Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Diagnostics / PerformanceData / CounterSetInstanceCounterDataSet.cs / 1305376 / CounterSetInstanceCounterDataSet.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics.PerformanceData { using System; using System.Threading; using System.Runtime.InteropServices; using System.ComponentModel; using System.Collections.Generic; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; ////// CounterData class is used to store actual raw counter data. It is the value element within /// CounterSetInstanceCounterDataSet, which is part of CounterSetInstance. /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CounterData { unsafe private Int64 * m_offset; ////// CounterData constructor /// /// counterId would come from CounterSet::AddCounter() parameter /// The memory location to store raw counter data //// [System.Security.SecurityCritical] unsafe internal CounterData(Int64 * pCounterData) { m_offset = pCounterData; * m_offset = 0; } ///// // /// Value property it used to query/update actual raw counter data. /// public Int64 Value { //// [System.Security.SecurityCritical] get { unsafe { return Interlocked.Read(ref (* m_offset)); } } //// // [System.Security.SecurityCritical] set { unsafe { Interlocked.Exchange(ref (* m_offset), value); } } } [System.Security.SecurityCritical] public void Increment() { unsafe { Interlocked.Increment (ref (*m_offset)); } } [System.Security.SecurityCritical] public void Decrement() { unsafe { Interlocked.Decrement (ref (*m_offset)); } } [System.Security.SecurityCritical] public void IncrementBy(Int64 value) { unsafe { Interlocked.Add (ref (*m_offset), value); } } ///// /// RawValue property it used to query/update actual raw counter data. /// This property is not thread-safe and should only be used /// for performance-critical single-threaded access. /// public Int64 RawValue { //// [System.Security.SecurityCritical] get { unsafe { return (* m_offset); } } //// // [System.Security.SecurityCritical] set { unsafe { * m_offset = value; } } } } ///// /// CounterSetInstanceCounterDataSet is part of CounterSetInstance class, and is used to store raw counter data /// for all counters added in CounterSet. /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CounterSetInstanceCounterDataSet : IDisposable { internal CounterSetInstance m_instance; private Dictionarym_counters; private Int32 m_disposed; unsafe internal byte * m_dataBlock; // // [System.Security.SecurityCritical] [SuppressMessage("Microsoft.Usage", "CA2208:InstantiateArgumentExceptionsCorrectly")] internal CounterSetInstanceCounterDataSet(CounterSetInstance thisInst) { m_instance = thisInst; m_counters = new Dictionary// // // // // // // // // (); unsafe { if (m_instance.m_counterSet.m_provider == null) { throw new ArgumentException(SR.GetString(SR.Perflib_Argument_ProviderNotFound, m_instance.m_counterSet.m_providerGuid), "ProviderGuid"); } if (m_instance.m_counterSet.m_provider.m_hProvider.IsInvalid) { throw new InvalidOperationException(SR.GetString(SR.Perflib_InvalidOperation_NoActiveProvider, m_instance.m_counterSet.m_providerGuid)); } m_dataBlock = (byte *) Marshal.AllocHGlobal(m_instance.m_counterSet.m_idToCounter.Count * sizeof(Int64)); if (m_dataBlock == null) { throw new InsufficientMemoryException(SR.GetString(SR.Perflib_InsufficientMemory_InstanceCounterBlock, m_instance.m_counterSet.m_counterSet, m_instance.m_instName)); } Int32 CounterOffset = 0; foreach (KeyValuePair CounterDef in m_instance.m_counterSet.m_idToCounter) { CounterData thisCounterData = new CounterData((Int64 *) (m_dataBlock + CounterOffset * sizeof(Int64))); m_counters.Add(CounterDef.Key, thisCounterData); // ArgumentNullException - CounterName is NULL // ArgumentException - CounterName already exists. uint Status = UnsafeNativeMethods.PerfSetCounterRefValue( m_instance.m_counterSet.m_provider.m_hProvider, m_instance.m_nativeInst, (uint) CounterDef.Key, (void *) (m_dataBlock + CounterOffset * sizeof(Int64))); if (Status != (uint) UnsafeNativeMethods.ERROR_SUCCESS) { Dispose(true); // ERROR_INVALID_PARAMETER or ERROR_NOT_FOUND switch (Status) { case (uint) UnsafeNativeMethods.ERROR_NOT_FOUND: throw new InvalidOperationException(SR.GetString(SR.Perflib_InvalidOperation_CounterRefValue, m_instance.m_counterSet.m_counterSet, CounterDef.Key, m_instance.m_instName)); default: throw new Win32Exception((int) Status); } } CounterOffset ++; } } } // // [System.Security.SecurityCritical] public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } //// // [System.Security.SecurityCritical] ~CounterSetInstanceCounterDataSet() { Dispose(false); } //// // [System.Security.SecurityCritical] private void Dispose(bool disposing) { if (Interlocked.Exchange(ref m_disposed, 1) == 0) { unsafe { if (m_dataBlock != null) { // Need to free allocated heap memory that is used to store all raw counter data. Marshal.FreeHGlobal((System.IntPtr)m_dataBlock); m_dataBlock = null; } } } } ///// // /// CounterId indexer to access specific CounterData object. /// /// CounterId that matches one CounterSet::AddCounter()call ///CounterData object with matched counterId public CounterData this[Int32 counterId] { get { if (m_disposed != 0) { return null; } try { return m_counters[counterId]; } catch (KeyNotFoundException) { return null; } catch { throw; } } } ////// CounterName indexer to access specific CounterData object. /// /// CounterName that matches one CounterSet::AddCounter() call ///CounterData object with matched counterName [SuppressMessage("Microsoft.Usage", "CA2208:InstantiateArgumentExceptionsCorrectly")] public CounterData this[String counterName] { get { if (counterName == null) { throw new ArgumentNullException("CounterName"); } if (counterName.Length == 0) { throw new ArgumentNullException("CounterName"); } if (m_disposed != 0) { return null; } try { Int32 CounterId = m_instance.m_counterSet.m_stringToId[counterName]; try { return m_counters[CounterId]; } catch (KeyNotFoundException) { return null; } catch { throw; } } catch (KeyNotFoundException) { return null; } catch { throw; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Diagnostics.PerformanceData { using System; using System.Threading; using System.Runtime.InteropServices; using System.ComponentModel; using System.Collections.Generic; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using Microsoft.Win32; ////// CounterData class is used to store actual raw counter data. It is the value element within /// CounterSetInstanceCounterDataSet, which is part of CounterSetInstance. /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CounterData { unsafe private Int64 * m_offset; ////// CounterData constructor /// /// counterId would come from CounterSet::AddCounter() parameter /// The memory location to store raw counter data //// [System.Security.SecurityCritical] unsafe internal CounterData(Int64 * pCounterData) { m_offset = pCounterData; * m_offset = 0; } ///// // /// Value property it used to query/update actual raw counter data. /// public Int64 Value { //// [System.Security.SecurityCritical] get { unsafe { return Interlocked.Read(ref (* m_offset)); } } //// // [System.Security.SecurityCritical] set { unsafe { Interlocked.Exchange(ref (* m_offset), value); } } } [System.Security.SecurityCritical] public void Increment() { unsafe { Interlocked.Increment (ref (*m_offset)); } } [System.Security.SecurityCritical] public