Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / BinaryMessageFormatter.cs / 1305376 / BinaryMessageFormatter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging { using System; using System.Runtime.Serialization; using System.Runtime.Serialization.Formatters; using System.Runtime.Serialization.Formatters.Binary; using System.Diagnostics; using System.IO; using System.ComponentModel; ////// /// Formatter class that serializes and deserializes objects into /// and from MessageQueue messages using binary format. /// public class BinaryMessageFormatter : IMessageFormatter { private BinaryFormatter formatter; internal const short VT_BINARY_OBJECT = 0x300; ////// /// Creates a new Binary message formatter object. /// public BinaryMessageFormatter() { this.formatter = new BinaryFormatter(); } ////// /// Creates a new Binary message formatter object /// with the given properties. /// public BinaryMessageFormatter(FormatterAssemblyStyle topObjectFormat, FormatterTypeStyle typeFormat) { this.formatter = new BinaryFormatter(); this.formatter.AssemblyFormat = topObjectFormat; this.formatter.TypeFormat = typeFormat; } ////// /// Determines how the top (root) object of a graph /// is laid out in the serialized stream. /// [MessagingDescription(Res.MsgTopObjectFormat), DefaultValueAttribute(FormatterAssemblyStyle.Simple)] public FormatterAssemblyStyle TopObjectFormat { get { return this.formatter.AssemblyFormat; } set { this.formatter.AssemblyFormat = value; } } ////// /// Determines how type descriptions are laid out in the /// serialized stream. /// [MessagingDescription(Res.MsgTypeFormat), DefaultValueAttribute(FormatterTypeStyle.TypesWhenNeeded)] public FormatterTypeStyle TypeFormat { get { return this.formatter.TypeFormat; } set { this.formatter.TypeFormat = value; } } ////// /// public bool CanRead(Message message) { if (message == null) throw new ArgumentNullException("message"); int variantType = message.BodyType; if (variantType != VT_BINARY_OBJECT) return false; return true; } ///When this method is called, the formatter will attempt to determine /// if the contents of the message are something the formatter can deal with. ////// /// This method is needed to improve scalability on Receive and ReceiveAsync scenarios. Not requiring /// thread safety on read and write. /// public object Clone() { return new BinaryMessageFormatter(TopObjectFormat, TypeFormat); } ////// /// This method is used to read the contents from the given message /// and create an object. /// public object Read(Message message) { if (message == null) throw new ArgumentNullException("message"); int variantType = message.BodyType; if (variantType == VT_BINARY_OBJECT) { Stream stream = message.BodyStream; return formatter.Deserialize(stream); } throw new InvalidOperationException(Res.GetString(Res.InvalidTypeDeserialization)); } ////// /// This method is used to write the given object into the given message. /// If the formatter cannot understand the given object, an exception is thrown. /// public void Write(Message message, object obj) { if (message == null) throw new ArgumentNullException("message"); Stream stream = new MemoryStream(); formatter.Serialize(stream, obj); message.BodyType = VT_BINARY_OBJECT; message.BodyStream = stream; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
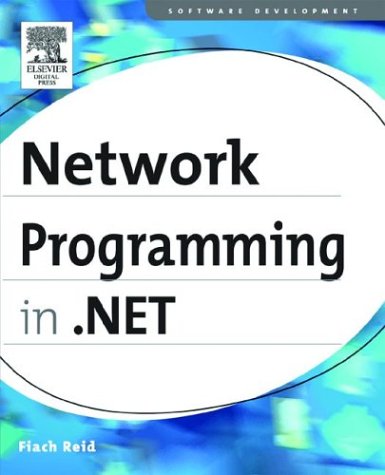
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScaleTransform.cs
- SemanticValue.cs
- BulletedListEventArgs.cs
- WebBrowserBase.cs
- SecurityToken.cs
- CodeEventReferenceExpression.cs
- DataListItemEventArgs.cs
- ResponseStream.cs
- FixedDSBuilder.cs
- HtmlElementErrorEventArgs.cs
- LicenseProviderAttribute.cs
- SQLDecimalStorage.cs
- TabControlEvent.cs
- GradientStop.cs
- SystemIPv4InterfaceProperties.cs
- InkCanvasInnerCanvas.cs
- ExtenderControl.cs
- CodeTypeReference.cs
- BrowserCapabilitiesFactory.cs
- DoubleAnimationUsingPath.cs
- XmlReturnReader.cs
- DataGridViewCellMouseEventArgs.cs
- PointAnimationBase.cs
- HtmlMeta.cs
- InternalBufferOverflowException.cs
- XmlNodeReader.cs
- Transform.cs
- ObjectViewEntityCollectionData.cs
- ValidatorUtils.cs
- GenerateHelper.cs
- SymbolPair.cs
- GestureRecognitionResult.cs
- Int32AnimationBase.cs
- ICspAsymmetricAlgorithm.cs
- FontSizeConverter.cs
- InvokeWebService.cs
- PeerPresenceInfo.cs
- login.cs
- PopupRootAutomationPeer.cs
- Roles.cs
- PrintingPermissionAttribute.cs
- ServiceDescriptions.cs
- SoapSchemaExporter.cs
- NodeInfo.cs
- WebHttpBindingElement.cs
- RepeaterItemEventArgs.cs
- ClientCredentials.cs
- DataGridViewCellStyleChangedEventArgs.cs
- ZoneLinkButton.cs
- TableStyle.cs
- ModuleBuilder.cs
- ApplicationId.cs
- DateTimeAutomationPeer.cs
- DataListCommandEventArgs.cs
- HasCopySemanticsAttribute.cs
- FileIOPermission.cs
- ItemChangedEventArgs.cs
- ProgressBarAutomationPeer.cs
- XmlBaseReader.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- ToolStripOverflow.cs
- CachedPathData.cs
- FrameDimension.cs
- InvalidCastException.cs
- BaseDataBoundControl.cs
- BitmapEffectRenderDataResource.cs
- SourceItem.cs
- SqlConnectionPoolProviderInfo.cs
- Journal.cs
- PageVisual.cs
- TimersDescriptionAttribute.cs
- DbTransaction.cs
- DataServiceQueryException.cs
- AdornerHitTestResult.cs
- TimeSpanOrInfiniteConverter.cs
- XmlBindingWorker.cs
- SafeRightsManagementPubHandle.cs
- WebPartUtil.cs
- DataBinding.cs
- WindowInteropHelper.cs
- InternalControlCollection.cs
- NativeObjectSecurity.cs
- BridgeDataRecord.cs
- FileSystemWatcher.cs
- ListInitExpression.cs
- OdbcDataAdapter.cs
- DependencyObject.cs
- PixelFormat.cs
- FocusTracker.cs
- XsltOutput.cs
- EventEntry.cs
- CheckoutException.cs
- AuthorizationRuleCollection.cs
- BoundColumn.cs
- GregorianCalendar.cs
- BinaryNode.cs
- DropDownHolder.cs
- GetIndexBinder.cs
- C14NUtil.cs
- RowType.cs