Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Util.cs / 1 / Util.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Diagnostics; ////// static utility function /// internal static class Util { ///forward slash char array for triming uris internal static readonly char[] ForwardSlash = new char[1] { '/' }; ////// static char[] for indenting whitespace when tracing xml /// private static char[] whitespaceForTracing = new char[] { '\r', '\n', ' ', ' ', ' ', ' ', ' ' }; ////// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncreferenceIdentity = delegate(Uri identity) { return identity; }; /// /// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncdereferenceIdentity = delegate(Uri identity) { return identity; }; /// /// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// internal static FuncReferenceIdentity { get { return referenceIdentity; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Justification = "Pending")] set { referenceIdentity = value; } } /// /// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// internal static FuncDereferenceIdentity { get { return dereferenceIdentity; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Justification = "Pending")] set { dereferenceIdentity = value; } } /// /// Checks the argument value for null and throw ArgumentNullException if it is null /// ///type of the argument to prevent accidental boxing of value types /// argument whose value needs to be checked /// name of the argument ///if value is null ///value internal static T CheckArgumentNull(T value, string parameterName) where T : class { if (null == value) { throw Error.ArgumentNull(parameterName); } return value; } /// /// Checks the string value is not empty /// /// value to check /// parameterName of public function ///if value is null ///if value is empty internal static void CheckArgumentNotEmpty(string value, string parameterName) { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyString, parameterName); } } ////// Checks the array value is not empty /// ///type of the argument to prevent accidental boxing of value types /// value to check /// parameterName of public function ///if value is null ///if value is empty or contains null elements internal static void CheckArgumentNotEmpty(T[] value, string parameterName) where T : class { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyArray, parameterName); } for (int i = 0; i < value.Length; ++i) { if (Object.ReferenceEquals(value[i], null)) { throw Error.Argument(Strings.Util_NullArrayElement, parameterName); } } } /// /// Validate MergeOption /// /// option to validate /// name of the parameter being validated ///if option is not valid ///option internal static MergeOption CheckEnumerationValue(MergeOption value, string parameterName) { switch (value) { case MergeOption.AppendOnly: case MergeOption.OverwriteChanges: case MergeOption.PreserveChanges: case MergeOption.NoTracking: return value; default: throw Error.ArgumentOutOfRange(parameterName); } } ////// get char[] for indenting whitespace when tracing xml /// /// how many characters to trace ///char[] internal static char[] GetWhitespaceForTracing(int depth) { char[] whitespace = Util.whitespaceForTracing; while (whitespace.Length <= depth) { char[] tmp = new char[2 * whitespace.Length]; tmp[0] = '\r'; tmp[1] = '\n'; for (int i = 2; i < tmp.Length; ++i) { tmp[i] = ' '; } System.Threading.Interlocked.CompareExchange(ref Util.whitespaceForTracing, tmp, whitespace); whitespace = tmp; } return whitespace; } ///new Uri(string uriString, UriKind uriKind) /// value /// kind ///new Uri(value, kind) internal static Uri CreateUri(string value, UriKind kind) { Uri result = new Uri(value, kind); return result; } ///new Uri(Uri baseUri, Uri requestUri) /// baseUri /// relativeUri ///new Uri(baseUri, requestUri) internal static Uri CreateUri(Uri baseUri, Uri requestUri) { Debug.Assert((null != baseUri) && baseUri.IsAbsoluteUri, "baseUri !IsAbsoluteUri"); Debug.Assert(String.IsNullOrEmpty(baseUri.Query) && String.IsNullOrEmpty(baseUri.Fragment), "baseUri has query or