void Decrement() { unsafe { Interlocked.Decrement (ref (*m_offset)); } } [System.Security.SecurityCritical] public void IncrementBy(Int64 value) { unsafe { Interlocked.Add (ref (*m_offset), value); } } ///// /// RawValue property it used to query/update actual raw counter data. /// This property is not thread-safe and should only be used /// for performance-critical single-threaded access. /// public Int64 RawValue { //// [System.Security.SecurityCritical] get { unsafe { return (* m_offset); } } //// // [System.Security.SecurityCritical] set { unsafe { * m_offset = value; } } } } ///// /// CounterSetInstanceCounterDataSet is part of CounterSetInstance class, and is used to store raw counter data /// for all counters added in CounterSet. /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class CounterSetInstanceCounterDataSet : IDisposable { internal CounterSetInstance m_instance; private Dictionarym_counters; private Int32 m_disposed; unsafe internal byte * m_dataBlock; // // [System.Security.SecurityCritical] [SuppressMessage("Microsoft.Usage", "CA2208:InstantiateArgumentExceptionsCorrectly")] internal CounterSetInstanceCounterDataSet(CounterSetInstance thisInst) { m_instance = thisInst; m_counters = new Dictionary// // // // // // // // // (); unsafe { if (m_instance.m_counterSet.m_provider == null) { throw new ArgumentException(SR.GetString(SR.Perflib_Argument_ProviderNotFound, m_instance.m_counterSet.m_providerGuid), "ProviderGuid"); } if (m_instance.m_counterSet.m_provider.m_hProvider.IsInvalid) { throw new InvalidOperationException(SR.GetString(SR.Perflib_InvalidOperation_NoActiveProvider, m_instance.m_counterSet.m_providerGuid)); } m_dataBlock = (byte *) Marshal.AllocHGlobal(m_instance.m_counterSet.m_idToCounter.Count * sizeof(Int64)); if (m_dataBlock == null) { throw new InsufficientMemoryException(SR.GetString(SR.Perflib_InsufficientMemory_InstanceCounterBlock, m_instance.m_counterSet.m_counterSet, m_instance.m_instName)); } Int32 CounterOffset = 0; foreach (KeyValuePair CounterDef in m_instance.m_counterSet.m_idToCounter) { CounterData thisCounterData = new CounterData((Int64 *) (m_dataBlock + CounterOffset * sizeof(Int64))); m_counters.Add(CounterDef.Key, thisCounterData); // ArgumentNullException - CounterName is NULL // ArgumentException - CounterName already exists. uint Status = UnsafeNativeMethods.PerfSetCounterRefValue( m_instance.m_counterSet.m_provider.m_hProvider, m_instance.m_nativeInst, (uint) CounterDef.Key, (void *) (m_dataBlock + CounterOffset * sizeof(Int64))); if (Status != (uint) UnsafeNativeMethods.ERROR_SUCCESS) { Dispose(true); // ERROR_INVALID_PARAMETER or ERROR_NOT_FOUND switch (Status) { case (uint) UnsafeNativeMethods.ERROR_NOT_FOUND: throw new InvalidOperationException(SR.GetString(SR.Perflib_InvalidOperation_CounterRefValue, m_instance.m_counterSet.m_counterSet, CounterDef.Key, m_instance.m_instName)); default: throw new Win32Exception((int) Status); } } CounterOffset ++; } } } // // [System.Security.SecurityCritical] public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } //// // [System.Security.SecurityCritical] ~CounterSetInstanceCounterDataSet() { Dispose(false); } //// // [System.Security.SecurityCritical] private void Dispose(bool disposing) { if (Interlocked.Exchange(ref m_disposed, 1) == 0) { unsafe { if (m_dataBlock != null) { // Need to free allocated heap memory that is used to store all raw counter data. Marshal.FreeHGlobal((System.IntPtr)m_dataBlock); m_dataBlock = null; } } } } ///// // /// CounterId indexer to access specific CounterData object. /// /// CounterId that matches one CounterSet::AddCounter()call ///CounterData object with matched counterId public