULES_TO_ACTIVATE = 282747, // 0x0004507B SP_NO_PARSE_FOUND = 0x0004502c, SPERR_FIRST = -2147201023, // 0x80045001 SPERR_LAST = -2147200893, // 0x80045083 //STG_E_FILENOTFOUND = -2147287038, // 0x80030002 CLASS_E_CLASSNOTAVAILABLE = -2147221231, // 0x80040111 REGDB_E_CLASSNOTREG = -2147221164, // 0x80040154 SPERR_UNSUPPORTED_FORMAT = -2147201021, // 0x80045003 //SPERR_DEVICE_BUSY = -2147201018, // 0x80045006 //SPERR_DEVICE_NOT_SUPPORTED = -2147201017, // 0x80045007 //SPERR_DEVICE_NOT_ENABLED = -2147201016, // 0x80045008 //SPERR_NO_DRIVER = -2147201015, // 0x80045009 SPERR_TOO_MANY_GRAMMARS = -2147200990, // 0x80045022 SPERR_INVALID_IMPORT = -2147200988, // 0x80045024 //SPERR_AUDIO_BUFFER_OVERFLOW = -2147200977, // 0x8004502F //SPERR_NO_AUDIO_DATA = -2147200976, // 0x80045030 //SPERR_NO_MORE_ITEMS = -2147200967, // 0x80045039 SPERR_NOT_FOUND = -2147200966, // 0x8004503A //SPERR_GENERIC_MMSYS_ERROR = -2147200964, // 0x8004503C SPERR_NOT_TOPLEVEL_RULE = -2147200940, // 0x80045054 //SPERR_NOT_ACTIVE_SESSION = -2147200925, // 0x80045063 //SPERR_SML_GENERATION_FAIL = -2147200921, // 0x80045067 SPERR_SHARED_ENGINE_DISABLED = -2147200906, // 0x80045076 SPERR_RECOGNIZER_NOT_FOUND = -2147200905, // 0x80045077 SPERR_AUDIO_NOT_FOUND = -2147200904, // 0x80045078 SPERR_NOT_SUPPORTED_FOR_INPROC_RECOGNIZER = -2147200893 // 0x80045083 } #endregion Enum #region SAPI constants internal static class SapiConstants { // SPProperties strings // internal const string SPPROP_RESOURCE_USAGE = "ResourceUsage"; // internal const string SPPROP_ACCURARY_THRESHOLD = "AccuraryThreshold"; //internal const string SPPROP_HIGH_CONFIDENCE_THRESHOLD = "HighConfidenceThreshold"; //internal const string SPPROP_NORMAL_CONFIDENCE_THRESHOLD = "NormalConfidenceThreshold"; //internal const string SPPROP_LOW_CONFIDENCE_THRESHOLD = "LowConfidenceThreshold"; internal const string SPPROP_RESPONSE_SPEED = "ResponseSpeed"; internal const string SPPROP_COMPLEX_RESPONSE_SPEED = "ComplexResponseSpeed"; //internal const string SPPROP_ADAPTATION_ON = "AdaptationOn"; internal const string SPPROP_CFG_CONFIDENCE_REJECTION_THRESHOLD = "CFGConfidenceRejectionThreshold"; // internal const UInt32 SPVALUETYPE // internal const UInt32 SPDF_PROPERTY = 0x1; // internal const UInt32 SPDF_REPLACEMENT = 0x2; // internal const UInt32 SPDF_RULE = 0x4; // internal const UInt32 SPDF_DISPLAYTEXT = 0x8; // internal const UInt32 SPDF_LEXICALFORM = 0x10; // internal const UInt32 SPDF_PRONUNCIATION = 0x20; // internal const UInt32 SPDF_AUDIO = 0x40; // internal const UInt32 SPDF_ALTERNATES = 0x80; internal const UInt32 SPDF_ALL = 0xff; // Throws exception if the specified Rule does not have a valid Id. static internal SRID SapiErrorCode2SRID (SAPIErrorCodes code) { if (code >= SAPIErrorCodes.SPERR_FIRST && code <= SAPIErrorCodes.SPERR_LAST) { return (SRID) ((int) SRID.SapiErrorUninitialized + (code - SAPIErrorCodes.SPERR_FIRST)); } else { switch (code) { case SAPIErrorCodes.SP_NO_RULE_ACTIVE: return SRID.SapiErrorNoRuleActive; case SAPIErrorCodes.SP_NO_RULES_TO_ACTIVATE: return SRID.SapiErrorNoRulesToActivate; case SAPIErrorCodes.SP_NO_PARSE_FOUND: return SRID.NoParseFound; case SAPIErrorCodes.S_FALSE: return SRID.UnexpectedError; default: return (SRID) unchecked (-1); } } } } #endregion #region Utility Class internal static class SAPIGuids { static internal readonly Guid SPDFID_WaveFormatEx = new Guid ("C31ADBAE-527F-4ff5-A230-F62BB61FF70C"); //static internal readonly Guid SPDFID_Text = new Guid ("7CEEF9F9-3D13-11d2-9EE7-00C04F797396"); } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
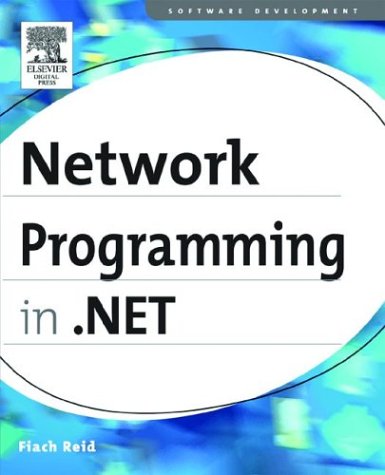
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SignerInfo.cs
- DoubleAnimationClockResource.cs
- JsonGlobals.cs
- DbConnectionPool.cs
- FtpWebRequest.cs
- XmlSchemaDocumentation.cs
- WinFormsUtils.cs
- DependencyPropertyDescriptor.cs
- Context.cs
- ProcessInfo.cs
- XmlSchemaComplexType.cs
- AffineTransform3D.cs
- ToolStripDropTargetManager.cs
- StrokeSerializer.cs
- CallbackValidator.cs
- WebServiceData.cs
- TextRange.cs
- SessionStateContainer.cs
- MethodBody.cs
- XamlPathDataSerializer.cs
- MeasureItemEvent.cs
- CacheChildrenQuery.cs
- ForEachDesigner.xaml.cs
- BindValidator.cs
- ControllableStoryboardAction.cs
- EditorAttribute.cs
- DataSourceCache.cs
- SerializerDescriptor.cs
- DrawListViewSubItemEventArgs.cs
- ElapsedEventArgs.cs
- RightsManagementPermission.cs
- IndexExpression.cs
- Assert.cs
- _TLSstream.cs
- BindUriHelper.cs
- SourceElementsCollection.cs
- COM2PropertyBuilderUITypeEditor.cs
- XmlAnyElementAttributes.cs
- DictionaryChange.cs
- CounterSetInstanceCounterDataSet.cs
- RotateTransform.cs
- CompositionTarget.cs
- SqlDataRecord.cs
- HwndStylusInputProvider.cs
- RewritingProcessor.cs
- UnionCodeGroup.cs
- WindowsContainer.cs
- RightsManagementInformation.cs
- _Rfc2616CacheValidators.cs
- AccessedThroughPropertyAttribute.cs
- GridPattern.cs
- WriteFileContext.cs
- TimeZoneInfo.cs
- ScopelessEnumAttribute.cs
- LineServicesRun.cs
- NativeMethods.cs
- DbDataRecord.cs
- TreeSet.cs
- SubclassTypeValidator.cs
- TimelineClockCollection.cs
- FreezableDefaultValueFactory.cs
- SignatureDescription.cs
- SurrogateEncoder.cs
- _IPv4Address.cs
- AutoGeneratedFieldProperties.cs
- DataGridViewDataConnection.cs
- RouteTable.cs
- URIFormatException.cs
- SpecialFolderEnumConverter.cs
- Journaling.cs
- TimelineCollection.cs
- XPathQilFactory.cs
- ConsoleCancelEventArgs.cs
- XmlSerializationWriter.cs
- XamlPoint3DCollectionSerializer.cs
- FlowDocument.cs
- XmlQueryCardinality.cs
- SafeFileMappingHandle.cs
- MaskedTextBox.cs
- SrgsRule.cs
- SatelliteContractVersionAttribute.cs
- ISAPIWorkerRequest.cs
- BaseCollection.cs
- HttpServerChannel.cs
- OperatingSystem.cs
- CompiledQuery.cs
- ExclusiveTcpTransportManager.cs
- ValidatorCompatibilityHelper.cs
- SimplePropertyEntry.cs
- GacUtil.cs
- Soap11ServerProtocol.cs
- TextDecorationLocationValidation.cs
- MessageHeaderException.cs
- HtmlInputText.cs
- PropertyDescriptorGridEntry.cs
- _Connection.cs
- XPathAxisIterator.cs
- PassportPrincipal.cs
- XpsViewerException.cs
- ModelItemImpl.cs