Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities.DurableInstancing / System / Activities / DurableInstancing / LoadRetryHandler.cs / 1305376 / LoadRetryHandler.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.DurableInstancing { using System; using System.Collections.Generic; using System.Runtime; using System.Runtime.DurableInstancing; sealed class LoadRetryHandler { BinaryHeapretryQueue; object syncLock; IOThreadTimer retryThreadTimer; public LoadRetryHandler() { this.retryQueue = new BinaryHeap (); this.syncLock = new object(); this.retryThreadTimer = new IOThreadTimer(new Action
Link Menu
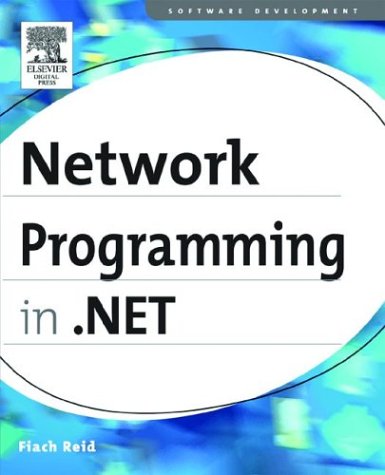
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StringBuilder.cs
- FileDialogPermission.cs
- VerificationAttribute.cs
- StoreAnnotationsMap.cs
- WindowsProgressbar.cs
- ConfigurationManagerHelper.cs
- Substitution.cs
- StateManagedCollection.cs
- SymbolPair.cs
- ArraySegment.cs
- Ipv6Element.cs
- MatrixCamera.cs
- XPathEmptyIterator.cs
- DesignerHelpers.cs
- OptimisticConcurrencyException.cs
- TextRange.cs
- GetWorkflowTree.cs
- EmissiveMaterial.cs
- StaticContext.cs
- AppSettingsReader.cs
- TimeSpanValidator.cs
- HttpCapabilitiesSectionHandler.cs
- DocumentScope.cs
- SecurityAlgorithmSuiteConverter.cs
- SchemaInfo.cs
- XmlLanguage.cs
- SystemIPv4InterfaceProperties.cs
- Event.cs
- EntityDataSourceSelectingEventArgs.cs
- TextEffect.cs
- SrgsText.cs
- XamlReader.cs
- TranslateTransform3D.cs
- _DisconnectOverlappedAsyncResult.cs
- QilFactory.cs
- CodeCompileUnit.cs
- StringCollection.cs
- Vector3D.cs
- CheckBoxAutomationPeer.cs
- TraceXPathNavigator.cs
- SqlTopReducer.cs
- DataGridViewCellEventArgs.cs
- HttpCapabilitiesSectionHandler.cs
- EventMap.cs
- Hashtable.cs
- LowerCaseStringConverter.cs
- PagesSection.cs
- HostedBindingBehavior.cs
- hresults.cs
- EdmItemCollection.OcAssemblyCache.cs
- DataSourceConverter.cs
- SoapException.cs
- BaseValidator.cs
- DrawingContext.cs
- Stroke.cs
- FunctionNode.cs
- DoubleLinkListEnumerator.cs
- RichTextBox.cs
- IgnoreFileBuildProvider.cs
- FieldReference.cs
- TreePrinter.cs
- Properties.cs
- SerializationHelper.cs
- HtmlInputButton.cs
- TypeBuilderInstantiation.cs
- HostedTransportConfigurationManager.cs
- Permission.cs
- KeyInfo.cs
- SetStoryboardSpeedRatio.cs
- StreamUpdate.cs
- ListMarkerLine.cs
- HwndHostAutomationPeer.cs
- DataListCommandEventArgs.cs
- GlobalItem.cs
- PKCS1MaskGenerationMethod.cs
- FormsAuthenticationModule.cs
- DbParameterHelper.cs
- SmtpFailedRecipientsException.cs
- WindowPattern.cs
- DataGridItemEventArgs.cs
- PassportAuthentication.cs
- InternalConfigSettingsFactory.cs
- FilterQuery.cs
- X509CertificateTokenFactoryCredential.cs
- URL.cs
- ProcessHostConfigUtils.cs
- UserControl.cs
- UnauthorizedWebPart.cs
- CommonGetThemePartSize.cs
- MessageSecurityOverTcp.cs
- backend.cs
- InfoCardArgumentException.cs
- HtmlEncodedRawTextWriter.cs
- SqlFacetAttribute.cs
- LineBreak.cs
- PrintPreviewDialog.cs
- sqlpipe.cs
- ApplicationGesture.cs
- OracleFactory.cs
- UrlPropertyAttribute.cs