CounterData this[Int32 counterId] { get { if (m_disposed != 0) { return null; } try { return m_counters[counterId]; } catch (KeyNotFoundException) { return null; } catch { throw; } } } ////// CounterName indexer to access specific CounterData object. /// /// CounterName that matches one CounterSet::AddCounter() call ///CounterData object with matched counterName [SuppressMessage("Microsoft.Usage", "CA2208:InstantiateArgumentExceptionsCorrectly")] public CounterData this[String counterName] { get { if (counterName == null) { throw new ArgumentNullException("CounterName"); } if (counterName.Length == 0) { throw new ArgumentNullException("CounterName"); } if (m_disposed != 0) { return null; } try { Int32 CounterId = m_instance.m_counterSet.m_stringToId[counterName]; try { return m_counters[CounterId]; } catch (KeyNotFoundException) { return null; } catch { throw; } } catch (KeyNotFoundException) { return null; } catch { throw; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
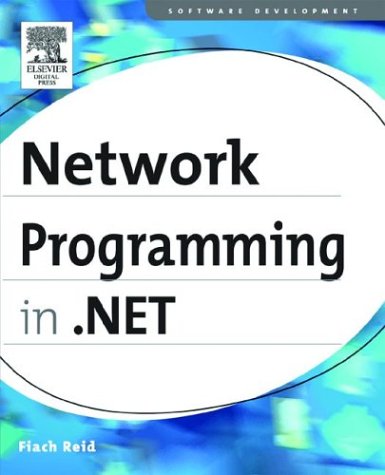
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SelectorItemAutomationPeer.cs
- JsonWriterDelegator.cs
- TypeConverterValueSerializer.cs
- AssemblyAssociatedContentFileAttribute.cs
- TextProviderWrapper.cs
- HtmlInputImage.cs
- SqlNotificationRequest.cs
- AnnotationResourceCollection.cs
- RoutedCommand.cs
- SecurityContext.cs
- EntityDataSourceDesignerHelper.cs
- Accessors.cs
- ColumnWidthChangingEvent.cs
- DataTemplateSelector.cs
- GenerateTemporaryTargetAssembly.cs
- BitmapData.cs
- ScrollChrome.cs
- SystemWebExtensionsSectionGroup.cs
- TypeReference.cs
- Matrix3DValueSerializer.cs
- ChannelServices.cs
- ChangeDirector.cs
- CodeBinaryOperatorExpression.cs
- UnknownBitmapDecoder.cs
- TcpChannelHelper.cs
- DetailsViewDeletedEventArgs.cs
- VSWCFServiceContractGenerator.cs
- ToolStripGripRenderEventArgs.cs
- InvokeProviderWrapper.cs
- SafeProcessHandle.cs
- DrawListViewColumnHeaderEventArgs.cs
- GPPOINT.cs
- FamilyMap.cs
- DataServiceRequest.cs
- URLString.cs
- CancellationToken.cs
- WindowsRichEditRange.cs
- WebPartTransformerAttribute.cs
- SmtpNegotiateAuthenticationModule.cs
- LexicalChunk.cs
- Accessible.cs
- EventHandlerService.cs
- AtomContentProperty.cs
- ClientFormsAuthenticationCredentials.cs
- WebMessageEncodingBindingElement.cs
- XmlDomTextWriter.cs
- TagMapCollection.cs
- SafeArrayRankMismatchException.cs
- BaseDataBoundControl.cs
- KerberosRequestorSecurityToken.cs
- CodeAccessPermission.cs
- DisplayMemberTemplateSelector.cs
- TextSpan.cs
- OdbcCommand.cs
- _OverlappedAsyncResult.cs
- ReadOnlyHierarchicalDataSourceView.cs
- WebPartAuthorizationEventArgs.cs
- StructuredCompositeActivityDesigner.cs
- DesignerObject.cs
- NodeInfo.cs
- ScrollBarRenderer.cs
- SaveFileDialog.cs
- RegistrySecurity.cs
- GridViewAutomationPeer.cs
- _LoggingObject.cs
- MetafileHeaderEmf.cs
- ExtendedPropertiesHandler.cs
- ThicknessAnimationBase.cs
- DodSequenceMerge.cs
- complextypematerializer.cs
- SetterTriggerConditionValueConverter.cs
- BaseHashHelper.cs
- ResourceReferenceExpression.cs
- MsmqAppDomainProtocolHandler.cs
- NamedElement.cs
- OpenFileDialog.cs
- InteropTrackingRecord.cs
- JoinSymbol.cs
- BitmapImage.cs
- ExpressionReplacer.cs
- InfoCardSymmetricAlgorithm.cs
- Misc.cs
- HierarchicalDataBoundControl.cs
- FileDialogCustomPlace.cs
- RolePrincipal.cs
- MiniCustomAttributeInfo.cs
- JpegBitmapEncoder.cs
- C14NUtil.cs
- ListControlConvertEventArgs.cs
- VectorCollectionConverter.cs
- DeviceContexts.cs
- DataGridViewRowEventArgs.cs
- XmlnsDictionary.cs
- FormParameter.cs
- WebPart.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- TimeZone.cs
- EventLogPermissionEntryCollection.cs
- FrameworkElement.cs
- WindowsTreeView.cs