Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / NetworkInformation / SystemIPv4InterfaceProperties.cs / 1 / SystemIPv4InterfaceProperties.cs
////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; internal class SystemIPv4InterfaceProperties:IPv4InterfaceProperties{ //these are only valid for ipv4 interfaces bool haveWins = false; bool dhcpEnabled = false; bool routingEnabled = false; bool autoConfigEnabled = false; bool autoConfigActive = false; uint index = 0; uint mtu = 0; //ipv4 addresses only GatewayIPAddressInformationCollection gatewayAddresses = null; IPAddressCollection dhcpAddresses = null; IPAddressCollection winsServerAddresses = null; internal IPAddressCollection dnsAddresses = null; /* // Consider removing. internal SystemIPv4InterfaceProperties(){ } */ internal SystemIPv4InterfaceProperties(FixedInfo fixedInfo, IpAdapterInfo ipAdapterInfo) { index = ipAdapterInfo.index; routingEnabled = fixedInfo.EnableRouting; dhcpEnabled = ipAdapterInfo.dhcpEnabled; haveWins = ipAdapterInfo.haveWins; gatewayAddresses = ipAdapterInfo.gatewayList.ToIPGatewayAddressCollection(); dhcpAddresses = ipAdapterInfo.dhcpServer.ToIPAddressCollection(); IPAddressCollection primaryWinsServerAddresses = ipAdapterInfo.primaryWinsServer.ToIPAddressCollection(); IPAddressCollection secondaryWinsServerAddresses = ipAdapterInfo.secondaryWinsServer.ToIPAddressCollection(); //concat the winsserver addresses winsServerAddresses = new IPAddressCollection(); foreach (IPAddress address in primaryWinsServerAddresses){ winsServerAddresses.InternalAdd(address); } foreach (IPAddress address in secondaryWinsServerAddresses){ winsServerAddresses.InternalAdd(address); } SystemIPv4InterfaceStatistics s = new SystemIPv4InterfaceStatistics(index); mtu = (uint)s.Mtu; if(ComNetOS.IsWin2K){ GetPerAdapterInfo(ipAdapterInfo.index); } else{ dnsAddresses = fixedInfo.DnsAddresses; } } internal IPAddressCollection DnsAddresses{ get { return dnsAddresses; } } /// /// Provides support for ip configuation information and statistics. /// Only valid for Ipv4 Uses WINS for name resolution. public override bool UsesWins{get {return haveWins;}} public override bool IsDhcpEnabled{ get { return dhcpEnabled; } } public override bool IsForwardingEnabled{get {return routingEnabled;}} //proto ///Auto configuration of an ipv4 address for a client /// on a network where a DHCP server /// isn't available. public override bool IsAutomaticPrivateAddressingEnabled{ get{ return autoConfigEnabled; } } // proto public override bool IsAutomaticPrivateAddressingActive{ get{ return autoConfigActive; } } ///Specifies the Maximum transmission unit in bytes. Uses GetIFEntry. //We cache this to be consistent across all platforms public override int Mtu{ get { return (int) mtu; } } public override int Index{ get { return (int) index; } } ///IP Address of the default gateway. internal GatewayIPAddressInformationCollection GetGatewayAddresses(){ return gatewayAddresses; } ///IP address of the DHCP sever. internal IPAddressCollection GetDhcpServerAddresses(){ return dhcpAddresses; } ///IP addresses of the WINS servers. internal IPAddressCollection GetWinsServersAddresses(){ return winsServerAddresses; } private void GetPerAdapterInfo(uint index) { if (index != 0){ uint size = 0; SafeLocalFree buffer = null; uint result = UnsafeNetInfoNativeMethods.GetPerAdapterInfo(index,SafeLocalFree.Zero,ref size); while (result == IpHelperErrors.ErrorBufferOverflow) { try { //now we allocate the buffer and read the network parameters. buffer = SafeLocalFree.LocalAlloc((int)size); result = UnsafeNetInfoNativeMethods.GetPerAdapterInfo(index,buffer,ref size); if ( result == IpHelperErrors.Success ) { IpPerAdapterInfo ipPerAdapterInfo = (IpPerAdapterInfo)Marshal.PtrToStructure(buffer.DangerousGetHandle(),typeof(IpPerAdapterInfo)); autoConfigEnabled = ipPerAdapterInfo.autoconfigEnabled; autoConfigActive = ipPerAdapterInfo.autoconfigActive; //get dnsAddresses dnsAddresses = ipPerAdapterInfo.dnsServerList.ToIPAddressCollection(); } } finally { if(dnsAddresses == null){ dnsAddresses = new IPAddressCollection(); } if (buffer != null) buffer.Close(); } } if(dnsAddresses == null){ dnsAddresses = new IPAddressCollection(); } if (result != IpHelperErrors.Success) { throw new NetworkInformationException((int)result); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. ////// namespace System.Net.NetworkInformation { using System.Net; using System.Net.Sockets; using System; using System.Runtime.InteropServices; using System.Collections; using System.ComponentModel; using System.Security.Permissions; using Microsoft.Win32; internal class SystemIPv4InterfaceProperties:IPv4InterfaceProperties{ //these are only valid for ipv4 interfaces bool haveWins = false; bool dhcpEnabled = false; bool routingEnabled = false; bool autoConfigEnabled = false; bool autoConfigActive = false; uint index = 0; uint mtu = 0; //ipv4 addresses only GatewayIPAddressInformationCollection gatewayAddresses = null; IPAddressCollection dhcpAddresses = null; IPAddressCollection winsServerAddresses = null; internal IPAddressCollection dnsAddresses = null; /* // Consider removing. internal SystemIPv4InterfaceProperties(){ } */ internal SystemIPv4InterfaceProperties(FixedInfo fixedInfo, IpAdapterInfo ipAdapterInfo) { index = ipAdapterInfo.index; routingEnabled = fixedInfo.EnableRouting; dhcpEnabled = ipAdapterInfo.dhcpEnabled; haveWins = ipAdapterInfo.haveWins; gatewayAddresses = ipAdapterInfo.gatewayList.ToIPGatewayAddressCollection(); dhcpAddresses = ipAdapterInfo.dhcpServer.ToIPAddressCollection(); IPAddressCollection primaryWinsServerAddresses = ipAdapterInfo.primaryWinsServer.ToIPAddressCollection(); IPAddressCollection secondaryWinsServerAddresses = ipAdapterInfo.secondaryWinsServer.ToIPAddressCollection(); //concat the winsserver addresses winsServerAddresses = new IPAddressCollection(); foreach (IPAddress address in primaryWinsServerAddresses){ winsServerAddresses.InternalAdd(address); } foreach (IPAddress address in secondaryWinsServerAddresses){ winsServerAddresses.InternalAdd(address); } SystemIPv4InterfaceStatistics s = new SystemIPv4InterfaceStatistics(index); mtu = (uint)s.Mtu; if(ComNetOS.IsWin2K){ GetPerAdapterInfo(ipAdapterInfo.index); } else{ dnsAddresses = fixedInfo.DnsAddresses; } } internal IPAddressCollection DnsAddresses{ get { return dnsAddresses; } } /// /// Provides support for ip configuation information and statistics. /// Only valid for Ipv4 Uses WINS for name resolution. public override bool UsesWins{get {return haveWins;}} public override bool IsDhcpEnabled{ get { return dhcpEnabled; } } public override bool IsForwardingEnabled{get {return routingEnabled;}} //proto ///Auto configuration of an ipv4 address for a client /// on a network where a DHCP server /// isn't available. public override bool IsAutomaticPrivateAddressingEnabled{ get{ return autoConfigEnabled; } } // proto public override bool IsAutomaticPrivateAddressingActive{ get{ return autoConfigActive; } } ///Specifies the Maximum transmission unit in bytes. Uses GetIFEntry. //We cache this to be consistent across all platforms public override int Mtu{ get { return (int) mtu; } } public override int Index{ get { return (int) index; } } ///IP Address of the default gateway. internal GatewayIPAddressInformationCollection GetGatewayAddresses(){ return gatewayAddresses; } ///IP address of the DHCP sever. internal IPAddressCollection GetDhcpServerAddresses(){ return dhcpAddresses; } ///IP