fragment"); Util.CheckArgumentNull(requestUri, "requestUri"); // there is a bug in (new Uri(Uri,Uri)) which corrupts the port of the result if out relativeUri is also absolute if (!requestUri.IsAbsoluteUri) { if (baseUri.OriginalString.EndsWith("/", StringComparison.Ordinal)) { if (requestUri.OriginalString.StartsWith("/", StringComparison.Ordinal)) { requestUri = new Uri(baseUri, Util.CreateUri(requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Relative)); } else { requestUri = new Uri(baseUri, requestUri); } } else { requestUri = Util.CreateUri(baseUri.OriginalString + "/" + requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Absolute); } } // consider: just use the orignal requestUri if it was is absolute and not care about the baseUri // always verify IsBaseOf after making absolute to deal with ".." relative Uri if (!UriUtil.UriInvariantInsensitiveIsBaseOf(baseUri, requestUri)) { throw Error.Argument(Strings.Context_ServiceRootNotBaseUri, "requestUri"); } return requestUri; } ////// does the array contain the value reference /// ///generic type /// array to search /// value being looked for ///true if value reference was found in array internal static bool ContainsReference(T[] array, T value) where T : class { return (0 <= IndexOfReference (array, value)); } /// dispose of the object and set the reference to null ///type that implements IDisposable /// object to dispose internal static void Dispose(ref T disposable) where T : class, IDisposable { Dispose(disposable); disposable = null; } /// dispose of the object ///type that implements IDisposable /// object to dispose internal static void Dispose(T disposable) where T : class, IDisposable { if (null != disposable) { disposable.Dispose(); } } /// /// index of value reference in the array /// ///generic type /// array to search /// value being looked for ///index of value reference in the array else (-1) internal static int IndexOfReference(T[] array, T value) where T : class { Debug.Assert(null != array, "null array"); for (int i = 0; i < array.Length; ++i) { if (object.ReferenceEquals(array[i], value)) { return i; } } return -1; } /// Checks whether the exception should not be handled. /// exception to test ///true if the exception should not be handled internal static bool DoNotHandleException(Exception ex) { return ((null != ex) && ((ex is System.StackOverflowException) || (ex is System.OutOfMemoryException) || (ex is System.Threading.ThreadAbortException))); } ///validate value is non-null ///type of value /// value /// error code to throw if null ///the non-null value internal static T NullCheck(T value, InternalError errorcode) where T : class { if (Object.ReferenceEquals(value, null)) { Error.ThrowInternalError(errorcode); } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// static utility functions // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Diagnostics; ////// static utility function /// internal static class Util { ///forward slash char array for triming uris internal static readonly char[] ForwardSlash = new char[1] { '/' }; ////// static char[] for indenting whitespace when tracing xml /// private static char[] whitespaceForTracing = new char[] { '\r', '\n', ' ', ' ', ' ', ' ', ' ' }; ////// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncreferenceIdentity = delegate(Uri identity) { return identity; }; /// /// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// private static FuncdereferenceIdentity = delegate(Uri identity) { return identity; }; /// /// ReferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// internal static FuncReferenceIdentity { get { return referenceIdentity; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Justification = "Pending")] set { referenceIdentity = value; } } /// /// DereferenceIdentity is a test hook to help verify we dont' use identity instead of editLink /// internal static FuncDereferenceIdentity { get { return dereferenceIdentity; } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Justification = "Pending")] set { dereferenceIdentity = value; } } /// /// Checks the argument