Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Design / Converters / FontNameConverter.cs / 1305376 / FontNameConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.MobileControls.Converters { using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Globalization; [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] internal sealed class FontNameConverter : TypeConverter { private StandardValuesCollection values; ////// Creates a new font name converter. /// public FontNameConverter() { // Sink an event to let us know when the installed // set of fonts changes. // SystemEvents.InstalledFontsChanged += new EventHandler(this.OnInstalledFontsChanged); } ////// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { return MatchFontName((string)value, context); } return base.ConvertFrom(context, culture, value); } ////// We need to know when we're finalized. /// ~FontNameConverter() { SystemEvents.InstalledFontsChanged -= new EventHandler(this.OnInstalledFontsChanged); } ////// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { FontFamily[] fonts = FontFamily.Families; Hashtable hash = new Hashtable(); for (int i = 0; i < fonts.Length; i++) { string name = fonts[i].Name; hash[name.ToLower(CultureInfo.InvariantCulture)] = name; } object[] array = new object[hash.Values.Count]; hash.Values.CopyTo(array, 0); Array.Sort(array); values = new StandardValuesCollection(array); } return values; } ////// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } private string MatchFontName(string name, ITypeDescriptorContext context) { Debug.Assert(name != null, "Expected an actual font name to match in FontNameConverter::MatchFontName."); // AUI 2300 if (name.Trim().Length == 0) { return String.Empty; } // Try a partial match // string bestMatch = null; IEnumerator e = GetStandardValues(context).GetEnumerator(); while (e.MoveNext()) { string fontName = e.Current.ToString(); if (String.Equals(fontName, name, StringComparison.OrdinalIgnoreCase)) { // For an exact match, return immediately // return fontName; } else if (fontName.StartsWith(name, StringComparison.OrdinalIgnoreCase)) { if (bestMatch == null || fontName.Length <= bestMatch.Length) { bestMatch = fontName; } } } if (bestMatch == null) { // no match... fall back on whatever was provided bestMatch = name; } return bestMatch; } ////// Called by system events when someone adds or removes a font. Here /// we invalidate our font name collection. /// private void OnInstalledFontsChanged(object sender, EventArgs e) { values = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.MobileControls.Converters { using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Globalization; [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] internal sealed class FontNameConverter : TypeConverter { private StandardValuesCollection values; ////// Creates a new font name converter. /// public FontNameConverter() { // Sink an event to let us know when the installed // set of fonts changes. // SystemEvents.InstalledFontsChanged += new EventHandler(this.OnInstalledFontsChanged); } ////// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Converts the given object to the converter's native type. /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value is string) { return MatchFontName((string)value, context); } return base.ConvertFrom(context, culture, value); } ////// We need to know when we're finalized. /// ~FontNameConverter() { SystemEvents.InstalledFontsChanged -= new EventHandler(this.OnInstalledFontsChanged); } ////// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (values == null) { FontFamily[] fonts = FontFamily.Families; Hashtable hash = new Hashtable(); for (int i = 0; i < fonts.Length; i++) { string name = fonts[i].Name; hash[name.ToLower(CultureInfo.InvariantCulture)] = name; } object[] array = new object[hash.Values.Count]; hash.Values.CopyTo(array, 0); Array.Sort(array); values = new StandardValuesCollection(array); } return values; } ////// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } private string MatchFontName(string name, ITypeDescriptorContext context) { Debug.Assert(name != null, "Expected an actual font name to match in FontNameConverter::MatchFontName."); // AUI 2300 if (name.Trim().Length == 0) { return String.Empty; } // Try a partial match // string bestMatch = null; IEnumerator e = GetStandardValues(context).GetEnumerator(); while (e.MoveNext()) { string fontName = e.Current.ToString(); if (String.Equals(fontName, name, StringComparison.OrdinalIgnoreCase)) { // For an exact match, return immediately // return fontName; } else if (fontName.StartsWith(name, StringComparison.OrdinalIgnoreCase)) { if (bestMatch == null || fontName.Length <= bestMatch.Length) { bestMatch = fontName; } } } if (bestMatch == null) { // no match... fall back on whatever was provided bestMatch = name; } return bestMatch; } ////// Called by system events when someone adds or removes a font. Here /// we invalidate our font name collection. /// private void OnInstalledFontsChanged(object sender, EventArgs e) { values = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
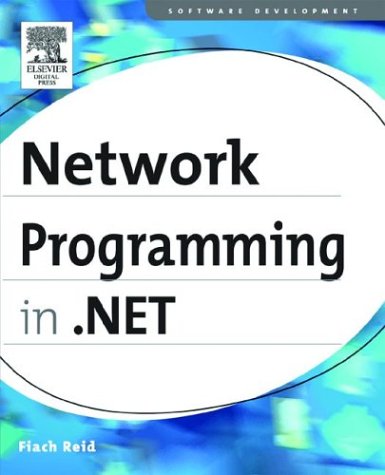
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PreviewPrintController.cs
- ObservableCollection.cs
- ListenerUnsafeNativeMethods.cs
- ResponseBodyWriter.cs
- LightweightEntityWrapper.cs
- RuntimeResourceSet.cs
- LinkedResource.cs
- _SpnDictionary.cs
- Add.cs
- ComponentSerializationService.cs
- BulletedListEventArgs.cs
- ExtensionSimplifierMarkupObject.cs
- CssClassPropertyAttribute.cs
- IconConverter.cs
- SystemTcpStatistics.cs
- ThreadStartException.cs
- GregorianCalendar.cs
- CopyOfAction.cs
- SHA512Managed.cs
- Constraint.cs
- PeerPresenceInfo.cs
- ApplicationInfo.cs
- Transform3D.cs
- InstanceKey.cs
- StringStorage.cs
- SiteMapPath.cs
- EntityDataSourceView.cs
- ConstructorArgumentAttribute.cs
- TextRunTypographyProperties.cs
- DeferrableContent.cs
- Version.cs
- DataBindingCollection.cs
- IndexExpression.cs
- Win32.cs
- CategoryAttribute.cs
- ColorComboBox.cs
- ExcCanonicalXml.cs
- XmlUTF8TextWriter.cs
- ExtentCqlBlock.cs
- RenderData.cs
- PartialList.cs
- DistributedTransactionPermission.cs
- DataProtection.cs
- HostedAspNetEnvironment.cs
- SnapLine.cs
- ListDictionary.cs
- QilInvoke.cs
- SqlUnionizer.cs
- SiteMapDataSource.cs
- WindowsListViewItemCheckBox.cs
- StoreUtilities.cs
- BaseResourcesBuildProvider.cs
- PropertiesTab.cs
- WebPartManager.cs
- IdnMapping.cs
- EmptyEnumerator.cs
- UshortList2.cs
- Menu.cs
- IdentityElement.cs
- ErrorWebPart.cs
- OracleDateTime.cs
- InputLanguageCollection.cs
- ToolboxItemAttribute.cs
- SecurityChannelListener.cs
- BamlResourceSerializer.cs
- DataObject.cs
- DataControlFieldTypeEditor.cs
- RegexRunner.cs
- ModulesEntry.cs
- SchemaHelper.cs
- ValidationHelper.cs
- DefaultHttpHandler.cs
- DeviceFilterDictionary.cs
- DrawingCollection.cs
- RelationshipNavigation.cs
- SystemInfo.cs
- CustomAttribute.cs
- Root.cs
- SafeTimerHandle.cs
- DiscardableAttribute.cs
- Vector3DAnimationUsingKeyFrames.cs
- ComboBoxHelper.cs
- DockAndAnchorLayout.cs
- ConvertBinder.cs
- Query.cs
- DataPagerCommandEventArgs.cs
- FrameSecurityDescriptor.cs
- ThemeDirectoryCompiler.cs
- ExpressionLexer.cs
- MultipartContentParser.cs
- AttributeQuery.cs
- Message.cs
- LocationReference.cs
- ICollection.cs
- ScriptHandlerFactory.cs
- EventDescriptorCollection.cs
- UIElementParagraph.cs
- SecurityHelper.cs
- HttpModulesSection.cs
- RadialGradientBrush.cs