Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / NetworkInformation / SystemUnicastIPAddressInformation.cs / 1305376 / SystemUnicastIPAddressInformation.cs
////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; /// /// Provides support for ip configuation information and statistics. /// /// Specifies the unicast addresses for an interface. ///OS < XP ////// internal class SystemUnicastIPAddressInformation:UnicastIPAddressInformation { private IpAdapterUnicastAddress adapterAddress; private long dhcpLeaseLifetime; private SystemIPAddressInformation innerInfo; internal IPAddress ipv4Mask; private SystemUnicastIPAddressInformation() { } internal SystemUnicastIPAddressInformation(IpAdapterInfo ipAdapterInfo, IPExtendedAddress address){ innerInfo = new SystemIPAddressInformation(address.address); DateTime tempdate = new DateTime(1970,1,1); tempdate = tempdate.AddSeconds(ipAdapterInfo.leaseExpires); dhcpLeaseLifetime = (long)((tempdate - DateTime.UtcNow).TotalSeconds); ipv4Mask = address.mask; } internal SystemUnicastIPAddressInformation(IpAdapterUnicastAddress adapterAddress, IPAddress ipAddress){ innerInfo = new SystemIPAddressInformation(adapterAddress,ipAddress); this.adapterAddress = adapterAddress; dhcpLeaseLifetime = adapterAddress.leaseLifetime; } ///public override IPAddress Address{get {return innerInfo.Address;}} public override IPAddress IPv4Mask{ get { if(Address.AddressFamily != AddressFamily.InterNetwork){ return new IPAddress(0); } return ipv4Mask; } } /// /// The address is a cluster address and shouldn't be used by most applications. public override bool IsTransient{ get { return (innerInfo.IsTransient); } } ////// This address can be used for DNS. public override bool IsDnsEligible{ get { return (innerInfo.IsDnsEligible); } } ///public override PrefixOrigin PrefixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.prefixOrigin; } } /// public override SuffixOrigin SuffixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.suffixOrigin; } } /// /// IPv6 only. Specifies the duplicate address detection state. Only supported /// for IPv6. If called on an IPv4 address, will throw a "not supported" exception. public override DuplicateAddressDetectionState DuplicateAddressDetectionState{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.dadState; } } ////// Specifies the valid lifetime of the address in seconds. public override long AddressValidLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.validLifetime; } } ////// Specifies the prefered lifetime of the address in seconds. public override long AddressPreferredLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.preferredLifetime; } } ////// /// Specifies the prefered lifetime of the address in seconds. public override long DhcpLeaseLifetime{ get { return dhcpLeaseLifetime; } } //helper method that marshals the addressinformation into the classes internal static UnicastIPAddressInformationCollection ToAddressInformationCollection(IntPtr ptr) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. UnicastIPAddressInformationCollection addressList = new UnicastIPAddressInformationCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterUnicastAddress addr = (IpAdapterUnicastAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterUnicastAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(new SystemUnicastIPAddressInformation(addr,ep.Address)); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterUnicastAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterUnicastAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(new SystemUnicastIPAddressInformation(addr,ep.Address)); } return addressList; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. ////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; /// /// Provides support for ip configuation information and statistics. /// /// Specifies the unicast addresses for an interface. ///OS < XP ////// internal class SystemUnicastIPAddressInformation:UnicastIPAddressInformation { private IpAdapterUnicastAddress adapterAddress; private long dhcpLeaseLifetime; private SystemIPAddressInformation innerInfo; internal IPAddress ipv4Mask; private SystemUnicastIPAddressInformation() { } internal SystemUnicastIPAddressInformation(IpAdapterInfo ipAdapterInfo, IPExtendedAddress address){ innerInfo = new SystemIPAddressInformation(address.address); DateTime tempdate = new DateTime(1970,1,1); tempdate = tempdate.AddSeconds(ipAdapterInfo.leaseExpires); dhcpLeaseLifetime = (long)((tempdate - DateTime.UtcNow).TotalSeconds); ipv4Mask = address.mask; } internal SystemUnicastIPAddressInformation(IpAdapterUnicastAddress adapterAddress, IPAddress ipAddress){ innerInfo = new SystemIPAddressInformation(adapterAddress,ipAddress); this.adapterAddress = adapterAddress; dhcpLeaseLifetime = adapterAddress.leaseLifetime; } ///public override IPAddress Address{get {return innerInfo.Address;}} public override IPAddress IPv4Mask{ get { if(Address.AddressFamily != AddressFamily.InterNetwork){ return new IPAddress(0); } return ipv4Mask; } } /// /// The address is a cluster address and shouldn't be used by most applications. public override bool IsTransient{ get { return (innerInfo.IsTransient); } } ////// This address can be used for DNS. public override bool IsDnsEligible{ get { return (innerInfo.IsDnsEligible); } } ///public override PrefixOrigin PrefixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.prefixOrigin; } } /// public override SuffixOrigin SuffixOrigin{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.suffixOrigin; } } /// /// IPv6 only. Specifies the duplicate address detection state. Only supported /// for IPv6. If called on an IPv4 address, will throw a "not supported" exception. public override DuplicateAddressDetectionState DuplicateAddressDetectionState{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.dadState; } } ////// Specifies the valid lifetime of the address in seconds. public override long AddressValidLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.validLifetime; } } ////// Specifies the prefered lifetime of the address in seconds. public override long AddressPreferredLifetime{ get { if (! ComNetOS.IsPostWin2K) throw new PlatformNotSupportedException(SR.GetString(SR.WinXPRequired)); return adapterAddress.preferredLifetime; } } ////// /// Specifies the prefered lifetime of the address in seconds. public override long DhcpLeaseLifetime{ get { return dhcpLeaseLifetime; } } //helper method that marshals the addressinformation into the classes internal static UnicastIPAddressInformationCollection ToAddressInformationCollection(IntPtr ptr) { //we don't know the number of addresses up front, so we create an arraylist //to temporarily store them. UnicastIPAddressInformationCollection addressList = new UnicastIPAddressInformationCollection(); //if there is no address, just return; if (ptr == IntPtr.Zero) return addressList; //get the first address IpAdapterUnicastAddress addr = (IpAdapterUnicastAddress)Marshal.PtrToStructure(ptr,typeof(IpAdapterUnicastAddress)); //determine the address family used to create the IPAddress AddressFamily family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; SocketAddress sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //unfortunately, the only way to currently create an ipaddress is through IPEndPoint IPEndPoint ep; if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); //add the ipaddress to the arraylist addressList.InternalAdd(new SystemUnicastIPAddressInformation(addr,ep.Address)); //repeat for all of the addresses while ( addr.next != IntPtr.Zero ) { addr = (IpAdapterUnicastAddress)Marshal.PtrToStructure(addr.next,typeof(IpAdapterUnicastAddress)); //determine the address family used to create the IPAddress family = (addr.address.addressLength > 16)?AddressFamily.InterNetworkV6:AddressFamily.InterNetwork; sockAddress = new SocketAddress(family,(int)addr.address.addressLength); Marshal.Copy(addr.address.address,sockAddress.m_Buffer,0,addr.address.addressLength); //use the endpoint to create the ipaddress if (family == AddressFamily.InterNetwork ) ep = (IPEndPoint)IPEndPoint.Any.Create(sockAddress); else ep = (IPEndPoint)IPEndPoint.IPv6Any.Create(sockAddress); addressList.InternalAdd(new SystemUnicastIPAddressInformation(addr,ep.Address)); } return addressList; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
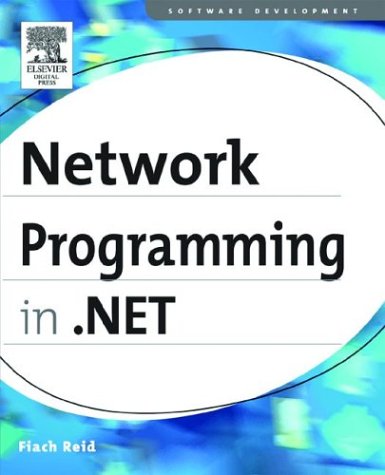
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegularExpressionValidator.cs
- ConfigXmlElement.cs
- QueryAccessibilityHelpEvent.cs
- ExeConfigurationFileMap.cs
- StatusBarDrawItemEvent.cs
- StreamInfo.cs
- updatecommandorderer.cs
- ToolboxComponentsCreatingEventArgs.cs
- StylusPointProperty.cs
- WindowsStartMenu.cs
- LayoutEvent.cs
- EntityKeyElement.cs
- RoleGroupCollectionEditor.cs
- IRCollection.cs
- AutomationPatternInfo.cs
- SmiMetaDataProperty.cs
- InstanceKeyCollisionException.cs
- HtmlFormWrapper.cs
- Style.cs
- ConfigurationElementProperty.cs
- Authorization.cs
- GroupStyle.cs
- GlyphsSerializer.cs
- EditBehavior.cs
- DBConcurrencyException.cs
- ContextBase.cs
- RemotingSurrogateSelector.cs
- ToolStripPanelRenderEventArgs.cs
- FileStream.cs
- CompositeFontFamily.cs
- RuntimeEnvironment.cs
- BuildTopDownAttribute.cs
- GroupBoxAutomationPeer.cs
- ModuleConfigurationInfo.cs
- NameTable.cs
- ContractHandle.cs
- _DynamicWinsockMethods.cs
- PageCache.cs
- DesignRelation.cs
- FontSourceCollection.cs
- UnmanagedBitmapWrapper.cs
- UnaryNode.cs
- ToolBarDesigner.cs
- HandleCollector.cs
- MetadataHelper.cs
- PresentationTraceSources.cs
- ProtectedProviderSettings.cs
- SelectedPathEditor.cs
- ReadOnlyCollectionBase.cs
- ArgIterator.cs
- ResourceManagerWrapper.cs
- DigitShape.cs
- SecurityProtocol.cs
- PersistenceTypeAttribute.cs
- HttpModuleAction.cs
- ImageClickEventArgs.cs
- SurrogateSelector.cs
- UInt64.cs
- BamlReader.cs
- StringBuilder.cs
- ObjectStateFormatter.cs
- WSMessageEncoding.cs
- DATA_BLOB.cs
- SamlSubject.cs
- TransformedBitmap.cs
- ValidatorAttribute.cs
- OciHandle.cs
- DataTransferEventArgs.cs
- NegotiateStream.cs
- TransactionManager.cs
- GeneralTransform3D.cs
- NamespaceList.cs
- ConnectionPointGlyph.cs
- CollectionType.cs
- TypefaceMap.cs
- CustomError.cs
- KeysConverter.cs
- EdmToObjectNamespaceMap.cs
- RoleBoolean.cs
- PanelDesigner.cs
- CodeVariableDeclarationStatement.cs
- recordstatefactory.cs
- NonNullItemCollection.cs
- MultiView.cs
- FreezableCollection.cs
- BamlLocalizableResource.cs
- BoundField.cs
- QuinticEase.cs
- _NetworkingPerfCounters.cs
- ProtocolsSection.cs
- GridViewColumnCollectionChangedEventArgs.cs
- ManipulationPivot.cs
- TargetException.cs
- TransportManager.cs
- Util.cs
- Simplifier.cs
- HtmlFormWrapper.cs
- TreeViewBindingsEditor.cs
- DtrList.cs
- CapabilitiesState.cs