addresses of the WINS servers. internal IPAddressCollection GetWinsServersAddresses(){ return winsServerAddresses; } private void GetPerAdapterInfo(uint index) { if (index != 0){ uint size = 0; SafeLocalFree buffer = null; uint result = UnsafeNetInfoNativeMethods.GetPerAdapterInfo(index,SafeLocalFree.Zero,ref size); while (result == IpHelperErrors.ErrorBufferOverflow) { try { //now we allocate the buffer and read the network parameters. buffer = SafeLocalFree.LocalAlloc((int)size); result = UnsafeNetInfoNativeMethods.GetPerAdapterInfo(index,buffer,ref size); if ( result == IpHelperErrors.Success ) { IpPerAdapterInfo ipPerAdapterInfo = (IpPerAdapterInfo)Marshal.PtrToStructure(buffer.DangerousGetHandle(),typeof(IpPerAdapterInfo)); autoConfigEnabled = ipPerAdapterInfo.autoconfigEnabled; autoConfigActive = ipPerAdapterInfo.autoconfigActive; //get dnsAddresses dnsAddresses = ipPerAdapterInfo.dnsServerList.ToIPAddressCollection(); } } finally { if(dnsAddresses == null){ dnsAddresses = new IPAddressCollection(); } if (buffer != null) buffer.Close(); } } if(dnsAddresses == null){ dnsAddresses = new IPAddressCollection(); } if (result != IpHelperErrors.Success) { throw new NetworkInformationException((int)result); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
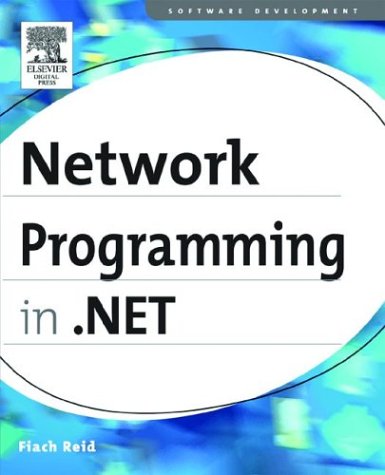
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnitControl.cs
- ToolStripTextBox.cs
- _NestedMultipleAsyncResult.cs
- _IPv6Address.cs
- TextBoxLine.cs
- ADRoleFactory.cs
- CollectionViewGroup.cs
- SimpleRecyclingCache.cs
- FontStyle.cs
- AudioFormatConverter.cs
- XPathSelfQuery.cs
- FixedFlowMap.cs
- UriTemplateTable.cs
- Int16Animation.cs
- XmlSchemaElement.cs
- ResourceReferenceExpressionConverter.cs
- ItemsChangedEventArgs.cs
- DrawingDrawingContext.cs
- HTMLTextWriter.cs
- InfoCardSymmetricAlgorithm.cs
- WebPartConnectionsDisconnectVerb.cs
- TypeUtils.cs
- RequestCache.cs
- NetStream.cs
- CommandManager.cs
- SuppressMessageAttribute.cs
- baseshape.cs
- __Filters.cs
- ProfileInfo.cs
- TerminateDesigner.cs
- MULTI_QI.cs
- SiteMapSection.cs
- WindowsSecurityToken.cs
- ImageCreator.cs
- ToolStripItemTextRenderEventArgs.cs
- ReceiveReply.cs
- XmlWriterTraceListener.cs
- ServerValidateEventArgs.cs
- ControlBuilder.cs
- OracleException.cs
- _SslState.cs
- PropertyToken.cs
- IUnknownConstantAttribute.cs
- SendMessageChannelCache.cs
- XAMLParseException.cs
- _ScatterGatherBuffers.cs
- DiscoveryInnerClientAdhoc11.cs
- BasicViewGenerator.cs
- BaseHashHelper.cs
- IProvider.cs
- Compilation.cs
- OleDbWrapper.cs
- RenderTargetBitmap.cs
- compensatingcollection.cs
- EntityParameterCollection.cs
- ProcessHostConfigUtils.cs
- DataGridViewToolTip.cs
- CodeParameterDeclarationExpressionCollection.cs
- RecommendedAsConfigurableAttribute.cs
- RelationshipEndMember.cs
- CallbackHandler.cs
- HatchBrush.cs
- ColumnPropertiesGroup.cs
- BinaryUtilClasses.cs
- BinaryFormatterWriter.cs
- IIS7WorkerRequest.cs
- StructuralType.cs
- UniqueConstraint.cs
- ConfigXmlCDataSection.cs
- RootProjectionNode.cs
- rsa.cs
- FilterEventArgs.cs
- XmlSchemaExporter.cs
- RegistrationServices.cs
- JoinTreeNode.cs
- dsa.cs
- XMLSyntaxException.cs
- LoadRetryHandler.cs
- RuntimeHelpers.cs
- MatrixStack.cs
- BitmapEffectInput.cs
- CodeMethodReturnStatement.cs
- XmlDataImplementation.cs
- TargetParameterCountException.cs
- ClientTargetCollection.cs
- VSDExceptions.cs
- LabelDesigner.cs
- SafeNativeMethodsMilCoreApi.cs
- TileModeValidation.cs
- Int32RectValueSerializer.cs
- DropShadowBitmapEffect.cs
- EastAsianLunisolarCalendar.cs
- WorkflowInlining.cs
- CurrentChangingEventManager.cs
- Variant.cs
- WebPartZone.cs
- OrderByBuilder.cs
- DiscardableAttribute.cs
- PartialCachingAttribute.cs
- SuppressIldasmAttribute.cs