value for null and throw ArgumentNullException if it is null /// ///type of the argument to prevent accidental boxing of value types /// argument whose value needs to be checked /// name of the argument ///if value is null ///value internal static T CheckArgumentNull(T value, string parameterName) where T : class { if (null == value) { throw Error.ArgumentNull(parameterName); } return value; } /// /// Checks the string value is not empty /// /// value to check /// parameterName of public function ///if value is null ///if value is empty internal static void CheckArgumentNotEmpty(string value, string parameterName) { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyString, parameterName); } } ////// Checks the array value is not empty /// ///type of the argument to prevent accidental boxing of value types /// value to check /// parameterName of public function ///if value is null ///if value is empty or contains null elements internal static void CheckArgumentNotEmpty(T[] value, string parameterName) where T : class { CheckArgumentNull(value, parameterName); if (0 == value.Length) { throw Error.Argument(Strings.Util_EmptyArray, parameterName); } for (int i = 0; i < value.Length; ++i) { if (Object.ReferenceEquals(value[i], null)) { throw Error.Argument(Strings.Util_NullArrayElement, parameterName); } } } /// /// Validate MergeOption /// /// option to validate /// name of the parameter being validated ///if option is not valid ///option internal static MergeOption CheckEnumerationValue(MergeOption value, string parameterName) { switch (value) { case MergeOption.AppendOnly: case MergeOption.OverwriteChanges: case MergeOption.PreserveChanges: case MergeOption.NoTracking: return value; default: throw Error.ArgumentOutOfRange(parameterName); } } ////// get char[] for indenting whitespace when tracing xml /// /// how many characters to trace ///char[] internal static char[] GetWhitespaceForTracing(int depth) { char[] whitespace = Util.whitespaceForTracing; while (whitespace.Length <= depth) { char[] tmp = new char[2 * whitespace.Length]; tmp[0] = '\r'; tmp[1] = '\n'; for (int i = 2; i < tmp.Length; ++i) { tmp[i] = ' '; } System.Threading.Interlocked.CompareExchange(ref Util.whitespaceForTracing, tmp, whitespace); whitespace = tmp; } return whitespace; } ///new Uri(string uriString, UriKind uriKind) /// value /// kind ///new Uri(value, kind) internal static Uri CreateUri(string value, UriKind kind) { Uri result = new Uri(value, kind); return result; } ///new Uri(Uri baseUri, Uri requestUri) /// baseUri /// relativeUri ///new Uri(baseUri, requestUri) internal static Uri CreateUri(Uri baseUri, Uri requestUri) { Debug.Assert((null != baseUri) && baseUri.IsAbsoluteUri, "baseUri !IsAbsoluteUri"); Debug.Assert(String.IsNullOrEmpty(baseUri.Query) && String.IsNullOrEmpty(baseUri.Fragment), "baseUri has query or fragment"); Util.CheckArgumentNull(requestUri, "requestUri"); // there is a bug in (new Uri(Uri,Uri)) which corrupts the port of the result if out relativeUri is also absolute if (!requestUri.IsAbsoluteUri) { if (baseUri.OriginalString.EndsWith("/", StringComparison.Ordinal)) { if (requestUri.OriginalString.StartsWith("/", StringComparison.Ordinal)) { requestUri = new Uri(baseUri, Util.CreateUri(requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Relative)); } else { requestUri = new Uri(baseUri, requestUri); } } else { requestUri = Util.CreateUri(baseUri.OriginalString + "/" + requestUri.OriginalString.TrimStart(Util.ForwardSlash), UriKind.Absolute); } } // consider: just use the orignal requestUri if it was is absolute and not care about the baseUri // always verify IsBaseOf after making absolute to deal with ".." relative Uri if (!UriUtil.UriInvariantInsensitiveIsBaseOf(baseUri, requestUri)) { throw Error.Argument(Strings.Context_ServiceRootNotBaseUri, "requestUri"); } return requestUri; } ////// does the array contain the value reference /// ///generic type /// array to search /// value being looked for ///true if value reference was found in array internal static bool ContainsReference(T[] array, T