Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / BaseHashHelper.cs / 1305600 / BaseHashHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // // // // Description: Static class to help work around hashing-related bugs. // //--------------------------------------------------------------------------- using System; using System.Reflection; // Assembly using System.Collections.Specialized; // HybridDictionary using MS.Internal.WindowsBase; // [FriendAccessAllowed] namespace MS.Internal { [FriendAccessAllowed] // defined in Base, used in Core and Framework internal static class BaseHashHelper { static BaseHashHelper() { // register bad types from WindowsBase MS.Internal.Hashing.WindowsBase.HashHelper.Initialize(); } [FriendAccessAllowed] // defined in Base, used in Core and Framework internal static void RegisterTypes(Assembly assembly, Type[] types) { HybridDictionary dictionary = DictionaryFromList(types); lock(_table) { _table[assembly] = dictionary; } } // Some types don't have reliable hash codes - the hashcode can change // during the lifetime of an object of that type. Such an object cannot // be used as the key of a hashtable or dictionary. This is where we // detect such objects, so the caller can find some other way to cope. [FriendAccessAllowed] // defined in Base, used in Core and Framework internal static bool HasReliableHashCode(object item) { // null doesn't actually have a hashcode at all. This method can be // called with a representative item from a collection - if the // representative is null, we'll be pessimistic and assume the // items in the collection should not be hashed. if (item == null) return false; Type type = item.GetType(); Assembly assembly = type.Assembly; HybridDictionary dictionary; lock(_table) { dictionary = (HybridDictionary)_table[assembly]; } if (dictionary == null) { dictionary = new HybridDictionary(); lock(_table) { _table[assembly] = dictionary; } } return !dictionary.Contains(type); } // populate a dictionary from the given list private static HybridDictionary DictionaryFromList(Type[] types) { HybridDictionary dictionary = new HybridDictionary(types.Length); for (int i=0; i
Link Menu
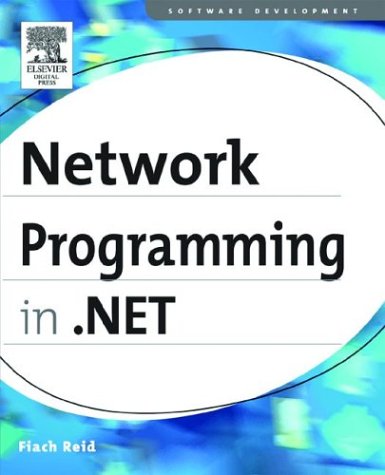
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FunctionUpdateCommand.cs
- iisPickupDirectory.cs
- ThreadStartException.cs
- COM2PictureConverter.cs
- DbConnectionPoolOptions.cs
- GeneralTransform3DCollection.cs
- DrawListViewItemEventArgs.cs
- WindowShowOrOpenTracker.cs
- XmlEventCache.cs
- ListViewItemCollectionEditor.cs
- TiffBitmapDecoder.cs
- PcmConverter.cs
- ProxyHelper.cs
- RowParagraph.cs
- XPathDocumentIterator.cs
- SmiContextFactory.cs
- CodeCommentStatement.cs
- documentsequencetextcontainer.cs
- CacheRequest.cs
- DataContext.cs
- DataContractSerializer.cs
- DataServiceRequestException.cs
- JsonSerializer.cs
- EventHandlerList.cs
- XmlHierarchicalEnumerable.cs
- EncodedStreamFactory.cs
- BinaryObjectReader.cs
- CustomSignedXml.cs
- ZipIOLocalFileDataDescriptor.cs
- UniformGrid.cs
- CodeTypeConstructor.cs
- _UriSyntax.cs
- DetailsViewModeEventArgs.cs
- Accessible.cs
- AccessDataSource.cs
- CodeDomConfigurationHandler.cs
- DomainConstraint.cs
- ResponseBodyWriter.cs
- MatrixKeyFrameCollection.cs
- ADMembershipUser.cs
- XmlIgnoreAttribute.cs
- BoundColumn.cs
- SortedList.cs
- LinkConverter.cs
- IriParsingElement.cs
- CompletionProxy.cs
- DesignerObjectListAdapter.cs
- DataControlButton.cs
- SqlAggregateChecker.cs
- TypeReference.cs
- SqlExpressionNullability.cs
- SessionViewState.cs
- ProvidePropertyAttribute.cs
- RIPEMD160.cs
- SevenBitStream.cs
- ThaiBuddhistCalendar.cs
- SequenceFullException.cs
- Restrictions.cs
- ResourcesGenerator.cs
- StylusOverProperty.cs
- RegexGroup.cs
- SchemaName.cs
- RelatedView.cs
- SoapAttributeOverrides.cs
- PnrpPeerResolverElement.cs
- TabPanel.cs
- SimpleType.cs
- CallbackCorrelationInitializer.cs
- ListView.cs
- BulletedList.cs
- WriteFileContext.cs
- XmlStreamStore.cs
- _SslStream.cs
- ChunkedMemoryStream.cs
- DeleteHelper.cs
- DrawingContextDrawingContextWalker.cs
- LinqDataSourceSelectEventArgs.cs
- ParagraphResult.cs
- Point4D.cs
- XmlCDATASection.cs
- Table.cs
- XmlSchemaComplexContentExtension.cs
- XPathDescendantIterator.cs
- ExtensionDataObject.cs
- XmlSchemaInferenceException.cs
- IdnElement.cs
- ScaleTransform.cs
- EncodingFallbackAwareXmlTextWriter.cs
- BasicViewGenerator.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- ObjectManager.cs
- NativeActivity.cs
- ComponentFactoryHelpers.cs
- DataSourceSelectArguments.cs
- Region.cs
- FixedSOMPageConstructor.cs
- RegexGroupCollection.cs
- UInt32Storage.cs
- ControlDesignerState.cs
- XmlAttributeHolder.cs