value) where T : class { return (0 <= IndexOfReference (array, value)); } /// dispose of the object and set the reference to null ///type that implements IDisposable /// object to dispose internal static void Dispose(ref T disposable) where T : class, IDisposable { Dispose(disposable); disposable = null; } /// dispose of the object ///type that implements IDisposable /// object to dispose internal static void Dispose(T disposable) where T : class, IDisposable { if (null != disposable) { disposable.Dispose(); } } /// /// index of value reference in the array /// ///generic type /// array to search /// value being looked for ///index of value reference in the array else (-1) internal static int IndexOfReference(T[] array, T value) where T : class { Debug.Assert(null != array, "null array"); for (int i = 0; i < array.Length; ++i) { if (object.ReferenceEquals(array[i], value)) { return i; } } return -1; } /// Checks whether the exception should not be handled. /// exception to test ///true if the exception should not be handled internal static bool DoNotHandleException(Exception ex) { return ((null != ex) && ((ex is System.StackOverflowException) || (ex is System.OutOfMemoryException) || (ex is System.Threading.ThreadAbortException))); } ///validate value is non-null ///type of value /// value /// error code to throw if null ///the non-null value internal static T NullCheck(T value, InternalError errorcode) where T : class { if (Object.ReferenceEquals(value, null)) { Error.ThrowInternalError(errorcode); } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
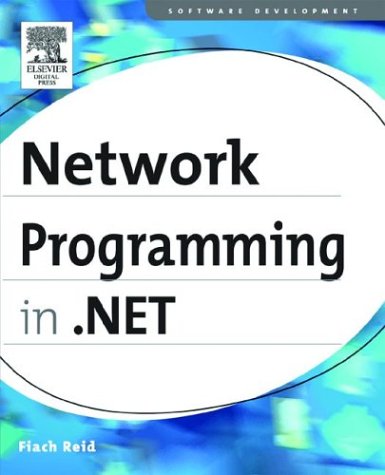
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AmbientValueAttribute.cs
- Types.cs
- DetailsViewDeleteEventArgs.cs
- ResourceDescriptionAttribute.cs
- SqlClientFactory.cs
- BinaryKeyIdentifierClause.cs
- ResourcePool.cs
- OrElse.cs
- WindowsToolbarAsMenu.cs
- ACL.cs
- SequenceNumber.cs
- MultiTouchSystemGestureLogic.cs
- LocalizableResourceBuilder.cs
- GACIdentityPermission.cs
- MatrixTransform3D.cs
- Int16KeyFrameCollection.cs
- ClassImporter.cs
- ResumeStoryboard.cs
- HwndAppCommandInputProvider.cs
- KeyGesture.cs
- FunctionQuery.cs
- XmlCharCheckingReader.cs
- RIPEMD160Managed.cs
- SlipBehavior.cs
- LoginUtil.cs
- XomlCompilerError.cs
- HttpServerVarsCollection.cs
- ParallelTimeline.cs
- DCSafeHandle.cs
- HostingEnvironmentSection.cs
- CryptoHandle.cs
- MinimizableAttributeTypeConverter.cs
- DrawingBrush.cs
- TabControl.cs
- PropertyTabAttribute.cs
- EpmContentSerializerBase.cs
- MultitargetingHelpers.cs
- FloaterBaseParaClient.cs
- ByteStack.cs
- DataGridViewSelectedCellCollection.cs
- ResourceDisplayNameAttribute.cs
- CellIdBoolean.cs
- WebServiceReceiveDesigner.cs
- ValidationResult.cs
- IntSecurity.cs
- DispatchOperationRuntime.cs
- DataException.cs
- BitVec.cs
- ProcessModelSection.cs
- ToolStripSettings.cs
- SchemaElement.cs
- GradientStopCollection.cs
- ToolBar.cs
- ToolStripDropDownMenu.cs
- ProcessHostServerConfig.cs
- Operators.cs
- ObjectDataSourceMethodEventArgs.cs
- ControlsConfig.cs
- PathSegmentCollection.cs
- EtwProvider.cs
- BitmapVisualManager.cs
- DoubleIndependentAnimationStorage.cs
- PackagePartCollection.cs
- TerminatorSinks.cs
- RadioButtonBaseAdapter.cs
- NameTable.cs
- Hex.cs
- ImageUrlEditor.cs
- RangeBaseAutomationPeer.cs
- DebugInfoGenerator.cs
- ImageMetadata.cs
- XamlGridLengthSerializer.cs
- altserialization.cs
- DbProviderConfigurationHandler.cs
- DBProviderConfigurationHandler.cs
- ConfigurationElement.cs
- RecommendedAsConfigurableAttribute.cs
- SigningDialog.cs
- WebBrowserPermission.cs
- XmlSchemaSimpleContentExtension.cs
- DependencySource.cs
- MsdtcWrapper.cs
- ExtenderControl.cs
- Maps.cs
- BooleanAnimationBase.cs
- WindowsIPAddress.cs
- VisualBrush.cs
- CryptoHelper.cs
- WCFModelStrings.Designer.cs
- ToolStripItemCollection.cs
- BitmapSource.cs
- SmiContextFactory.cs
- DtrList.cs
- HasCopySemanticsAttribute.cs
- CodePrimitiveExpression.cs
- HttpNamespaceReservationInstallComponent.cs
- GeometryCollection.cs
- DesignerForm.cs
- RawMouseInputReport.cs
- ServiceDiscoveryElement.cs